代码重构 (作业)
发布于: 2020 年 10 月 03 日
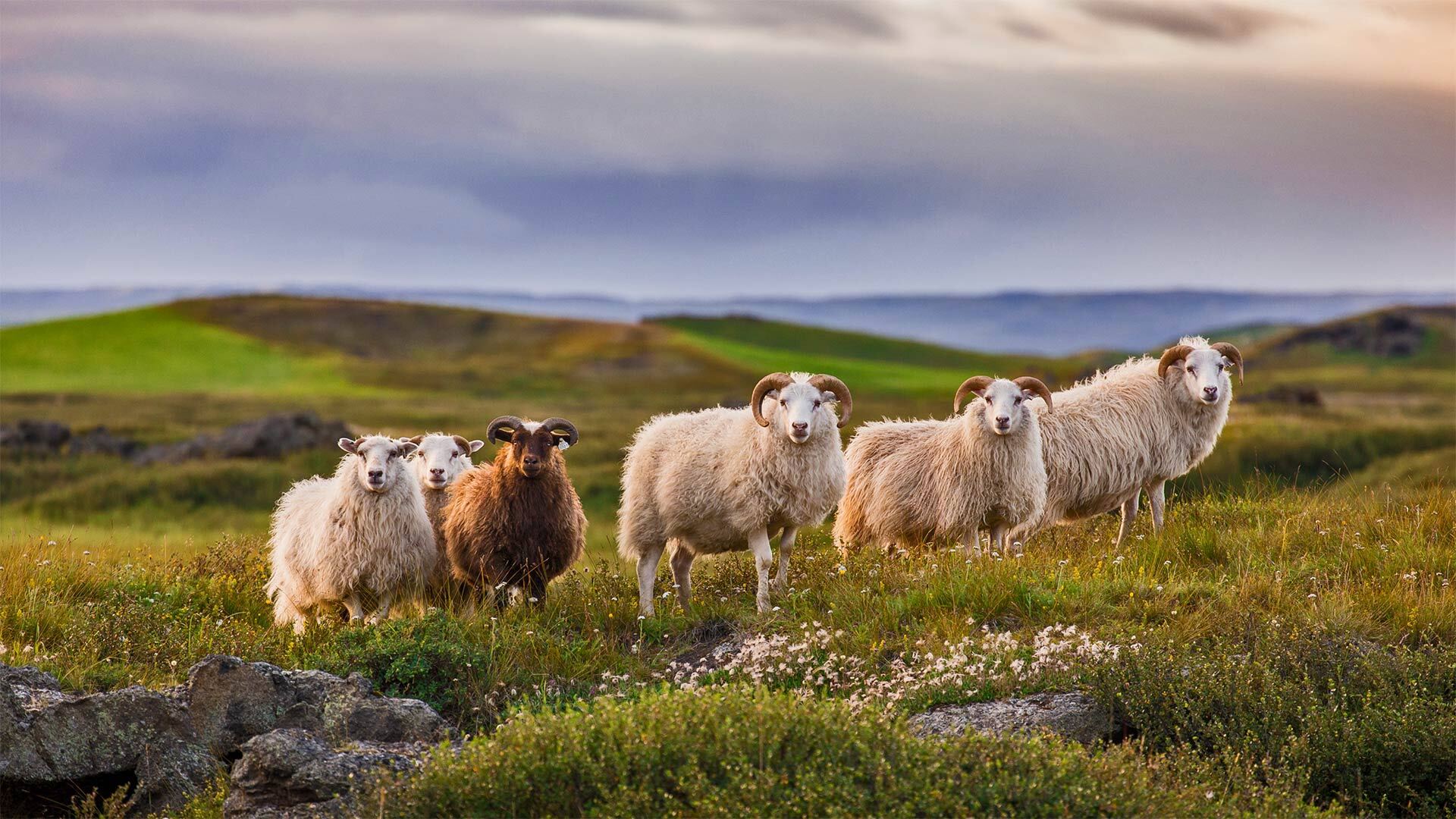
一、 手写一个单例模式的实现代码 ( 拍照提交作业 )
饿汉式加载
public class Singleton { private Singleton() {} private final static Singleton instance = new Singleton(); public static Singleton getInstance() { return instance; }}
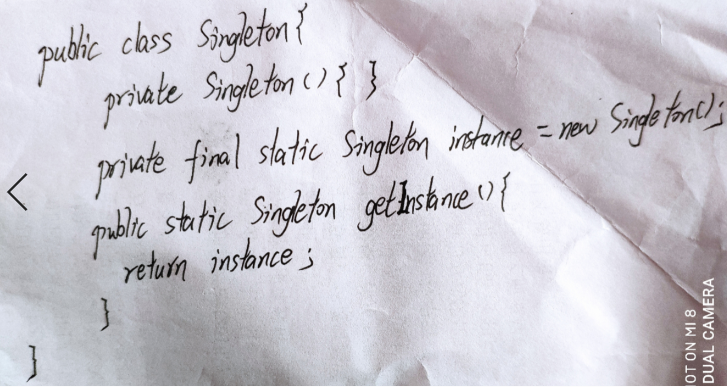
懒汉式加载
public class Singleton { private Singleton() { } private static volatile Singleton instance = null; public static Singleton getInstance() { if (instance == null) { synchronized (instance) { if (instance == null) { instance = new Singleton(); } } } return instance; }}
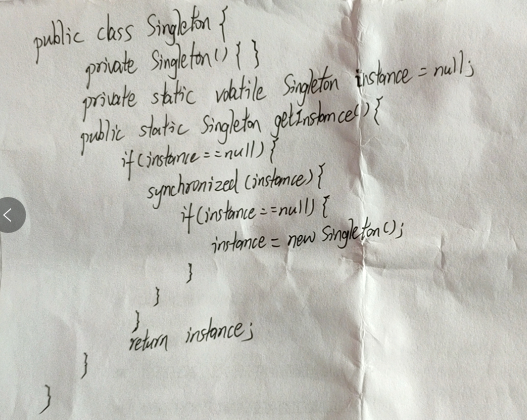
二、使用组合设计模式编写程序,打印输出图1窗口,窗口组件的树结构如图2,打印输出如图3
图1
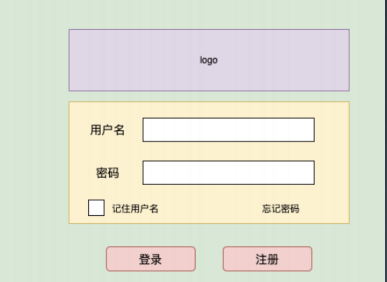
图2
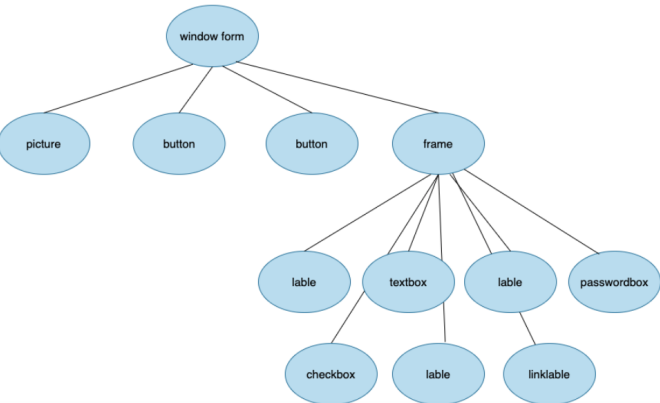
图3
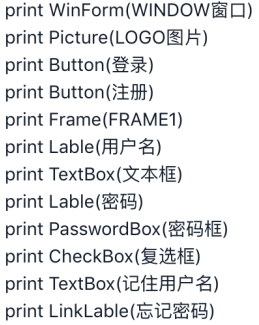
代码实现:
定义组件的基类Component
public class Component { private String text; public Component(String text){ this.text=text; } public void draw(){ System.out.println("print "+this.getClass().getSimpleName()+"("+this.text+")"); }}
定义组件容器的基类Container,容器也属于组件
public class Container extends Component { List<Component> components = null; public Container(String text) { super(text); components = new ArrayList<>(); } @Override public void draw() { super.draw(); int size = components.size(); for (int i = 0; i < size; i++) { components.get(i).draw(); } } public void addComponent(Component component) { this.components.add(component); }}
