week3 代码重构 学习总结
3.1 设计模式:使用设计模式优化排序工具包的设计
第二周学习了软件设计(OOD)的原则:
开闭原则
依赖倒置原则
里氏替换原则
接口隔离原则
单一职责原则
OOD的目标:强内聚,低耦合
设计模式:创建模式、结构模式、行为模式
框架
应用程序
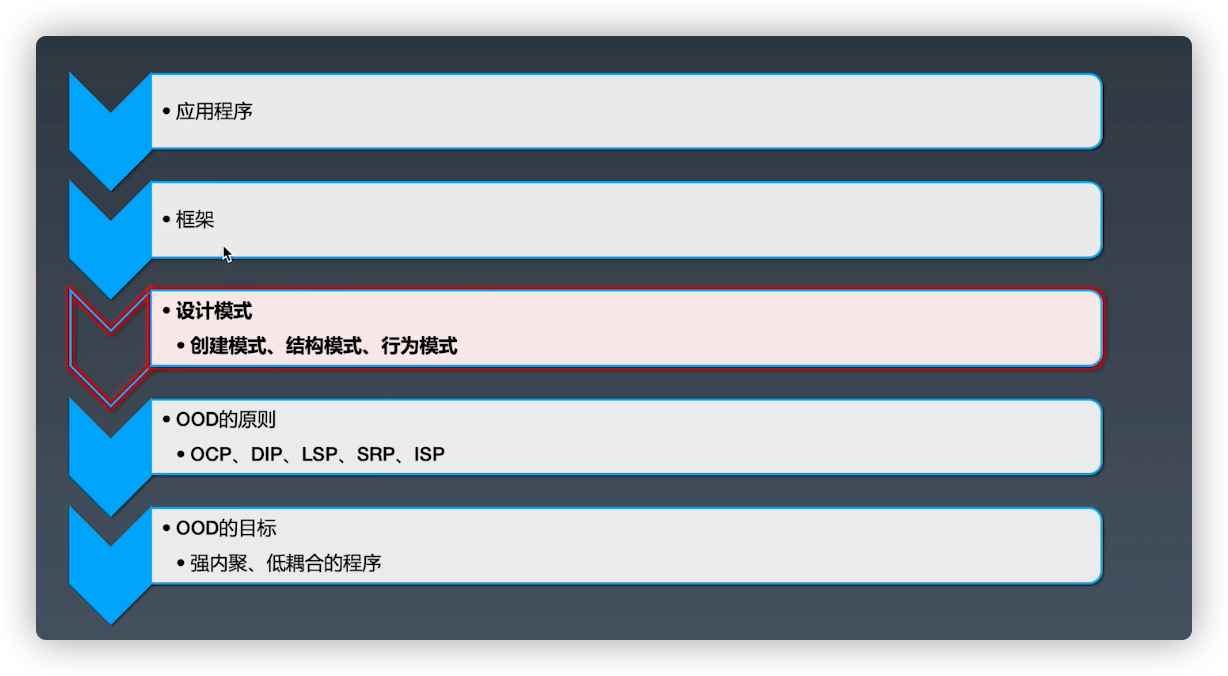
框架是如何设计的?框架下的设计模式是什么?设计模式遵循的原则是什么?
设计模式的定义:
每一种模式描述了一种问题解决的通用解决方案。这种问题在我们的环境中,不停的出现
设计模式是一种可重复使用的解决方案
一个设计模式的四个部分:
模式的名称:由少量的字组成的名称,有助于表达我们的设计
待解问题:描述了何时需要运用这种模式,以及运用模式的环境(上下文)
解决方案:描述了组成设计的元素(类和对象),他们的关联、职责、以及合作。这种解决方案是抽象的,它不代表具体的实现。
