手写单例和组合模式运用实例
发布于: 2020 年 06 月 24 日
手写单例
两种实现方式:
1、懒汉式;
2、饿汉式。
如下图:
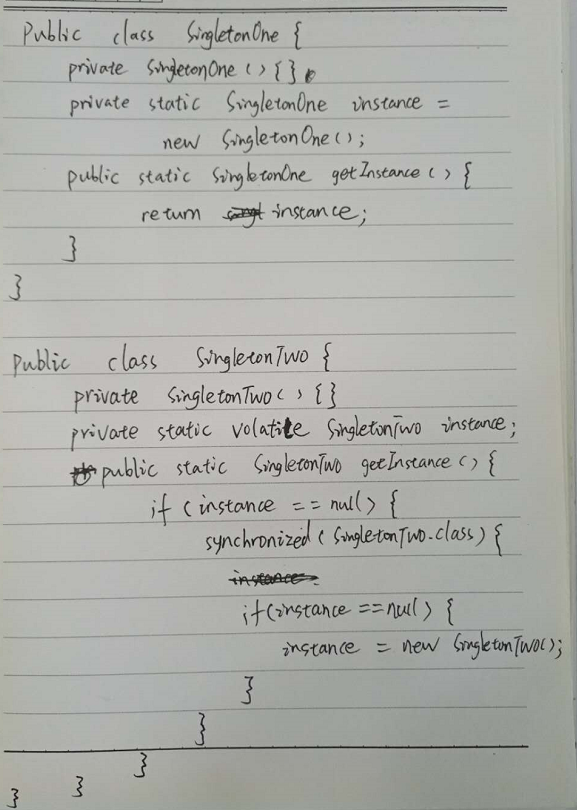
一个组合模式实例
请用组合设计模式编写程序,打印输出图 1 的窗口,窗口组件的树结构如图 2 所示,打印输出示例参考图 3。
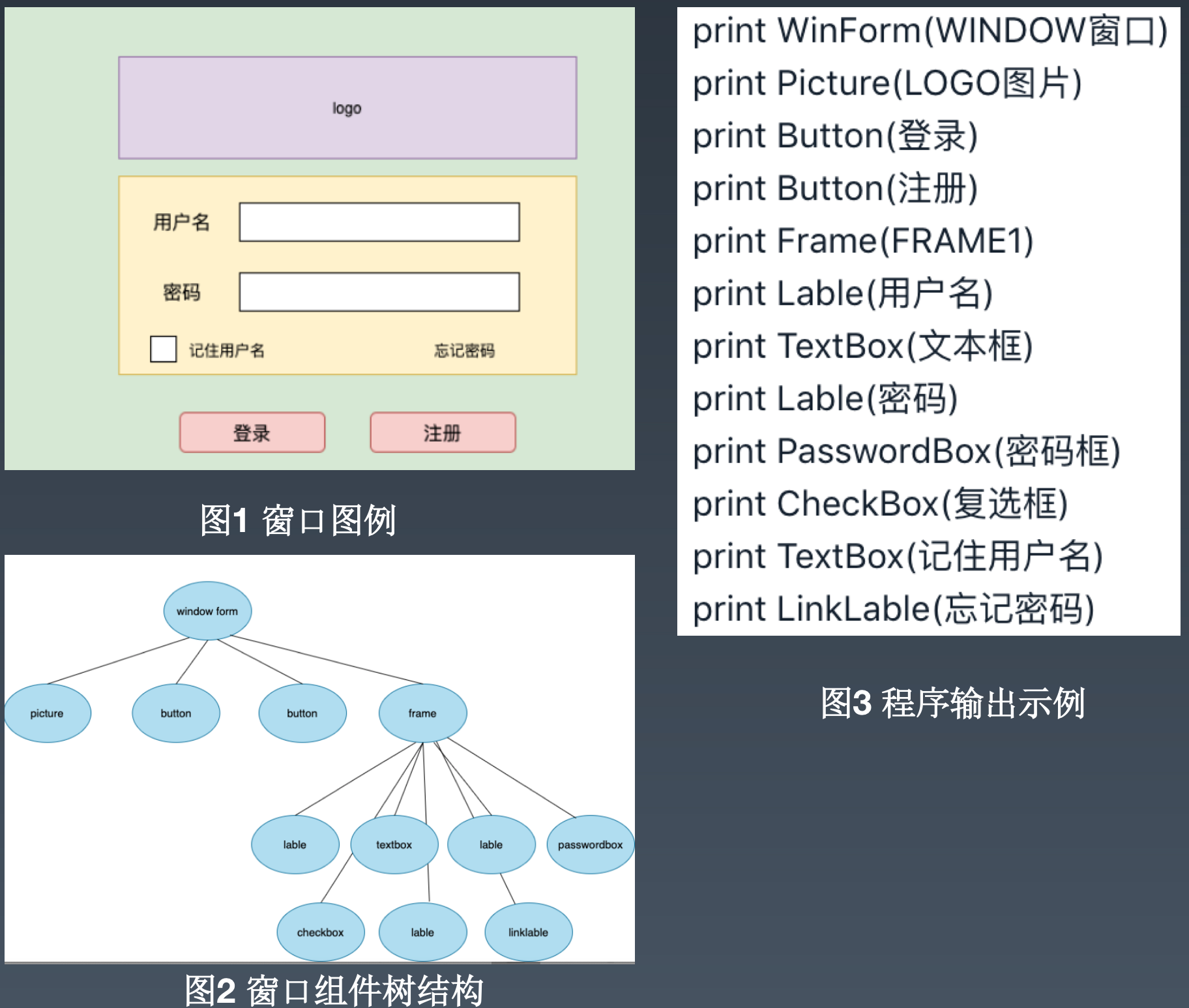
代码实现如下:
1、定义组件抽象
package study;/** * @author linyurong * @date 2020/6/24 9:52 */public interface Component { /** * 打印 */ void print(); /** * 添加子组件 * @param child */ void addChild(Component child); /** * 删除子组件 * @param child */ void removeChild(Component child);}
2、定义一个实现基类:
package study;import java.util.ArrayList;import java.util.List;import java.util.Objects;/** * @author linyurong * @date 2020/6/24 9:55 */public class BaseComponent implements Component { private String name; protected List<Component> childList; public BaseComponent() {} public BaseComponent(String name) { this.name = name; this.childList = new ArrayList<>(16); } @Override public void print() { System.out.printf("print %s(%s)\n", getClass().getSimpleName(), name); for (Component child : childList) { child.print(); } } @Override public void addChild(Component child) { if (Objects.nonNull(child)) { childList.add(child); } } @Override public void removeChild(Component child) { childList.remove(child); } @Override public boolean equals(Object obj) { return super.equals(obj); } @Override public int hashCode() { return super.hashCode(); }}
3、定义出各种组件,继承上述基类,重新构造方法
