架构师训练营第 1 期 - 第三周课后练习
发布于: 2020 年 10 月 02 日
请在草稿纸上手写一个单例模式的实现代码,拍照提交作业。
Singleton是一个线程安全的单例,通过私有的构造方法保证只能从内部创建。getInstance方法第一次执行的时候,JVM会去加载内部类SingletonHolder。加载类的时候,JVM会保证只初始化一次,这样多个线程同时调用getInstance方法的时候,也不会有线程安全的问题
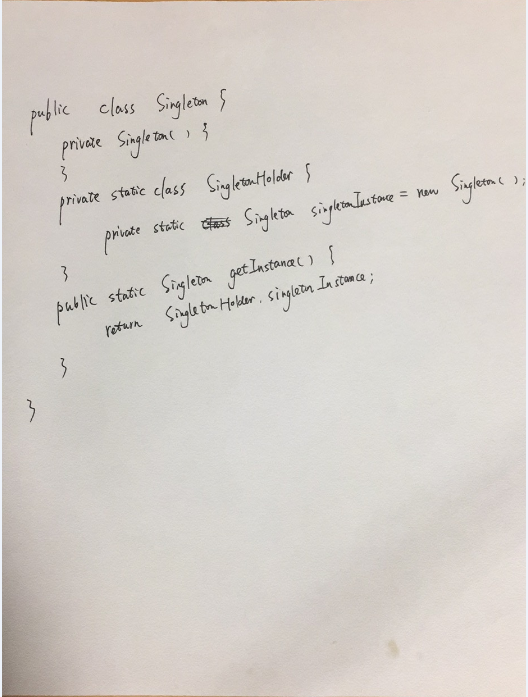
请用组合设计模式编写程序,打印输出图 1 的窗口,窗口组件的树结构如图 2 所示,打印输出示例参考图 3。
组合模式是一种将对象组合成树状结构以表示部分和整体的设计模式,通过组合模式,单个对象和组合对象具有使用上的一致性,组合对象使用起来就跟单个对象一样。
Widget接口里面定义了print方法,子类都使用这个方法打印控件,hasChildWidgets用来区分树状结构中的单个控件和组合控件,如果是组合控件,getChilds会返回包含的子控件。
public interface Widget { String getName(); void addChild(Widget widget); List<Widget> getChilds(); boolean hasChildWidgets(); void print();}
BaseWidget是所有子类的基类,print方法里面定义了打印的行为,先打印自己,然后存在子控件的话,再打印子控件。
public abstract class BaseWidget implements Widget { private String name; public BaseWidget(String name) { this.name = name; } public String getName() { return name; } protected abstract void printSelf(); public void print() { printSelf(); if (hasChildWidgets() && getChilds() != null) { for(Widget child : getChilds()) { child.print(); } } }}
SimpleWidget是单个控件需要继承的类,不包含子控件。
public abstract class SimpleWidget extends BaseWidget { public SimpleWidget(String name) { super(name); } @Override public void addChild(Widget widget) { } @Override public List<Widget> getChilds() { return null; } @Override public boolean hasChildWidgets() { return false; }}
ComplexWidget是组合控件需要继承的类,可以包含子控件。
public abstract class ComplexWidget extends BaseWidget { public ComplexWidget(String name) { super(name); } private List<Widget> childs; @Override public void addChild(Widget widget) { if (childs == null) { childs = new ArrayList<>(); } childs.add(widget); } @Override public List<Widget> getChilds() { return childs; } @Override public boolean hasChildWidgets() { return true; }}
Picture,Button,Label,TextBox,PasswordBox,CheckBox,LinkLabel都继承了SimpleWidget
public class Picture extends SimpleWidget { public Picture(String name) { super(name); } @Override protected void printSelf() { System.out.println("print Picture(" + getName() + ")"); }}
public class Button extends SimpleWidget { public Button(String name) { super(name); } @Override protected void printSelf() { System.out.println("print Button(" + getName() + ")"); }}
public class Label extends SimpleWidget { public Label(String name) { super(name); } @Override protected void printSelf() { System.out.println("print Label(" + getName() + ")"); }}
public class TextBox extends SimpleWidget { public TextBox(String name) { super(name); } @Override protected void printSelf() { System.out.println("print TextBox(" + getName() + ")"); }}
public class PasswordBox extends SimpleWidget { public PasswordBox(String name) { super(name); } @Override protected void printSelf() { System.out.println("print PasswordBox(" + getName() + ")"); }}
public class CheckBox extends SimpleWidget { public CheckBox(String name) { super(name); } @Override protected void printSelf() { System.out.println("print CheckBox(" + getName() + ")"); }}
public class LinkLabel extends SimpleWidget { public LinkLabel(String name) { super(name); } @Override protected void printSelf() { System.out.println("print LinkLabel(" + getName() + ")"); }}
WinForm,Frame都继承了ComplexWidget
public class WinForm extends ComplexWidget { public WinForm(String name) { super(name); } @Override public void printSelf() { System.out.println("print WinForm(" + getName() + ")"); }}
public class Frame extends ComplexWidget { public Frame(String name) { super(name); } @Override protected void printSelf() { System.out.println("print Frame(" + getName() + ")"); }}
创建控件,并组合成树状结构,执行顶层控件的print方法
public class Main { public static void main(String[] args) { Widget window = new WinForm("WINDOW窗口"); window.addChild(new Picture("LOGO图片")); window.addChild(new Button("登录")); window.addChild(new Button("注册")); Widget frame = new Frame("FRAME1"); frame.addChild(new Label("用户名")); frame.addChild(new TextBox("文本框")); frame.addChild(new Label("密码")); frame.addChild(new PasswordBox("密码框")); frame.addChild(new CheckBox("复选框")); frame.addChild(new TextBox("记住用户名")); frame.addChild(new LinkLabel("忘记密码")); window.addChild(frame); window.print(); }}
打印输出如下
print WinForm(WINDOW窗口)print Picture(LOGO图片)print Button(登录)print Button(注册)print Frame(FRAME1)print Label(用户名)print TextBox(文本框)print Label(密码)print PasswordBox(密码框)print CheckBox(复选框)print TextBox(记住用户名)print LinkLabel(忘记密码)
划线
评论
复制
发布于: 2020 年 10 月 02 日阅读数: 41
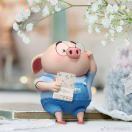
Anyou Liu
关注
还未添加个人签名 2019.05.24 加入
还未添加个人简介
评论