结论:运用这种方式带来的利和弊、主要是指它对系统的弹性、扩展性、和可移植性的影响。
设计模式的分类:
分类方案:
简单工厂模式
通过配置文件实现开闭原则
3.2 设计模式:Singleton单例模式
单例模式保证产生单一实例,就是说一个类产生一个实例。使用单例模式,原因有二:
只有一个实例,可以减少实例频繁创建和销毁带来的资源消耗
当多个用户使用这个实例的时候,便于进行统一控制
前者是性能需求,后者是功能需求
两种实现方式
方式一
私有的构造函数,保证类实例只能通过getinstance()方法获得
公有的静态方法,返回实例对象
饿汉模式&懒汉模式
方法二中getinstance的修饰符synchronized一定要加上,否则可能产生多重实例
尽量使用方法一构造单实例
单例模式中的成员是多线程重用的,可能会产生意想不到的结果,所以尽量将单例模式设计成无状态对象(只提供服务,不保存状态)
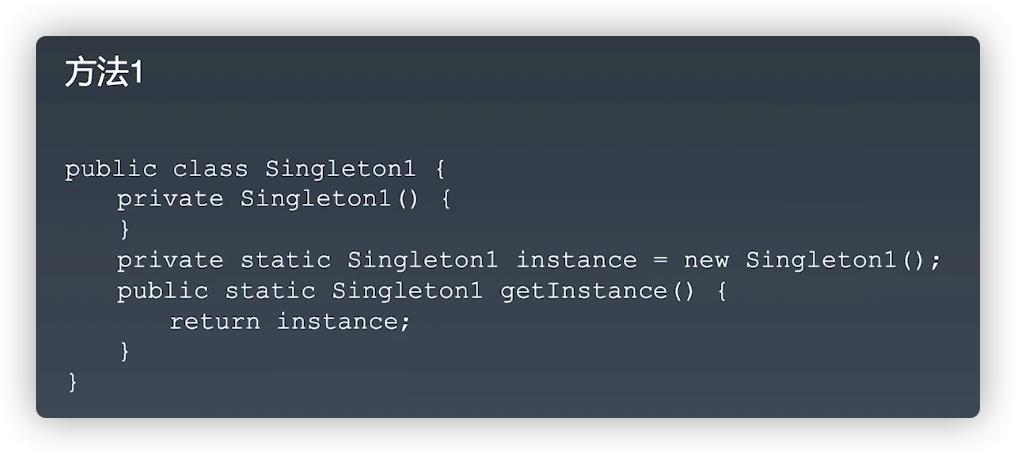
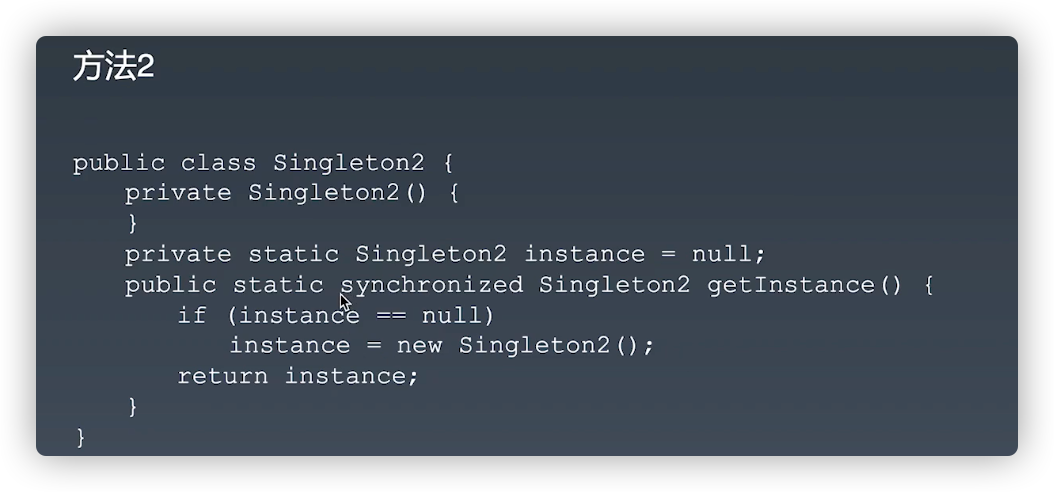
适配器模式
把已经存在的方法适配成我们需要的方法、接口,从而实现开闭原则、实现接口策略、实现依赖倒置。
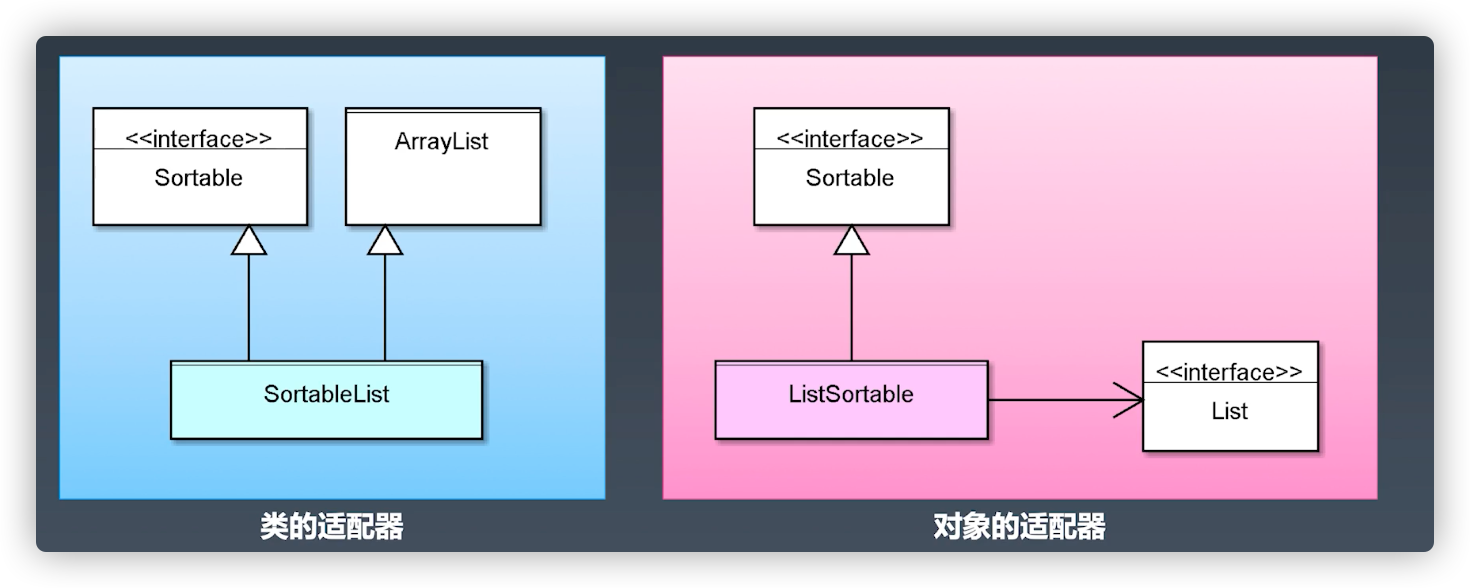
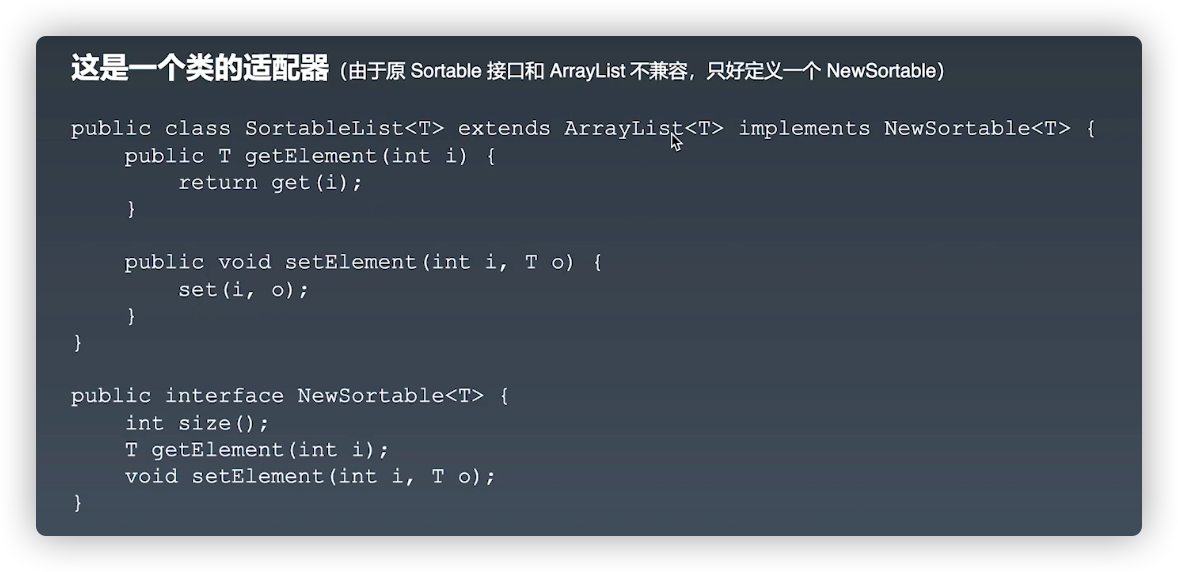
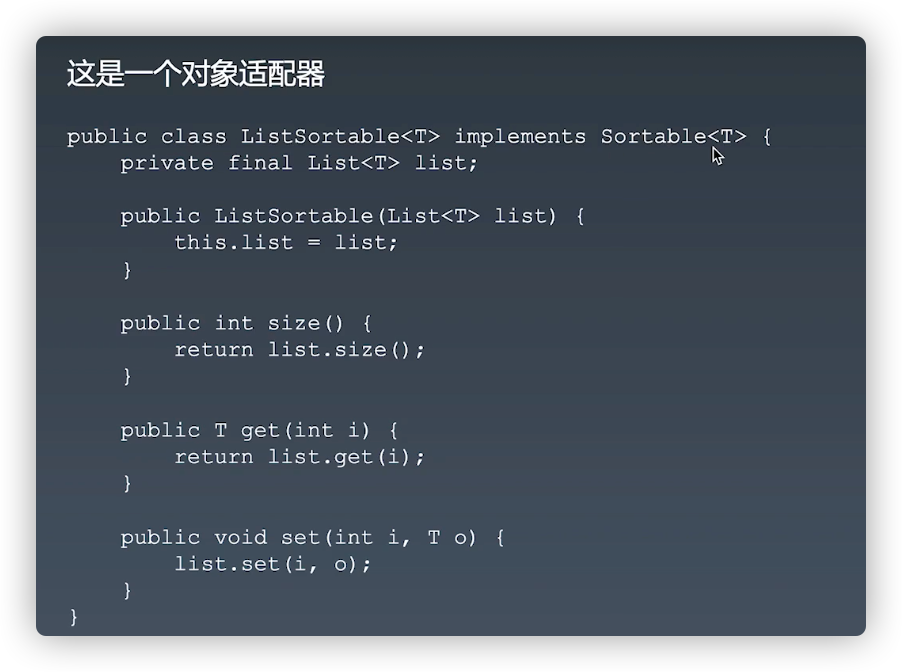
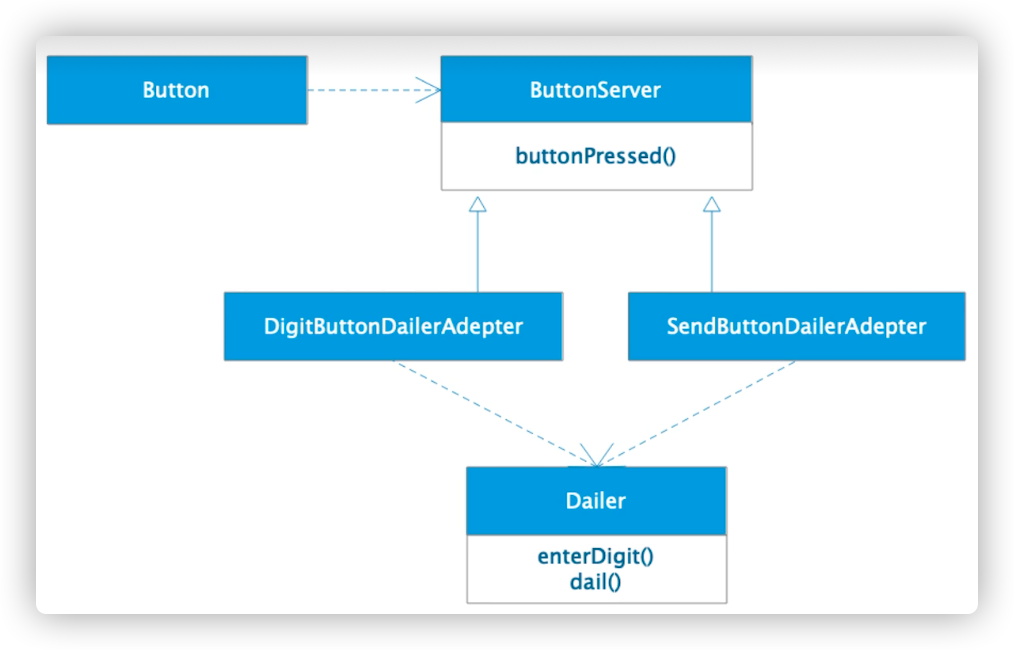
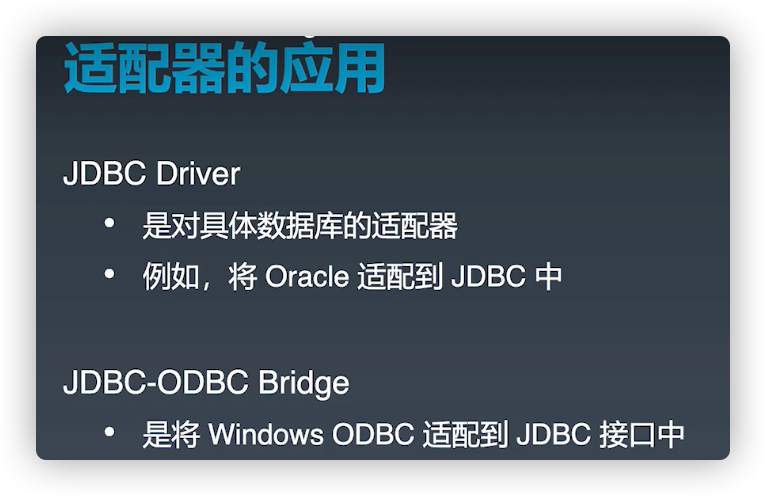
3.3 JUnit中的设计模式(上)
单元测试框架
