【数据结构】Java 常用集合类 ArrayDeque
ArrayDeque 整体设计
ArrayDeque 使用循环数组实现的双端队列实现,继承自抽象类 AbstractCollection,同时实现了 Deque 接口(和 Cloneable、Serializable 接口)。双端队列可以实现单端队列的先入先出的方式,也可以实现栈结构的先入后出的方式。ArrayDeque 是非线程安全的。
Queue 是一个单端队列,Deque 是一个双端队列。
ArrayDeque 属性和构造函数
tail
表示的是下一个要加入的元素的索引,不是当前最后一个元素的索引。数组的大小必须是 2^n。由
allocateElements()
方法实现。
ArrayDeque 添加元素
ArrayDeque 可以在队首或队尾添加元素:
add
、offer
方法相当于 addLast
在队首插入数据,head
向前移动一位,插入数据。计算 head
坐标方法:
在队尾插入数据,直接插入 tail
位置的元素,并计算下一次 tail
坐标:
在计算 head
和 tail
坐标时采用<u>环形队列</u>的形式,如果当前坐标在队列的头部或尾部,下一次坐标移动到队列的另一端。
ArrayDeque 扩容
当 head
和 tail
相等时,需要进行数组扩容,变为原来容量的两倍:
首先记录当前 head
的位置(p
),然后把容量变为原来的两倍,之后把 head
右侧的元素(包含 head
)复制到新数组的开头(0 - r
位置),再把 head
左侧的元素也复制到新数组(r - n
位置)。
原队列:(H
表示 head
,T
表示 tail
)
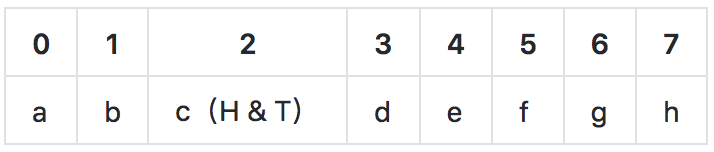
扩容后:

ArrayDeque 获取元素
ArrayDeque 有多种获取元素的方法,
在方向上分为两类:获取队首或队尾元素
在方式上分为两类:获取到元素后从队列中删除或不删除
对不存在元素处理:抛出异常或返回 null
返回 head
元素,队列不变
head
元素出队列
返回 tail
前一位元素
tail
前一位元素出队列
ArrayDeque 删除元素
ArrayDeque 不仅可以移除队首或队尾元素,还可以指定元素移除,或移除第一次(或最后一次)出现的元素:
删除时调用 delete
方法实现,会执行数组拷贝:
版权声明: 本文为 InfoQ 作者【Alex🐒】的原创文章。
原文链接:【http://xie.infoq.cn/article/ea34ff7be8fa023efb357aed2】。
本文遵守【CC-BY 4.0】协议,转载请保留原文出处及本版权声明。
评论