面试官问:Spring Boot 中 Tomcat 是怎么启动的
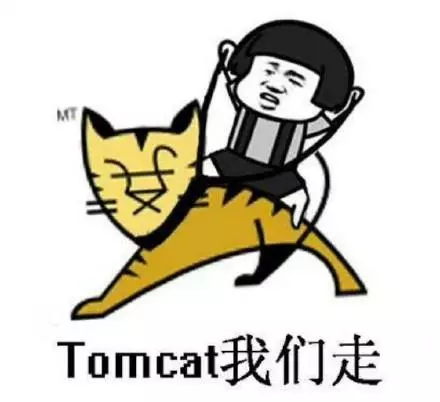
来源 | cnblogs.com/54chensongxia/p/13294470.html
Spring Boot一个非常突出的优点就是不需要我们额外再部署Servlet容器,它内置了多种容器的支持。我们可以通过配置来指定我们需要的容器。
本文以我们平时最常使用的容器Tomcat为列来介绍以下两个知识点:
Spring Boot是怎么整合启动Tomcat容器的;
在Spring Boot中,怎么进行Tomcat的深度配置。
Spring Boot整合启动Tomcat的流程
对于看源代码,每个人都有自己的方法。我自己在看源代码的时候喜欢结合IDEA的Debug功能一起看。比如说现在我们要研究Spring Boot是在哪个环节点启动Tomcat的,我的思路是:Tomcat在启动时会调用各个组件的init方法和start方法,那么我只需要在这些方法上打上端点,然后就能在调用栈上看出Spring Boot是在哪个环节点启用Tomcat的了。
按照这个思路,我在Tomcat的Connector组件的init方法上打了端点,通过调用栈能很清楚的看出Spring Boot是在容器的onRefresh方法中调用Tomcat的。
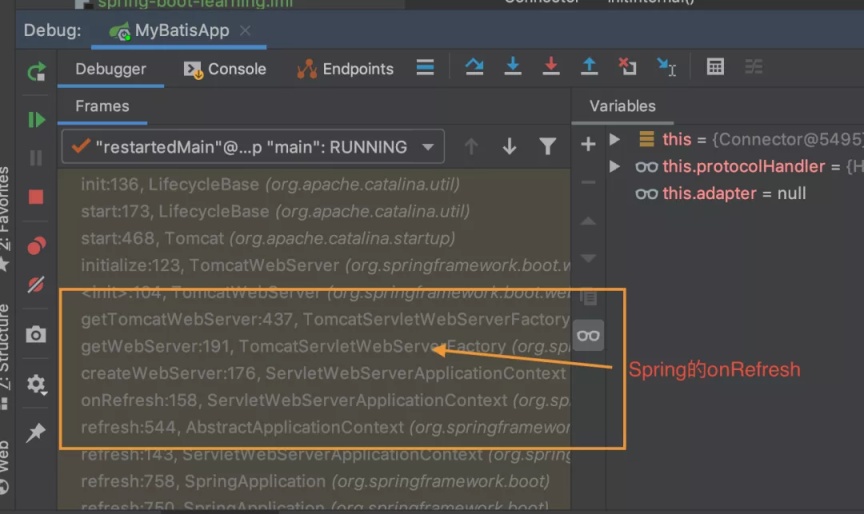
找到了调用点,那么一切都好办了。从上面的方法中可以看出,重点内容就在this.createWebServer()这个方法中。
在Spring Boot中使用的容器类是ServletWebServerApplicationContext系列的容器,这个系列的容器可以内嵌Web容器。这个我们可以从这个容器的属性和方法中可以看出来。
下面是TomcatServletWebServerFactory的getWebServer方法。
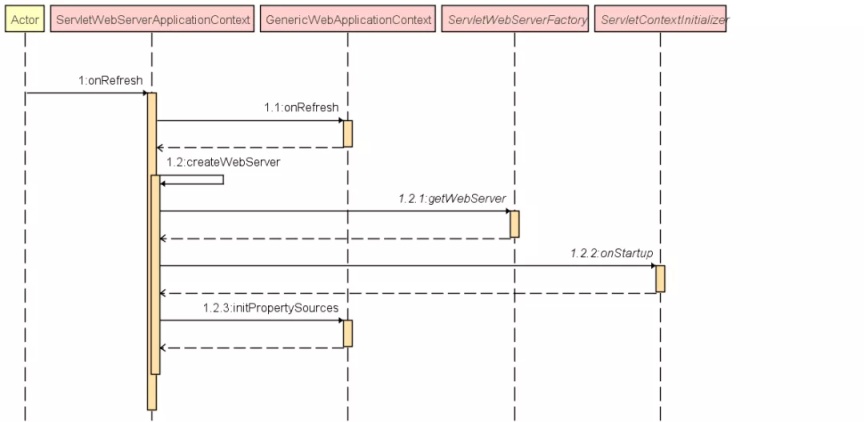
至此Spring Boot内嵌的Tomcat已将顺序启动了。那么Spring Boot是在什么时候注册DispatchServlet的呢?