package study;/** * @author linyurong * @date 2020/6/24 10:01 */public class WinForm extends BaseComponent { public WinForm(String name) { super(name); }}public class Button extends BaseComponent { public Button(String name) { super(name); }}public class CheckBox extends BaseComponent { public CheckBox(String name) { super(name); }}public class Frame extends BaseComponent { public Frame(String name) { super(name); }}public class Label extends BaseComponent { public Label(String name) { super(name); }}public class LinkLabel extends BaseComponent { public LinkLabel(String name) { super(name); }}public class PasswordBox extends BaseComponent { public PasswordBox(String name) { super(name); }}public class Picture extends BaseComponent { public Picture(String name) { super(name); }}public class TextBox extends BaseComponent { public TextBox(String name) { super(name); }}
4、客户端实现类
package study;/** * @author linyurong * @date 2020/6/24 10:02 */public class Main { public static void main(String[] args) { WinForm winForm = new WinForm("WINDOW窗口"); winForm.addChild(new Picture("LOGO图片")); winForm.addChild(new Button("登录")); winForm.addChild(new Button("注册")); Frame frame = new Frame("Frame1"); frame.addChild(new Label("用户名")); frame.addChild(new TextBox("文本框")); frame.addChild(new Label("密码")); frame.addChild(new PasswordBox("密码框")); frame.addChild(new CheckBox("复选框")); frame.addChild(new TextBox("记住用户名")); frame.addChild(new LinkLabel("忘记密码")); winForm.addChild(frame); winForm.print(); }}
5、运行结果
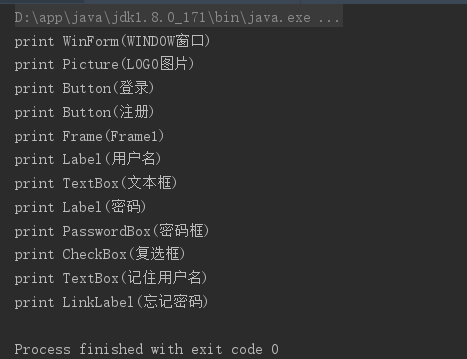
划线
评论
复制
发布于: 2020 年 06 月 24 日阅读数: 74
版权声明: 本文为 InfoQ 作者【林昱榕】的原创文章。
原文链接:【http://xie.infoq.cn/article/c6e65bb3e3be6cb99e6ea5788】。
本文遵守【CC-BY 4.0】协议,转载请保留原文出处及本版权声明。
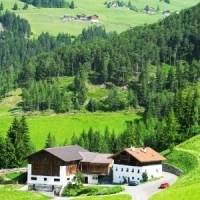
林昱榕
关注
开心生活,努力工作。 2018.02.13 加入
还未添加个人简介
评论