江帅帅:精通 Spring Boot 系列 04
1. Web 开发的支持
使用 Spring Boot 实现 Web 开发更加便捷了,因为直接依赖 spring-boot-starter-web 模块即可支持 Web 开发,此模块预定义了 Web 开发中常用的依赖包,还有内嵌的 Tomcat 作为默认 Web 容器。
2. Thymeleaf 模板引擎
目前,多数企业级应用开发中都支持前后端分离,但还有少数离不开视图层技术,Spring Boot 提供了很多模板引擎来支持视图层技术,比如 Thymeleaf、Freemarker、Velocity。
Thymeleaf 是官方推荐使用的新一代 Java 模板引擎,并支持 HTML 原型,模板表达式在脱离运行环境下不污染 HTML 结构,能让前端直接通过浏览器查看基本样式,也能让后端使用真实数据查看展示效果。
3. 整合使用 Thymeleaf 模板
3.1. 创建工程
创建一个 Spring Boot 工程,编辑 pom.xml 文件,添加 web 和 thymeleaf 依赖。另外,App 启动类与之前一致。
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
</dependencies>
3.2. 添加视图文件
在 src/main/resources/templates 目录下,新建 nicebook.html 文件。
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>良心好书</title>
</head>
<body>
<table border="1">
<tr>
<td>序号</td>
<td>好书</td>
<td>作者</td>
</tr>
<tr th:each="book:${books}">
<td th:text="${book.id}"></td>
<td th:text="${book.name}"></td>
<td th:text="${book.author}"></td>
</tr>
</table>
</body>
</html>
3.3. 配置 Thymeleaf
如果想自定义 Thymeleaf 配置参数,可以在 application.properties 文件中进行配置,常见的配置选项如下:
# 模板文件存放位置
spring.thymeleaf.prefix=classpath:/templates/
# 是否开启缓存,默认为 true,开发时可设置为 false
spring.thymeleaf.cache=true
# 检查模板位置是否存在,默认为 true
spring.thymeleaf.check-template-location=true
# 检查模板是否存在,默认为 true
spring.thymeleaf.check-template=true
# 模板文件后缀设置
spring.thymeleaf.suffix=.html
# 模板文件编码设置
spring.thymeleaf.encoding=UTF-8
# Content-Type 配置
spring.thymeleaf.servlet.content-type=text/html
3.4. 创建 POJO
public class Book {
private Integer id;
private String name;
private String author;
// getter 和 setter 方法
}
3.5. 创建 BookController 控制器
@Controller
public class BookController {
@GetMapping("/books")
public ModelAndView books() {
List<Book> bookList = new ArrayList<>()
Book book1 = new Book();
book1.setId(1);
book1.setName("《码农翻身:用故事给技术加点料》");
book1.setAuthor("刘欣");
Book book2 = new Book();
book2.setId(2);
book2.setName("《漫画算法:小灰的算法之旅(全彩)》");
book2.setAuthor("魏梦舒");
bookList.add(book1);
bookList.add(book2);
ModelAndView mv = new ModelAndView();
mv.addObject("bookList");
mv.setViewName("nicebook");
return mv;
}
}
3.6. 运行测试
浏览器中访问:http://localhost:8080/books,即可看到如下页面。
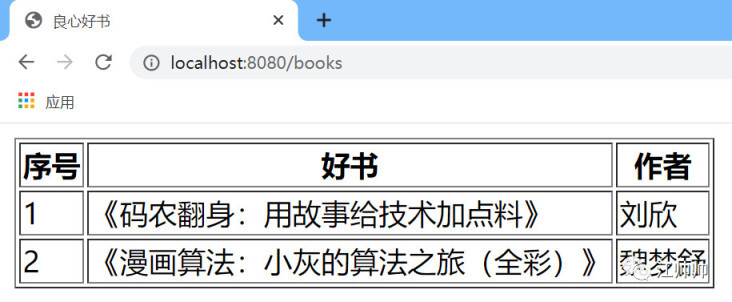
4. Thymeleaf 的支持
Spring Boot 通过 org.springframework.boot.autoconfigure.thymeleaf 包为 Thymeleaf 提供了自动配置,涉及到的类如下:
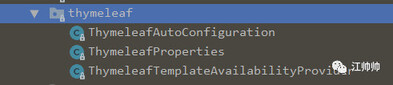