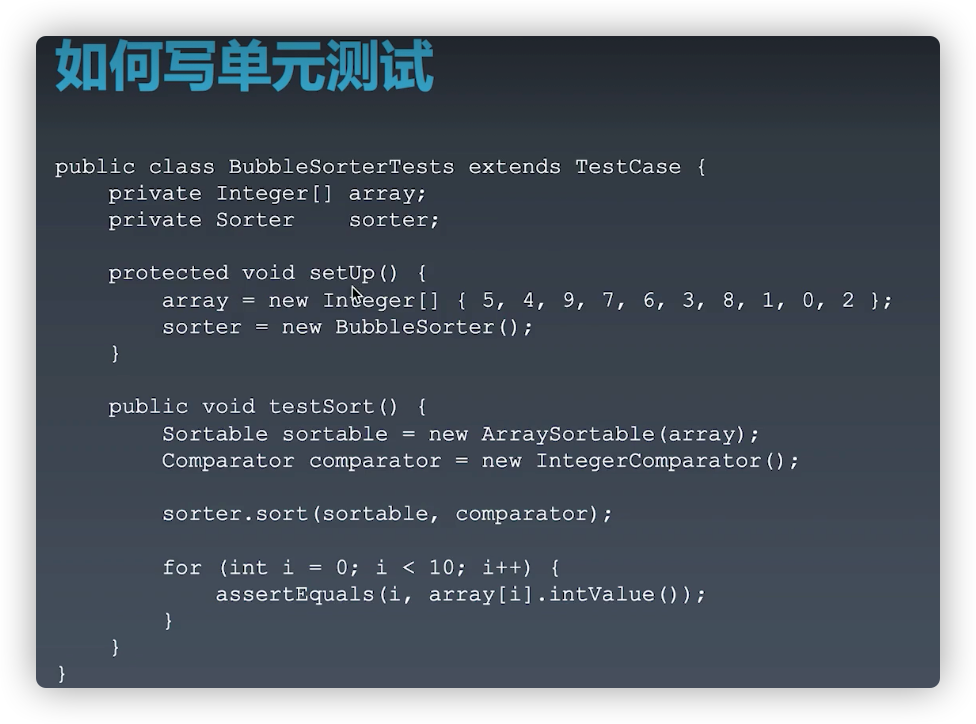
实现一个单元测试的步骤:
创建测试类、从TestCase类派生
初始化
覆盖基类的方法:portected void setUp()
书写测试方法
命名规则:public void testXyz()
清除环境
覆盖基类的方法:portected void tearDown()
模版方法模式
模版方法模式是扩展功能的最基本的模式之一
是一种类的行为模式
通过继承的方法实现扩展
基类负责算法的模版和骨架
子类负责算法的具体实现
模版方法的实现
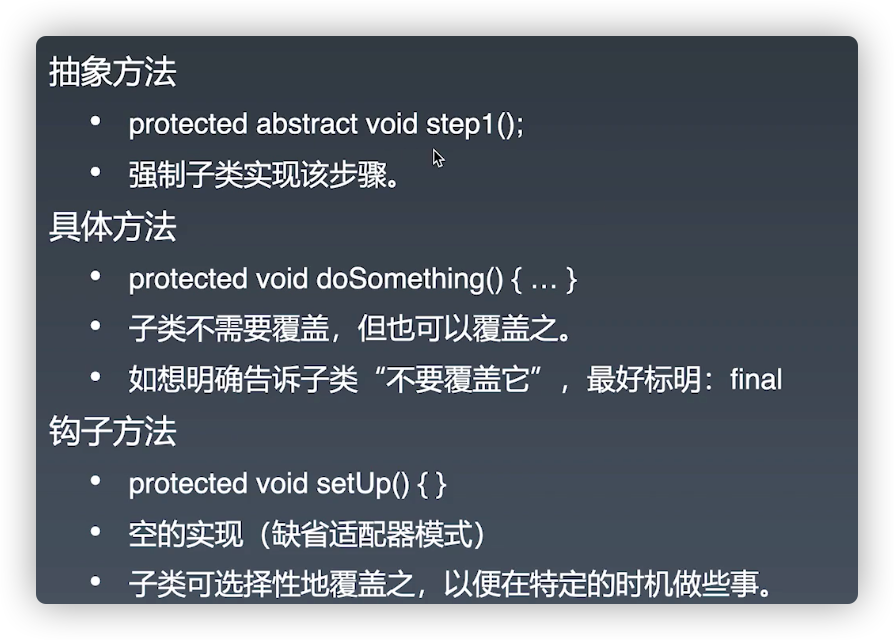
java servelt中的模版方法
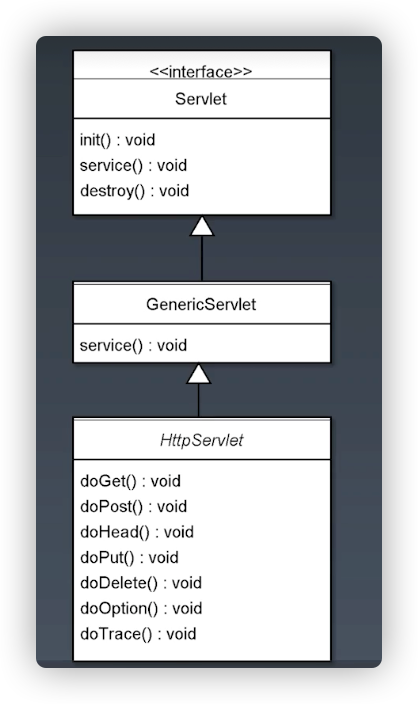
测试sortable
