LeetCode 题解:622. 设计循环队列,使用数组,JavaScript,详细注释
发布于: 2020 年 09 月 16 日
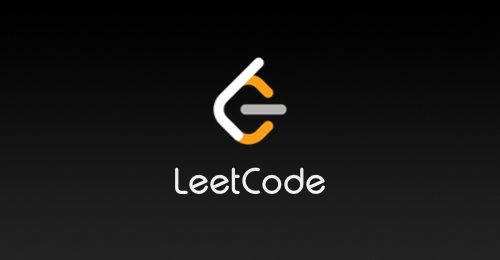
原题链接:https://leetcode-cn.com/problems/design-circular-queue/
解题思路:
采用两个指针,头指针指向队首,尾指针指向入队元素插入队列的位置,尾指针-1则为队尾。
进行出入队列操作时,头尾指针都加1,注意处理指针移出队列范围时,要循环到队列内部的问题。
/** * Initialize your data structure here. Set the size of the queue to be k. * @param {number} k */var MyCircularQueue = function (k) { this.capacity = k; // 队列的长度 this.head = 0; // 队首的位置 this.tail = 0; // 元素入队时存储的位置,this.tail - 1为队尾 this.queue = new Array(k).fill(null); // 创建数组存储队列};/** * Insert an element into the circular queue. Return true if the operation is successful. * @param {number} value * @return {boolean} */MyCircularQueue.prototype.enQueue = function (value) { // 判断队列是否已满 if (this.isFull()) { return false; } // 入队元素存入尾指针位置 this.queue[this.tail] = value; // 尾指针向后移动一位,需要处理增加之后循环到头部 this.tail = (this.tail + 1 + this.capacity) % this.capacity; return true;};/** * Delete an element from the circular queue. Return true if the operation is successful. * @return {boolean} */MyCircularQueue.prototype.deQueue = function () { // 判断队列是否为空 if (this.isEmpty()) { return false; } // 将头指针位置置空 this.queue[this.head] = null; // 头指针向后移动一位,需要处理增加之后循环到头部 this.head = (this.head + 1 + this.capacity) % this.capacity; return true;};/** * Get the front item from the queue. * @return {number} */MyCircularQueue.prototype.Front = function () { // 头指针的位置即为队首 return this.queue[this.head] !== null ? this.queue[this.head] : -1;};/** * Get the last item from the queue. * @return {number} */MyCircularQueue.prototype.Rear = function () { // 尾指针的位置为要插入元素的位置,队尾位置在尾指针-1 // 需要注意处理this.tail - 1为-1时,循环到队列尾部 const lastIndex = (this.tail + this.capacity - 1) % this.capacity; return this.queue[lastIndex] !== null ? this.queue[lastIndex] : -1;};/** * Checks whether the circular queue is empty or not. * @return {boolean} */MyCircularQueue.prototype.isEmpty = function () { // 当头指针为空,表示队列已经无元素 return this.queue[this.head] === null;};/** * Checks whether the circular queue is full or not. * @return {boolean} */MyCircularQueue.prototype.isFull = function () { // 当尾指针有值时,表示已经无法插入元素 return this.queue[this.tail] !== null;};
划线
评论
复制
发布于: 2020 年 09 月 16 日阅读数: 38
版权声明: 本文为 InfoQ 作者【Lee Chen】的原创文章。
原文链接:【http://xie.infoq.cn/article/d60c4982f9de81db31fc12bfa】。文章转载请联系作者。
Lee Chen
关注
还未添加个人签名 2018.08.29 加入
还未添加个人简介
评论