基于 localStorage 实现一个具有过期时间的 DAO 库
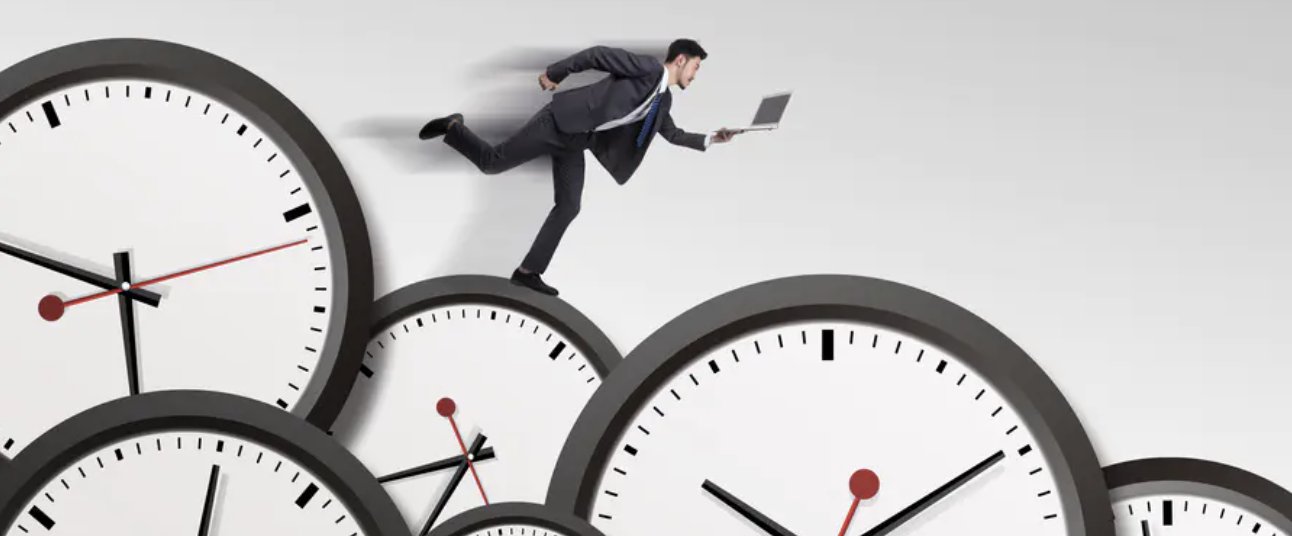
本文主要解决原生 localStorage 无法设置过期时间的问题,并通过封装,来实现一个操作便捷,功能强大的 localStorage 库,关于库封装的一些基本思路和模式,我将采用之前写的如何用不到200行代码写一款属于自己的js类库中类似的方法,感兴趣的朋友可以学习,交流。
设计思路
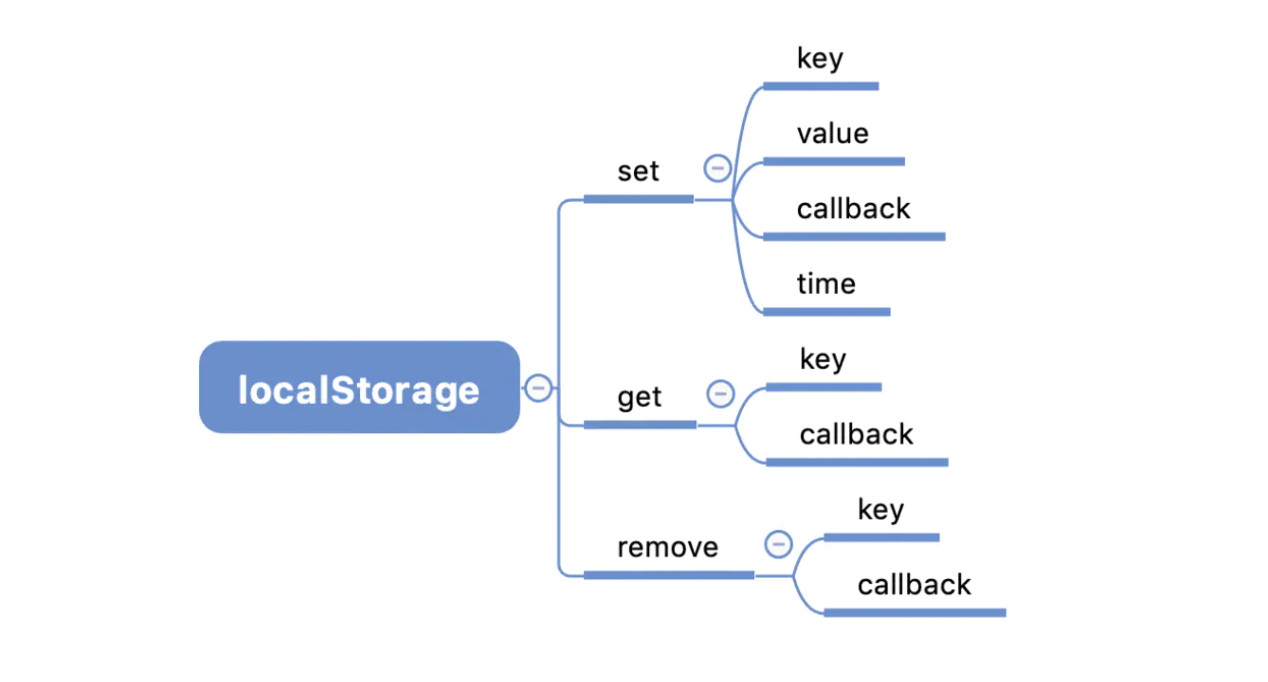
我们将基于 localStorage 原始 api 进行扩展,让其支持失效时间,操作完成后的回调。在文章的最后,我将给出库的完成代码,接下来我们就一步步实现吧。
正文
首先,我们来设计库的基本框架:
如上可以发现,我们的 storage 会有三个核心 api,分别为 set,get,remove,我们使用 localStorage 作为基础库支持,当然你也可以将上面的库换成 sessionStorage 或者其他。
有了基本骨架,我们就可以实现基本功能的封装,这里我们先在原型中加一个属性,来列出数据操作中的各个状态。
为了实现过期时间,我们有两种思路,第一种是先将一个过期时间存到 storage 中,每次操作都检查一遍是否过期,但是这种方案意味着对不同的键就要设置不同的过期时间的 storage 与之对应,这样会占用额外的库内存,维护起来也不方便。另一种方法就是将过期时间存放到键值中,将时间和值通过标识符分隔,每次取的时候从值中截取过期时间,再将真实的值取出来返回,这种方案不会添加额外的键值对存储,维护起来也相对简单,所以我们采用这种方案。为了区分不同的库对象,我们还可以添加键前缀,如下:
基于这个思想,我们就可以接下来的实现了。
getKey——修饰 key 的方法,不影响用户对真实 key 的影响
set 实现
get 实现
remove 实现
在 api 的实现过程中,由于某种误操作很可能导致 storage 报错,所以建议最好用 trycatch 包裹,这样可以避免影响后面的逻辑。
接下来我们可以这么使用:
完整源码
大家也可以基于此扩展更强大的功能,如果有更好的想法,欢迎微信搜索 趣谈前端 和我交流,探讨。
版权声明: 本文为 InfoQ 作者【徐小夕】的原创文章。
原文链接:【http://xie.infoq.cn/article/b79b8a9cfac38472cb3eab5bc】。文章转载请联系作者。
评论