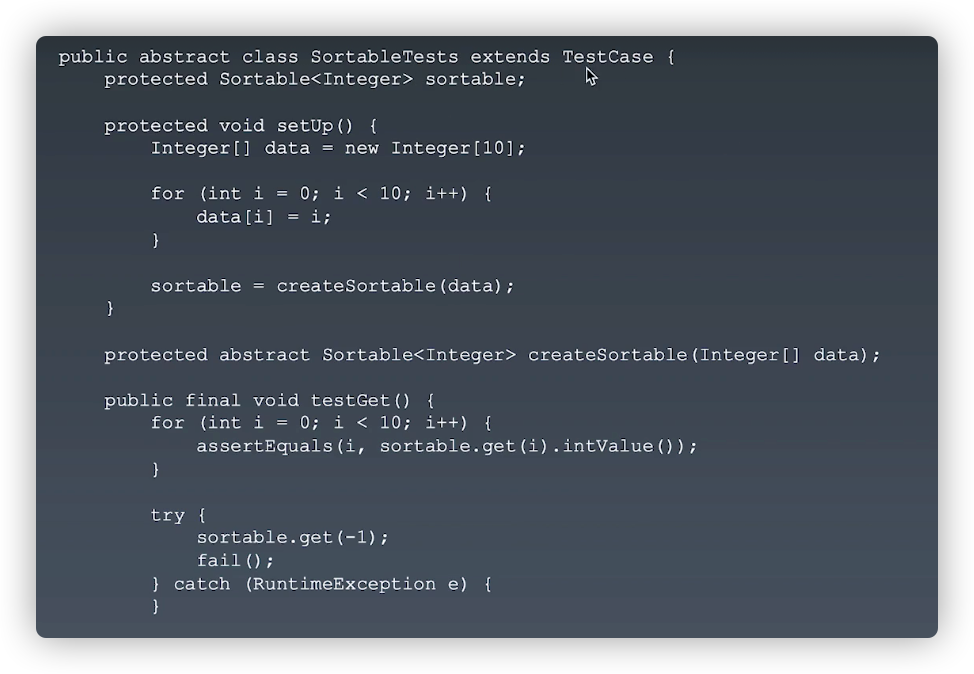
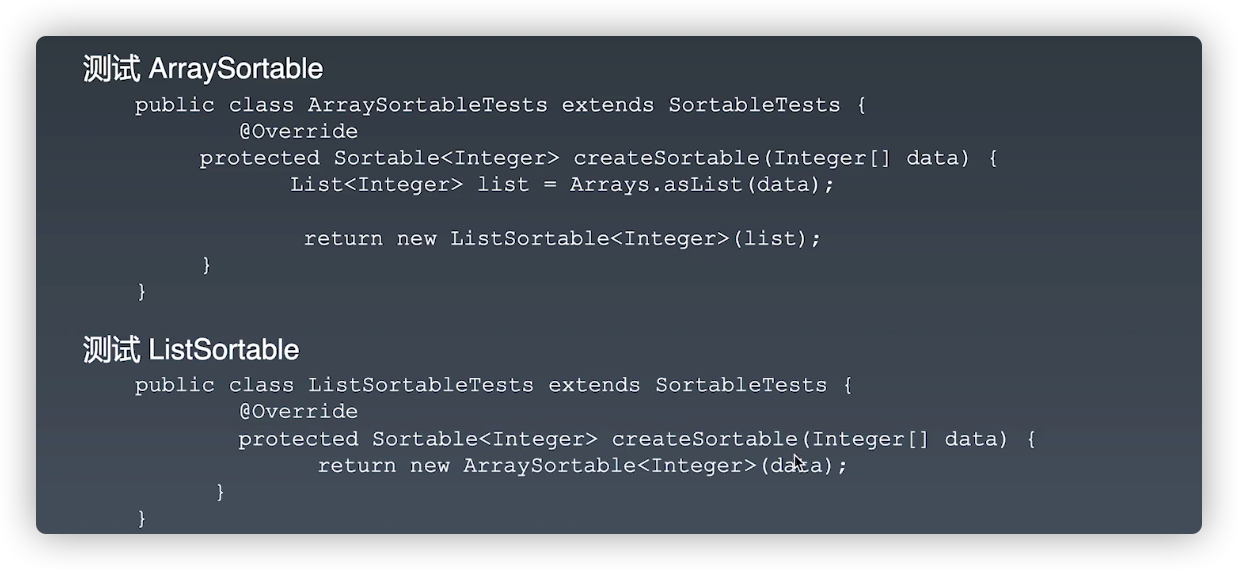
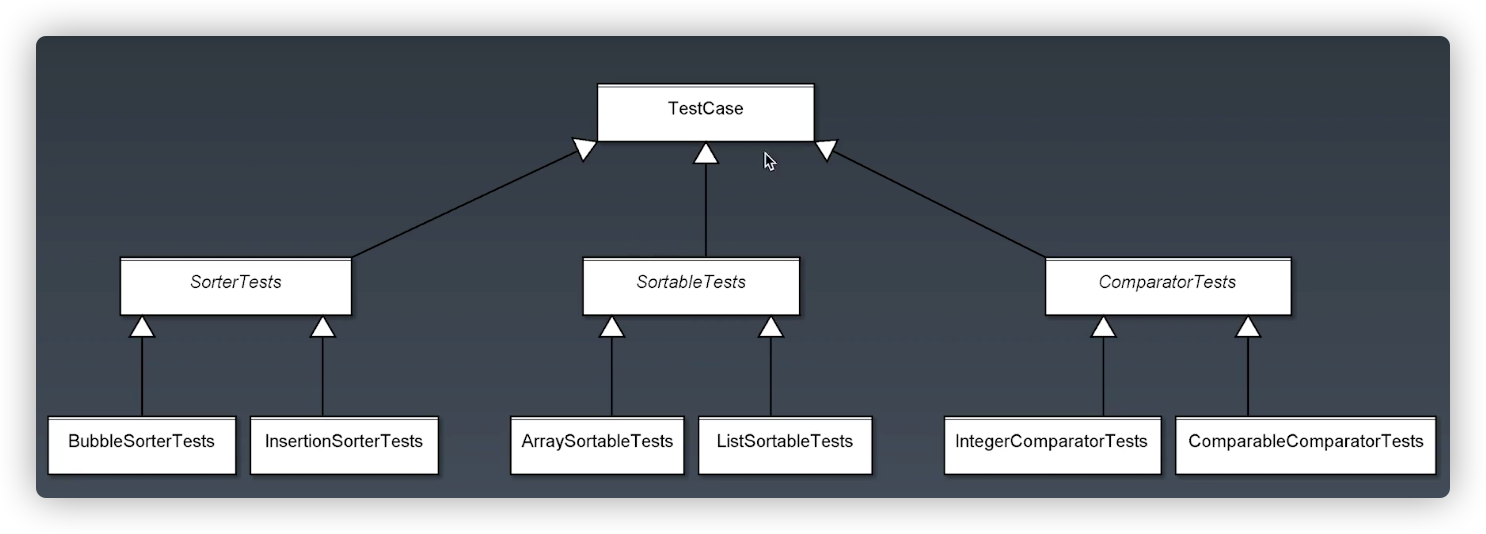
策略模式
策略模式是扩展功能的另一种基本模式
它是一种对象的行为模式
通过组合的方式实现扩展
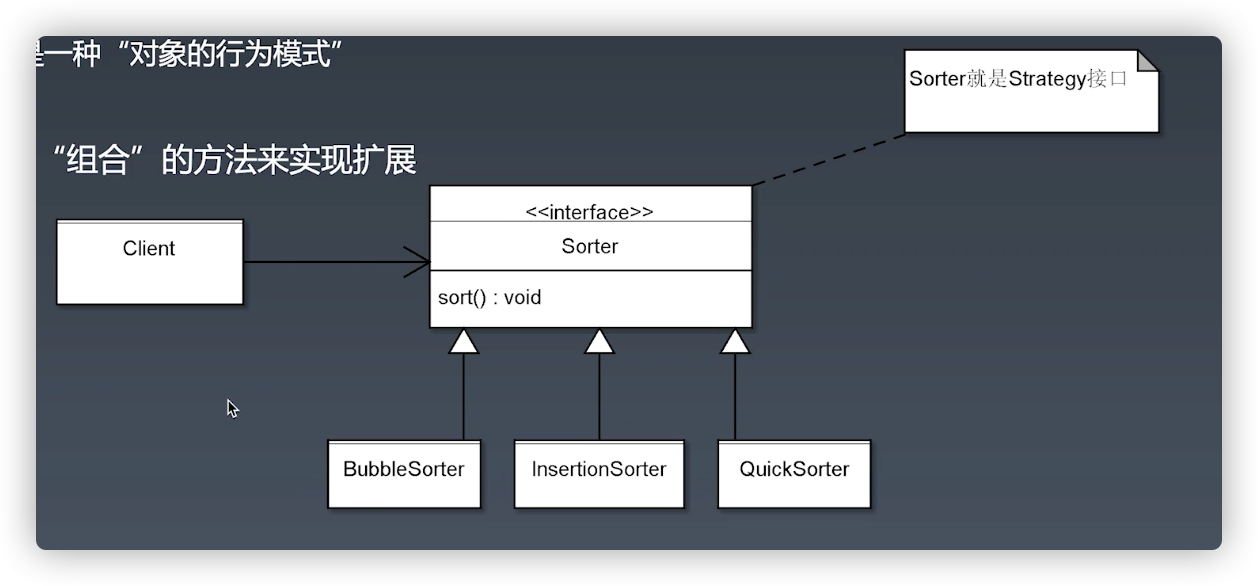
策略模式和模版方法的结合
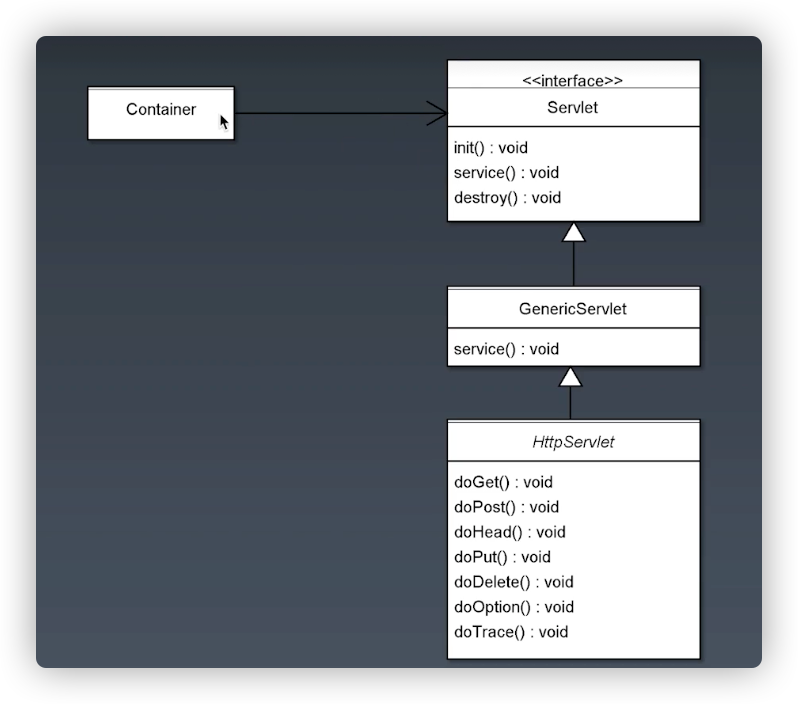
3.4 JUnit中的设计模式(下)
参数化的单元测试
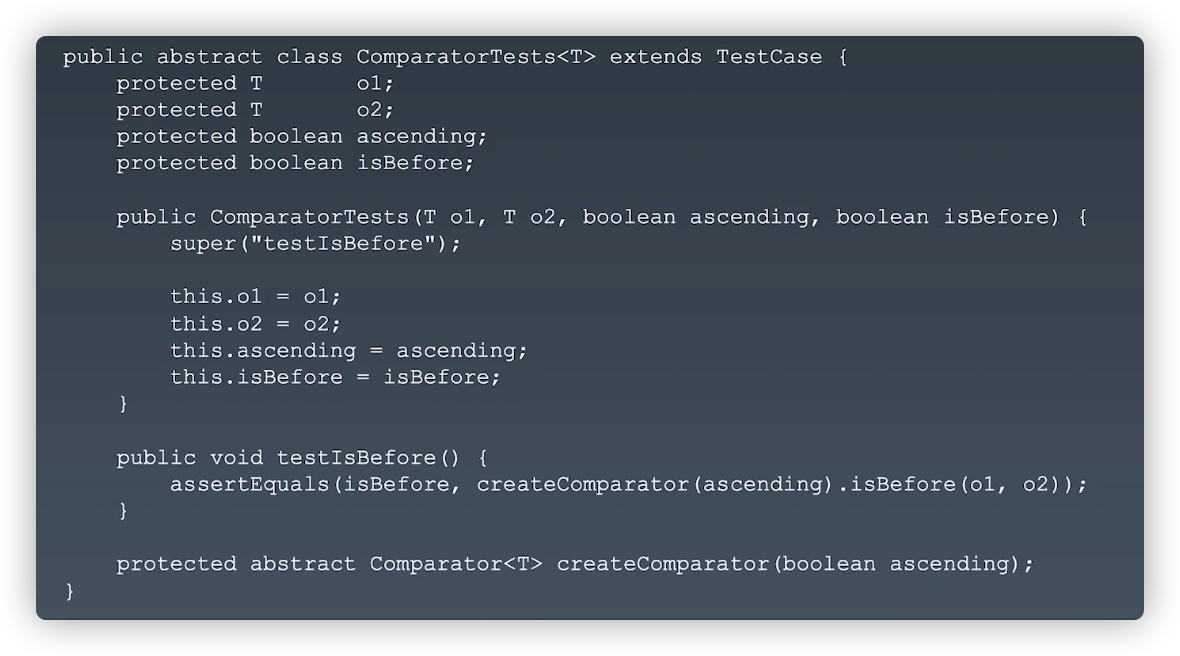
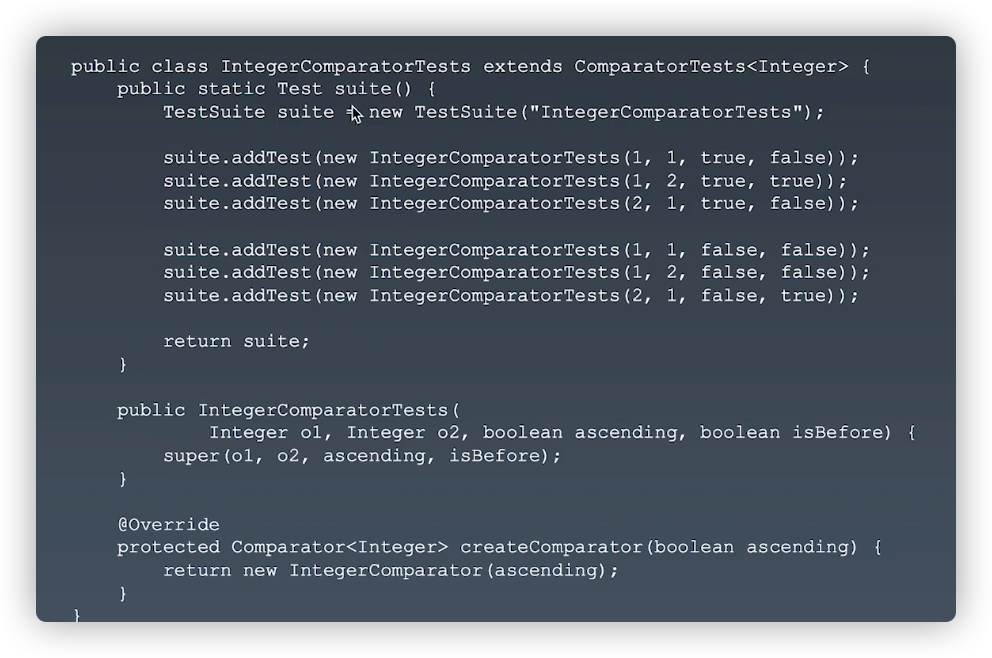
组合模式
是一种对象的结构模式
