Week3 作业
发布于: 2020 年 06 月 21 日
作业一:请在草稿纸上手写一个单例模式的实现代码,拍照提交作业。
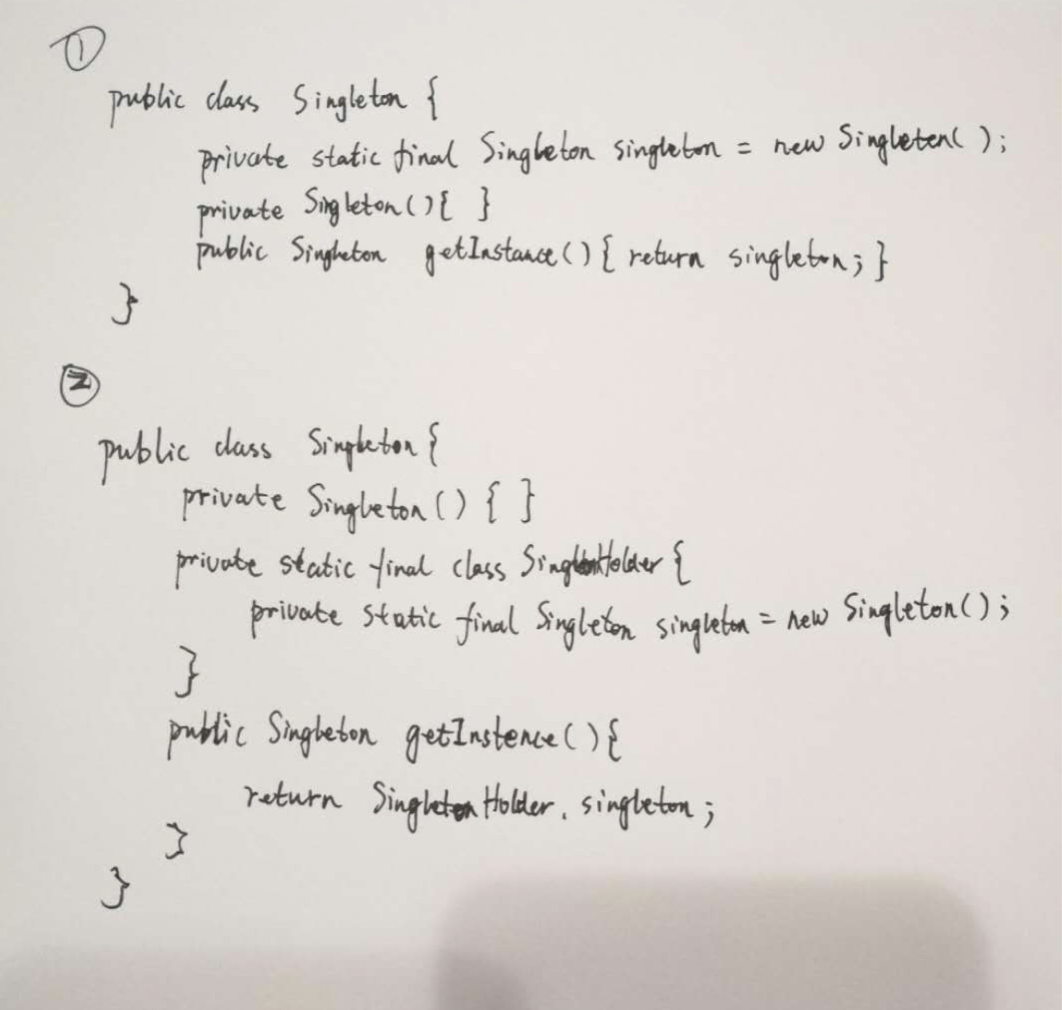
作业二:请用组合设计模式编写程序,打印输出图 1 的窗口,窗口组件的树结构如图 2 所示,打印输出示例参考图 3。
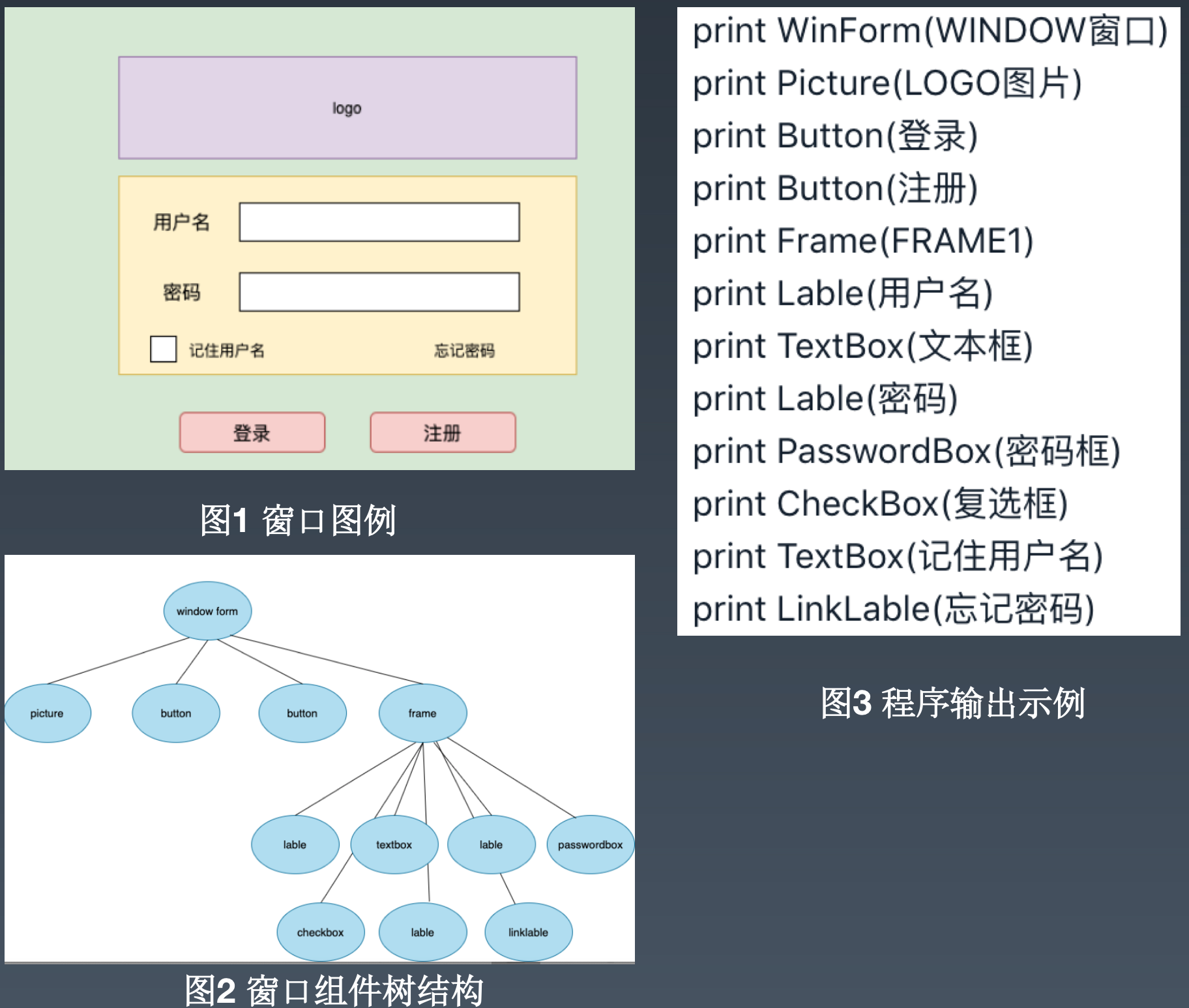
类图:
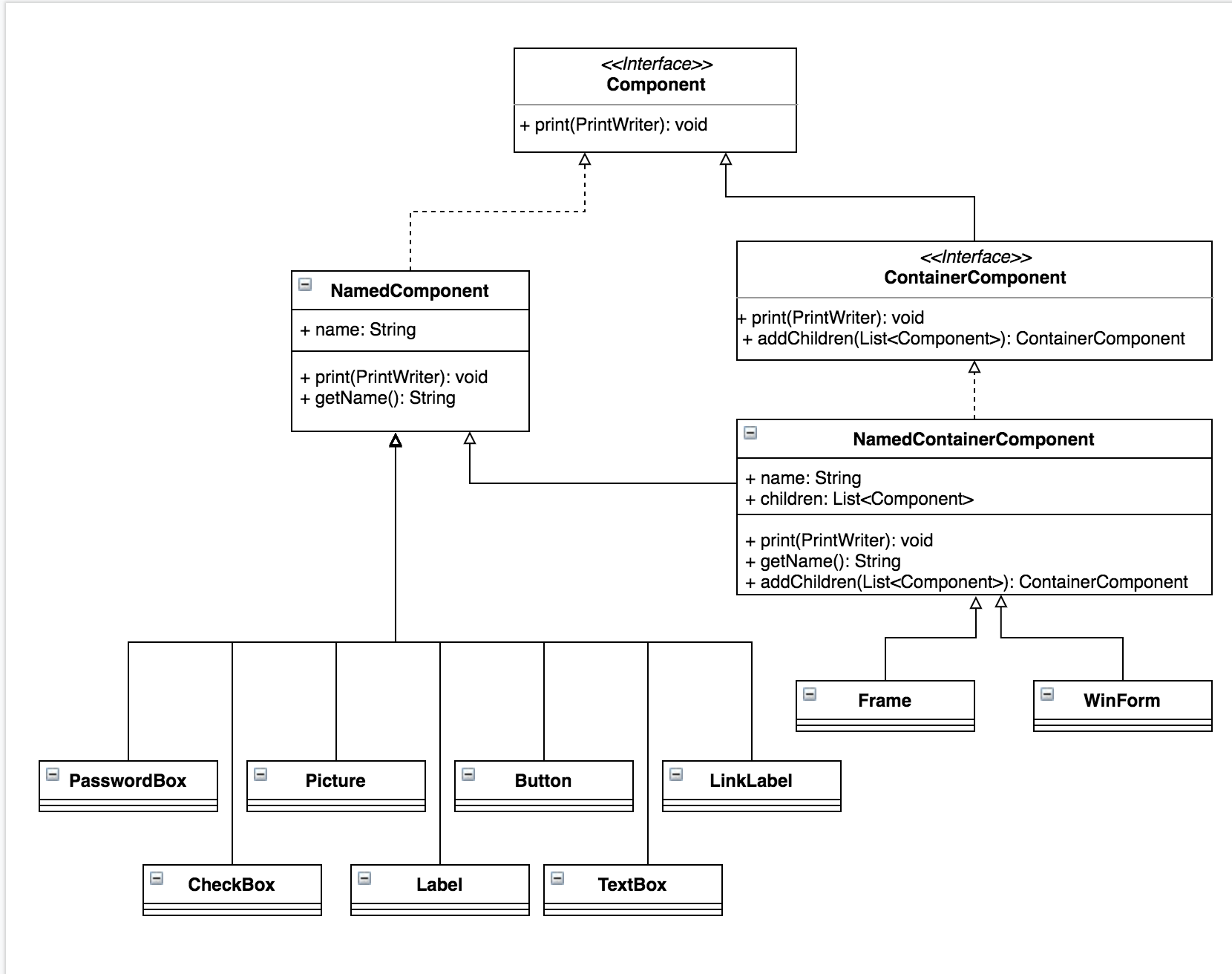
Component.java 文件:(为了简便,所有接口和实现类都写在该文件中)
package com.xql.arch.week3;import java.io.PrintWriter;import java.util.Arrays;import java.util.LinkedList;import java.util.List;public interface Component { void print(PrintWriter printWriter);}interface ContainerComponent extends Component { ContainerComponent addChildren(List<Component> children);}abstract class NamedComponent implements Component { private String name; public NamedComponent(String name) { this.name = name; } @Override public void print(PrintWriter printWriter) { printWriter.println("print " + getClass().getSimpleName() + "(" + this.getName() + ")"); } public String getName() { return this.name; }}abstract class NamedContainerComponent extends NamedComponent implements ContainerComponent { private List<Component> children; public NamedContainerComponent(String name) { super(name); this.children = new LinkedList<>(); } public Component addChildren(Component... children) { return