架构师训练营第三周课后作业
发布于: 2020 年 06 月 25 日
作业一:
请在草稿纸上手写一个单例模式的实现代码,拍照提交作业。
单例模式 延迟初始化
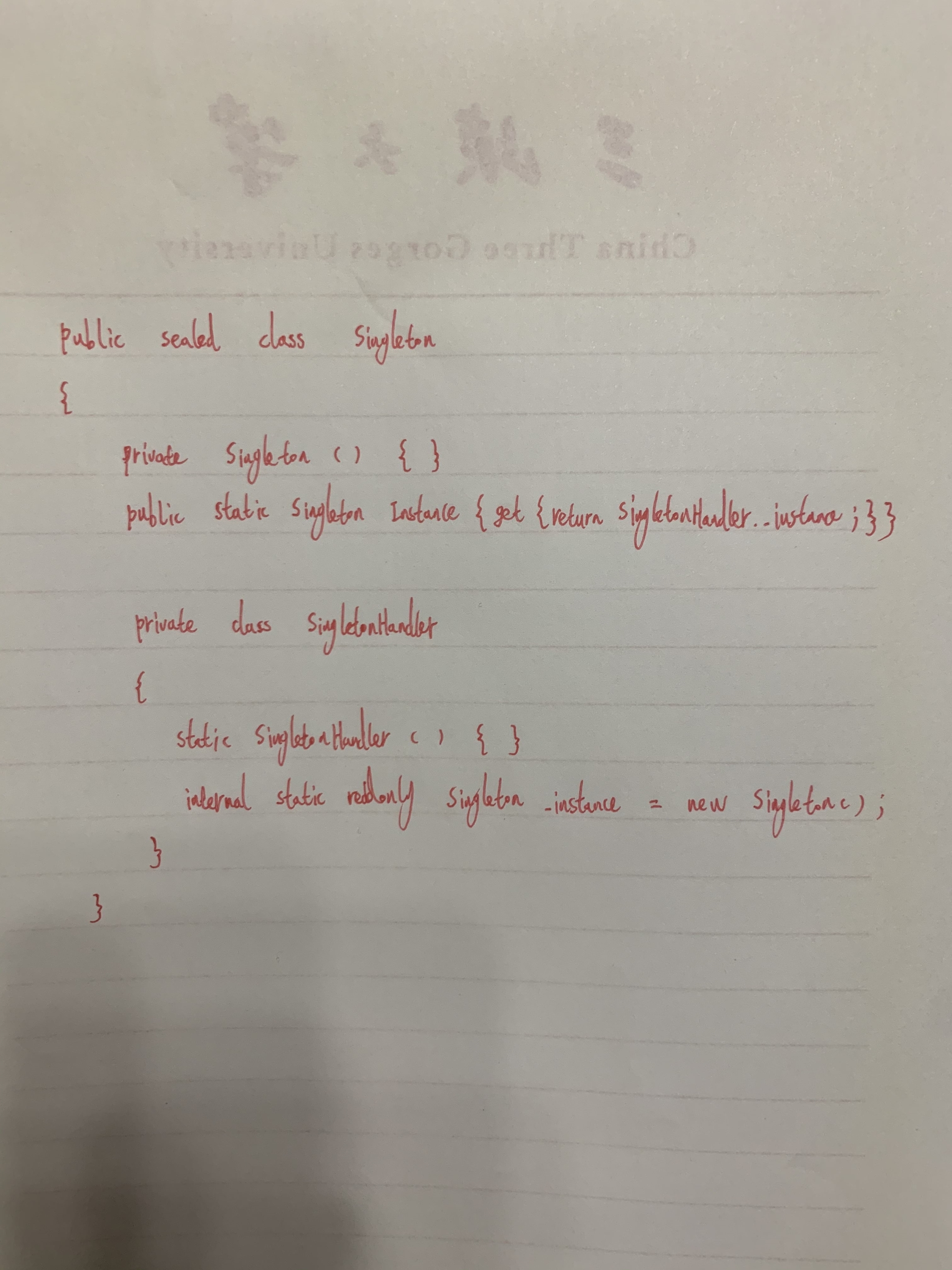
请用组合设计模式编写程序,打印输出图 1 的窗口,窗口组件的树结构如图 2 所示,打印输出示例参考图 3。
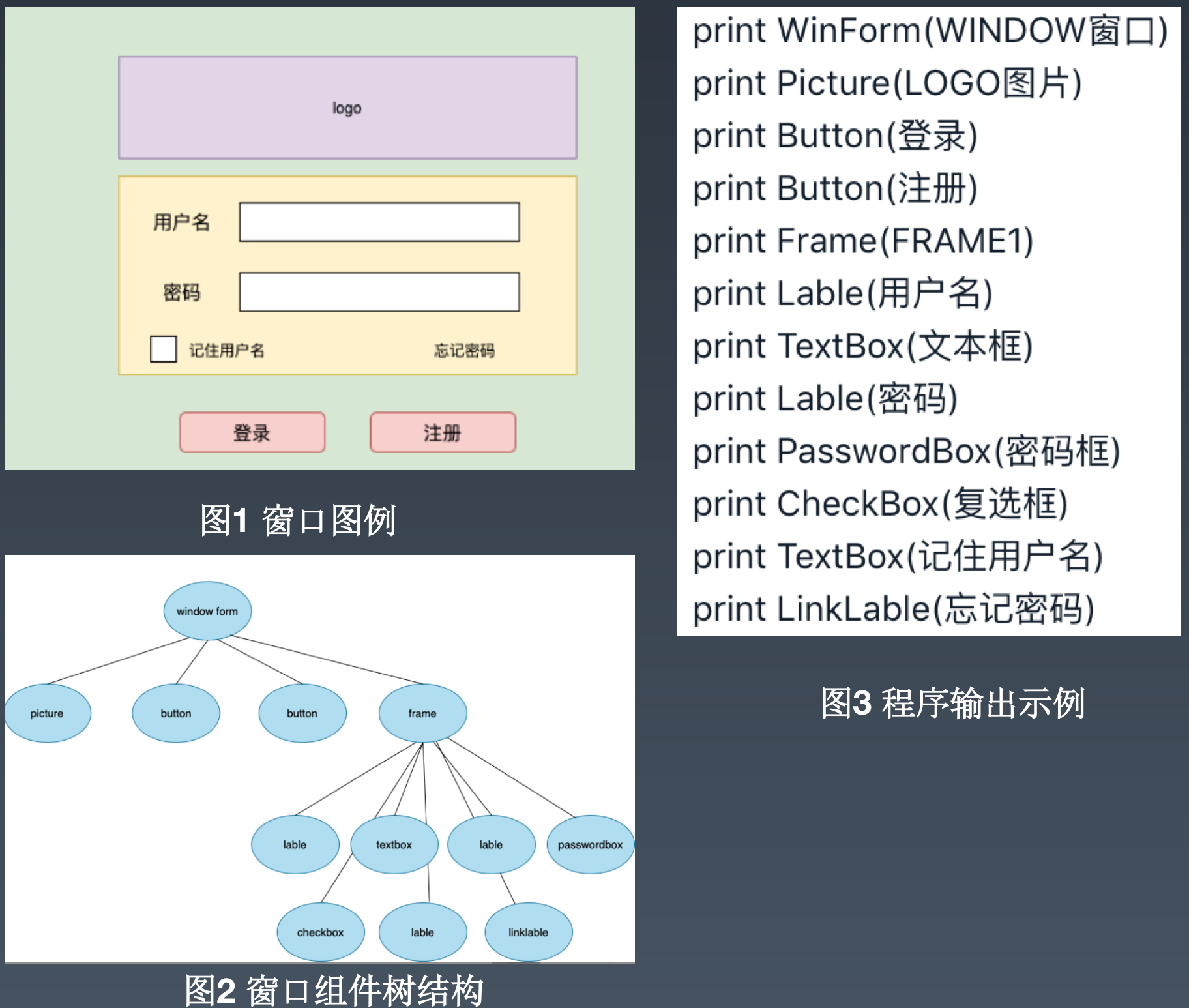
using System;using System.Collections.Generic;namespace ConsoleApp1{ class Program { static void Main(string[] args) { WinForm component = new WinForm("WINDOWS窗口"); component.Add(new Picture("LOGO图片")); component.Add(new Button("登录")); component.Add(new Button("注册")); Container frame = new Frame("FRAME1"); frame.Add(new Label("用户名")); frame.Add(new TextBox("文本框")); frame.Add(new Label("密码")); frame.Add(new PasswordBox("密码框")); frame.Add(new CheckBox("复选框")); frame.Add(new Label("记住用户名")); frame.Add(new LinkLable("忘记密码")); component.Add(frame); component.Print(); Console.ReadKey(); } } public abstract class Component { protected string Name; protected Component(string name) { this.Name = name; } public abstract void Print(); } public abstract class Container : Component { private readonly IList<Component> _childenComponents = new List<Component>(); protected Container(string name) : base(name) { } public void Add(Component component) { this._childenComponents.Add(component); } public override void Print() { foreach (var item in _childenComponents) { item.Print(); } } } public class WinForm : Container { public WinForm(string name) : base(name) { } public override void Print() { Console.WriteLine($"print WinForm({this.Name})"); base.Print(); } } public class Frame : Container { public Frame(string