架构师训练营第三周作业
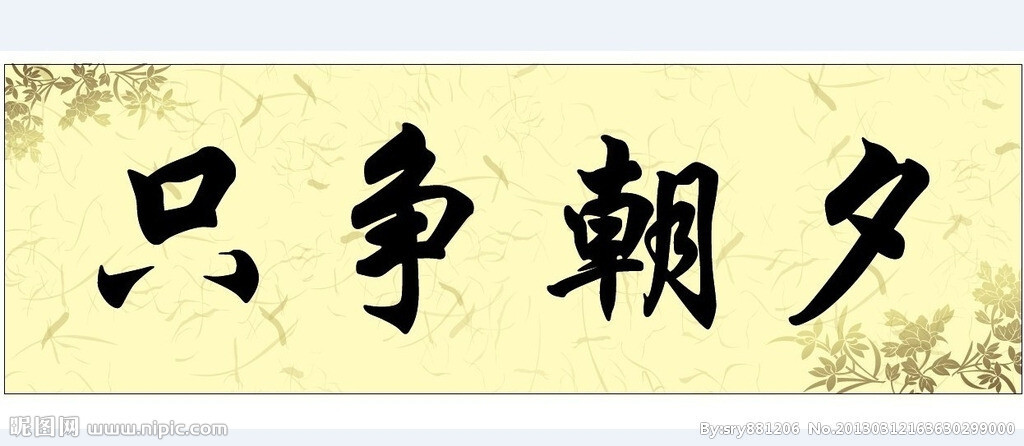
1. 请在草稿纸上手写一个单例模式的实现代码,拍照提交作业。
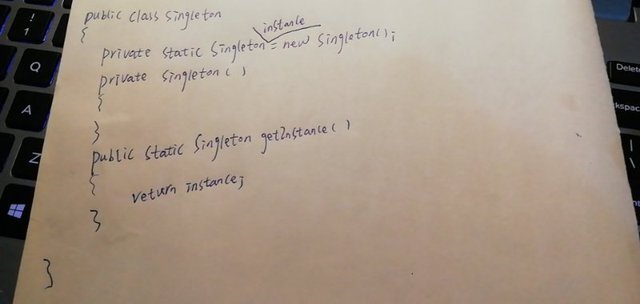
请用组合设计模式编写程序,打印输出图 1 的窗口,窗口组件的树结构如图 2 所示,打印输出示例参考图 3。
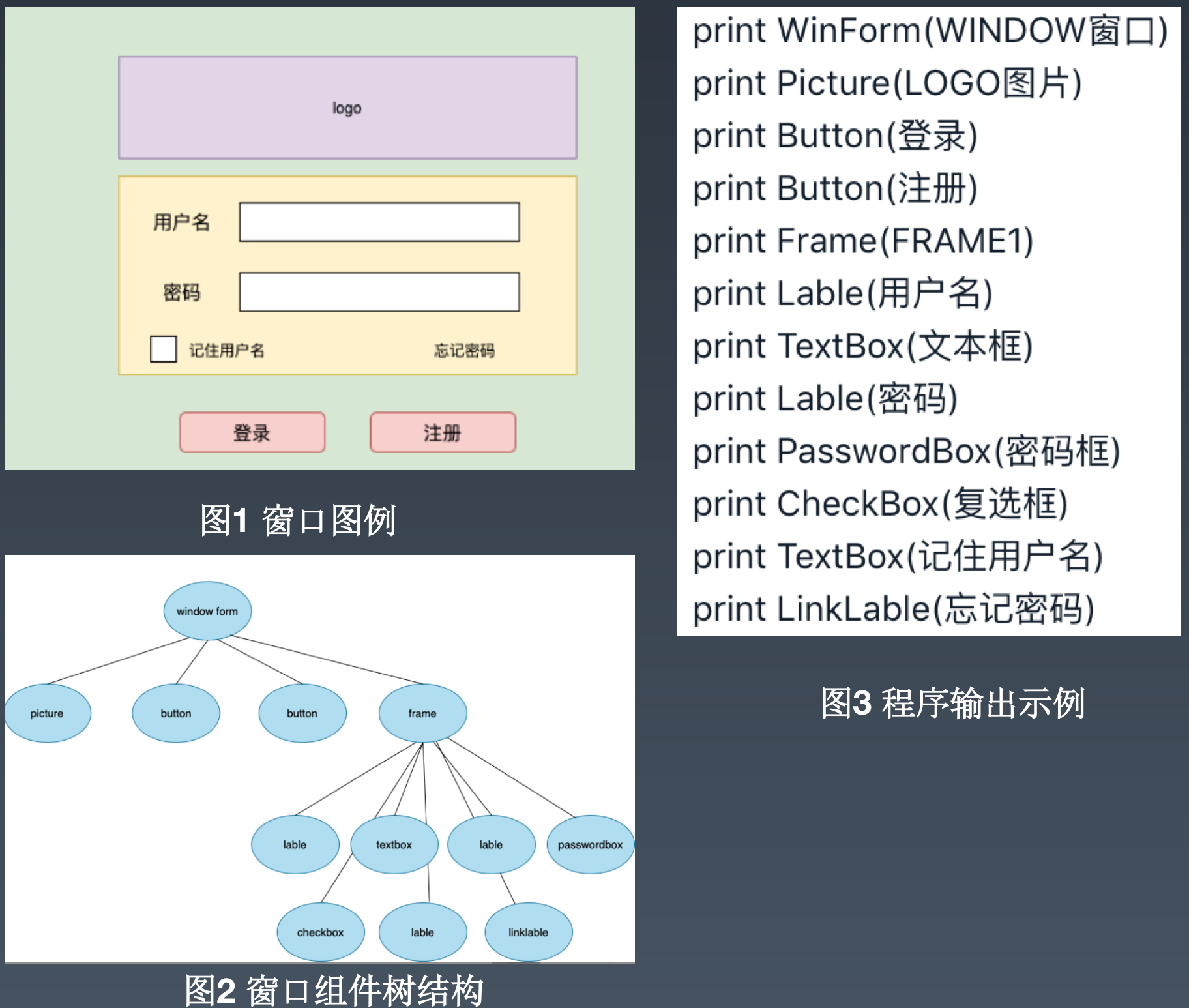
public interface Control {
public void Do();
}
public class WinForm implements Control {
private String name ;
private Frame frame = new Frame("");
public WinForm(String name){
this.name = name ;
}
public void Do(){
System.out.println( StringUtil.outputString( this.getClass().getName(), this.name) ) ;
frame.Do() ;
}
public void AddControl(Control control){
frame.AddControl(control) ;
}
}
import java.util.ArrayList;
import java.util.List;
public class Frame implements Control {
private String name;
private List<Control> subObjs = new ArrayList<>();
public Frame(String name){
this.name = name ;
}
public void Do(){
if ( !name.equals("") ){
System.out.println( StringUtil.outputString( this.getClass().getName(), this.name) ) ;
}
for (Control obj : subObjs){
obj.Do();
}
}
public void AddControl(Control control){
subObjs.add(control) ;
}
}
public class Button implements Control {
private String name ;
public Button(String name){
this.name = name ;
}
public void Do(){
System.out.println( StringUtil.outputString( this.getClass().getName(), this.name) ) ;
}
}
public class CheckBox implements Control{
private String name ;
public CheckBox(String name){
this.name = name ;
}
public void Do(){
System.out.println( StringUtil.outputString( this.getClass().getName(), this.name) ) ;
}
}
public class Lable implements Control{
private String content ;
public Lable(String content){
this.content = content ;
}
public void Do(){
System.out.println( StringUtil.outputString( this.getClass().getName(), this.content) ) ;
}
}
public class LinkLable implements Control {
private String content ;