this.addChildren(Arrays.asList(children)); } @Override public ContainerComponent addChildren(List<Component> children) { assert children != null; this.children.addAll(children); return this; } @Override public void print(PrintWriter printWriter) { super.print(printWriter); this.children.forEach(component -> component.print(printWriter)); }}class WinForm extends NamedContainerComponent { public WinForm(String name) { super(name); }}class Frame extends NamedContainerComponent { public Frame(String name) { super(name); }}class Picture extends NamedComponent { public Picture(String name) { super(name); }}class Button extends NamedComponent { public Button(String name) { super(name); }}class Label extends NamedComponent { public Label(String name) { super(name); }}class TextBox extends NamedComponent { public TextBox(String name) { super(name); }}class PasswordBox extends NamedComponent { public PasswordBox(String name) { super(name); }}class CheckBox extends NamedComponent { public CheckBox(String name) { super(name); }}class LinkLabel extends NamedComponent { public LinkLabel(String name) { super(name); }}
ComponentTest.java : 用来测试Component的输出是否满足预期结果
package com.xql.arch.week3;import org.junit.Assert;import org.junit.Test;import java.io.PrintWriter;import java.io.StringWriter;public class ComponentTest { @Test public void testWinForm() { Component winForm = new WinForm("WINDOW窗口").addChildren( new Picture("LOGO图片"), new Button("登录"), new Button("注册"), new Frame("FRAME1").addChildren( new Label("用户名"), new TextBox("文本框"), new Label("密码"), new PasswordBox("密码框"), new CheckBox("复选框"), new TextBox("记住用户名"), new LinkLabel("忘记密码") ) ); StringWriter out = new StringWriter(); PrintWriter writer = new PrintWriter(out); winForm.print(writer); System.out.println(out.toString()); String expected = "print WinForm(WINDOW窗口)\n" + "print Picture(LOGO图片)\n" + "print Button(登录)\n" + "print Button(注册)\n" + "print Frame(FRAME1)\n" + "print Label(用户名)\n" + "print TextBox(文本框)\n" + "print Label(密码)\n" + "print PasswordBox(密码框)\n" + "print CheckBox(复选框)\n" + "print TextBox(记住用户名)\n" + "print LinkLabel(忘记密码)\n"; Assert.assertEquals(expected, out.toString()); }}
划线
评论
复制
发布于: 2020 年 06 月 21 日阅读数: 54
版权声明: 本文为 InfoQ 作者【Shawn】的原创文章。
原文链接:【http://xie.infoq.cn/article/de4c3b8ea79ed02e0872c8fd1】。未经作者许可,禁止转载。
Shawn
关注
还未添加个人签名 2017.10.23 加入
还未添加个人简介
评论