面向对象的设计模式
发布于: 2020 年 06 月 25 日
1.手写单例模式
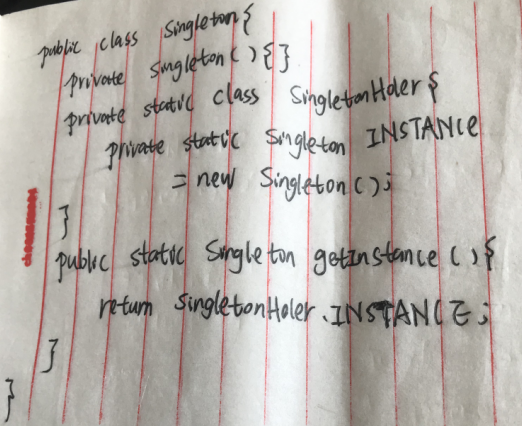
2.组合模式
2.1 自定义组件接口
/** * 自定义组件接口 */public interface Component { /**打印*/ public void printName(); /**添加子组件*/ public void addChild(Component child);}
2.2 按钮组件
import java.util.ArrayList;import java.util.List;import javax.swing.JButton;/** * 按钮组件 * 继承Swing的JButton并实现自定义组件接口 */public class MyJButton extends JButton implements Component{ private static final long serialVersionUID = 1L; private String name = null; List<Component> childComponents = new ArrayList<Component>(); public MyJButton(String printStr,String title){ super(title); this.name = printStr; } public void printName() { // 先把自己输出 System.out.println("print " + name); // 如果还包含其他子组件,那么就输出这些子组件对象 if (null != childComponents) { // 输出当前对象的子组件对象 for (Component component : childComponents) { // 递归地进行子组件相应方法的调用,输出每个子组件对象 component.printName(); } } } @Override public void addChild(Component child) { childComponents.add(child); }}
2.3 复选框组件
import java.util.ArrayList;import java.util.List;import javax.swing.JCheckBox;/** * 复选框组件 * 继承Swing的JCheckBox并实现自定义组件接口 */public class MyJCheckBox extends JCheckBox implements Component{ private static final long serialVersionUID = 1L; private String name = null; List<Component> childComponents = new ArrayList<Component>(); public MyJCheckBox(String printStr,String title){ super(title); this.name = printStr; } public void printName() { // 先把自己输出 System.out.println("print " + name); // 如果还包含其他子组件,那么就输出这些子组件对象 if (null != childComponents) { // 输出当前对象的子组件对象 for (Component component : childComponents) { // 递归地进行子组件相应方法的调用,输出每个子组件对象 component.printName(); } } } @Override public void addChild(Component child) { childComponents.add(child); }}
2.4 Frame组件
import java.util.ArrayList;import java.util.List;import javax.swing.JFrame;/** * Frame组件 * 继承Swing的JFrame并实现自定义组件接口 */public class MyJFrame extends JFrame implements Component{ private static final long serialVersionUID = 1L; private String name = null; private String position = null; List<Component> childComponents = new ArrayList<Component>(); public MyJFrame(String printStr,String title){ super(title); this.name = printStr; } public void printName() { if(name != null){ // 先把自己输出 System.out.println("print " + name); } // 如果还包含其他子组件,那么就输出这些子组件对象 if (null != childComponents) { // 输出当前对象的子组件对象 for (Component component : childComponents) { // 递归地进行子组件相应方法的调用,输出每个子组件对象 component.printName(); } } } @Override public void setVisible(boolean b) { super.setVisible(b); this.printName(); } @Override public void addChild(Component child) { super.add((java.awt.Component)child,position); childComponents.add(child); } public void addChildren(Component child,String position) { this.position = position; addChild(child); }}
2.5 Label组件
import java.util.ArrayList;import java.util.List;import javax.swing.ImageIcon;import javax.swing.JLabel;/** * Label组件 * 继承Swing的JLabel并实现自定义组件接口 */public class MyJLabel extends JLabel implements Component{ private static final long serialVersionUID = 1L; private String name = null; List<Component> childComponents = new ArrayList<Component>(); public MyJLabel(String printStr,String title){ super(title); this.name = printStr; } public MyJLabel(String printStr,ImageIcon imageIcon) { super(imageIcon); this.name = printStr; } public void printName() { // 先把自己输出 System.out.println("print " + name); // 如果还包含其他子组件,那么就输出这些子组件对象 if (null != childComponents) { // 输出当前对象的子组件对象 for (Component component : childComponents) { // 递归地进行子组件相应方法的调用,输出每个子组件对象 component.printName(); } } } @Override public void addChild(Component child) { childComponents.add(child); }}
2.6 panel组件
