1
架构训练营第三周作业
发布于: 2020 年 11 月 08 日
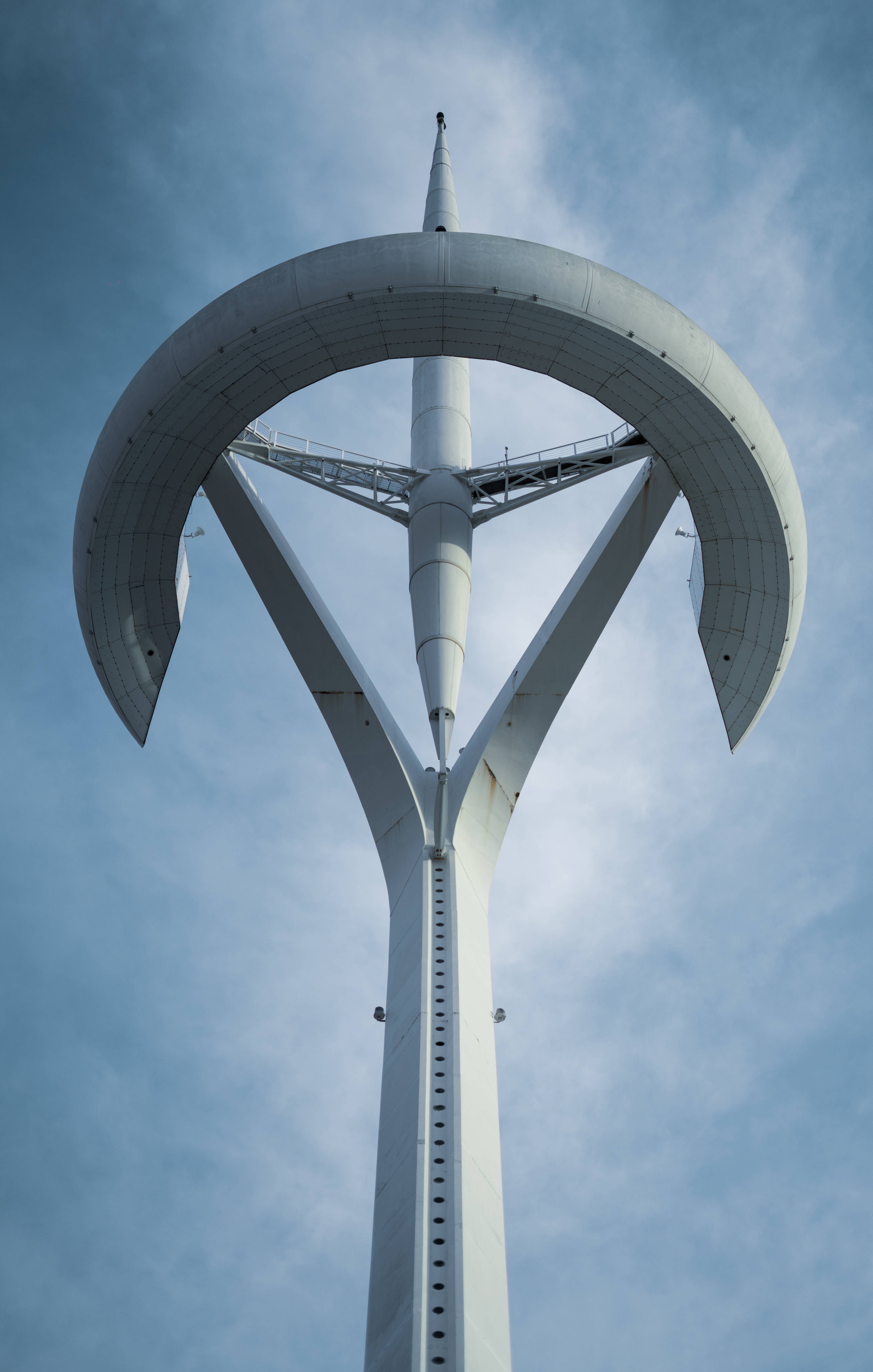
手写一个单例实现
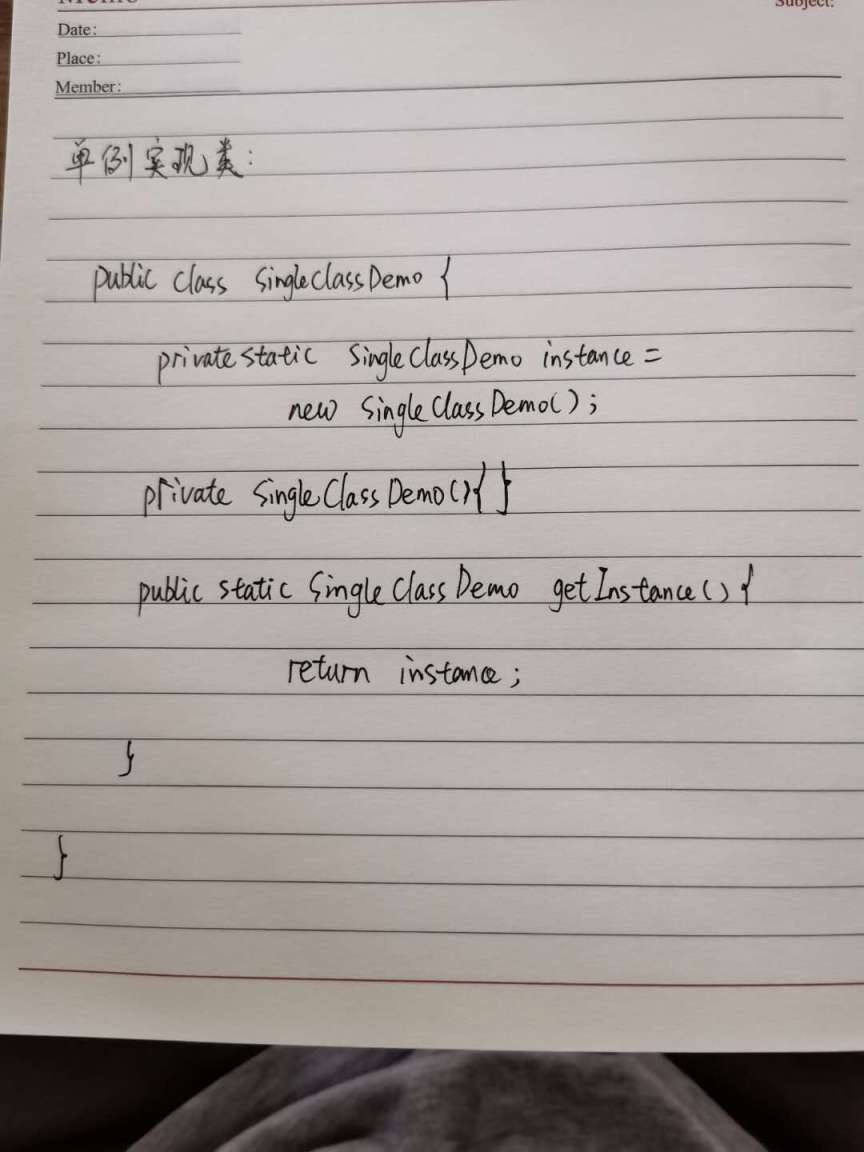
基于组合模式,打印 windows 窗口树结构
类的 UML 结构图如下:
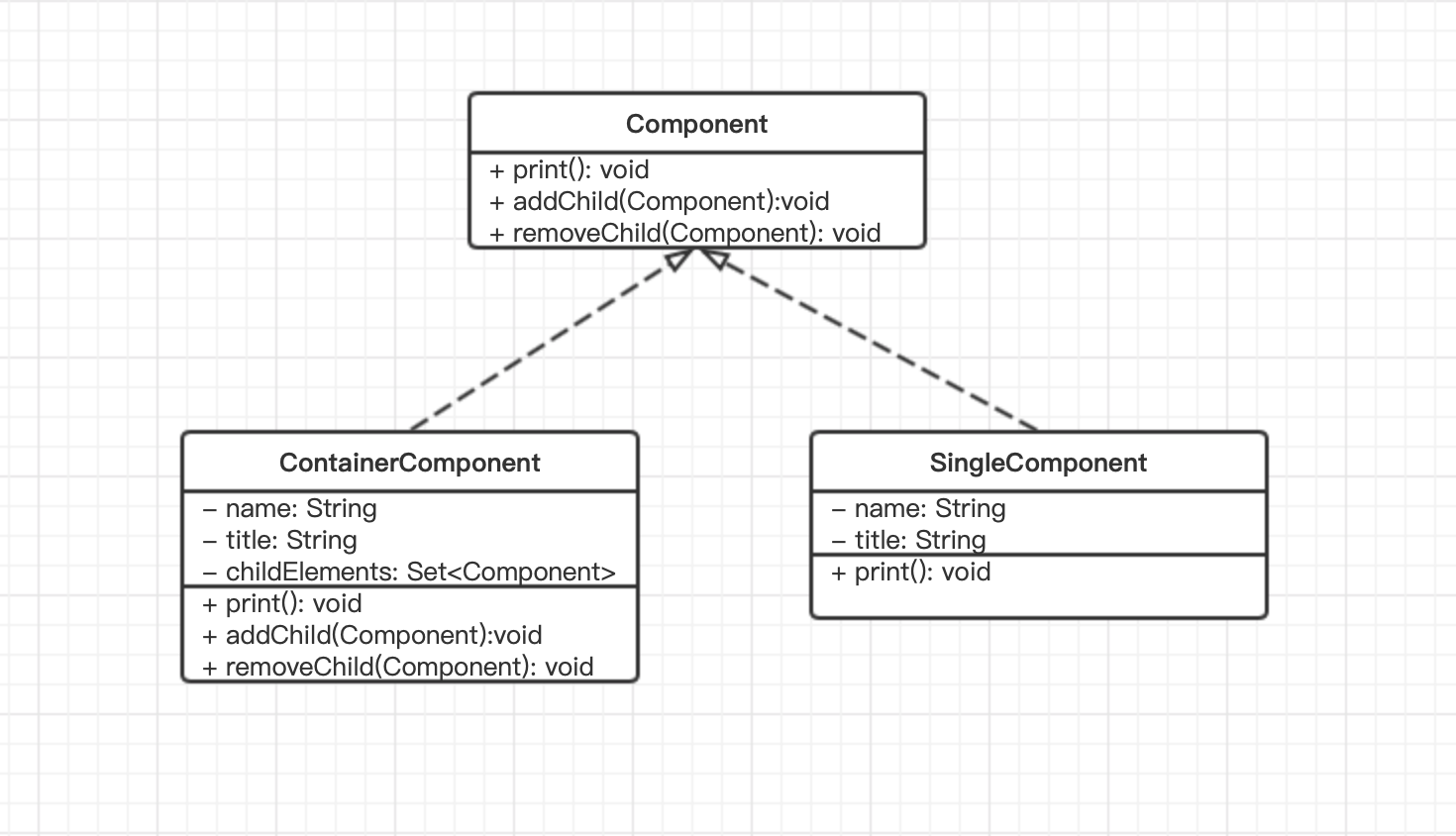
输出结果如下:
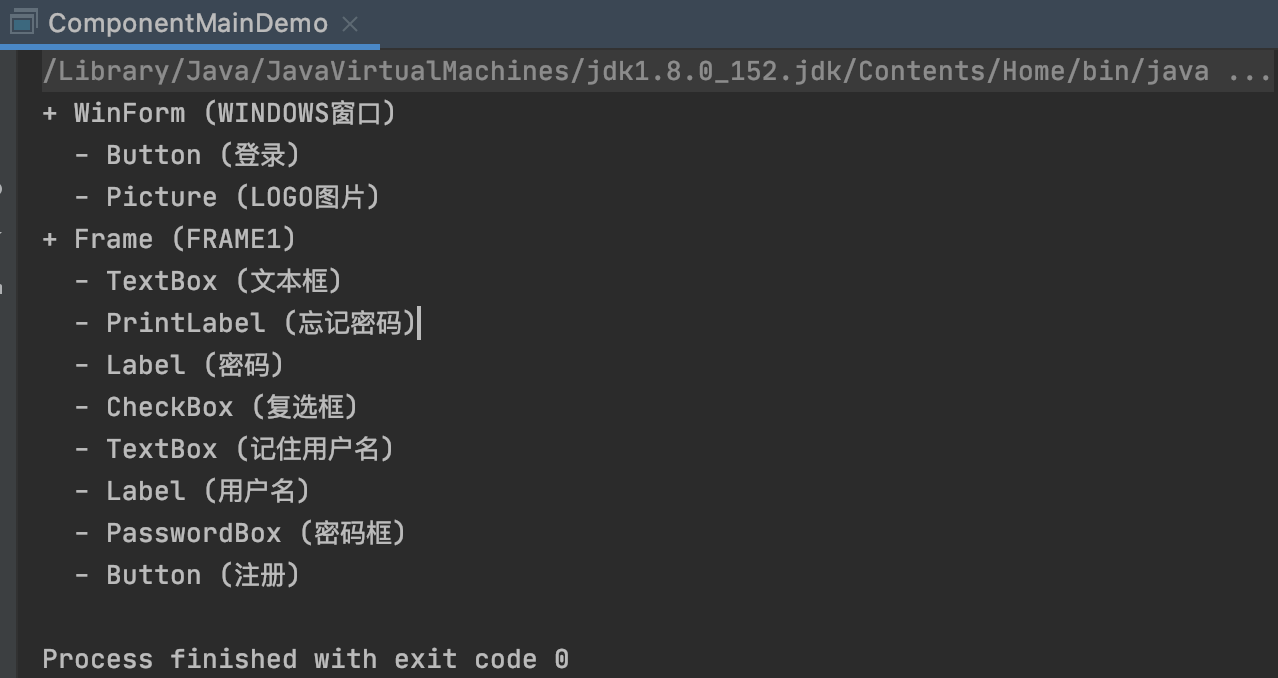
代码实现:
接口类:Component
package week3;
/**
* @Description: 定义组件的基本行为
* @Author: easonlee
* @CreateDate 2020/11/8
*/
public interface Component {
/***
* 打印自身和子组件内容
*/
void print();
/***
* 增加子节点
* @param child
*/
void addChild(Component child);
/***
* 删除子节点
* @param child
*/
void removeChild(Component child);
}
复制代码
包含子节点的实现类:ContainerComponent
package week3;
import java.util.HashSet;
import java.util.Set;
/**
* @Description: TODO
* @Author: easonlee
* @CreateDate 2020/11/8
*/
public class ContainerComponent implements Component{
private String name;
private String title;
private Set<Component> childElements;
public ContainerComponent(String name, String title){