name) : base(name) { } public override void Print() { Console.WriteLine($"print Frame({this.Name})"); base.Print(); } } public class Picture : Component { public Picture(string name) : base(name) { } public override void Print() { Console.WriteLine($"print Picture({this.Name})"); } } public class Button : Component { public Button(string name) : base(name) { } public override void Print() { Console.WriteLine($"print Button({this.Name})"); } } public class Label : Component { public Label(string name) : base(name) { } public override void Print() { Console.WriteLine($"print Label({this.Name})"); } } public class TextBox : Component { public TextBox(string name) : base(name) { } public override void Print() { Console.WriteLine($"print TextBox({this.Name})"); } } public class PasswordBox : Component { public PasswordBox(string name) : base(name) { } public override void Print() { Console.WriteLine($"print PasswordBox({this.Name})"); } } public class CheckBox : Component { public CheckBox(string name) : base(name) { } public override void Print() { Console.WriteLine($"print CheckBox({this.Name})"); } } public class LinkLable : Component { public LinkLable(string name) : base(name) { } public override void Print() { Console.WriteLine($"print LinkLable({this.Name})"); } }}
运行结果:
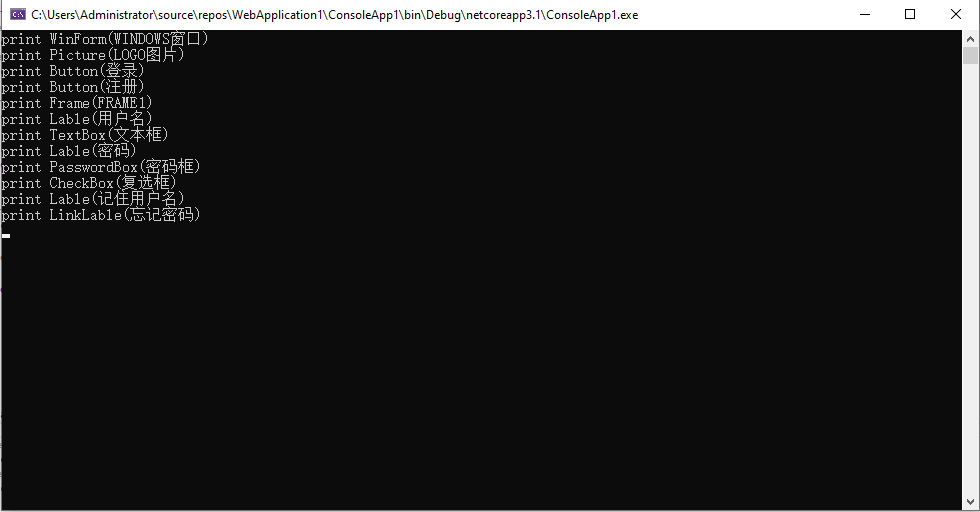
Java版:
