「架构师训练营」第 3 周作业
发布于: 2020 年 11 月 07 日
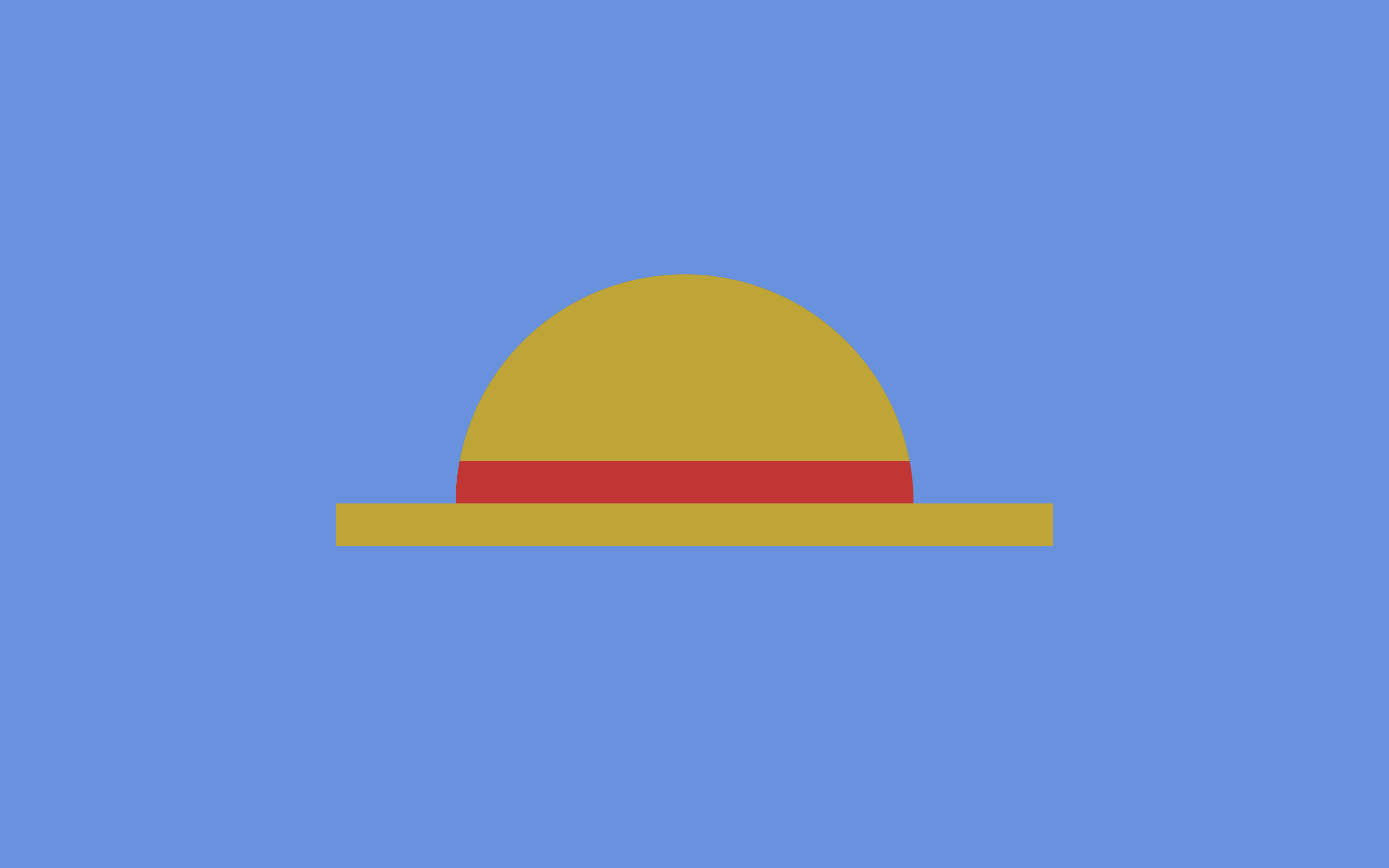
请在草稿纸上手写一个单例模式的实现代码,拍照提交作业。
