架构师训练营第三周作业
发布于: 2020 年 10 月 04 日
1、请在草稿纸上手写一个单例模式的实现代码,拍照提交作业。(我写了一遍,本人的字太不堪入目了,还是机打吧🤦♂️)
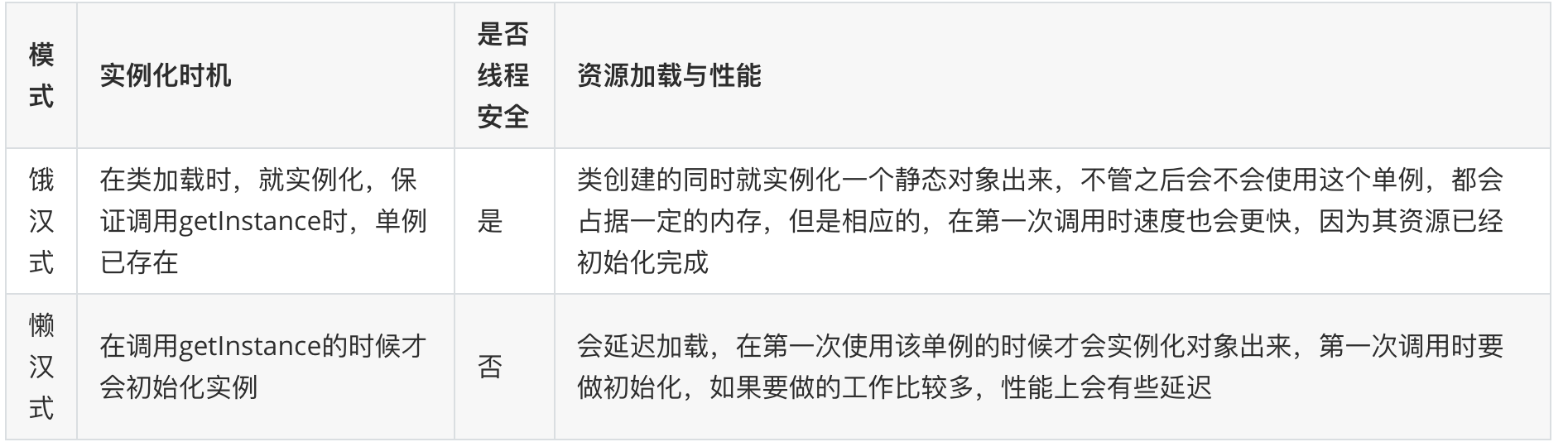
饿汉式:
public class Singleton { private Singleton() {} private static final Singleton single = new Singleton(); public static Singleton getInstance() { return single; } }
懒汉式:
public class Singleton { private Singleton() {} private static Singleton single=null; public static Singleton getInstance() { if (single == null) { single = new Singleton(); } return single; } }
上述懒汉式实现方式非线程安全,在多线程环境下会出现多实例问题,违背设计初衷,
public class Singleton { // volatitle 关键字防止指令重排, private static volatile Singleton instance = null; private Singleton() {} public static Singleton getInstance() { if (instance == null){ synchronized(Singleton.class){ if (instance == null) instance = new Singleton(); } } return instance; }}
上述代码使用双重检测以及volatitle关键字保证懒汉式在多线程下同样达到单例模式效果
其他实现方式还有静态内部类和枚举的方式实现到单例模式
2、请用组合设计模式编写程序,打印输出图 1 的窗口,窗口组件的树结构如图 2 所示,打印输出示例参考图 3。
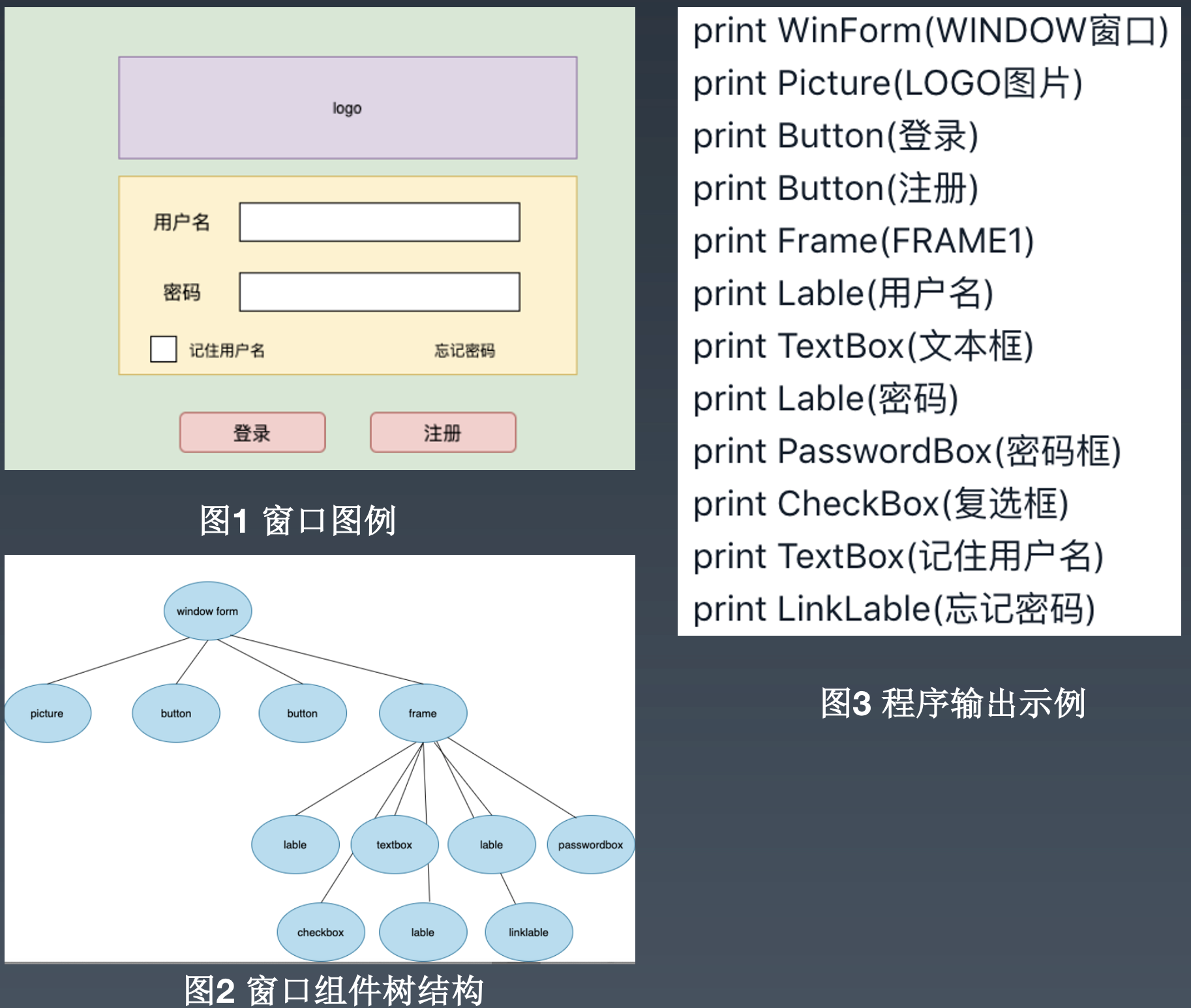
组件基类
public abstract class Component { /** * 组件名称 */ private String componentName; public Component(String componentName) { this.componentName = componentName; } /** * 添加容器组件 * @param fromComponent */ public abstract void addComposite(Component fromComponent); /** * 定义统一业务方法 */ public abstract void print(); public String getComponentName() { return componentName; }}
windowfrom容器组件
public class WindowFrom extends Component { private List<Component> list = new ArrayList<>(); public WindowFrom(String componentName) { super(componentName); } @Override public void addComposite(Component fromComponent) { list.add(fromComponent); } @Override public void print() { System.out.println(getClass().getSimpleName()+"(" + super.getComponentName() + ")"); for (Component item : list) { item.print(); } }}