package com.example.thirdweek;import java.util.ArrayList;import java.util.List;public class Main { public static void main(String[] args) { WinForm component = new WinForm("WINFORM窗口"); component.addComponent(new Picture("LOGO图片")); component.addComponent(new Button("登录")); component.addComponent(new Button("注册")); Container frame = new Frame("FRAME1"); frame.addComponent(new Label("用户名")); frame.addComponent(new TextBox("文本框")); frame.addComponent(new Label("密码")); frame.addComponent(new PasswordBox("密码框")); frame.addComponent(new CheckBox("复选框")); frame.addComponent(new Label("记住用户名")); frame.addComponent(new LinkLable("忘记密码")); component.addComponent(frame); component.print(); } public static abstract class Component { protected String name; protected Component(String name) { this.name = name; } public abstract void print(); } public static abstract class Container extends Component { private List<Component> childenComponents; protected Container(String name) { super(name); this.childenComponents = new ArrayList<Component>(); } public void addComponent(Component component) { this.childenComponents.add(component); } @Override public void print() { for (Component component : this.childenComponents) { component.print(); } } } public static class WinForm extends Container { public WinForm(String name) { super(name); } @Override public void print() { System.out.println(String.format("print WinForm(%S)", this.name)); super.print(); } } public static class Frame extends Container { public Frame(String name) { super(name); } @Override public void print() { System.out.println(String.format("print Frame(%S)", this.name)); super.print(); } } public static class Picture extends Component { public Picture(String name) { super(name); } @Override public