架构师训练营 week3
发布于: 2020 年 06 月 24 日
1. 请在草稿纸上手写一个单例模式的实现代码,拍照提交作业。
采用懒加载的方式初始化
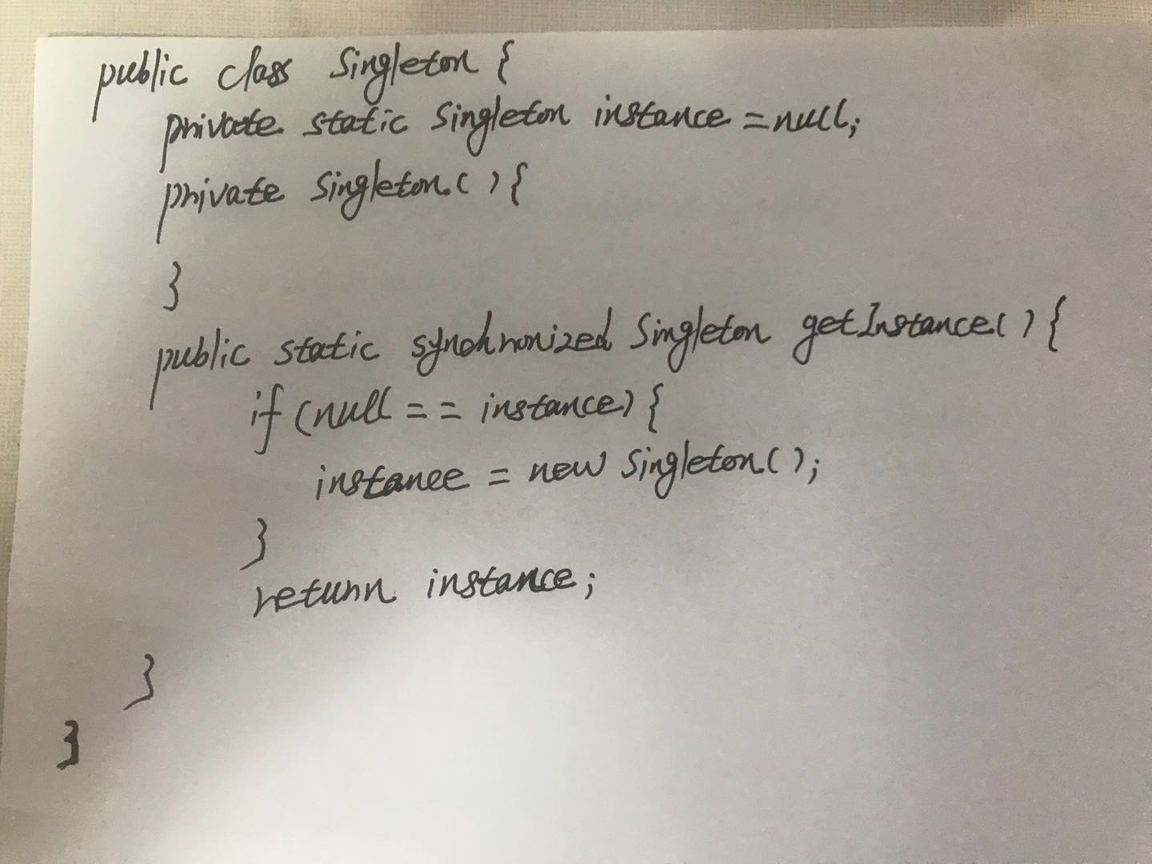
2. 请用组合设计模式编写程序,打印输出图 1 的窗口,窗口组件的树结构如图 2 所示,打印输出示例参考图 3。
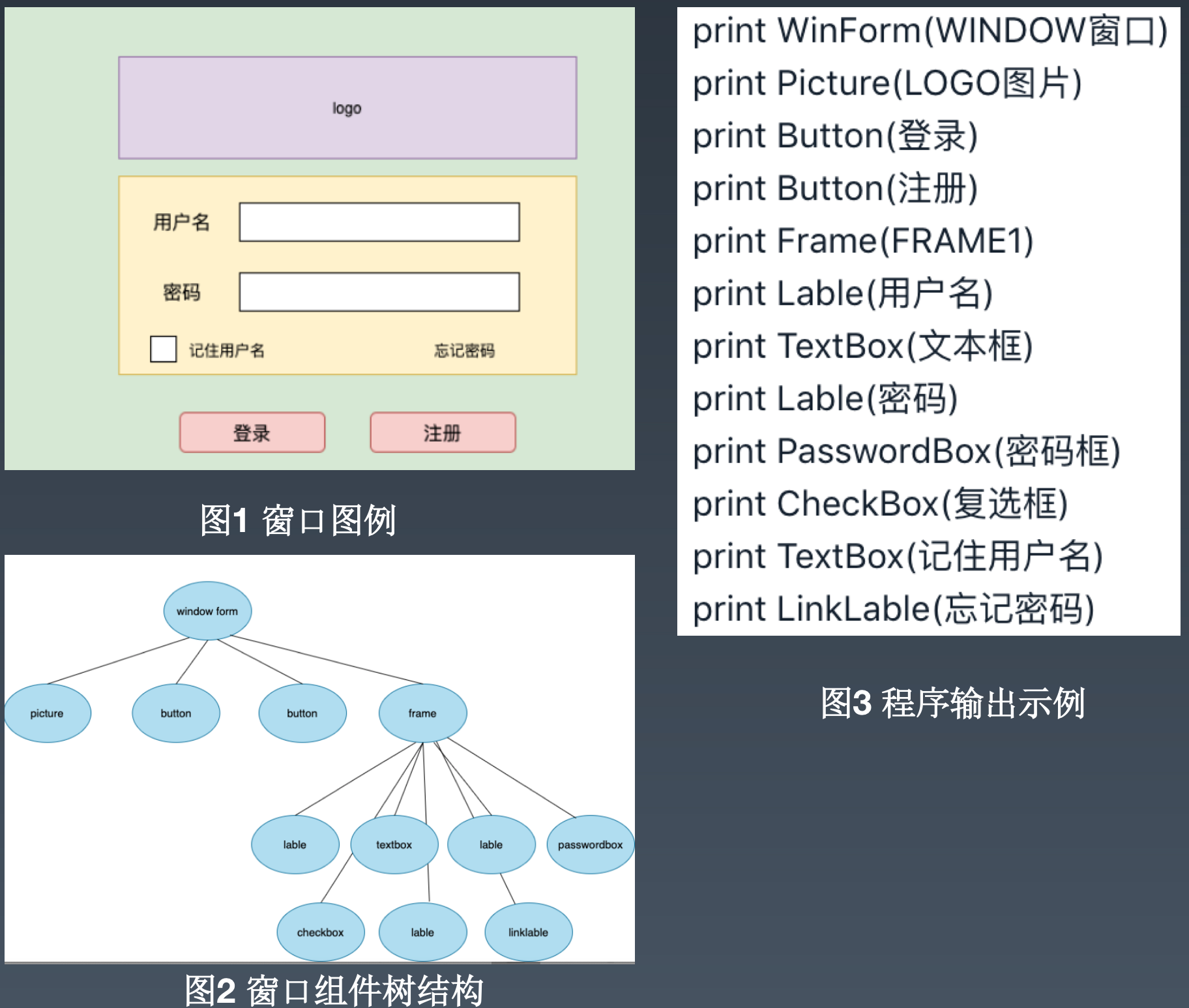
本次采用Java语言实现:
定义一个公共操作接口类:
public interface IPrint { void print();}
定义Component类,实现IPrint接口
public class Component implements IPrint { private String name; public Component(String name) { this.name = name; } public String getName() { return this.name; } @Override public void print() { System.out.println("print " + this.getClass().getSimpleName() + "(" + this.getName() + ")"); } public void add(Component c) { throw new UnsupportedOperationException("不支持添加组件"); } public void remove(Component c) { throw new UnsupportedOperationException("不支持删除组件"); }}
定义Frame容器类,可以加入子Component
import java.util.ArrayList;import java.util.List;public class Frame extends Component { private List<Component> childs; public Frame(String name) { super(name); this.childs = new ArrayList<>(); } @Override public void add(Component