public LinkLable(String content){
this.content = content ;
}
public void Do(){
System.out.println( StringUtil.outputString( this.getClass().getName(), this.content) ) ;
}
}
public class PasswordBox implements Control{
private String name ;
public PasswordBox(String name){
this.name = name ;
}
public void Do(){
System.out.println( StringUtil.outputString( this.getClass().getName(), this.name) ) ;
}
}
public class Picture implements Control{
private String name ;
public Picture(String name){
this.name = name ;
}
public void Do(){
System.out.println( StringUtil.outputString( this.getClass().getName(), this.name) ) ;
}
}
public class StringUtil {
public static String outputString(String typeName, String content){
return String.format("print %s(%s)", typeName, content) ;
}
}
public class TextBox implements Control {
private String name ;
public TextBox(String name){
this.name = name ;
}
public void Do(){
System.out.println( StringUtil.outputString( this.getClass().getName(), this.name) ) ;
}
}
public class windowns {
public static void main(String[] args){
WinForm winFrm = new WinForm("WINDOW窗口") ;
winFrm.AddControl(new Picture("LOGO图片")) ;
winFrm.AddControl(new Button("登录")) ;
winFrm.AddControl(new Button("注册")) ;
Frame frame = new Frame("FRAME1") ;
frame.AddControl(new Lable("用户名"));
frame.AddControl(new TextBox("文本框"));
frame.AddControl(new Lable("密码"));
frame.AddControl(new PasswordBox("密码框"));
frame.AddControl(new CheckBox("复选框"));
frame.AddControl(new TextBox("记住用户名"));
frame.AddControl(new LinkLable("忘记密码")) ;
winFrm.AddControl(frame) ;
winFrm.Do();
}
}
输出
print WinForm(WINDOW窗口)
print Picture(LOGO图片)
print Button(登录)
print Button(注册)
print Frame(FRAME1)
print Lable(用户名)
print TextBox(文本框)
print Lable(密码)
print PasswordBox(密码框)
print CheckBox(复选框)
print TextBox(记住用户名)
print LinkLable(忘记密码)
评论