void print() { System.out.println(String.format("print Picture(%S)", this.name)); } } public static class Button extends Component { public Button(String name) { super(name); } @Override public void print() { System.out.println(String.format("print Button(%S)", this.name)); } } public static class Label extends Component { public Label(String name) { super(name); } @Override public void print() { System.out.println(String.format("print Label(%S)", this.name)); } } public static class TextBox extends Component { public TextBox(String name) { super(name); } @Override public void print() { System.out.println(String.format("print TextBox(%S)", this.name)); } } public static class PasswordBox extends Component { public PasswordBox(String name) { super(name); } @Override public void print() { System.out.println(String.format("print PasswordBox(%S)", this.name)); } } public static class CheckBox extends Component { public CheckBox(String name) { super(name); } @Override public void print() { System.out.println(String.format("print CheckBox(%S)", this.name)); } } public static class LinkLable extends Component { public LinkLable(String name) { super(name); } @Override public void print() { System.out.println(String.format("print LinkLable(%S)", this.name)); } }}
运行结果:
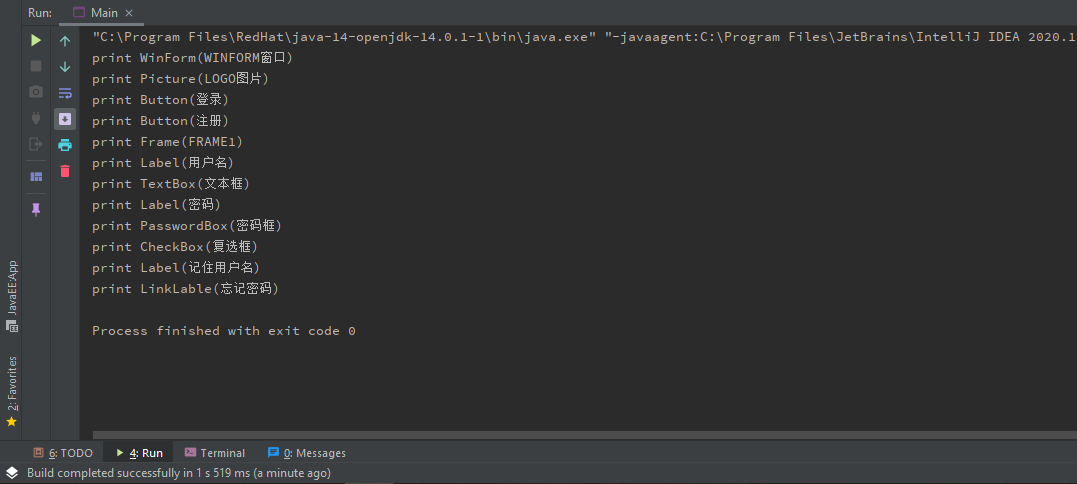
划线
评论
复制
发布于: 2020 年 06 月 25 日 阅读数: 42
版权声明: 本文为 InfoQ 作者【赵凯】的原创文章。
原文链接:【http://xie.infoq.cn/article/ababdacdbd14ba31b2735d357】。未经作者许可,禁止转载。
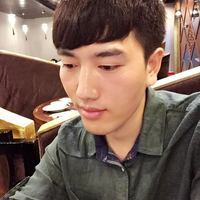
赵凯
关注
还未添加个人签名 2017.12.07 加入
还未添加个人简介
评论