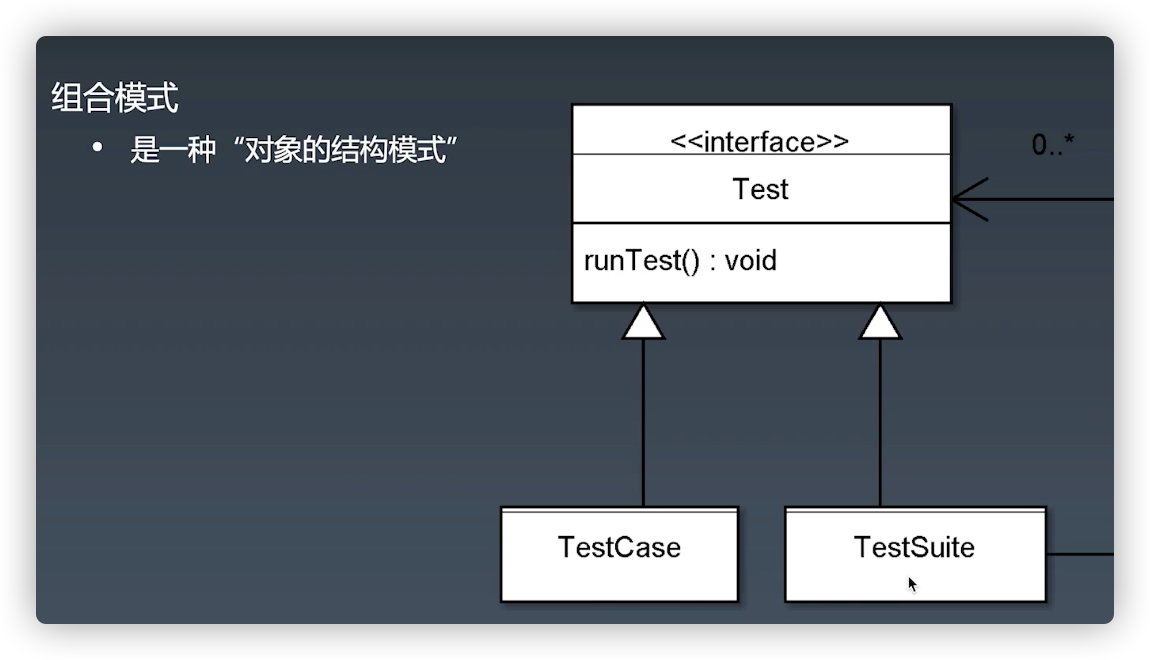
组合模式的应用
文件系统AWT控件
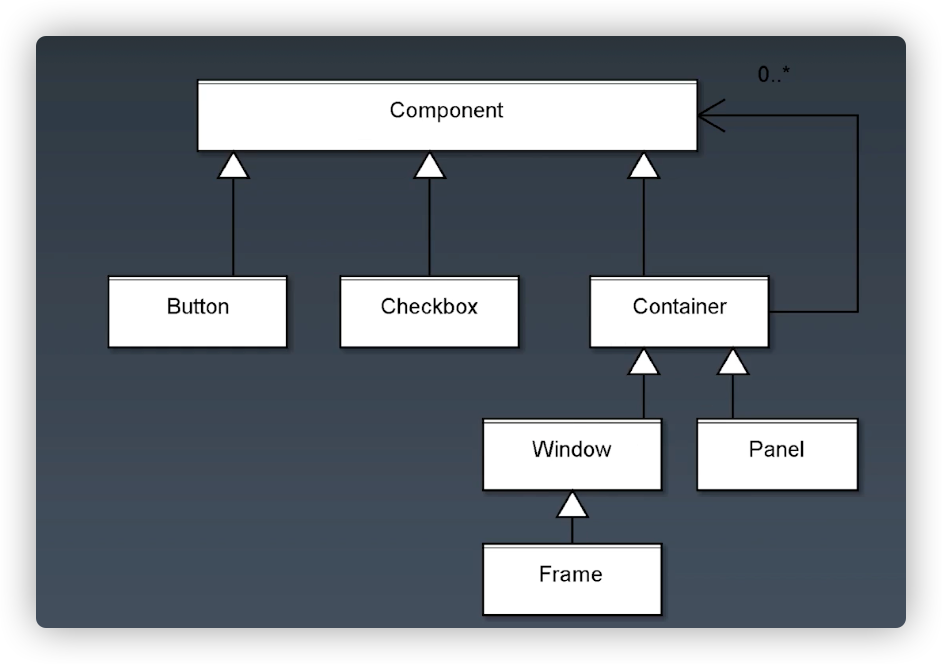
测试排序程序的性能
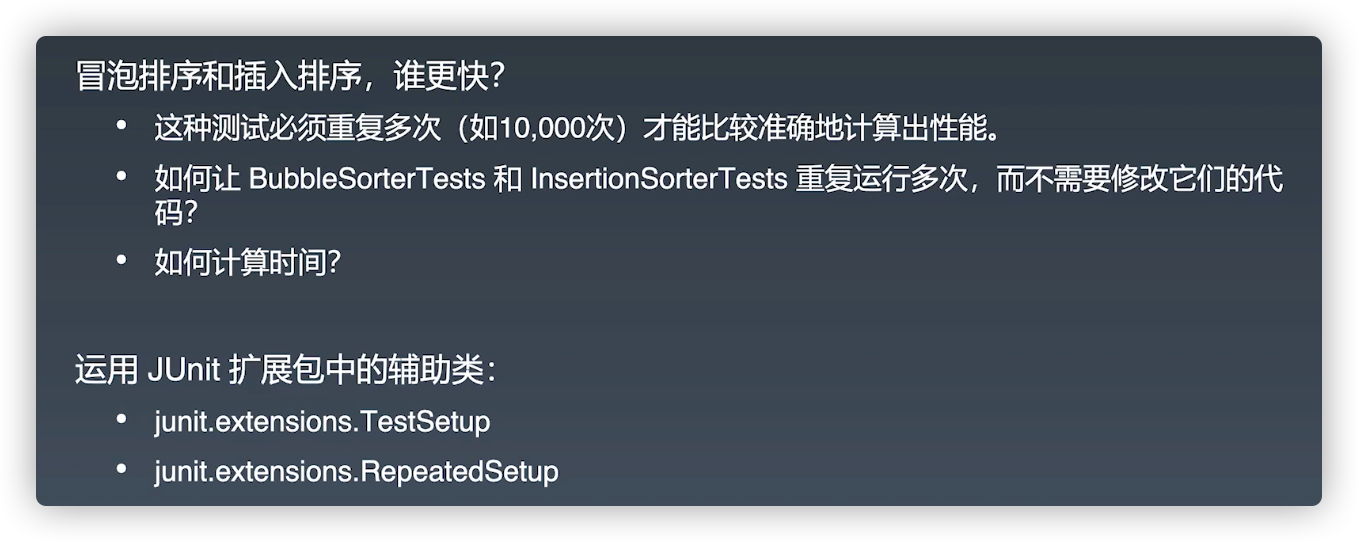
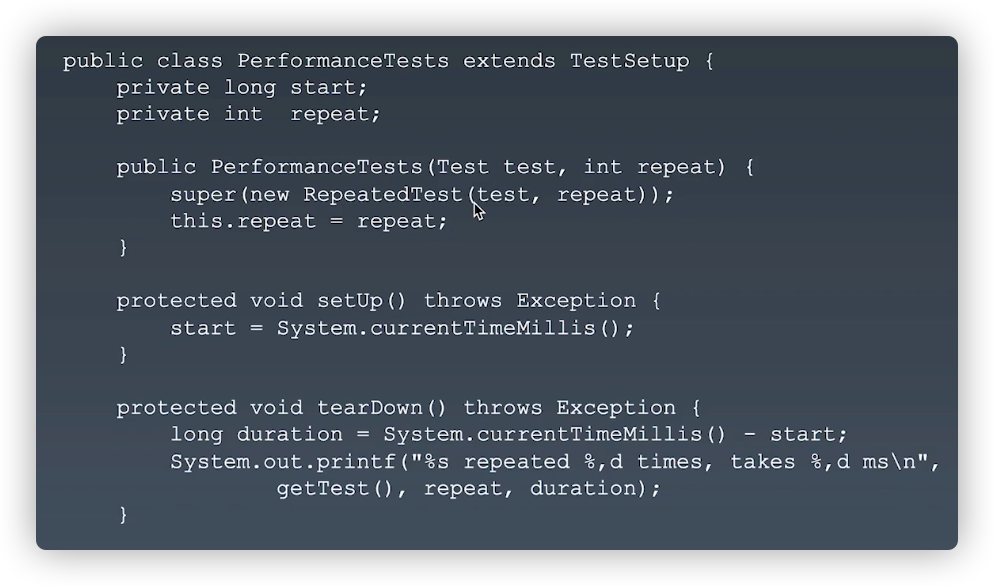
装饰器模式
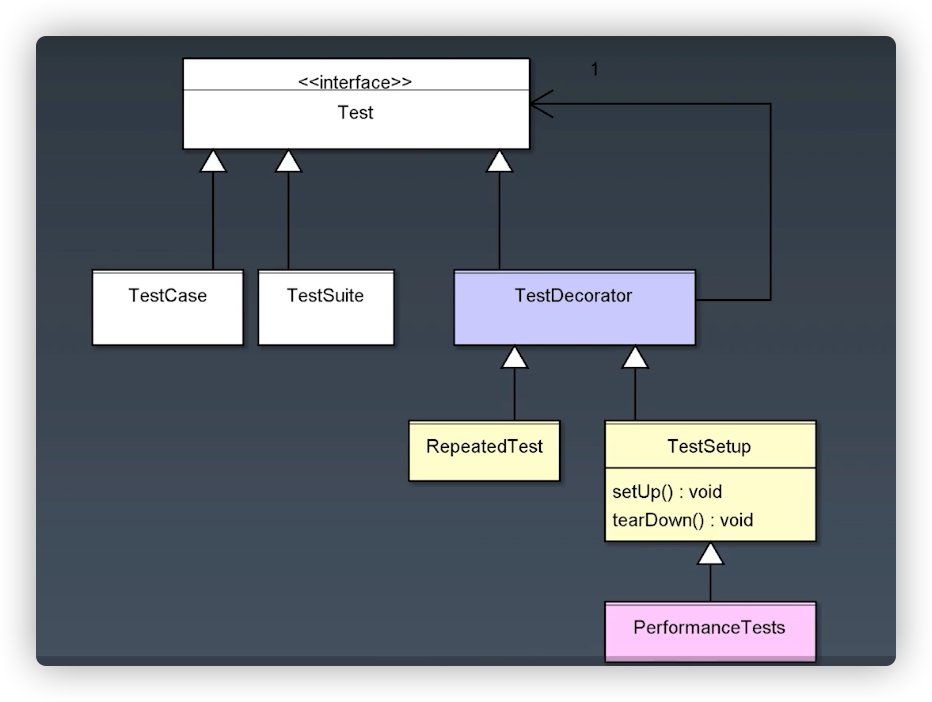
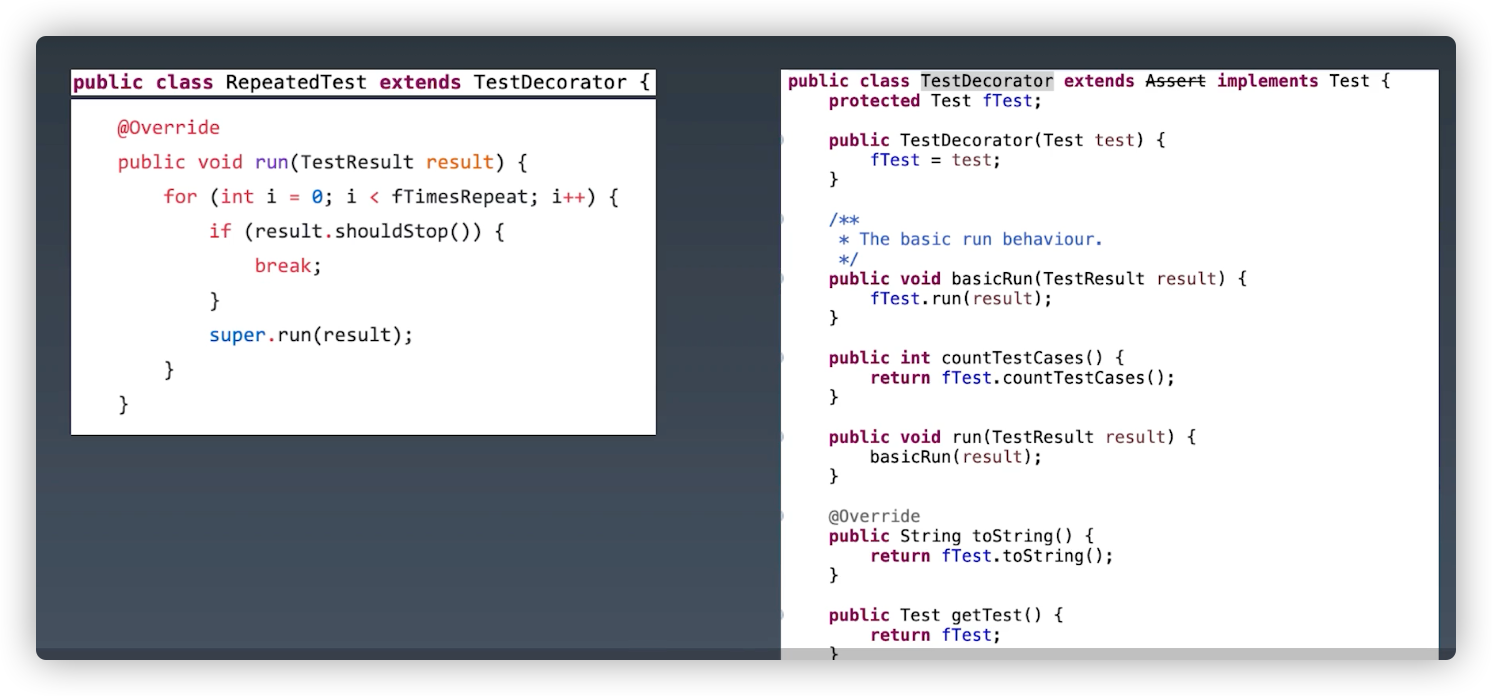
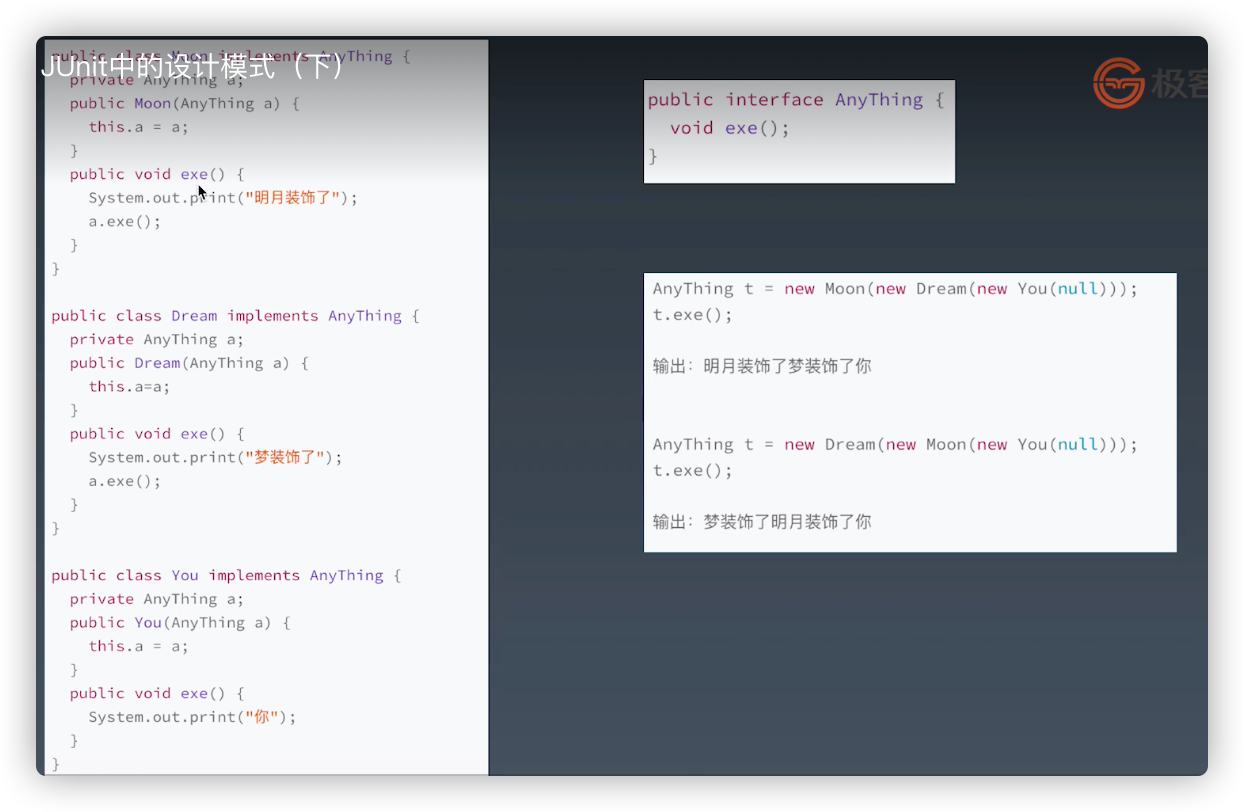
装饰器的应用
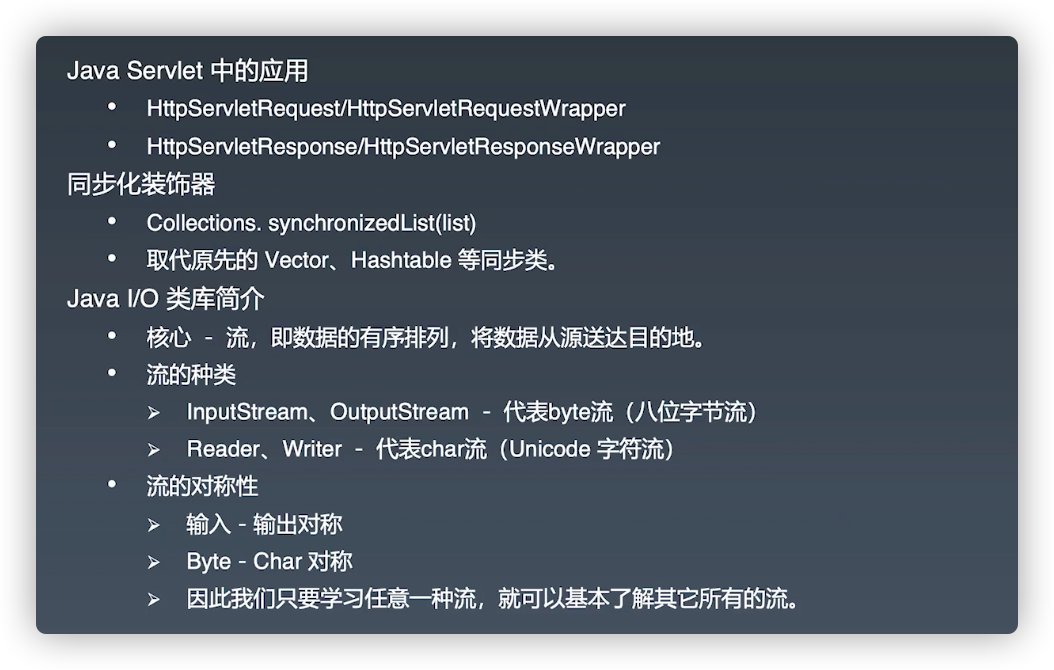
3.5 Spring中的设计模式
软件设计过程中,可以重复使用的设计方案;重复的问题,可复用的解决方案