c) { this.childs.add(c); } @Override public void remove(Component c) { this.childs.remove(c); } @Override public void print() { System.out.println("print " + this.getClass().getSimpleName() + "(" + this.getName() + ")"); for (Component c : this.childs) { c.print(); } }}
定义Component的子类Button
public class Button extends Component { public Button(String name) { super(name); }}
定义Component的子类CheckBox
public class CheckBox extends Component { public CheckBox(String name) { super(name); }}
定义Component的子类Label
public class Label extends Component { public Label(String name) { super(name); }}
定义Component的子类LinkLabel
public class LinkLabel extends Component { public LinkLabel(String name) { super(name); }}
定义Component的子类PasswordBox
public class PasswordBox extends Component { public PasswordBox(String name) { super(name); }}
定义Component的子类Picture
public class Picture extends Component { public Picture(String name) { super(name); }}
定义Component的子类Textbox
public class Textbox extends Component { public Textbox(String name) { super(name); }}
定义Frame的子类WinForm
public class WinForm extends Frame { public WinForm(String name) { super(name); }}
主程序入口Main
public class Main { public static void main(String[] args) { WinForm form = new WinForm("WINDOW窗体"); Picture pic = new Picture("LOGO图片"); form.add(pic); Button btn1 = new Button("登录"); form.add(btn1); Button btn2 = new Button("注册"); form.add(btn2); Frame frame = new Frame("FRAME1"); Label nameLabel = new Label("用户名"); frame.add(nameLabel); Textbox textbox = new Textbox("文本框"); frame.add(textbox); Label pwdLabel = new Label("密码"); frame.add(pwdLabel); PasswordBox passwordBox = new PasswordBox("密码框"); frame.add(passwordBox); CheckBox checkBox = new CheckBox("复选框"); frame.add(checkBox); Textbox rememberTextbox = new Textbox("记住用户名"); frame.add(rememberTextbox); LinkLabel linkLabel = new LinkLabel("忘记密码"); frame.add(linkLabel); form.add(frame); form.print(); }}
程序最后的输出结果:
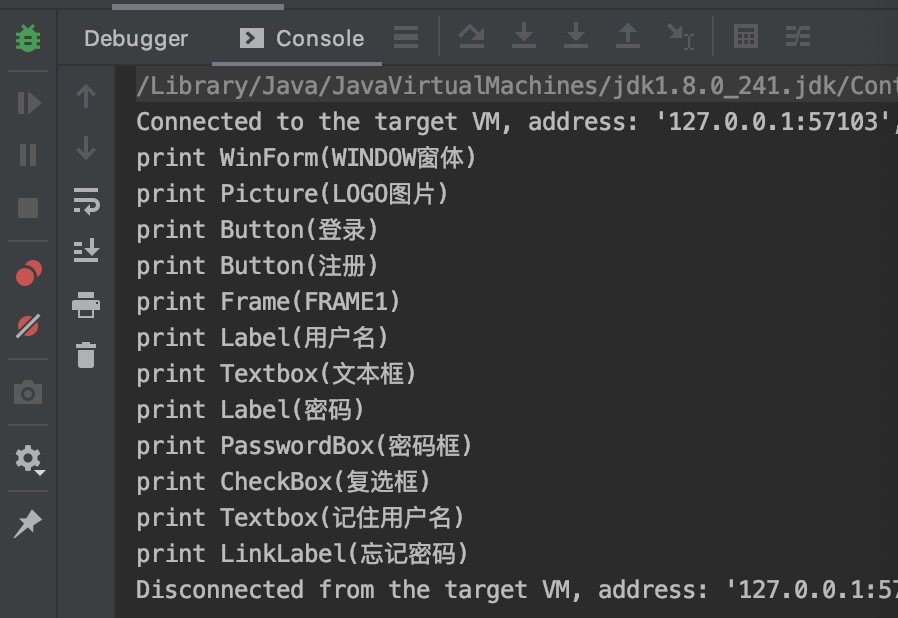
划线
评论
复制
发布于: 2020 年 06 月 24 日阅读数: 48
devfan
关注
还未添加个人签名 2017.11.12 加入
还未添加个人简介
评论