请用组合设计模式编写程序,打印输出图 1 的窗口,窗口组件的树结构如图 2 所示,打印输出示例参考图 3。
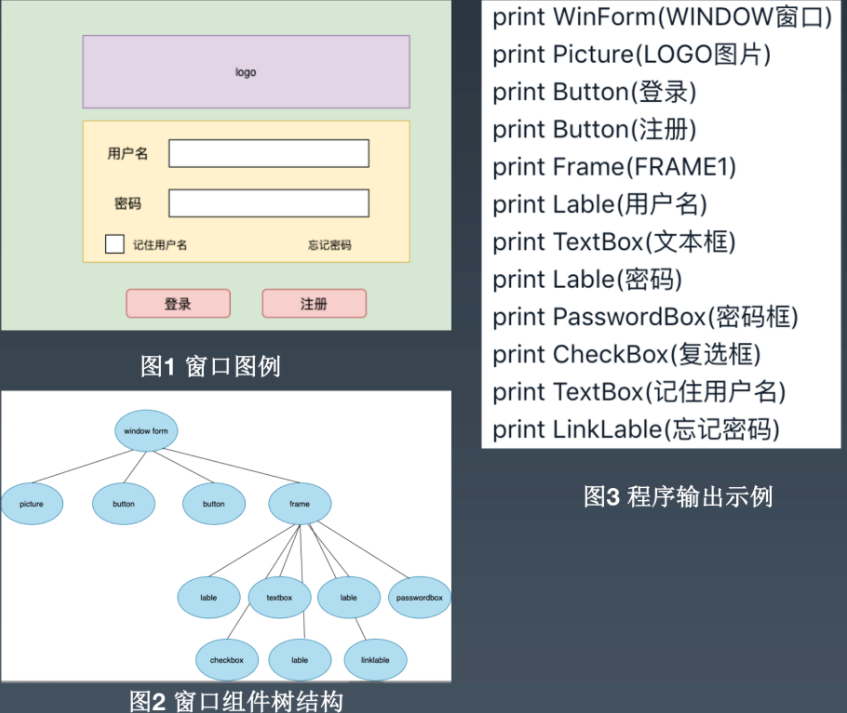
