Ribbon 使用及其内核原理剖析
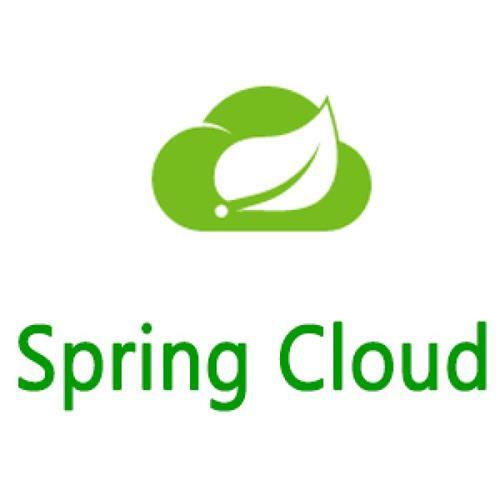
1.什么是Ribbon
Spring Cloud Ribbon是 基于Netflix Ribbon 实现的一套客户端的负载均衡工具,Ribbon客户端组件提供一系列的完善的配置,如超时,重试等。通过Load Balancer(LB)获取到服务提供的所有机器实例,Ribbon会自动基于某种规则(轮询,随机)去调用这些服务。Ribbon也可以实现我们自己的负载均衡算法。
1.1 什么是客户端的负载均衡
进程内的LB,他是一个类库集成到消费端,通过消费端进行获取提供者的地址。
生活中: 类似与你去火车站排队进站(有三条通道),只要是正常人,都会排队到人少的队伍中去。
程序中: 我们消费端 能获取到服务提供者地址列表,然后根据某种策略去获取一个地址进行调用。
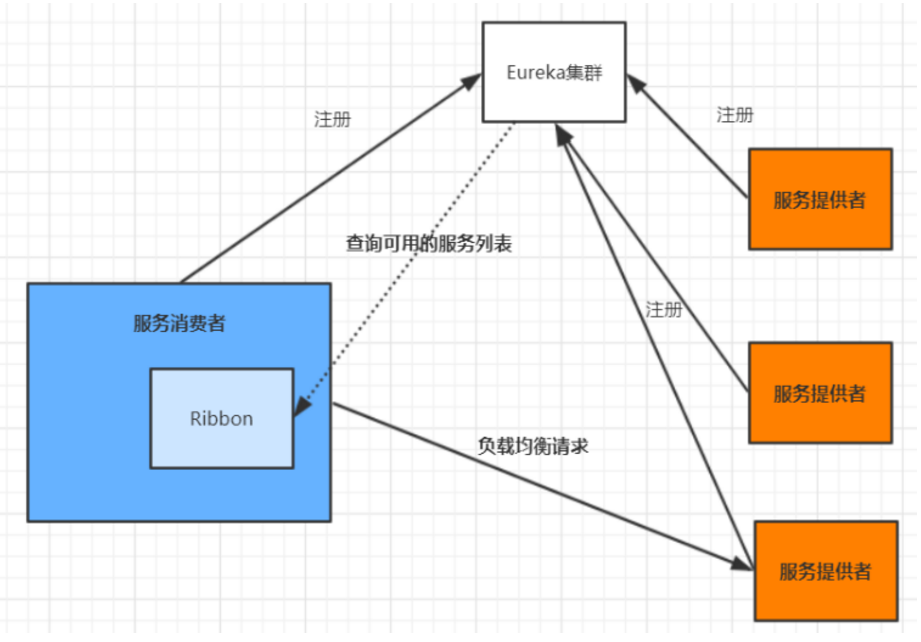
1.2 什么是服务端的负载均衡
生活中:类似与你去火车站排队进站的时候,有一个火车站的引导员告诉你说三号通道人少,你去三号通道排队。
程序中:就是你消费者调用的是ng的地址,由ng来选择 请求的转发(轮询 权重,ip_hash等)
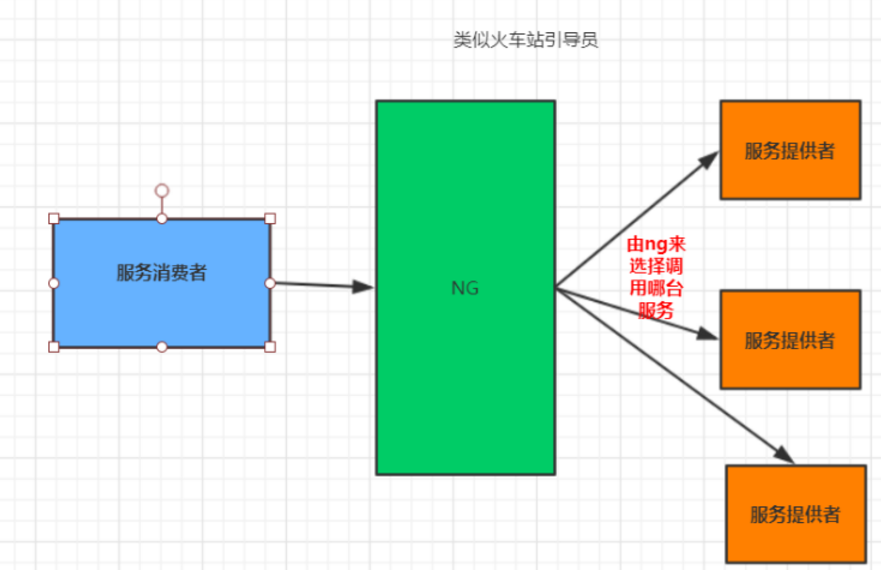
2. 快速整合Ribbon
引入依赖
添加@LoadBalanced注解
3. Ribbon内核原理
3.1 Ribbon调用流程
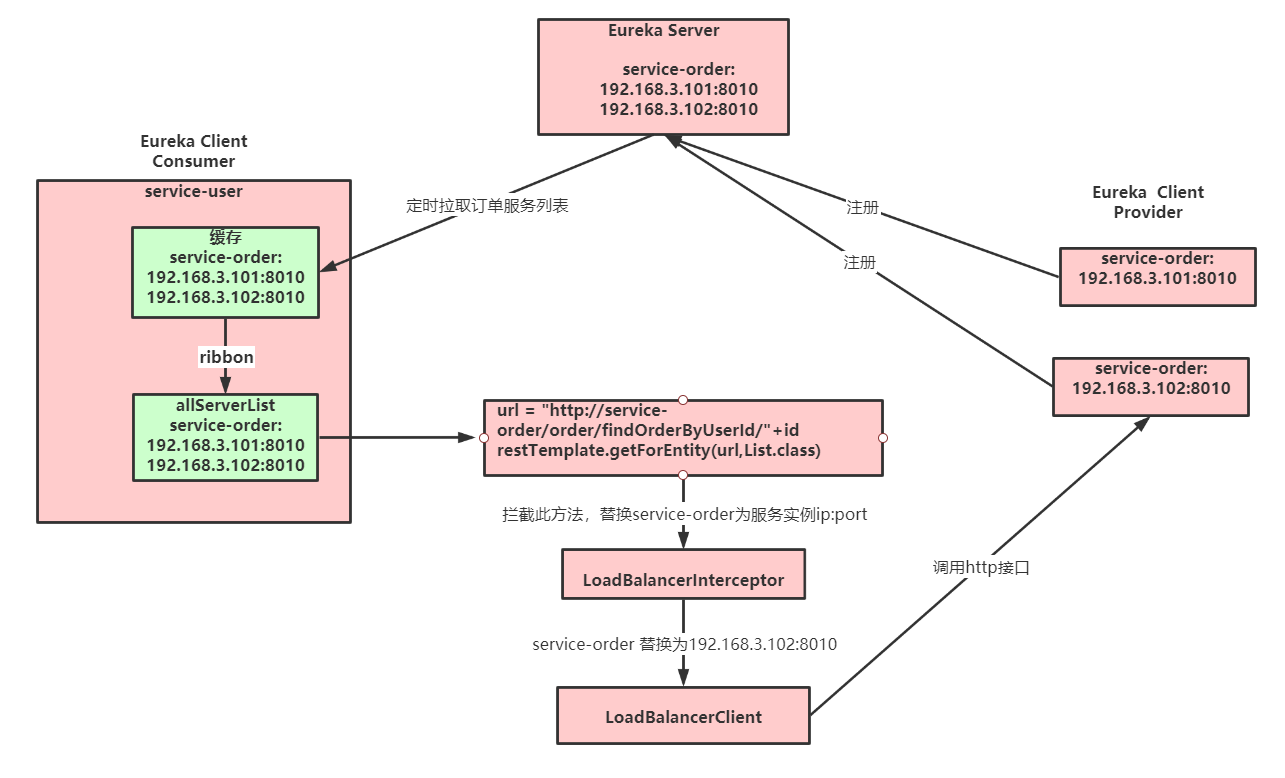
参考图:https://www.processon.com/view/link/5e7466dce4b027d999bdaddb