this.name = name;
this.title = title;
childElements = new HashSet<>();
}
@Override
public void print() {
StringBuffer content = new StringBuffer();
content.append("+");
content.append(" ").append(name).append(" ").append("(").append(title).append(")");
System.out.println(content);
for (Component c:childElements){
c.print();
}
}
@Override
public void addChild(Component child) {
childElements.add(child);
}
@Override
public void removeChild(Component child) {
childElements.remove(child);
}
}
复制代码
不含子节点的实现类:SingleComponent
package week3;
/**
* @Description: TODO
* @Author: easonlee
* @CreateDate 2020/11/8
*/
public class SingleComponent implements Component{
private String name;
private String title;
public SingleComponent(String name, String title){
this.name = name;
this.title = title;
}
@Override
public void print() {
StringBuffer content = new StringBuffer();
content.append(" ").append("-");
content.append(" ").append(name).append(" ").append("(").append(title).append(")");
System.out.println(content);
}
@Override
public void addChild(Component child) {
throw new UnsupportedOperationException("对象不支持此功能");
}
@Override
public void removeChild(Component child) {
throw new UnsupportedOperationException("对象不支持此功能");
}
}
复制代码
运行的 main 类:ComponentMainDemo
package week3;
/**
* @Description: TODO
* @Author: easonlee
* @CreateDate 2020/11/8
*/
public class ComponentMainDemo {
public static void main(String[] args) {
Component root = new ContainerComponent("WinForm","WINDOWS窗口");
Component logo = new SingleComponent("Picture","LOGO图片");
Component loginButton = new SingleComponent("Button","登录");
Component regisButton = new SingleComponent("Button","注册");
Component inputFrame = new ContainerComponent("Frame","FRAME1");
Component userNameLabel = new SingleComponent("Label","用户名");
Component userNameInput = new SingleComponent("TextBox","文本框");
Component passwordLabel = new SingleComponent("Label","密码");
Component passwordInput = new SingleComponent("PasswordBox","密码框");
Component rememberCheck = new SingleComponent("CheckBox","复选框");
Component remember = new SingleComponent("TextBox","记住用户名");
Component forgetLabel = new SingleComponent("PrintLabel","忘记密码");
root.addChild(logo);
root.addChild(loginButton);
root.addChild(regisButton);
root.addChild(inputFrame);
inputFrame.addChild(userNameLabel);
inputFrame.addChild(userNameInput);
inputFrame.addChild(passwordLabel);
inputFrame.addChild(passwordInput);
inputFrame.addChild(rememberCheck);
inputFrame.addChild(remember);
inputFrame.addChild(forgetLabel);
root.print();
}
}
复制代码
划线
评论
复制
发布于: 2020 年 11 月 08 日阅读数: 57
版权声明: 本文为 InfoQ 作者【李日盛】的原创文章。
原文链接:【http://xie.infoq.cn/article/942ffe6553b41b8d19dad269c】。文章转载请联系作者。
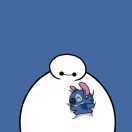
李日盛
关注
好架构=低成本+可实现 2018.01.22 加入
还未添加个人简介
评论 (1 条评论)