架构师训练营 性能压测命题作业
性能压测的时候,随着并发量的增加,系统响应时间和吞吐量如何变化?为什么?
系统都会有一个瓶颈,也就是系统能支持的最大并发数量,当并发逐渐增大的时候,响应时间通常是会随着并发不断的增加而缓慢降低的,而一旦并发超过系统的最大承受能力,系统的响应时间就会急剧下降。
系统响应时间在并发上升初期会缓慢上升,而达到临界点后会急剧上升
举个例子,一个系统同时只能支撑100的并发,超过100并发后,后面过来的请求就只能阻塞等待,而一个请求执行耗时是x,那么超过100并发后,后面来的100请求耗时就是等待的时间加自己执行的时间,就是2x,假如后面来了1000个请求呢?那请求的耗时就会最大要到11x,已经慢了10倍。
而如果后面来的请求如果不是阻塞等待,而是一起竞争cpu资源的话,cpu假设x时间最多处理100个请求,这时候分在每个请求上的cpu时间是x/100,那这时候如果有200个请求过来的话,x时间,每个请求只能分到x/200,而每个请求至少要x/100的时间才能完成,那就需要2x的时间才能完成着200个请求,而如果来了1000个请求,那就需要10x的时间才能完成。
而且线程上下文切换还会有性能损耗,如果访问的数据库,数据库连接的资源也有限,需要排队等待,等等,所以,并发升高后应用的耗时是会逐渐上升的,只是在没达到系统瓶颈前,耗时上升应该比较缓慢,而上升到一个瓶颈,耗时就会急剧上升。
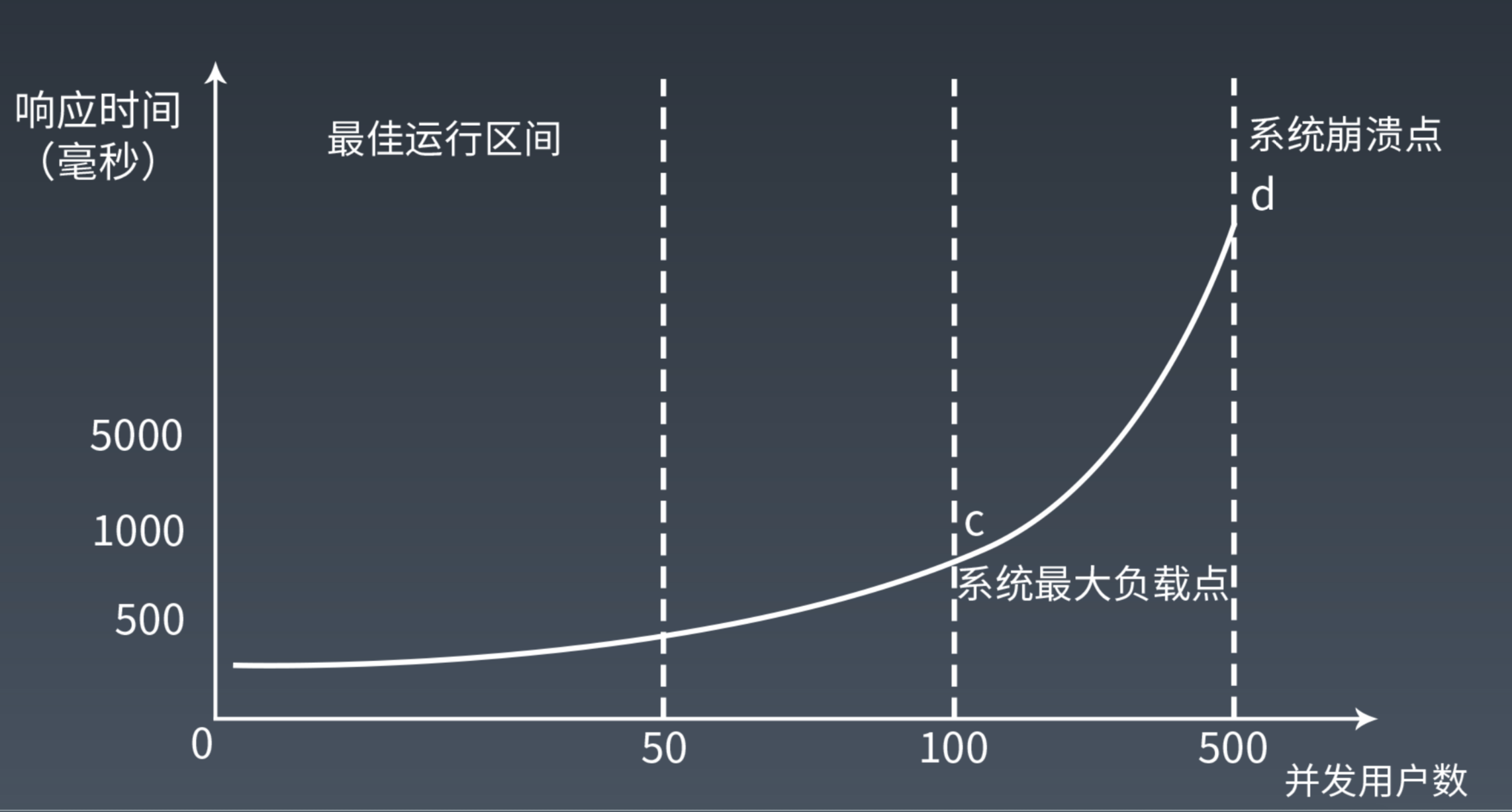
吞吐量在早期会随着并发升高很快上升,而随着系统响应时间在临界点的急剧上升,吞吐量会开始急剧下降
吞吐量的计算公式是:吞吐量=并发数/平均响应时间
初期因为并发数不断上升,但是平均响应时间上升并不明显,所以吞吐量会不断升高。
而响应时间在达到临界点后急剧上升,吞吐量也就随着继续降低。
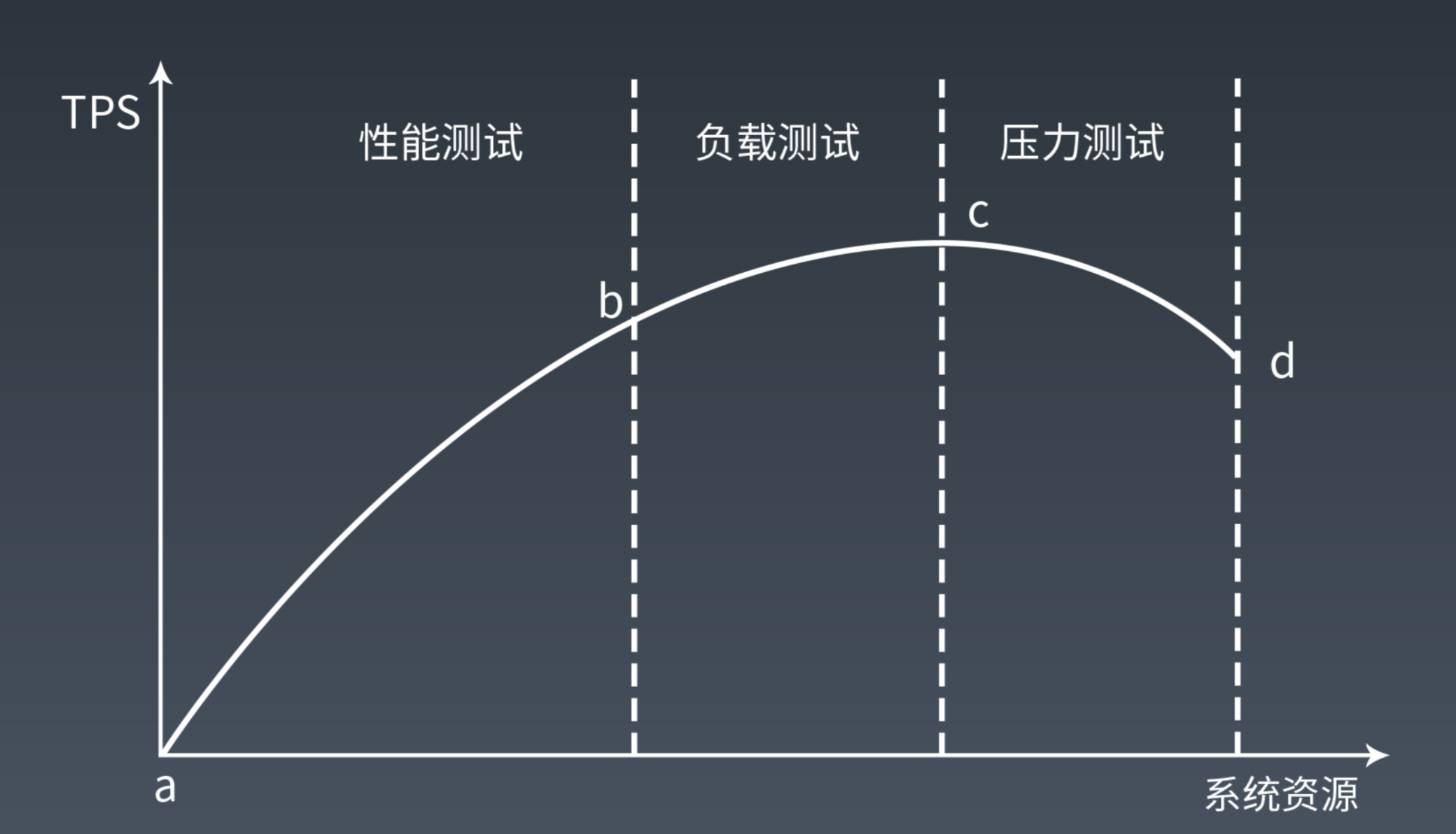
用你熟悉的编程语言写一个 web 性能压测工具,输入参数:URL,请求总次数,并发数。输出参数:平均响应时间,95% 响应时间。用这个测试工具以 10 并发、100 次请求压测 www.baidu.com。
最后输出:
版权声明: 本文为 InfoQ 作者【Cloud.】的原创文章。
原文链接:【http://xie.infoq.cn/article/d21943f0643c6261b52a8ca79】。未经作者许可,禁止转载。
评论