3.2 Ribbon负载均衡策略
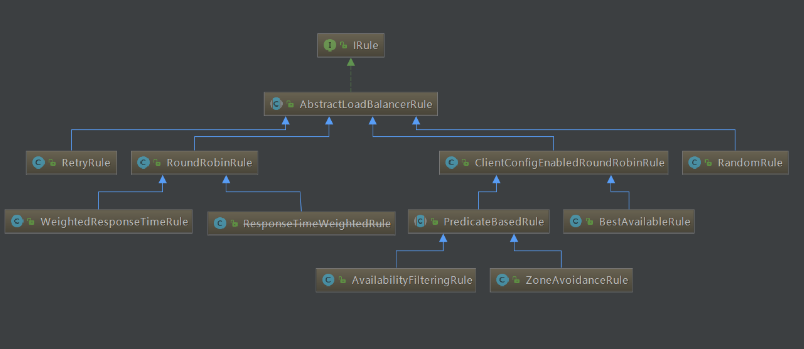
RandomRule: 随机选择一个Server。
RetryRule: 对选定的负载均衡策略机上重试机制,在一个配置时间段内当选择Server不成功,则一直尝试使用subRule的方式选择一个可用的server。
RoundRobinRule: 轮询选择, 轮询index,选择index对应位置的Server。
AvailabilityFilteringRule: 过滤掉一直连接失败的被标记为circuit tripped的后端Server,并过滤掉那些高并发的后端Server或者使用一个AvailabilityPredicate来包含过滤server的逻辑,其实就是检查status里记录的各个Server的运行状态。
BestAvailableRule: 选择一个最小的并发请求的Server,逐个考察Server,如果Server被tripped了,则跳过。
WeightedResponseTimeRule: 根据响应时间加权,响应时间越长,权重越小,被选中的可能性越低。
ZoneAvoidanceRule: 复合判断Server所在区域的性能和Server的可用性选择Server。
3.2.1 修改默认负载均衡策略
3.2.2 自定义负载均衡策略
1)自定义负载算法
2)在SpringBoot主程序扫描的包外定义配置类
注意:自定义的负载均衡策略不能写在@SpringbootApplication注解的@CompentScan扫描得到的地方,否则自定义的配置类就会被所有的 RibbonClients共享。
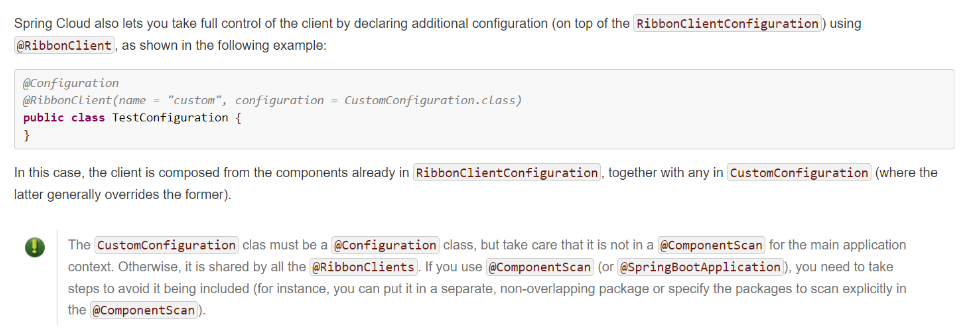