1.
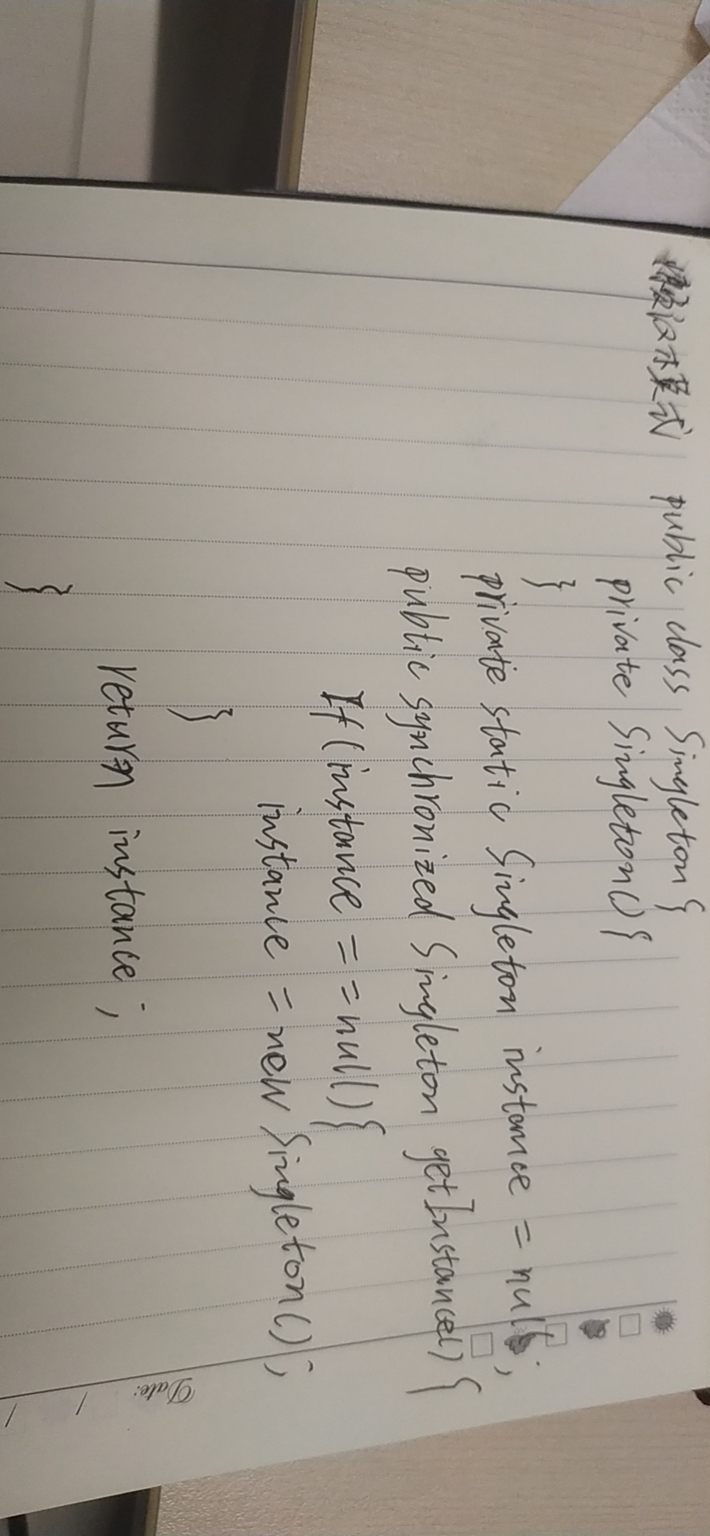
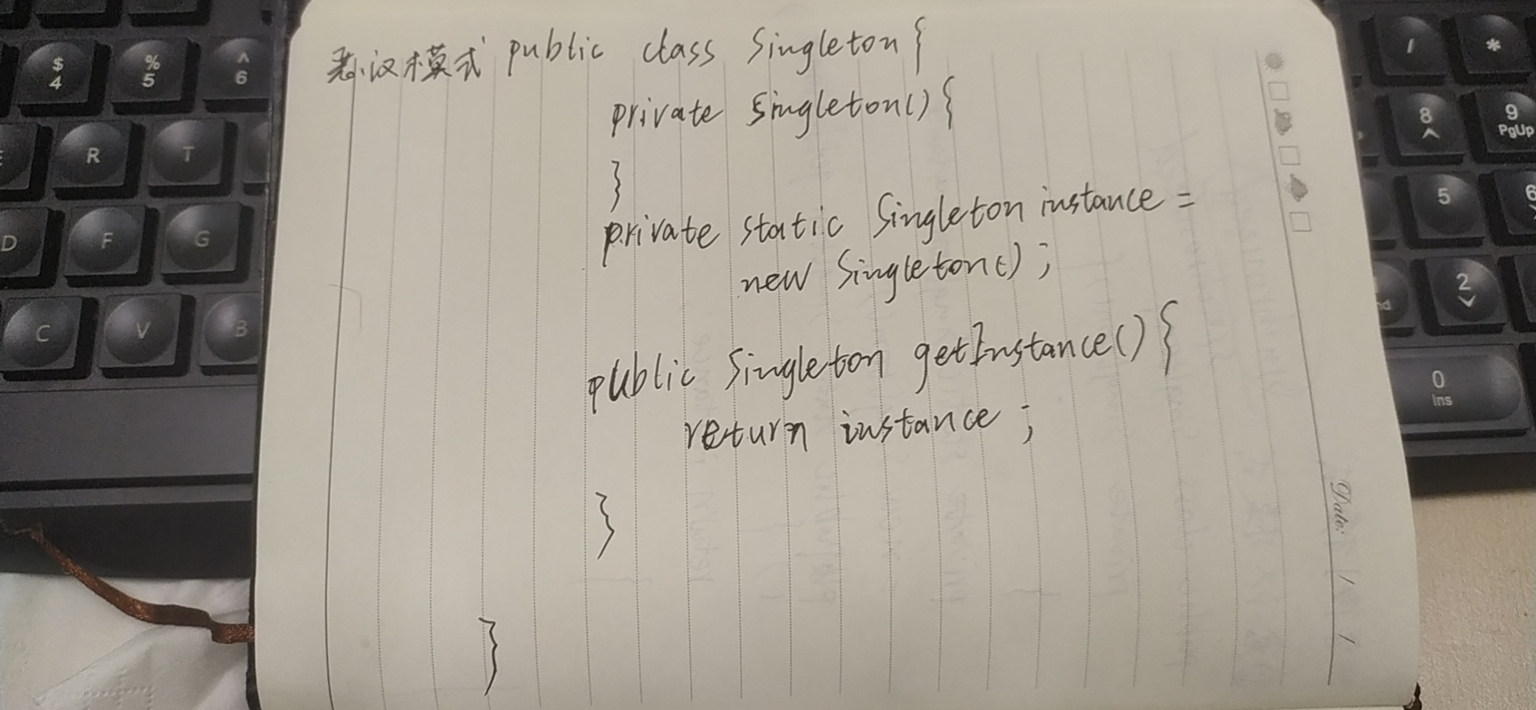
2.
