单例与组合模式代码实现
发布于: 2020 年 06 月 24 日
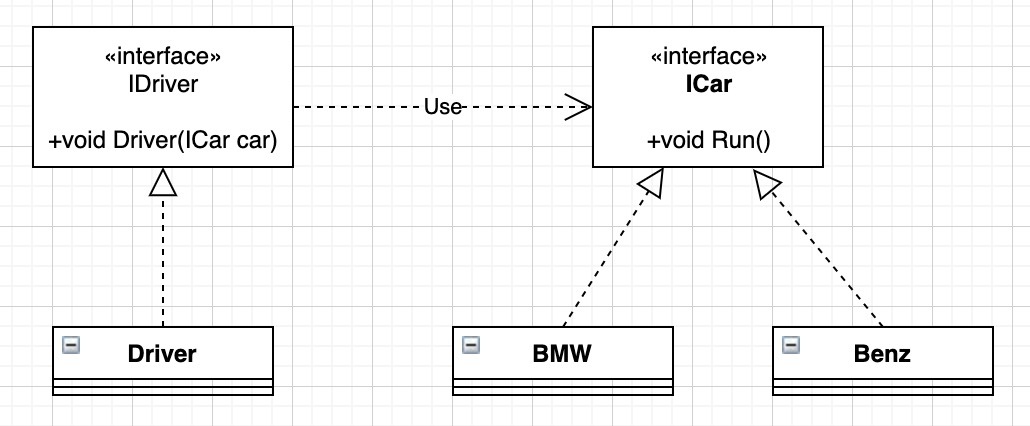
一、Golang单例
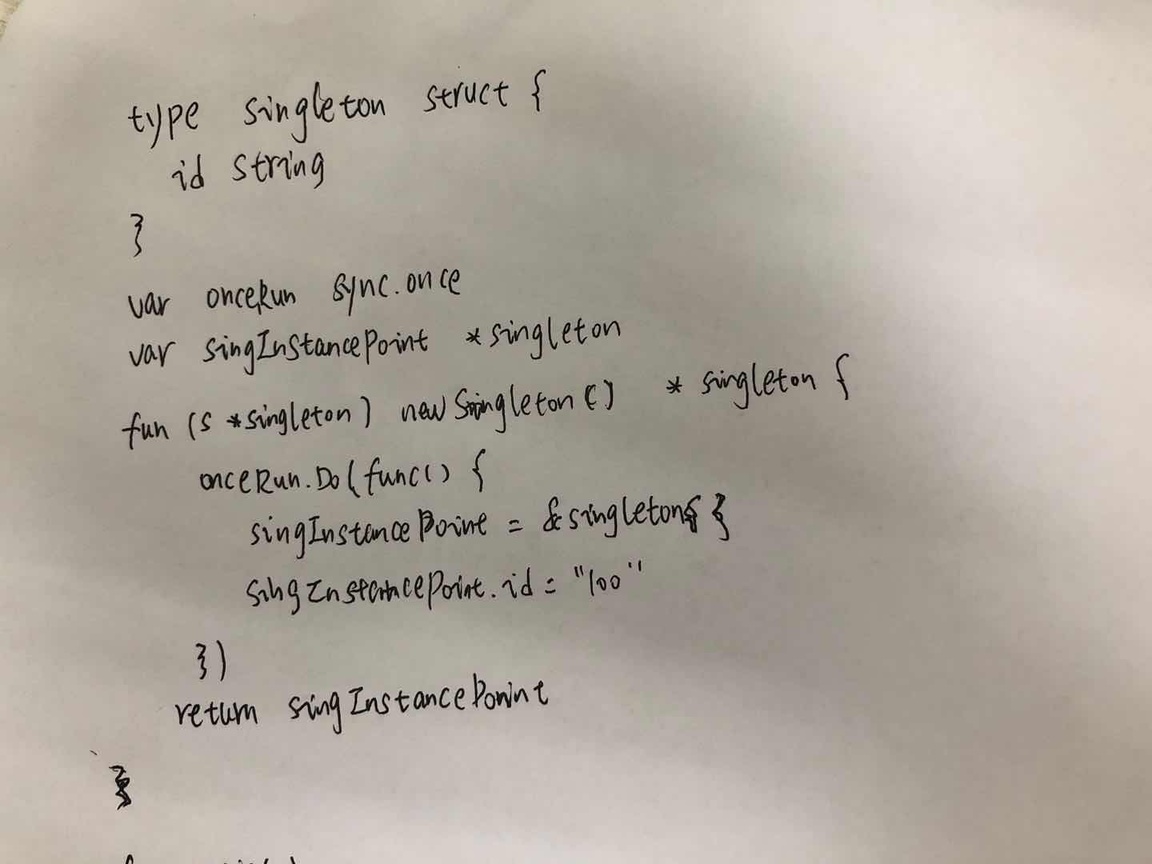
func main(){ ins1:=new(singleton).newSingleton() ins2:=new(singleton).newSingleton() fmt.Printf("ins1.id=%x&ins2.id=%x/n",unsafe.Pointer(&ins1.id),unsafe.Pointer(&ins2.id))}
通过上面的验证代码将可以看到,虽然new了连个实例,他们其实都是对singINstancePoint单例对象的一个引用。
二、组合模式实现
Java实现
//抽象的组件对象,为组合中的对象声明接口,实现接口的缺省行为public abstract class Component { //子组件对象可能有的功能方法 public abstract void someOperation(String preStr); public void addChild(Component child) { //缺省的实现,抛出异常,因为叶子对象没有这个功能,或子类未实现这个功能 throw new UnsupportedOperationException("对象不支持此功能"); } public void removeChild(Component child) { //缺省的实现,抛出异常,因为叶子对象没有这个功能,或子类未实现这个功能 throw new UnsupportedOperationException("对象不支持此功能"); } public Component getChildren(int index) { //缺省的实现,抛出异常,因为叶子对象没有这个功能,或子类未实现这个功能 throw new UnsupportedOperationException("对象不支持此功能"); }}/* 组合类*/public class Composite extends Component { //组件的名字 private String name = ""; public Composite(String name) { this.name = name; } //用来存储组合对象中包含的子组件对象 private List<Component> childComponents = null; //此处用于输出组件的树形结构,通常在里面需要实现递归的调用 @Override public void someOperation(String preStr) { // 先把自己输出 System.out.println(preStr + "+" + name); // 如果还包含其他子组件,那么就输出这些子组件对象 if (null != childComponents) { // 添加一个空格,表示向后缩进一个空格 preStr += " "; // 输出当前对象的子组件对象 for (Component component : childComponents) { // 递归地进行子组件相应方法的调用,输出每个子组件对象 component.someOperation(preStr); } } } //向组合对象中添加组件对象 public void addChild(Component child) { //延迟初始化 if (null == childComponents) { childComponents = new ArrayList<Component>(); } childComponents.add(child); } //从组合对象中移除组件对象 public void removeChild(Component child) { if (null != childComponents) { childComponents.remove(child); } } //根据索引获取组合对象中对应的组件对象 public Component getChildren(int index) { if (null != childComponents) { if (index >= 0 && index < childComponents.size()) { return childComponents.get(index); } } return null; }}public class Leaf extends Component { //组件的名字 private String name = ""; public Leaf(String name) { this.name = name; } //此处用于输出组件的树形结构 @Override public void someOperation(String preStr) { System.out.println(preStr + "-" + name); }}public class main { //客户端中使用Component接口来操作组合对象结构 public static void main(String[] args) { // 定义多个Composite组合对象 