设计模式示例
单例设计模式
Singleton保证一个类产生一个实例。带来好处:
l 单一实例,减少实例频繁创建和销毁带来的资源销毁。
l 多个用户使用该实例,便于统一控制。
饿汉单例实例特点:
l 构造函数私有;
l 通过私有静态成员变量创建;
l 通过公有静态方法返回该实例对象;
懒汉单例实例特点(性能稍差,推荐饿汉单例模式):
l 构造函数私有;
l 通过私有静态成员变量创建,但为null;
l 通过公有静态方法返回该实例对象,如果实例=null,创建并返回(该方法注意加锁);
下图是饿汉单例模式实现
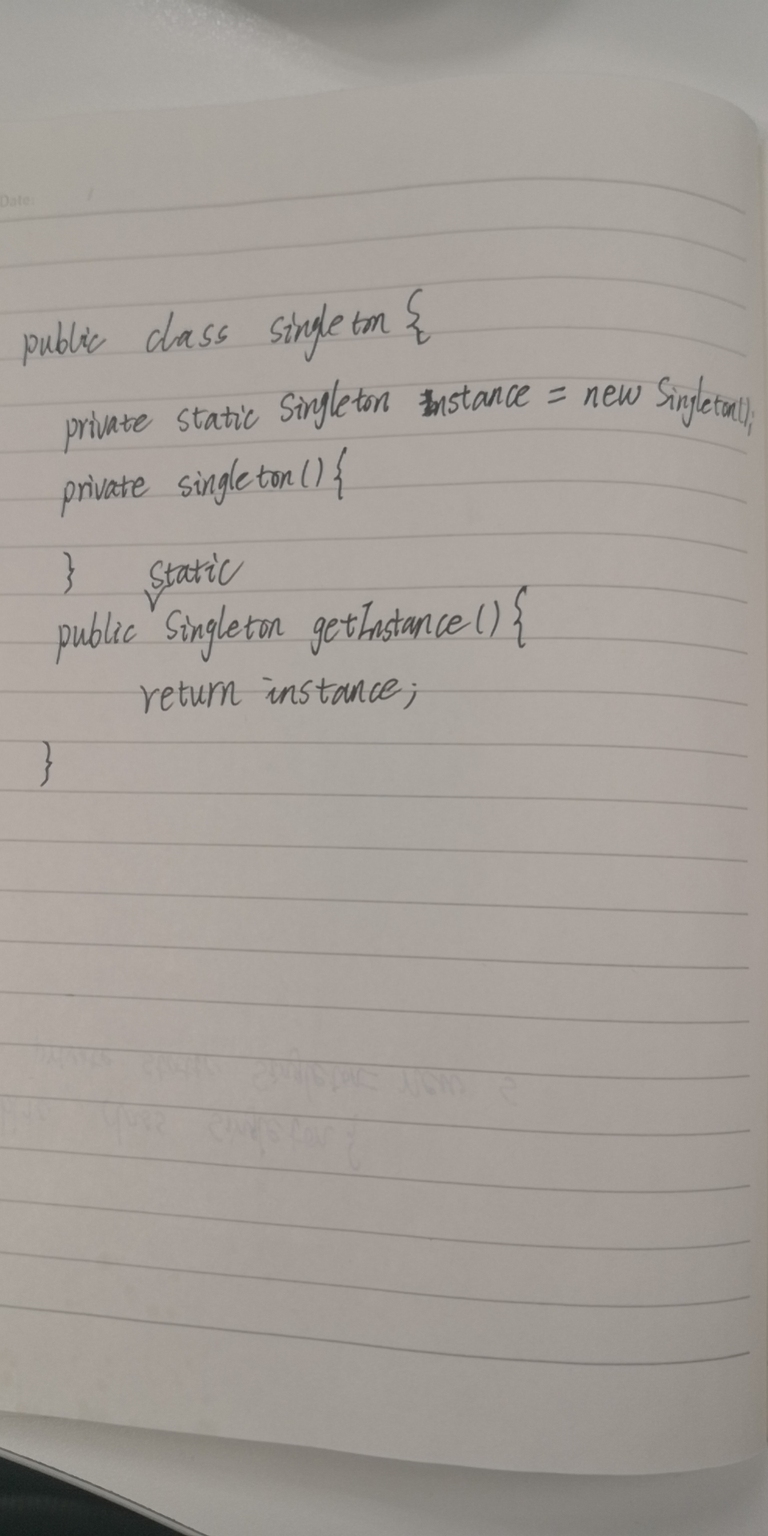
组合设计模式
组合模式是一种对象的结构模式,用于把一组相似的对象当做一个单一的对象,用来表示部分及整体的关系。