根节点接口:
package cn.edu.ldu.test;import java.util.ArrayList;/** * 定义分支节点(窗口) */public interface IBranch { /** * 打印分支节点信息 * @return */ public String print(); /** * 增加分支节点 * @param branch */ public void addBranch(IBranch branch); /** * 增加叶子节点 * @param leaf */ public void addLeaf(ILeaf leaf); /** * 获得子集 * @return */ public ArrayList getChildren();}
叶子节点接口:
package cn.edu.ldu.test;/** * 定义叶子节点 */public interface ILeaf { /** * 打印叶子节点的信息 * @return */ public String print();}
窗口实现:
package cn.edu.ldu.test;import java.util.ArrayList;public class Window implements IBranch{ /** * 组件 */ private String component; /** * 组件展示内容 */ private String text; /** * 子集 */ private ArrayList children = new ArrayList(); public Window(String component,String text) { this.component = component; this.text = text; } @Override public String print() { // TODO Auto-generated method stub return "print " + component + "(" + text + ")"; } @Override public void addBranch(IBranch branch) { // TODO Auto-generated method stub children.add(branch); } @Override public void addLeaf(ILeaf leaf) { // TODO Auto-generated method stub children.add(leaf); } @Override public ArrayList getChildren() { // TODO Auto-generated method stub return children; } }
叶子节点实现:
package cn.edu.ldu.test;public class LeafPrint implements ILeaf{ /** * 组件 */ private String component; /** * 组件展示内容 */ private String text; public LeafPrint(String component,String text) { // TODO Auto-generated constructor stub this.component = component; this.text = text; } @Override public String print() { // TODO Auto-generated method stub return "print " + component + "(" + text + ")"; }}
测试类:
package cn.edu.ldu.test;import java.util.ArrayList;import cn.edu.ldu.util.File;public class ClientTest { public static void main(String[] args) { //定义窗口 IBranch window = new Window("winform","window窗口"); //定义logo ILeaf logo = new LeafPrint("Picture","logo图片"); //定义frame IBranch frame = new Window("frame","frame1"); //定义frame内部内容 ILeaf lable = new LeafPrint("lable","用户名"); ILeaf textBox = new LeafPrint("textBox","文本框"); ILeaf pwdLable = new LeafPrint("lable","密码"); ILeaf pwdBox = new LeafPrint("passwordbox","密码框"); ILeaf checkbox = new LeafPrint("CheckBox","复选框"); ILeaf remtextBox = new LeafPrint("textBox","记住用户名"); ILeaf linkLable = new LeafPrint("LinkLable","忘记密码"); //定义按钮 ILeaf loginButton = new LeafPrint("Button","登录"); ILeaf regButton = new LeafPrint("Button","注册"); window.addLeaf(logo); window.addBranch(frame); window.addLeaf(loginButton); window.addLeaf(regButton); frame.addLeaf(lable); frame.addLeaf(textBox); frame.addLeaf(pwdLable); frame.addLeaf(pwdBox); frame.addLeaf(checkbox); frame.addLeaf(remtextBox); System.out.println(window.print()); //打印出来 getChildrenInfo(window.getChildren()); } /** * 递归遍历组件 * @param arrayList */ private static void getChildrenInfo(ArrayList arrayList){ int length = arrayList.size(); for(int m = 0;m<length;m++){ Object item = arrayList.get(m); //如果是叶子节点就直接打印出来名称 if(item instanceof ILeaf){ System.out.println(((ILeaf) item).print()); }else { //如果是分支节点就先打印分支节点的名称,再递归遍历子节点 System.out.println(((IBranch)item).print()); getChildrenInfo(((IBranch)item).getChildren()); } } }}
打印结果:
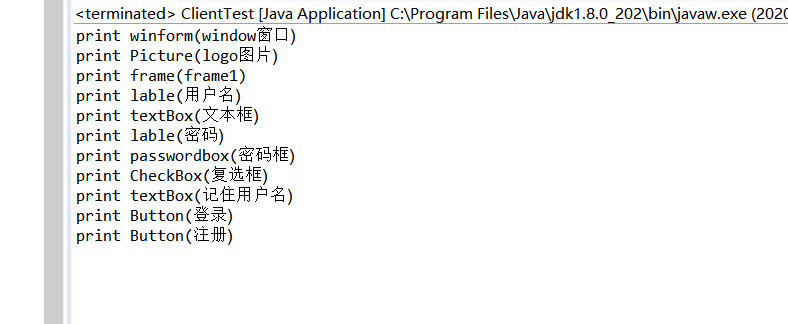
划线
评论
复制
发布于: 2020 年 11 月 07 日阅读数: 27
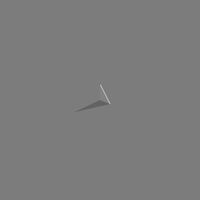
小黄鱼
关注
码农 2018.12.27 加入
还未添加个人简介
评论