C++ 定时器的实现
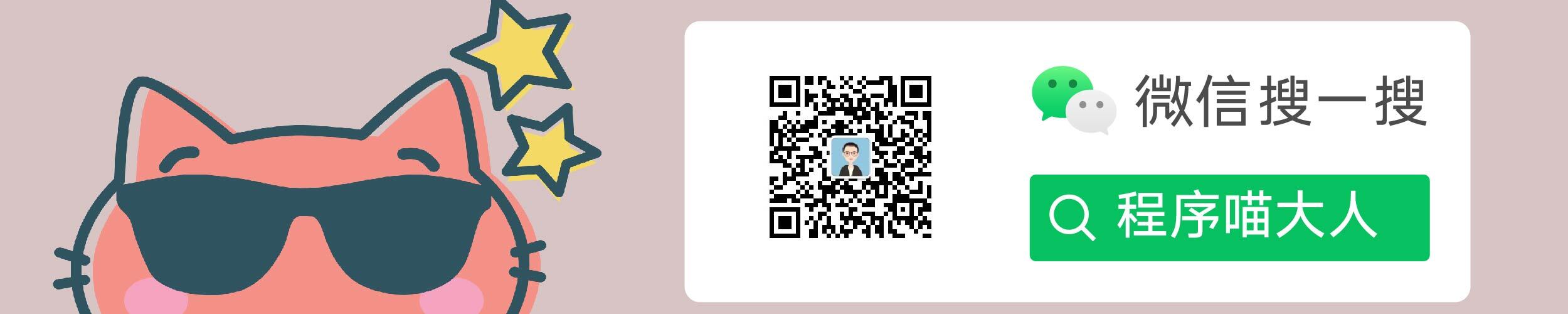
在上一篇文章里,我分享了关于C++线程池的实现的内容。今天,我们来讲下C++定时器的实现。
个人认为一个完备的定时器需要有如下功能:
在某一时间点执行某一任务
在某段时间后执行某一任务
重复执行某一任务N次,任务间隔时间T
那么如何实现定时器呢?下面是我自己实现的定时器逻辑,源码链接最后会附上。
定时器中主要的数据结构
优先级任务队列:队列中存储任务,每个任务会添加时间戳,最近的时间戳的任务会先出队。
锁和条件变量:当有任务需要执行时,用于通知正在等待的线程从任务队列中取出任务执行。
线程池:各个任务会放在线程池中执行。
下面是相关代码:
初始化
在构造函数中初始化,主要是配置好内部的线程池,线程池中常驻的线程数目前设为4。关于线程池部分可以参考https://www.jianshu.com/p/41b7a1013907
如何开启定时器功能?
打开内部的线程池功能,用于执行放入定时器中的任务,同时新开一个线程,循环等待任务到来后送入线程池中执行。
如何关闭定时器功能?
这里是使用running_标志位控制,标志位为false,调度线程的循环就会自动退出,就不会继续等待任务执行。
如何在某一时间点执行任务?
根据时间戳构造InternalS,放入队列中
如何在某段时间后执行任务?
根据当前时间加上时间段构造出时间戳从而构造InternalS,放入队列中
如何循环执行任务?
首先为这个循环任务生成标识ID,外部可以通过ID来取消此任务继续执行,代码如下,内部以类似递归的方式循环执行任务。
如何取消循环任务的执行?
定时器内部有repeated_id_state_map数据结构,用于存储循环任务的ID,当取消任务执行时,将此ID从repeated_id_state_map中移除,循环任务就会自动取消。
简单的测试代码
完整代码
完整代码可在github中查看。https://github.com/fightingwangzq/wzq_utils/blob/master/timer/include/timer/timer.h
版权声明: 本文为 InfoQ 作者【程序喵大人】的原创文章。
原文链接:【http://xie.infoq.cn/article/f64199976dffc7687f00ec090】。文章转载请联系作者。
评论