import java.util.ArrayList;import java.util.List;import javax.swing.JPanel;/** * panel组件 * 继承Swing的JPanel并实现自定义组件接口 */public class MyJPanel extends JPanel implements Component{ private static final long serialVersionUID = 1L; private String name = null; List<Component> childComponents = new ArrayList<Component>(); public MyJPanel(){ super(); } public MyJPanel(String printStr){ super(); this.name = printStr; } public void printName() { if(name != null){ // 先把自己输出 System.out.println("print " + name); } // 如果还包含其他子组件,那么就输出这些子组件对象 if (null != childComponents) { // 输出当前对象的子组件对象 for (Component component : childComponents) { // 递归地进行子组件相应方法的调用,输出每个子组件对象 component.printName(); } } } @Override public void addChild(Component child) { super.add((java.awt.Component)child); childComponents.add(child); }}
2.7 PasswordField组件
import java.util.ArrayList;import java.util.List;import javax.swing.JPasswordField;/** * PasswordField组件 * 继承Swing的JPasswordField并实现自定义组件接口 */public class MyJPasswordField extends JPasswordField implements Component{ private static final long serialVersionUID = 1L; private String name = ""; List<Component> childComponents = new ArrayList<Component>(); public MyJPasswordField(String printStr){ super(18); this.name = printStr; } public void printName() { // 先把自己输出 System.out.println("print " + name); // 如果还包含其他子组件,那么就输出这些子组件对象 if (null != childComponents) { // 输出当前对象的子组件对象 for (Component component : childComponents) { // 递归地进行子组件相应方法的调用,输出每个子组件对象 component.printName(); } } } @Override public void addChild(Component child) { childComponents.add(child); }}
2.8 TextField组件
import java.util.ArrayList;import java.util.List;import javax.swing.JTextField;/** * TextField组件 * 继承Swing的JTextField并实现自定义组件接口 */public class MyJTextField extends JTextField implements Component{ private static final long serialVersionUID = 1L; private String name; List<Component> childComponents = new ArrayList<Component>(); public MyJTextField(String printStr){ super(18); this.name = printStr; } public void printName() { // 先把自己输出 System.out.println("print " + name); // 如果还包含其他子组件,那么就输出这些子组件对象 if (null != childComponents) { // 输出当前对象的子组件对象 for (Component component : childComponents) { // 递归地进行子组件相应方法的调用,输出每个子组件对象 component.printName(); } } } @Override public void addChild(Component child) { childComponents.add(child); }}
2.9 生成窗口并打印组件信息
public class Client { public static void main(String[] args){ MyJFrame jf = new MyJFrame("WindowForm(WINDOS窗口)","测试窗口"); jf.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE); jf.setSize(300, 200); MyJPanel p3 = new MyJPanel(); MyJLabel jLabel = new MyJLabel("picture(LOGO图片)",new ImageIcon("E:\\1.png")); MyJPanel p1 = new MyJPanel("Frame(FRAME1)"); MyJLabel label1 = new MyJLabel("Label(用户名)", "用户名"); MyJTextField text1 = new MyJTextField("Textbox(文本框)"); MyJLabel label2 = new MyJLabel("Label(密码)", "密 码"); MyJPasswordField text2 = new MyJPasswordField("Passwordbox(密码框)"); MyJCheckBox jCheckBox = new MyJCheckBox("Textbox(记住用户名)","记住用户名"); MyJLabel label3 = new MyJLabel("Linklabel(忘记密码)", "忘记密码"); MyJPanel p2 = new MyJPanel(); MyJButton login = new MyJButton("Button(登录)", "登录"); MyJButton reg = new MyJButton("Button(注册)", "注册"); p1.addChild(label1); p1.addChild(text1); p1.addChild(label2); p1.addChild(text2); p1.addChild(jCheckBox); p1.addChild(label3); p2.addChild(login); p2.addChild(reg); p3.addChild(jLabel); jf.addChildren(p3,BorderLayout.NORTH); jf.addChildren(p1,BorderLayout.CENTER); jf.addChildren(p2,BorderLayout.SOUTH); jf.setVisible(true); }}
划线
评论
复制
发布于: 2020 年 06 月 25 日 阅读数: 24
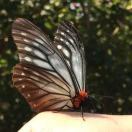
WW
关注
还未添加个人签名 2019.06.04 加入
还未添加个人简介
评论