配置Listener、Filter和Servlet
Spring Boot配置Listener、Filter和Servlet可以參考我之前写的文章Spring Boot使用嵌入式容器,那怎么配置自定义Filter呢
推荐使用ServletListenerRegistrationBean、FilterRegistrationBean和ServletRegistrationBean的方式注册Listener、Filter和Servlet。
Spring Boot注册DispatcherServlet
在传统的Spring MVC项目中,我们都会在web.xml中注册DispatcherServlet这个入口类,那么在Spring Boot中是在哪里注册的呢?
大家如果看Spring Boot的源代码,这边有个小技巧大家可以参考下。就是Spring Boot把之前传统项目中的配置项都通过AutoConfig的形式做配置了。所以这边在寻找DispatcherServlet是在哪里配置的也可以顺着这个思路去寻找。在IDEA的类查找功能中输入DispatcherServlet关键字,我们能看到一个DispatcherServletAutoConfiguration类。从名字上就能看出这个类是DispatcherServlet的自动配置类,我们点进去看下是否是在这个类内部注册的DispatcherServlet?
好了通过这边的介绍,我们直到DispatcherServlet是通过DispatcherServletAutoConfiguration这个自动配置类注册的。
Spring Boot中关于Tomcat的一些其他配置
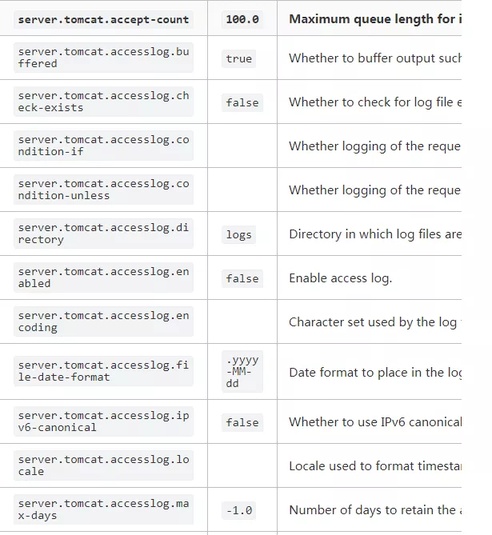
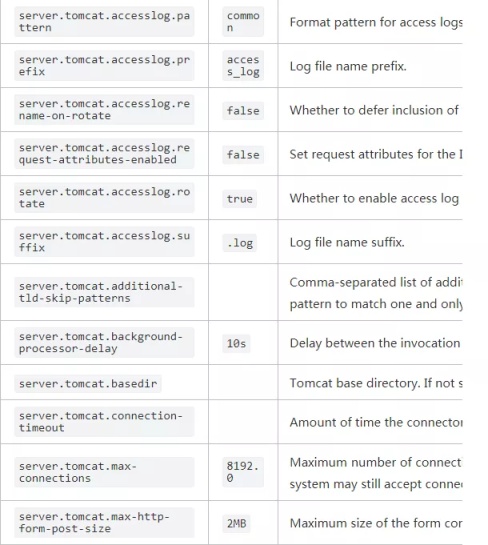
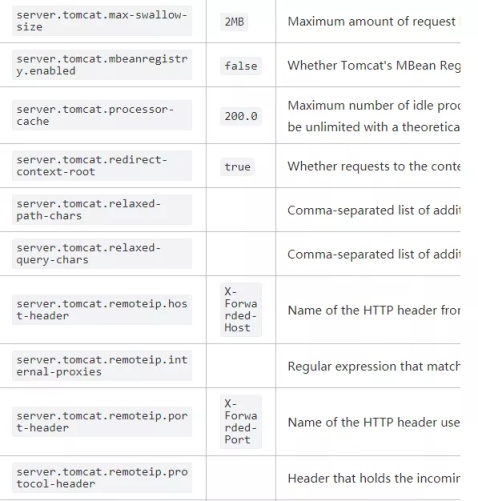

server:
port: ${port:9999}
tomcat:
accept-count: 200
#最好进行这段配置,默认会在tmp目录下创建,Linux有时会有定时任务删除tmp目录下的内容
basedir: my-tomcat
accesslog:
enabled: true
pattern: '%t %a "%r" %s %S (%b M) (%D ms)'
max-http-post-size: 2MB
max-swallow-size: 2M
uri-encoding: GBK
threads:
max: 100
min-spare: 10
具体使用时可以参考Spring Boo官网关于Tomcat的配置。
一些其他类
Spring Boot还提供了很多自定义类,让用户对Tomcat的组件做自定义配置。这个符合Spring的设计哲学:只提供选择,而不是强制用户使用某项技术。
关于Tomcat的自定义配置类还有以下几个,大家可以按需使用。
WebServerFactoryCustomizer接口:自定义Web容易工厂
WebServerFactoryCustomizerBeanPostProcessor处理类:WebServerFactoryCustomizer类通过WebServerFactoryCustomizerBeanPostProcessor类生效
TomcatConnectorCustomizer:连接器自定义处理类
TomcatContextCustomizer:Context自定义接口
评论