3)使用@RibbonClient指定负载均衡策略,在SpringBoot主程序添加 @RibbonClient 引入配置类
3.3 Ribbon相关接口
参考: org.springframework.cloud.netflix.ribbon.RibbonClientConfiguration
IClientConfig:Ribbon的客户端配置,默认采用DefaultClientConfigImpl实现。
IRule:Ribbon的负载均衡策略,默认采用ZoneAvoidanceRule实现,该策略能够在多区域环境下选出最佳区域的实例进行访问。
IPing:Ribbon的实例检查策略,默认采用DummyPing实现,该检查策略是一个特殊的实现,实际上它并不会检查实例是否可用,而是始终返回true,默认认为所有服务实例都是可用的。
ServerList:服务实例清单的维护机制,默认采用ConfigurationBasedServerList实现。
ServerListFilter:服务实例清单过滤机制,默认采ZonePreferenceServerListFilter,该策略能够优先过滤出与请求方处于同区域的服务实例。
ILoadBalancer:负载均衡器,默认采用ZoneAwareLoadBalancer实现,它具备了区域感知的能力。
修改配置:
4 Ribbon源码分析
源码分析: https://www.processon.com/view/link/5e7466dce4b027d999bdaddb
版权声明: 本文为 InfoQ 作者【程序员Fox】的原创文章。
原文链接:【http://xie.infoq.cn/article/ecee1435fccb990f3f23a2c03】。文章转载请联系作者。
评论