LeetCode 题解:105. 从前序与中序遍历序列构造二叉树,递归 + 数组切割,JavaScript,详细注释
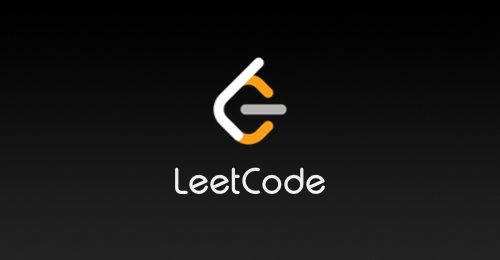
原题连接:https://leetcode-cn.com/problems/construct-binary-tree-from-preorder-and-inorder-traversal/
解题思路:
参考了多种解法,逐渐优化(补充国外大佬解法)和[动画演示 105. 从前序与中序遍历序列构造二叉树](https://leetcode-cn.com/problems/construct-binary-tree-from-preorder-and-inorder-traversal/solution/dong-hua-yan-shi-105-cong-qian-xu-yu-zhong-xu-bian/)。
假设有一个二叉树如下:
前序遍历的结果是: [1,2,4,5,3,6,7]
中序遍历的结果是: [4,2,5,1,6,3,7]
可以将其从根节点 1,拆分成两个子树,如下:
左子树:
前序遍历的结果是: [2,4,5]
中序遍历的结果是: [4,2,5]
右子树:
前序遍历的结果是: [3,6,7]
中序遍历的结果是: [6,3,7]
我们可以按照步骤 3,将左右子树继续按照根节点继续拆分,可以总结出如下规律:
* 二叉树的根节点始终在preorder[0]
* 二叉树可以按照根节点在inorder
中的位置rootIndex
,将preorder
和inorder
拆分成左右两个子树的结果,如下:
左子树遍历结果:
```
preorder = preorder.slice(1, rootIndex + 1);
inorder = inorder.slice(0, rootIndex);
```
右子树遍历结果:
```
preorder = preorder.slice(rootIndex + 1);
inorder = inorder.slice(rootIndex + 1);
```
经过步骤 4 的分析,我们可以按照以下逻辑进行递归:
* 每次递归用preorder[0]
的值生成一个根节点。
* 在通过preorder[0]
找到其在inorder
中的位置rootIndex
,将preorder
和inorder
切割成两部分,分别进行下一层的递归。
* 将下层递归生成的左右子树,连接到当前根节点上。
* 递归完成时将当前根节点返回,供上一层递归连接。
* preorder
和inorder
中的所有值都生成过节点之后,也就完成了整个二叉树的生成。
版权声明: 本文为 InfoQ 作者【Lee Chen】的原创文章。
原文链接:【http://xie.infoq.cn/article/c776ac474f0c82eeb823d6e35】。文章转载请联系作者。
评论