我终于弄懂了 Python 的装饰器(四)
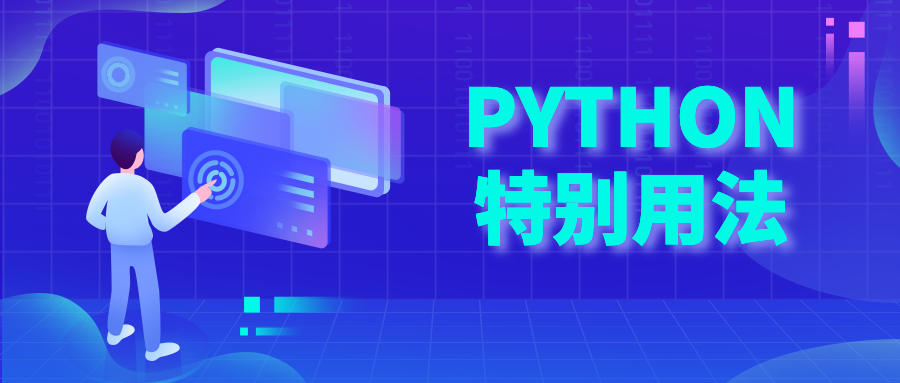
此系列文档:
四、装饰器的用法
通用装饰器
如要制作通用装饰器(无论参数如何,您都可以将其应用于任何函数或方法),则只需使用*args, **kwargs
:
建议先深入了解一下:Python中 args 和 *kwargs的用法
最佳做法:装饰器
注意:
装饰器是在Python 2.4中引入的,因此请确保您的代码将在> = 2.4上运行。
装饰器使函数调用变慢。(请记住这点)
您不能取消装饰功能。(有一些技巧,可以创建可以被删除的装饰器,但是没有人使用它们。)因此,一旦装饰了一个函数,就对所有代码进行了装饰。
装饰器包装函数,这会使它们难以调试。(这在Python> = 2.5时有所调整;请参见以下内容。)
该functools
模块是在Python 2.5中引入的。
它包括函数functools.wraps()
,该函数将修饰后的函数的名称,模块和文档字符串复制到其包装器中。
(有趣的事是:functools.wraps()
也是一个装饰器!)
Python本身提供了一些装饰:property
,staticmethod
,等。
Django使用装饰器来管理缓存和查看权限。
伪造的内联异步函数调用。
如何使用链式装饰器?
本文首发于BigYoung小站:http://www.bigyoung.cn
版权声明: 本文为 InfoQ 作者【Young先生】的原创文章。
原文链接:【http://xie.infoq.cn/article/99409190c7b7a586c6add44ed】。未经作者许可,禁止转载。
评论