Component winForm = new Composite("WINDOW窗口"); Component cframe = new Composite("FRAME1"); // 定义多个Leaf叶子对象 Component lflogo = new Leaf("LOGO图片"); Component lflogin = new Leaf("登录Button"); Component lfregister = new Leaf("注册Button"); Component cUname = new Leaf("用户名Label"); Component cUnameVal = new Leaf("用户名TextBox"); Component cPass = new Leaf("密码Label"); Component cPassVal = new Leaf("密码TextBox"); Component remLabel = new Composite("记住用户名Label"); Component remChkBox = new Leaf("记住用户名CheckBox"); Component linkLabel = new Leaf("忘记密码LinkLabel"); // 组合成为树形的对象结构 winForm.addChild(lflogo); winForm.addChild(lflogin); winForm.addChild(lfregister); winForm.addChild(cframe); cframe.addChild(cUname); cframe.addChild(cUnameVal); cframe.addChild(cPass); cframe.addChild(cPassVal); remLabel.addChild(remChkBox); cframe.addChild(remLabel); cframe.addChild(linkLabel); // 调用根对象的输出功能输出整棵树 winForm.someOperation(""); }}
程序输出如下 :
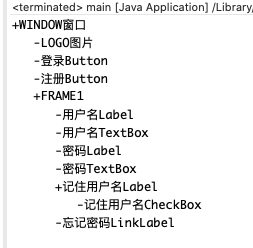
Golang实现
//接⼝,关于树的遍历操作都在这⾥//抽象组件(Component)角色type tree interface { do()}//树的中间节点 组合对象(Composite)角色type node struct { *list.List //(匿名)组合即继承,node拥有了list的特性 name string}func (n node) do() { //定义即实现,node实现了tree接⼝ for e := n.Front(); e != nil; e = e.Next() { //node拥有了list的特性 e.Value.(tree).do() } fmt.Println(n.name + " node")}func (n node) addSub(sub tree) { //PushBack inserts a new element e with value v at the back of list l and returns e. //在节点n后增加一个子节点 n.PushBack(sub)}//树的叶⼦ 叶子对象角色type leaf struct{ name string }func (l leaf) do() { //定义即实现,leaf实现了tree接⼝ fmt.Println(l.name + " leaf")}func main() { //定义树的节点(组合对象) root := node{list.New(), "WinForm(WINDOWS窗口)"} //定义叶子节点 lLogo := leaf{"Picture(Logo图片)"} lLogin := leaf{"Button(登录)"} lRegister := leaf{"Button(注册)"} nFrame := node{list.New(), "Frame(FRAME1)"} nFrame.addSub(leaf{"LinkLabel(忘记密码)"}) nFrame.addSub(leaf{"TextBox(记住用户名)"}) nFrame.addSub(leaf{"CheckBox复选框"}) nFrame.addSub(leaf{"PasswordBox密码框"}) nFrame.addSub(leaf{"Lable密码"}) nFrame.addSub(leaf{"TextBox(文本框)"}) nFrame.addSub(leaf{"Lable用户名"}) //构造树的结构 root.addSub(nFrame) root.addSub(lRegister) root.addSub(lLogin) root.addSub(lLogo) //遍历树 root.do()}
运行结果如下
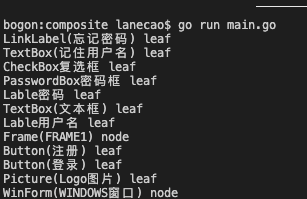
划线
评论
复制
发布于: 2020 年 06 月 24 日阅读数: 63
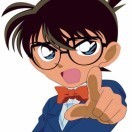
Lane
关注
还有梦想 2018.07.05 加入
还未添加个人简介
评论