frame容器组件
public class Frame extends Component { private List<Component> list = new ArrayList<>(); public Frame(String componentName) { super(componentName); } @Override public void addComposite(Component fromComponent) { list.add(fromComponent); } @Override public void print() { System.out.println(getClass().getSimpleName()+"(" + super.getComponentName() + ")"); for (Component item : list) { item.print(); } }}
按钮组件
public class Button extends Component { public Button(String componentName) { super(componentName); } @Override public void addComposite(Component fromComponent) { } @Override public void print() { System.out.println(getClass().getSimpleName()+"(" + super.getComponentName() + ")"); }}
其他组件checkbox、label、linklabel、textbox、passwordbox、picture类似
client调用代码
public static void main(String[] args) { WindowFrom windowFrom = new WindowFrom("WINDOW窗口"); windowFrom.addComposite(new Picture("LOGO图片")); windowFrom.addComposite(new Button("登录")); windowFrom.addComposite(new Button("注册")); Frame frame = new Frame("FRAME1"); windowFrom.addComposite(frame); frame.addComposite(new Label("用户名")); frame.addComposite(new TextBox("文本框")); frame.addComposite(new Label("密码")); frame.addComposite(new PasswordBox("密码框")); frame.addComposite(new CheckBox("复选框")); frame.addComposite(new Label("记住用户名")); frame.addComposite(new LinkLabel("忘记密码")); windowFrom.print();}
infoQ 没有markdown好用,弄代码太费事了😭
划线
评论
复制
发布于: 2020 年 10 月 04 日 阅读数: 15
版权声明: 本文为 InfoQ 作者【四夕晖】的原创文章。
原文链接:【http://xie.infoq.cn/article/d676792ef09be86a4bbb2c41a】。
本文遵守【CC BY-NC】协议,转载请保留原文出处及本版权声明。
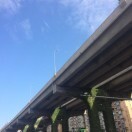
四夕晖
关注
还未添加个人签名 2018.01.16 加入
还未添加个人简介
评论