socket 通信,你还会实现么?
发布于: 2020 年 08 月 11 日
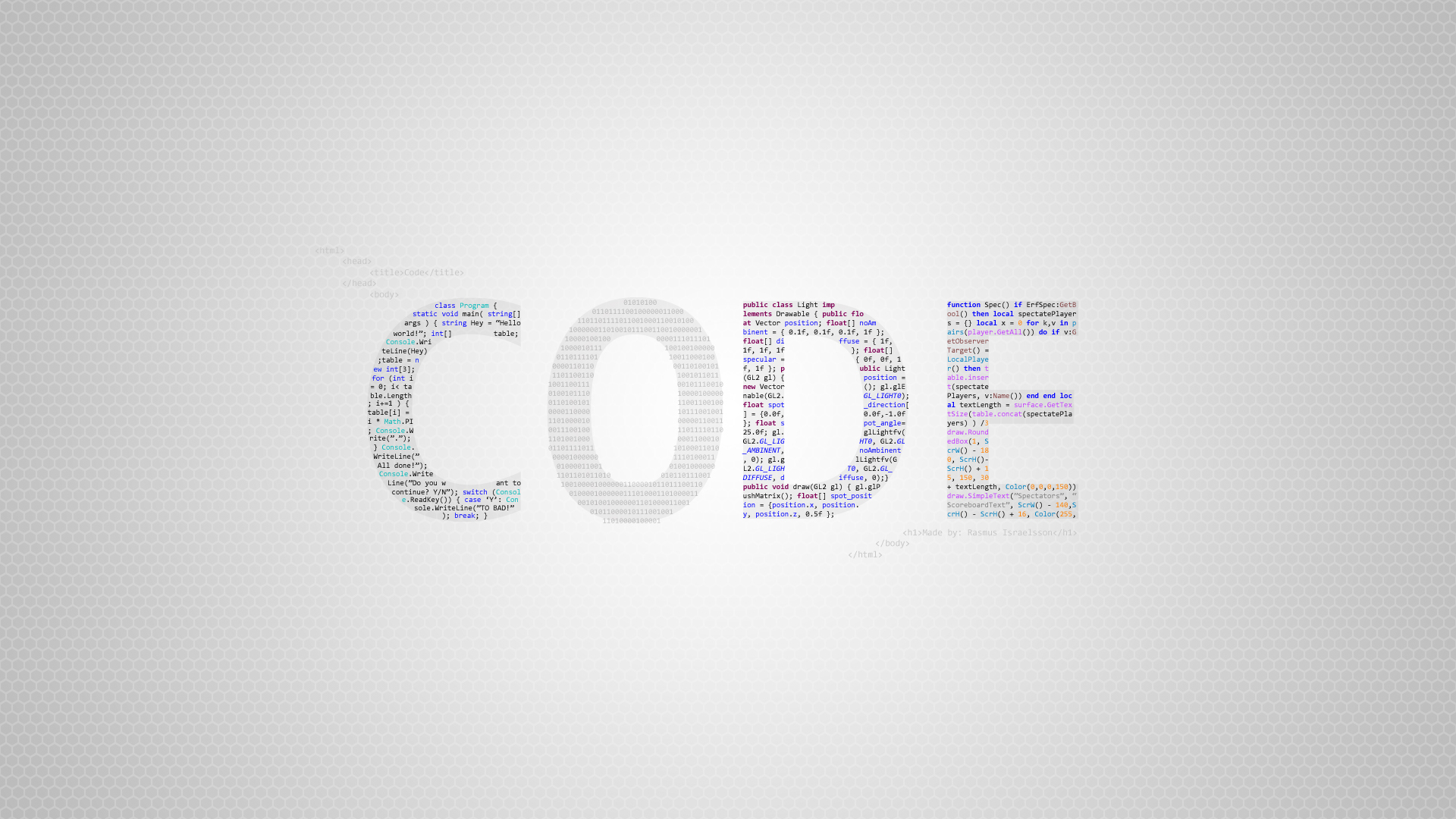
前言
最近碰到好几个朋友问我,如何实现一个简单的Socket通信。因为好多情况下,还在使用socket通信,来进行数据的传输。比如银行业务。
今天我们就上一个简单的demo。
客户端
闲言碎语不多絮,通信的双方,称为客户端、服务端。
Socket通信,采用的tcp协议,将数据转为流的形式,进行传输。
客户端代码如下:
/*** User: 小隐乐乐* Date: 2020-08-10* Time: 10:22* Description:* Socket通信客户端*/public class Client { public static void main(String[] args) { Socket socket = null; OutputStream os = null; PrintWriter pw = null; InputStream is = null; InputStreamReader isr = null; BufferedReader br = null; try { socket = new Socket("127.0.0.1", 9090); // 获取输出流向服务端写入数据 os = socket.getOutputStream(); pw = new PrintWriter(os); pw.write("用户名:admin 密码:123"); pw.flush(); socket.shutdownOutput(); // 获取输入流接受服务端返回的信息 is = socket.getInputStream(); isr = new InputStreamReader(is); br = new BufferedReader(isr); String message = null; while ((message = br.readLine()) != null) { System.out.println("服务端说:" + message); } socket.shutdownInput(); } catch (UnknownHostException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); } finally { try { if (br != null) { br.close(); } } catch (IOException e) { e.printStackTrace(); } try { if (isr != null) { isr.close(); } } catch (IOException e) { e.printStackTrace(); } try { if (is != null) { is.close(); } } catch (IOException e) { e.printStackTrace(); } if (pw != null) { pw.close(); } try { if (os != null) { os.close(); } } catch (IOException e) { e.printStackTrace(); } try { if (socket != null) { socket.close(); } } catch (IOException e) { e.printStackTrace(); } } }}
非常简单,没有过多装饰的demo代码,提供大家以参考
服务端
服务端,进行接收客户端数据,完成处理后,响应客户端。一般来说,都是保持短连接状态,即,发送 -> 接收 -> 响应 -> 结束。
至于,长连接、短连接的区分,以及如何选择,大家可以自行学习。此处不多做介绍。
服务端代码如下:
/*** User: 薛乐乐* Date: 2020-08-10* Time: 10:22* Description:* 服务端线程类*/public class ServerSocketThread extends Thread{ private Socket socket; public ServerSocketThread(Socket socket) { this.socket = socket; } @Override public void run() { InputStream is = null; InputStreamReader isr = null; BufferedReader br = null; OutputStream os = null; PrintWriter pw = null; try { // socket获取字节输入流 is = socket.getInputStream(); // 将字节输入流转字符输入流 isr = new InputStreamReader(is); // 将字符输入流转行输入流 br = new BufferedReader(isr); // String message = null; while ((message = br.readLine()) != null) { System.out.println("客户端发来消息:" + message); } // 必须先关闭输入流才能获取下面的输出流 socket.shutdownInput(); // 获取输出流 os = socket.getOutputStream(); pw = new PrintWriter(os); pw.write("欢迎您进入socket"); pw.flush(); // 关闭输入流 socket.shutdownOutput(); } catch (Exception e) { e.printStackTrace(); } finally { // 关闭资源 if (pw != null) { pw.close(); } try { if (br != null) { br.close(); } } catch (IOException e) { e.printStackTrace(); } try { if (isr != null) { isr.close(); } } catch (IOException e) { e.printStackTrace(); } try { if (os != null) { os.close(); } } catch (IOException e) { e.printStackTrace(); } try { if (br != null) { br.close(); } } catch (IOException e) { e.printStackTrace(); } try { if (isr != null) { isr.close(); } } catch (IOException e) { e.printStackTrace(); } try { if (is != null) { is.close(); } } catch (IOException e) { e.printStackTrace(); } } }}
/*** User: 薛乐乐* Date: 2020-08-10* Time: 10:22* Description:* Socket通信服务端* 1:建立服务端监听socket* 2:等待接受连接请求* 3:接受请求后建立socket连接* 4:输入、输出流* 5:关闭socket以及相关资源*/public class Server { private static ServerSocket SERVER_SOCKET =null;; static{ try { SERVER_SOCKET = new ServerSocket(9090); } catch (IOException e) { e.printStackTrace(); } } public static void main(String[] args) { try { System.out.println("******服务器已启动,等待客户端连接*****"); Socket socket = null; while(true){ //循环监听客户端的连接 socket = SERVER_SOCKET.accept(); //新建一个线程ServerSocket,并开启 new ServerSocketThread(socket).start(); } } catch (IOException e) { e.printStackTrace(); } }}
服务端,循环监听客户端的连接,进行数据交互。
demo奉上,希望大家能够理解。更多的,原理内容,可以回顾java 核心的通信篇。
学的再多,不要忘记最初的核心技术啊。
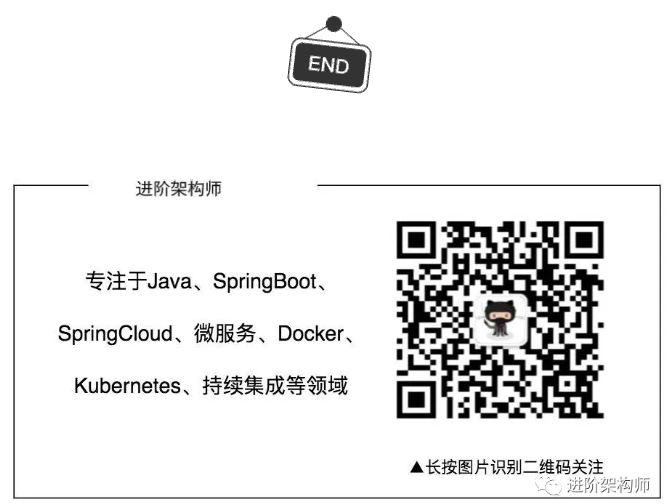
划线
评论
复制
发布于: 2020 年 08 月 11 日阅读数: 67
版权声明: 本文为 InfoQ 作者【小隐乐乐】的原创文章。
原文链接:【http://xie.infoq.cn/article/91e3b16b336ea0ff970a34967】。文章转载请联系作者。
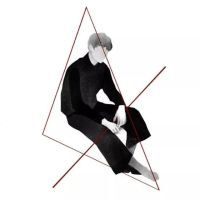
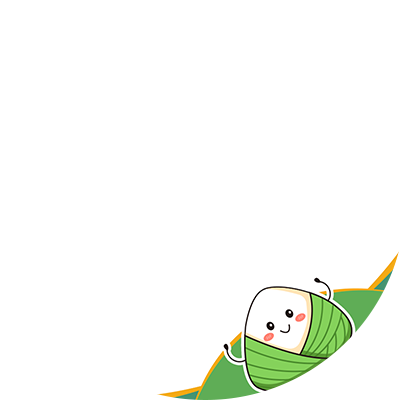
小隐乐乐
关注
学会思考,才是进步的一大本质要求 2017.04.28 加入
公众号:进阶架构师 作者
评论