【week03】作业 1
.net版本单例模式
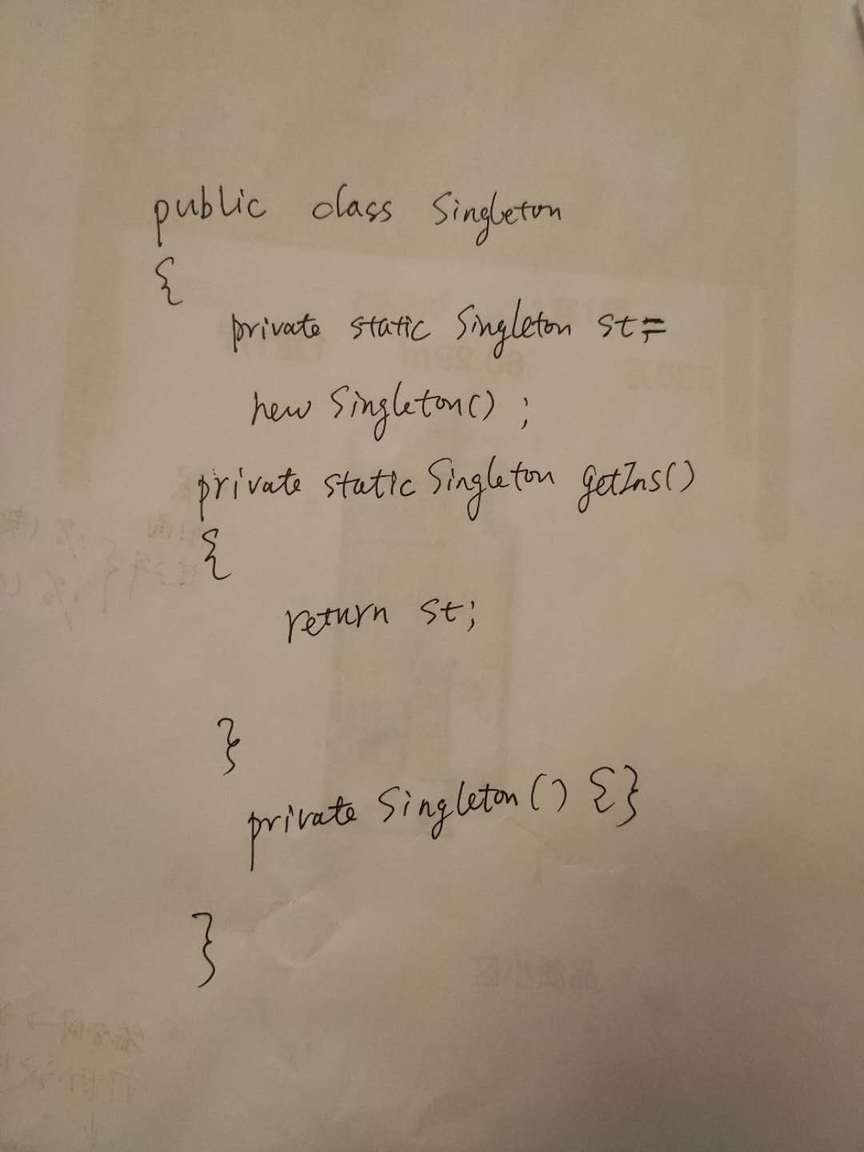
作业2
public interface IControl
{
void Print();
string ControlName { get; set; }
}
public abstract class BaseControl : IControl, IEnumerable<BaseControl>
{
private List<BaseControl> Children = new List<BaseControl>();
public BaseControl(string controlName)
{
this.ControlName = controlName;
}
public void AddChild(BaseControl child)
{
this.Children.Add(child);
}
IEnumerator<BaseControl> IEnumerable<BaseControl>.GetEnumerator()
{
return this.Children.GetEnumerator();
}
System.Collections.IEnumerator System.Collections.IEnumerable.GetEnumerator()
{
return this.Children.GetEnumerator();
}
public virtual void Print()
{
this.PrintSelf();
foreach (var child in this.Children)
{
child.Print();
}
}
protected abstract void PrintSelf();
private string name;
public string ControlName
{
get
{
return this.name;
}
set
{
this.name = value;
}
}
}
public class WinForm : BaseControl
{
public WinForm(string name) :
base(name)
{ }
protected override void PrintSelf()
{
Console.WriteLine("Print WinForm({0})", this.ControlName);
}
}
public class Picture : BaseControl
{
public Picture(string name) :
base(name)
{ }
protected override void PrintSelf()
{
Console.WriteLine("Print Picture({0})", this.ControlName);
}
}
public class Button: BaseControl
{
public Button(string name) :
base(name)
{ }
protected override void PrintSelf()
{
Console.WriteLine("Print Button({0})", this.ControlName);
}
}
public class Frame : BaseControl
{
public Frame(string name) :
base(name)
{ }
protected override void PrintSelf()
{
Console.WriteLine("Print Frame({0})", this.ControlName);
}
}
public class Label : BaseControl
{
public Label(string name) :
base(name)
{ }
protected override void PrintSelf()
{
Console.WriteLine("Print Label({0})", this.ControlName);
}
}
public class TextBox : BaseControl
{
public TextBox(string name) :
base(name)
{ }
protected override void PrintSelf()
{
Console.WriteLine("Print TextBox({0})", this.ControlName);
}
}
public class Password : BaseControl
{
public Password(string name) :
base(name)
{ }
protected override void PrintSelf()
{
Console.WriteLine("Print Password({0})", this.ControlName);
}
}
public class CheckBox : BaseControl
{
public CheckBox(string name) :
base(name)
{ }
protected override void PrintSelf()
{
Console.WriteLine("Print CheckBox({0})", this.ControlName);
}
}
public class LinkLabel : BaseControl
{
public LinkLabel(string name) :
base(name)
{ }
protected override void PrintSelf()
{
Console.WriteLine("Print LinkLabel({0})", this.ControlName);
}
}
class Program
{
static void Main(string[] args)
{
WinForm form = new WinForm("WINDOW窗口");
form.AddChild(new Picture("LOGO图片"));
form.AddChild(new Button("登录"));
form.AddChild(new Button("注册"));
Frame f = new Frame("FRAME1");
form.AddChild(f);
f.AddChild(new Label("用户名"));
f.AddChild(new TextBox("文本框"));
f.AddChild(new Label("密码"));
f.AddChild(new Password("密码框"));
f.AddChild(new CheckBox("复选框"));
f.AddChild(new Label("记住用户名"));
f.AddChild(new LinkLabel("忘记密码"));
form.Print();
Console.Read();
}
}
评论