使用场景:描述部分及整体层次结构,例如:树形结构;忽略单个对象和组合对象之间区别,统一使用组合对象中的所有对象。
优点:1.高层调用低层。2.节点自由增肌。
缺点:使用组合模式时,部分和整体的声明都是实现类,非接口,违反依赖倒置。
下面我们使用组合设计模式编写代码,打印输出图 1 的窗口,窗口组件的树结构如图 2 所示,打印输出示例参考图 3。
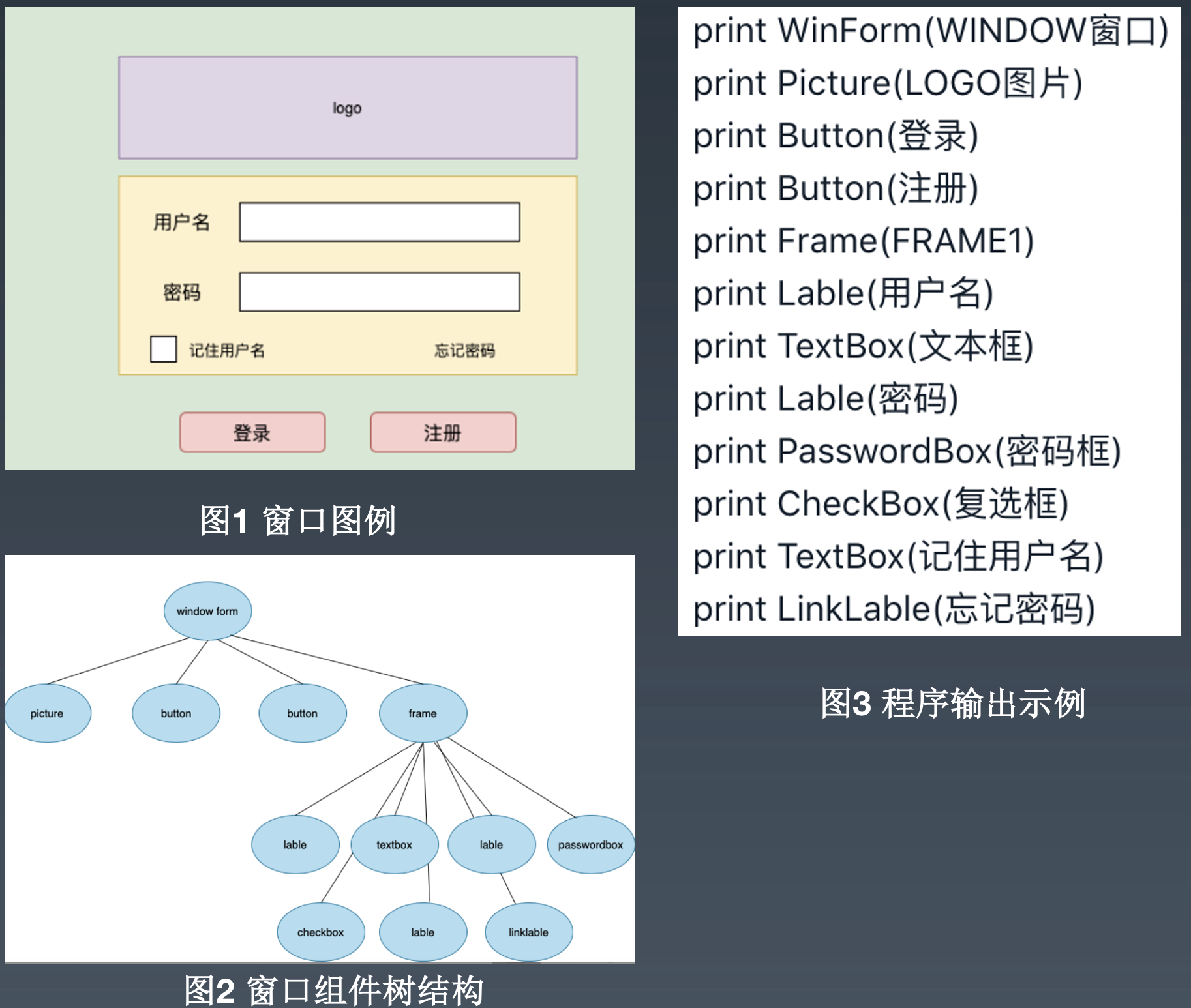
1.组件抽象类
2.容器类
3.组件元素类
4.main类
版权声明: 本文为 InfoQ 作者【Mars】的原创文章。
原文链接:【http://xie.infoq.cn/article/9da886f5a0c35eeb8c5492d4b】。文章转载请联系作者。
评论