OOD
OOD - Open Close Principle
DIP - Dependency Inversion Principle
LSP - Liskov Substitution Principle
SRP - Single Responsibility Principle
ISP - Interface Segregation Principle
The Open Close Principle states that the design and writing of the code should be done in a way that new functionality should be added with minimum changes in the existing code. The design should be done in a way to allow the adding of new functionality as new classes, keeping as much as possible existing code unchanged.
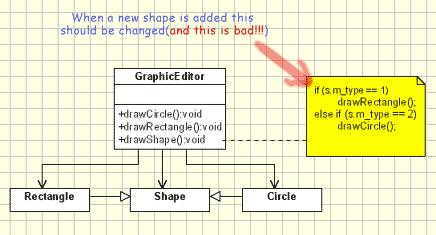
Liskov Substitution Principle
Dependency Inversion Principle
The Single Responsibility Principle is a simple and intuitive principle, but in practice it is sometimes hard to get it right.
The Interface Segregation Principle states that clients should not be forced to implement interfaces they don't use. Instead of one fat interface many small interfaces are preferred based on groups of methods, each one serving one submodule.
小结:
根据Design Principles不同的定义,重新认识了coding里的creational patterns, behavioral patterns, structural patterns。每一个定义都对应一段code,有助于不同项目里规划和设计,同时可以梳理复杂的逻辑。
Reference:
https://www.oodesign.com/interface-segregation-principle.html
评论