四人帮的GOF设计模式
23中设计模式
依赖注入DI与控制反转IoC
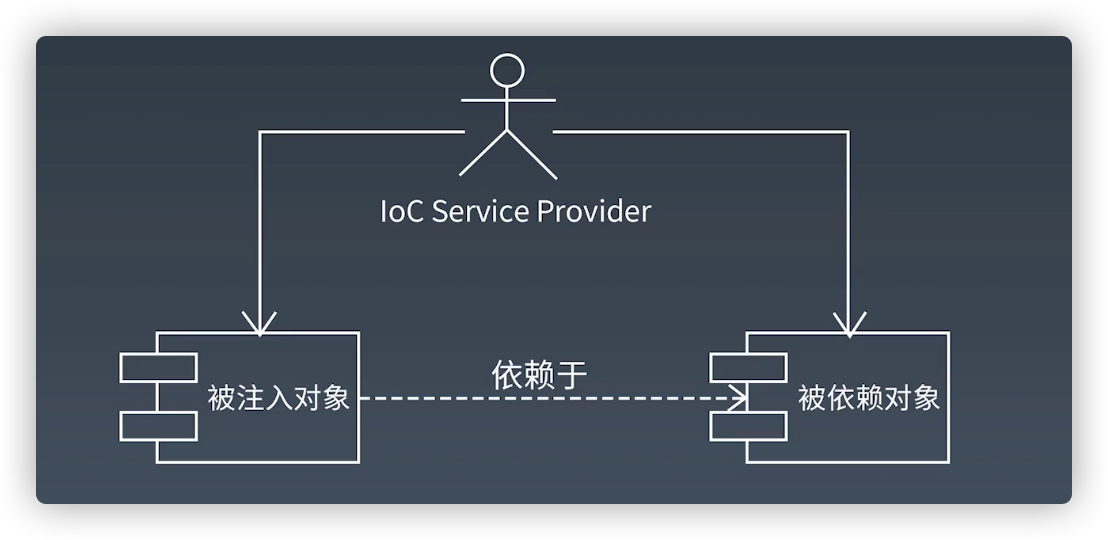
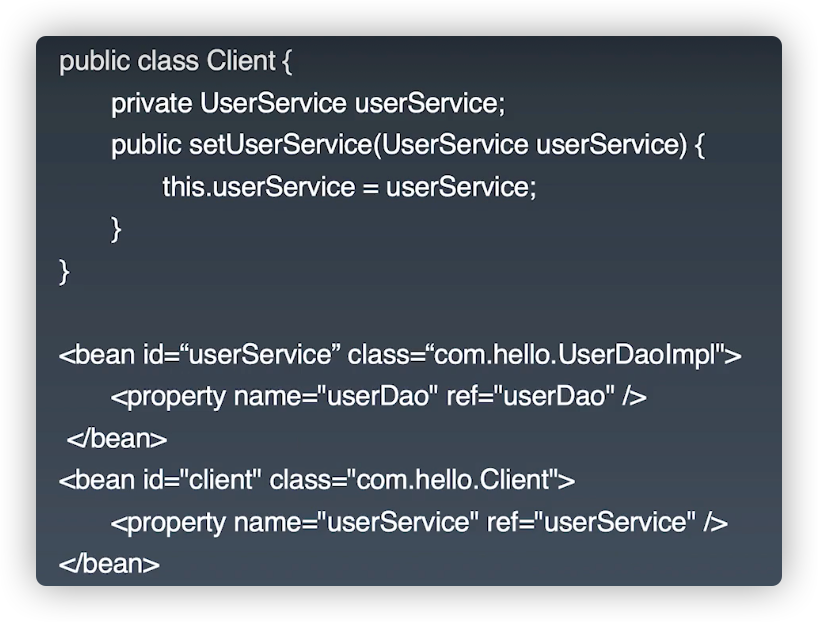
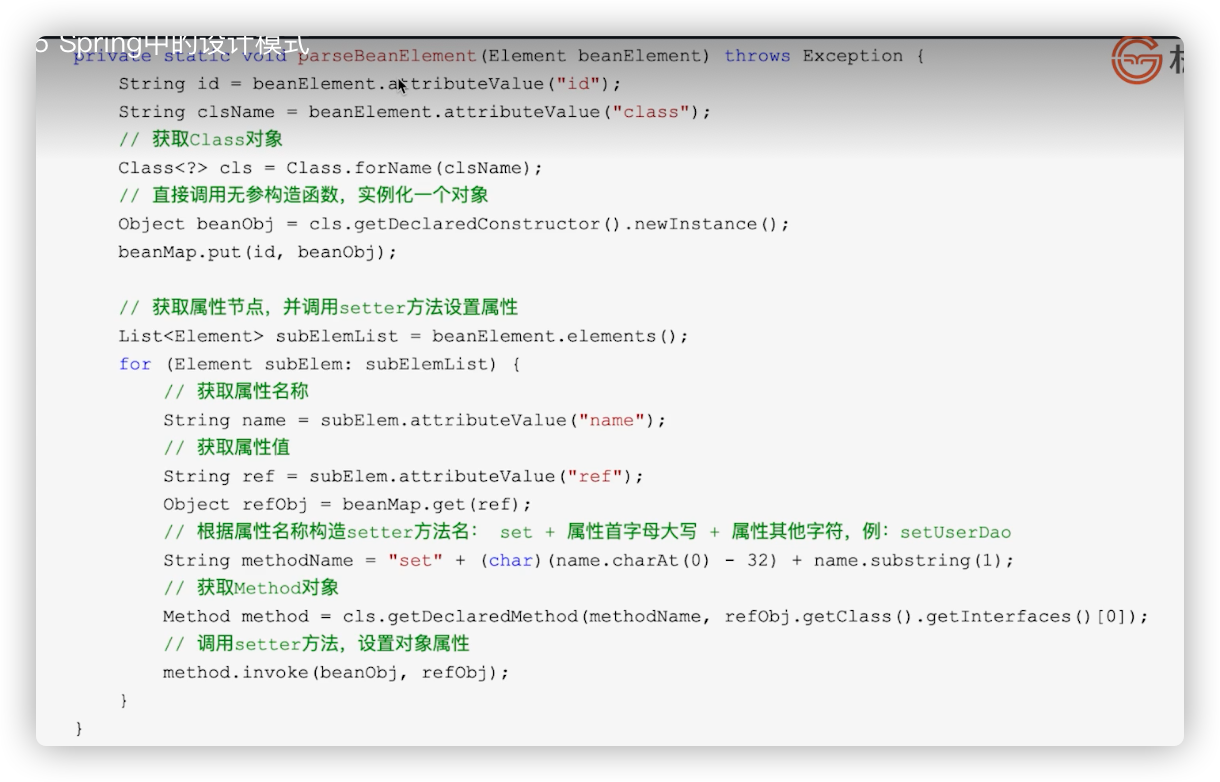
Spring MVC模式
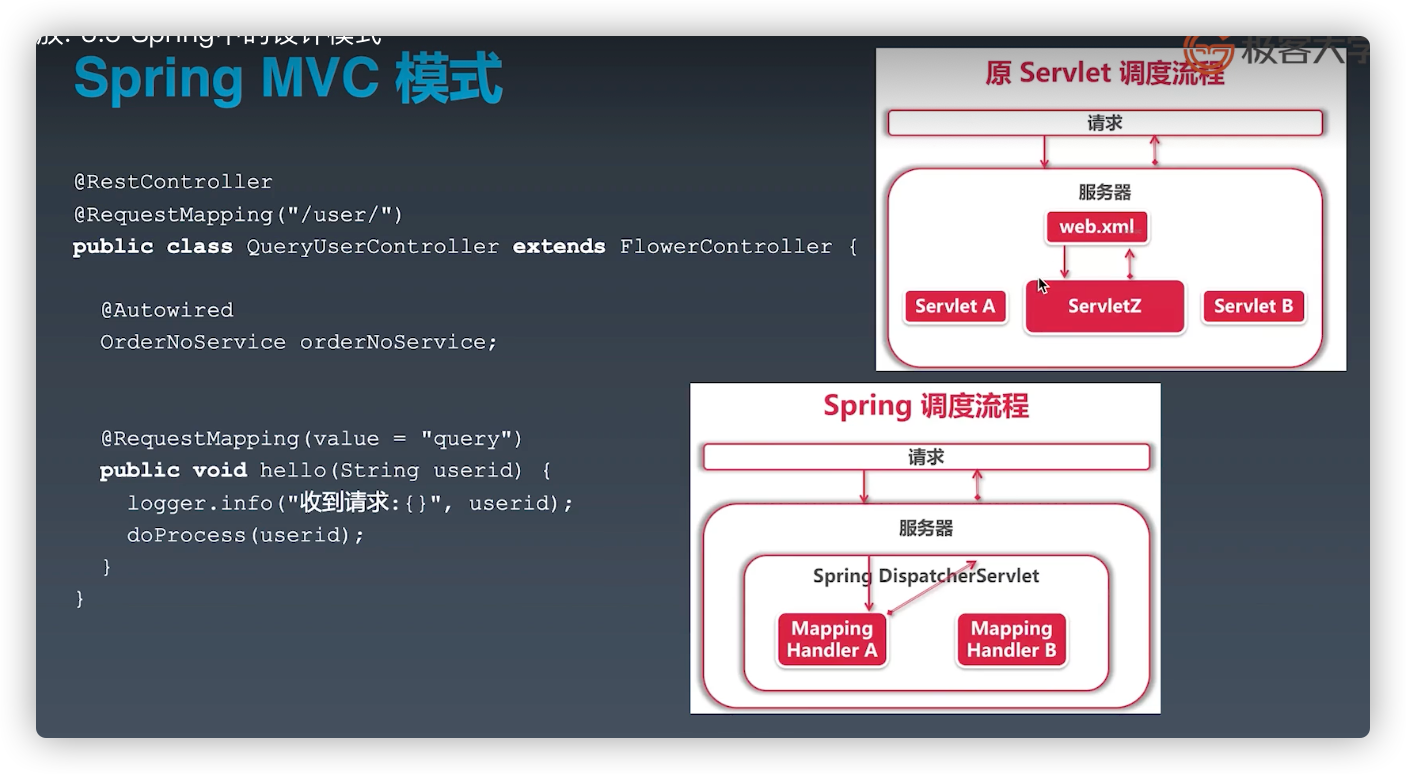
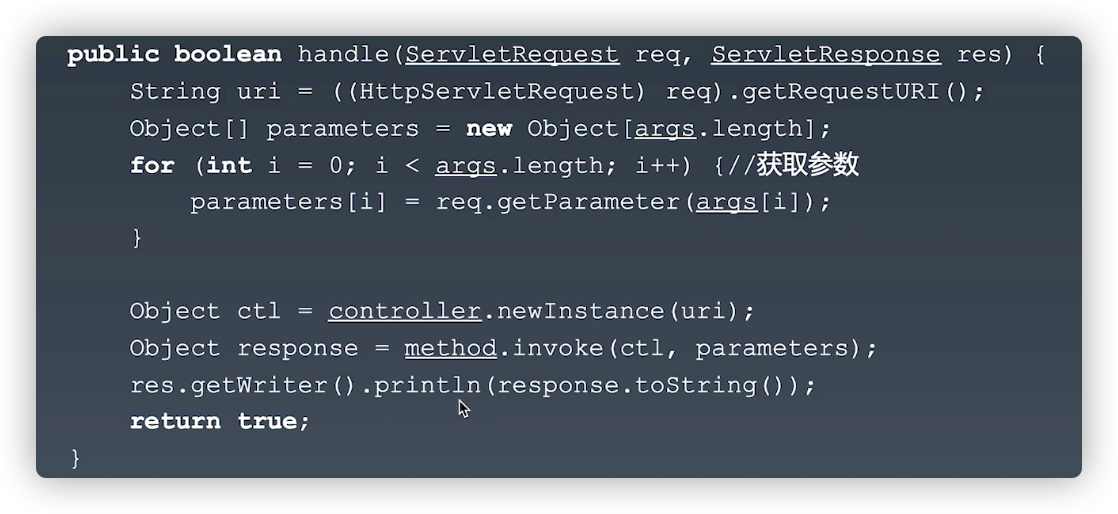
3.6 设计模式案例:Intel 大数据SQL引擎&Panthera设计模式
大数据SQL引擎
oracle - > Hive
hive支持标准的sql
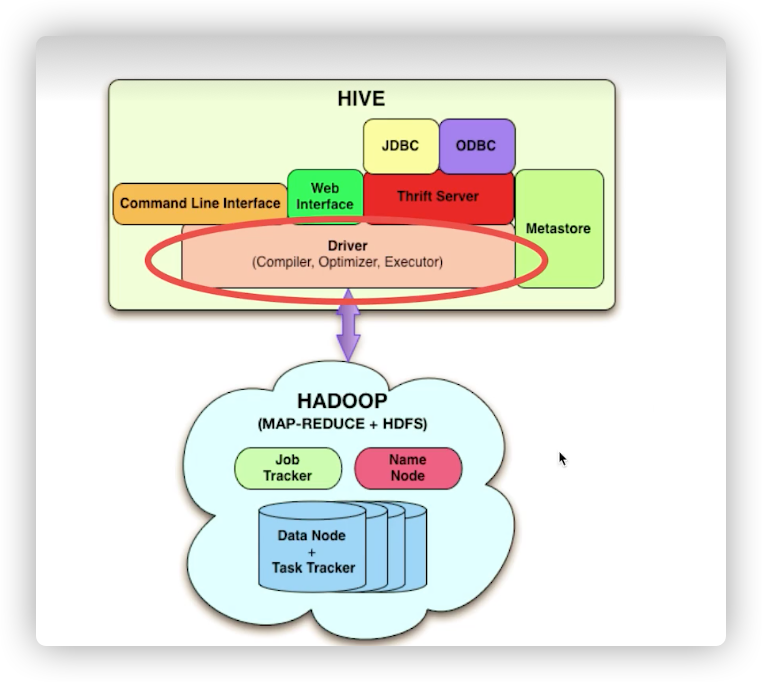