其中 ThymeleafAutoConfiguration 和 ThymeleafProperties 类是比较重要的,前者对集成所需要的 Bean 进行自动配置,后者主要读取 application.properties 配置文件,可自定义 Thymeleaf 的属性和默认配置。
ThymeleafProperties 类部分源码如下:
@ConfigurationProperties(
prefix = "spring.thymeleaf"
)
public class ThymeleafProperties {
private static final Charset DEFAULT_ENCODING;
public static final String DEFAULT_PREFIX = "classpath:/templates/";
public static final String DEFAULT_SUFFIX = ".html";
private boolean checkTemplate = true;
private boolean checkTemplateLocation = true;
private String prefix = "classpath:/templates/";
private String suffix = ".html";
private String mode = "HTML";
private Charset encoding;
private boolean cache;
private Integer templateResolverOrder;
private String[] viewNames;
private String[] excludedViewNames;
private boolean enableSpringElCompiler;
private boolean renderHiddenMarkersBeforeCheckboxes;
private boolean enabled;
private final ThymeleafProperties.Servlet servlet;
private final ThymeleafProperties.Reactive reactive;
...
}
5. 拓展:Thymeleaf 常用语法
5.1. 使用 URL
通过 @{…} 来处理常见 URL。
<a th:href="@{http://www.naixuejiaoyu.com}">奈学教育</a>
<a th:href="@{/}">奈学教育</a>
<a th:href="@{books/java/one.png}">奈学教育</a>
5.2. 使用表达式
主要用来从模板中的 WebContext 获取param、request、session 和 application 中的属性。使用 ${x} 即可返回存储在 Thymeleaf 上下文中的变量 x 或作为 request 作用域中的属性。
${param.x} 能够返回名为 x 的请求参数;
${session.x} 能够返回名为 x 的 HttpSession 作用域中的属性;
${application.x} 能够返回名为 x 的 ServletContext 作用域中的属性。
5.3. 使用字符串
如果需要对一段文字中的某一处进行替换,可以使用 |…| 这种便捷方式,但不能包含其他常量、条件表达式,只能包含变量表达式 x即可返回存储在Thymeleaf上下文中的变量x或作为request作用域中的属性。¨G7G¨K25K如果需要对一段文字中的某一处进行替换,可以使用∣…∣这种便捷方式,但不能包含其他常量、条件表达式,只能包含变量表达式{…},有一定局限性。
<span th:text="|hello, ${userName}|"></span>
5.4. 使用运算符
平时看到的算术运算符和逻辑运算符都可以使用。
5.5. 使用条件判断
可以使用 th:if 和 th:unless 属性进行条件判断,前者条件成立时显示,后者不成立时才显示。也可以使用 Switch 结构,默认选项使用 * 来表示。
<a th:href="index.html" th:if=${name != null}>奈学教育</a>
<div th:switch="${books}">
<p th:case="'Java'">Java 从入门到逃难</p>
<p th:case="'Python'">Python 从入门到逃难</p>
</div>
5.6. 使用循环
使用 th:each 即可实现循环。
<tr th:each="book : ${bookList}">
<td th:text="${book.id}"></td>
<td th:text="${book.name}"></td>
<td th:text="${book.author}"></td>
</tr>
5.7. 使用内置对象
通过 # 可以直接访问 Thymeleaf 的内置对象。
#dates:日期
#calendars:日历
#numbers:数值格式化
#strings:字符串格式化
#objects:对象
#maps:Map 操作工具
#aggregates:操作数组或集合的工具
#bools:布尔
#sets:Set 操作工具
#messages:消息
#arrays:Array 操作工具
#lists:List 操作工具
来源于:奈学开发者社区-江帅帅
评论