定义具体组件类Button、Label、CheckBox等,以Button类为例,其他都一样
public class Button extends Component { public Button(String text) { super(text); }}
定义具体容器类WinForm、Frame,以WinForm为例
public class WinForm extends Container{ public WinForm(String text) { super(text); }}
编写测试代码如下
public class MainPage { public static void main(String[] args) { Container winForm = new WinForm("WINDOW窗口"); winForm.addComponent(new Picture("LOGO图片")); winForm.addComponent(new Button("登录")); winForm.addComponent(new Button("注册")); Frame frame = new Frame("FRAME1"); frame.addComponent(new Label("用户名")); frame.addComponent(new TextBox("文本框")); frame.addComponent(new Label("密码")); frame.addComponent(new PasswordBox("密码框")); frame.addComponent(new CheckBox("复选框")); frame.addComponent(new Label("记住用户名")); frame.addComponent(new Linkable("忘记密码")); winForm.addComponent(frame); winForm.draw(); }}
执行结果如图
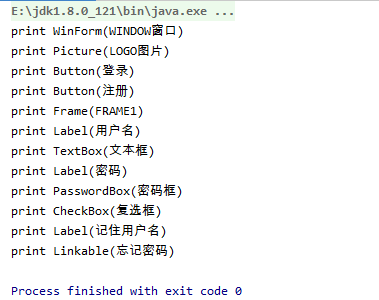
划线
评论
复制
发布于: 2020 年 10 月 03 日 阅读数: 19
版权声明: 本文为 InfoQ 作者【胡家鹏】的原创文章。
原文链接:【http://xie.infoq.cn/article/9cb15b523fe6521138563a3e1】。未经作者许可,禁止转载。
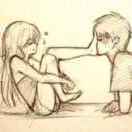
胡家鹏
关注
还未添加个人签名 2018.04.28 加入
还未添加个人简介
评论