编译、优化、执行
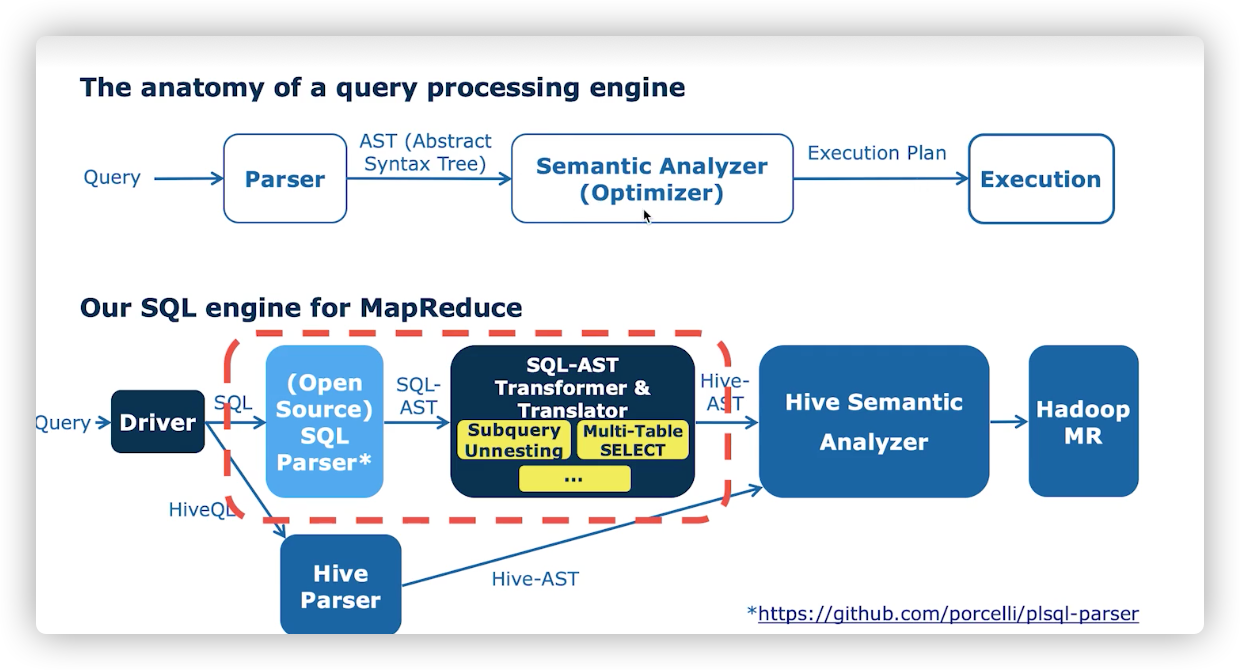
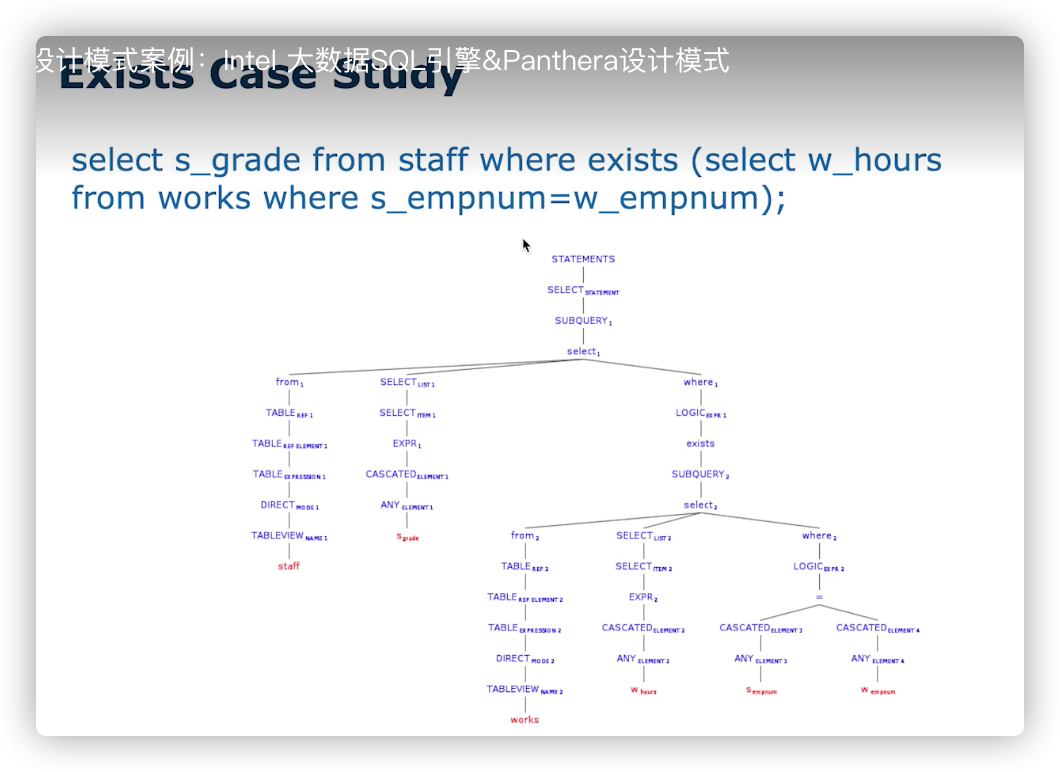
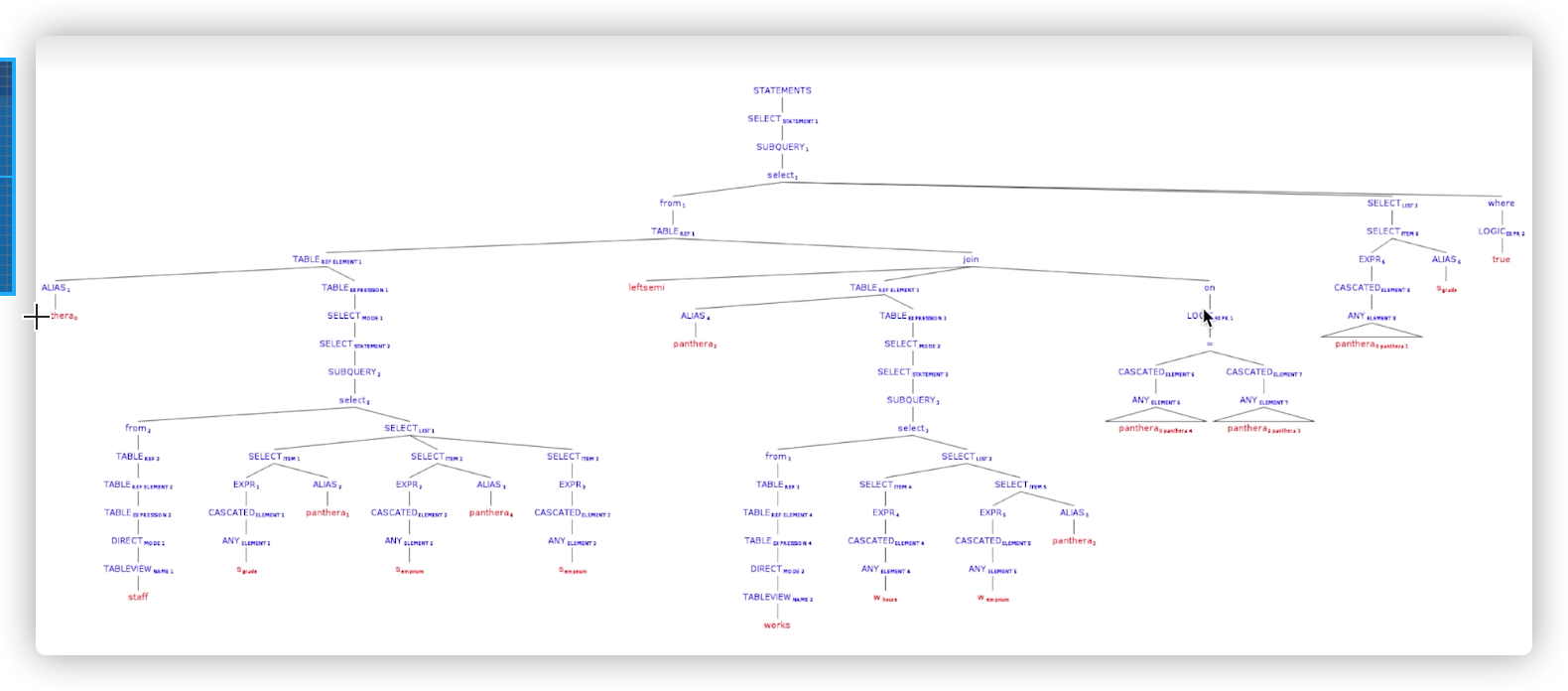
3.7 第三周课后练习
作业一:
1. 请在草稿纸上手写一个单例模式的实现代码,拍照提交作业。
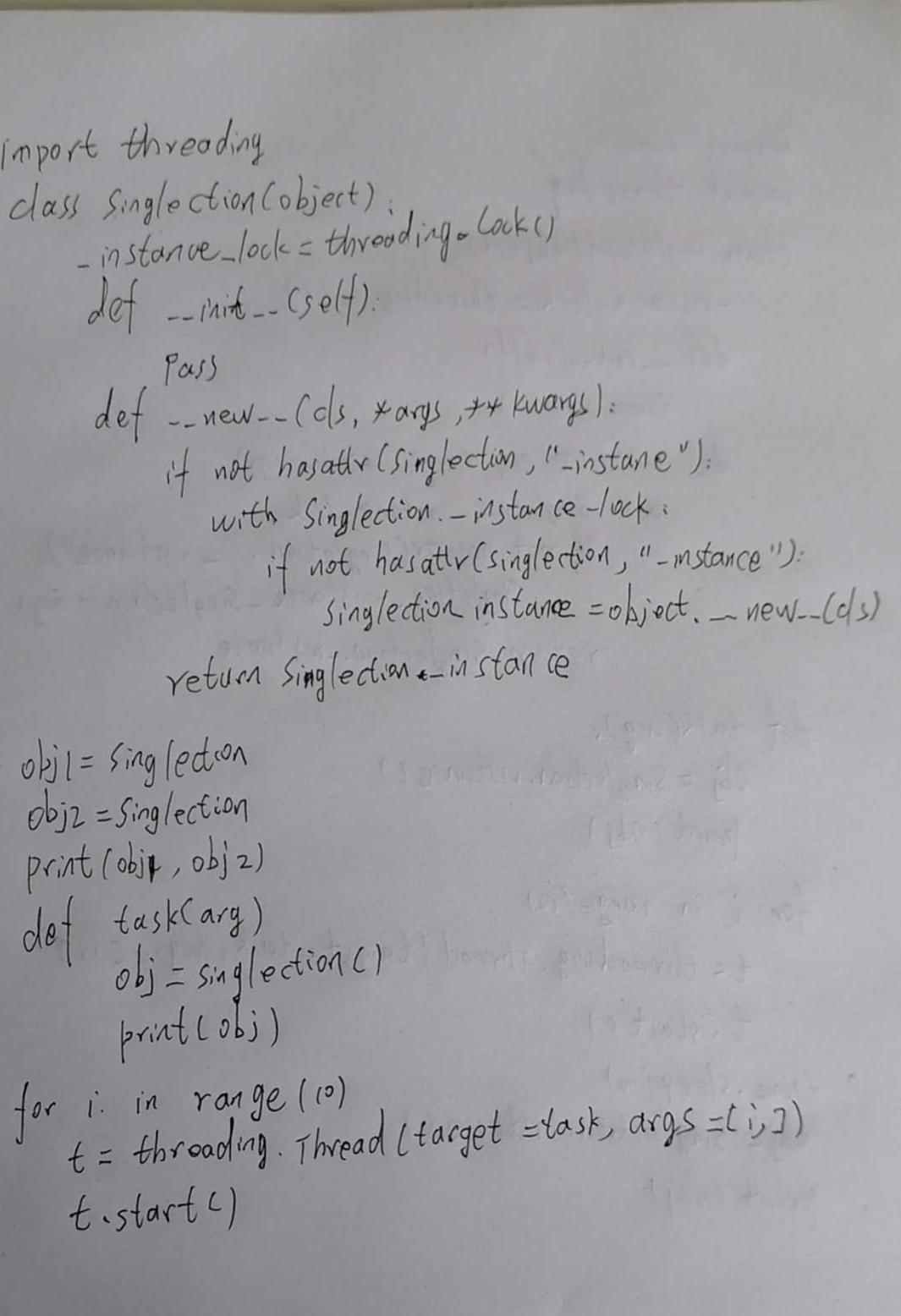
2. 请用组合设计模式编写程序,打印输出图 1 的窗口,窗口组件的树结构如图 2 所示,打印输出示例参考图 3。
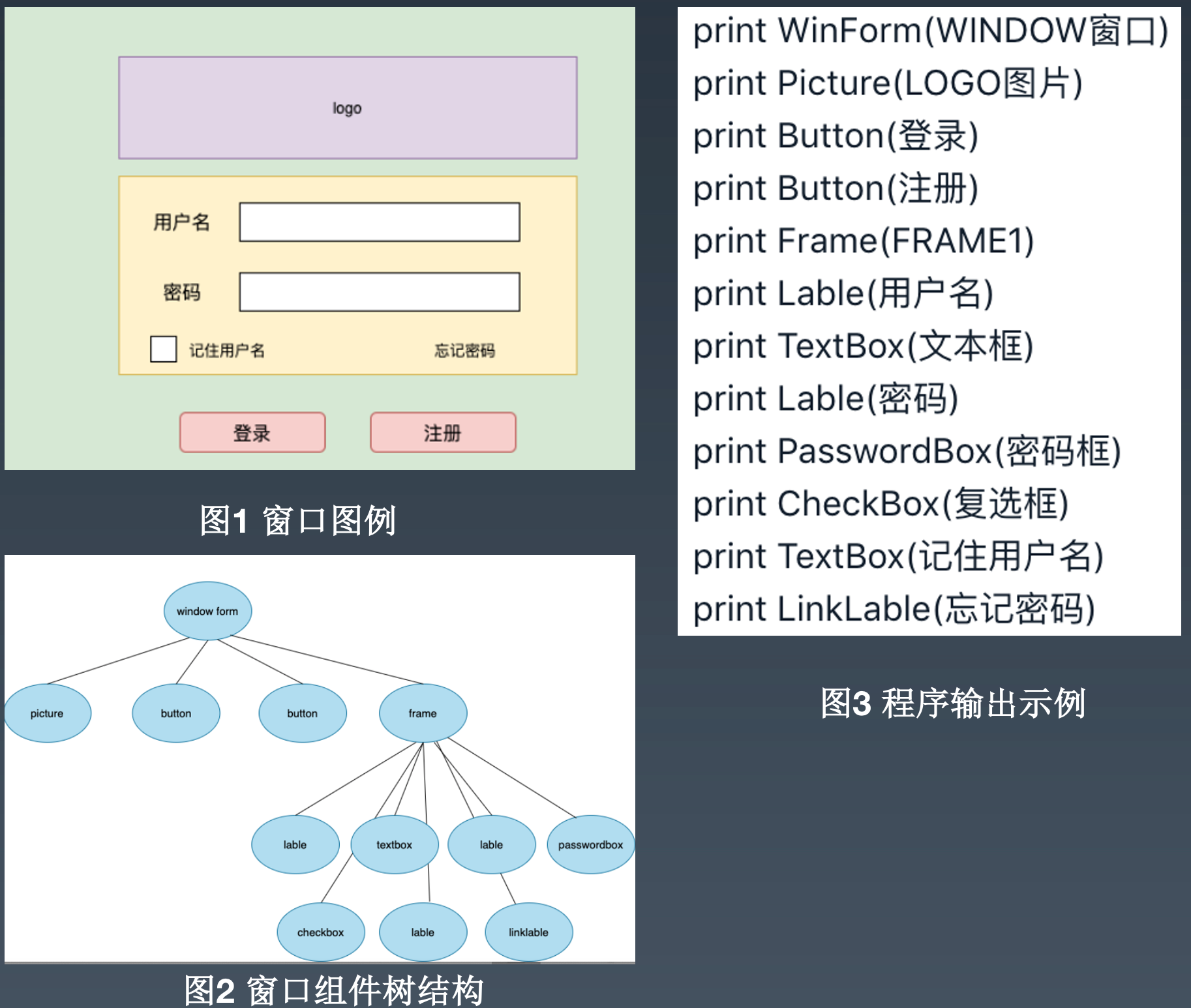
作业二:
根据当周学习情况,完成一篇学习总结
作业提交:
作业提交地址: https://jinshuju.net/f/K2tVxc
评论 (1 条评论)