架构学习(三)作业
发布于: 2020 年 06 月 24 日
手写一个单例模式
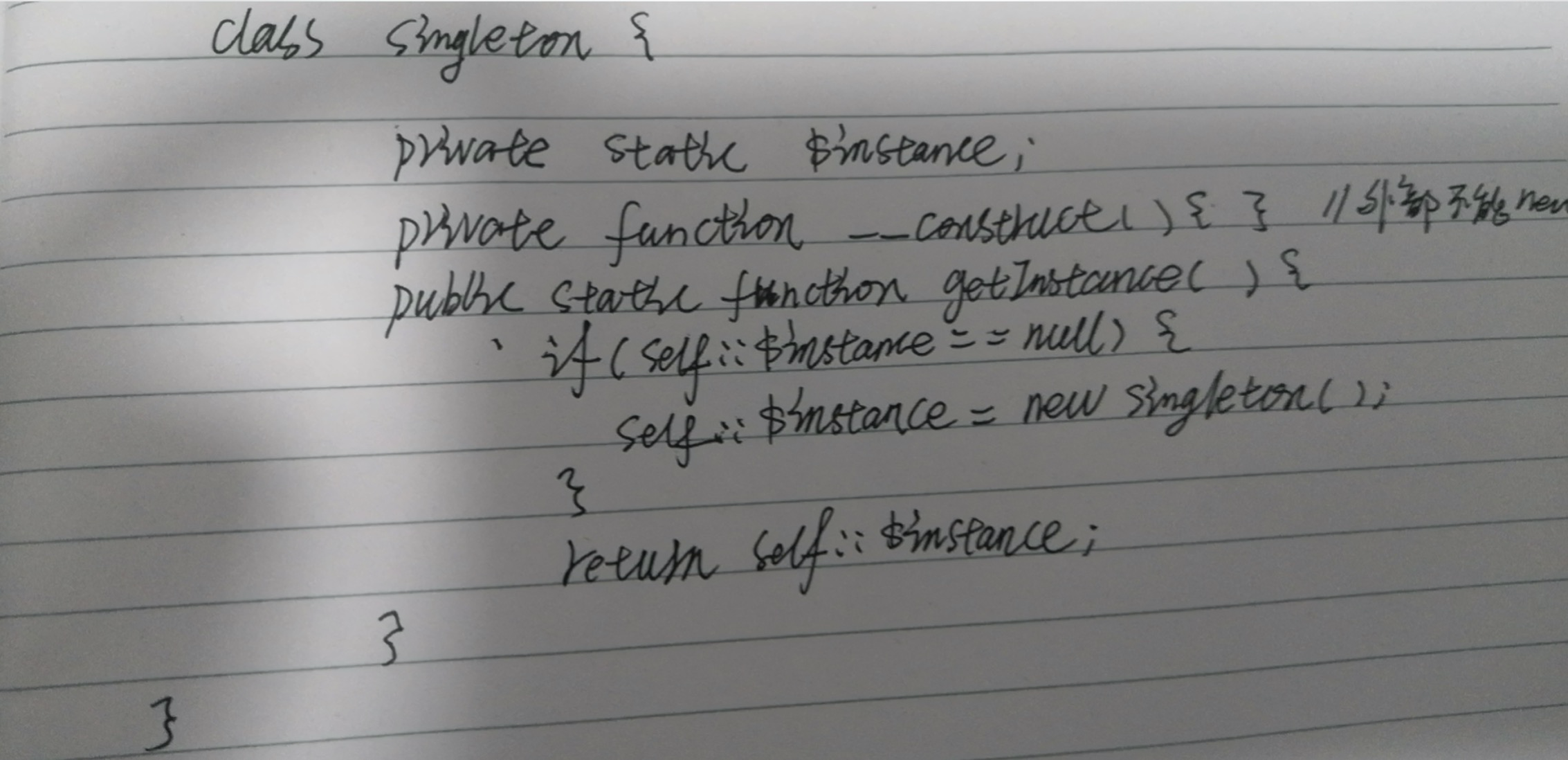
组合设计模式编写程序
请用组合设计模式编写程序,打印输出图 1 的窗口,窗口组件的树结构如图 2 所示,打印输出示例参考图 3。
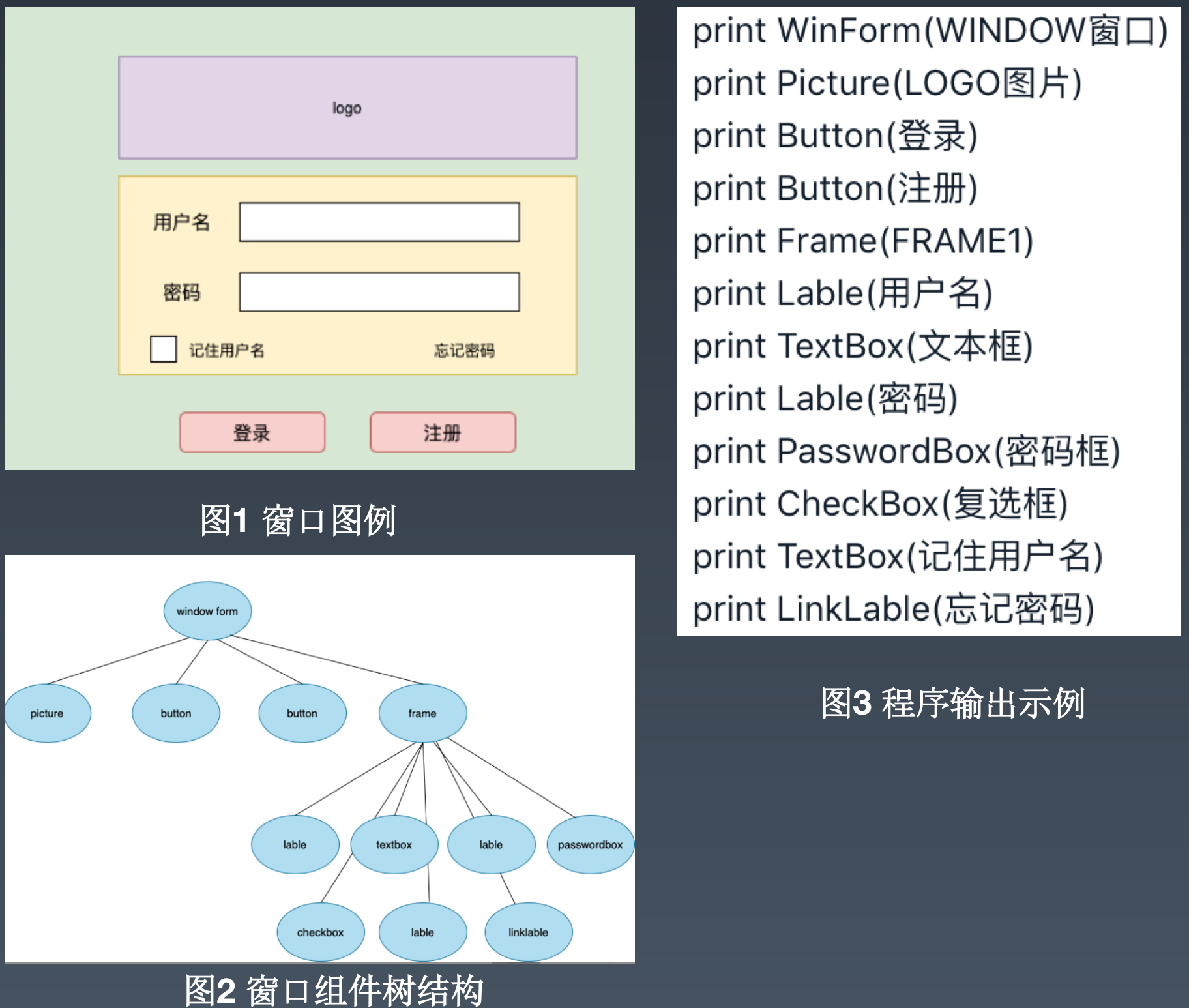
参考图
<?php/** * Created by PhpStorm. * User: ruicewang * Date: 2020/6/24 * Time: 4:10 PM */interface IConponent { function add(IConponent $conponent); function fprint();}class Window implements IConponent { private $conponentList = []; private $name; public function __construct($name) { $this->name = $name; } public function add(IConponent $conponent) { array_push($this->conponentList,$conponent); } function fprint() { echo "print WinForm({$this->name})\n"; if($this->conponentList){ foreach ($this->conponentList as $conponent) { $conponent->fprint(); } } }}class Picture implements IConponent { private $conponentList = []; private $name; public function __construct($name) { $this->name = $name; } public function add(IConponent $conponent) { array_push($this->conponentList,$conponent); } function fprint() { echo "print Picture({$this->name})\n"; if($this->conponentList){ foreach ($this->conponentList as $conponent) { $conponent->fprint(); } } }}class Button implements IConponent { private $conponentList = []; private $name; public function __construct($name) { $this->name = $name; } public function add(IConponent $conponent) { array_push($this->conponentList,$conponent); } function fprint() { echo "print Button({$this->name})\n"; if($this->conponentList){ foreach ($this->conponentList as $conponent) { $conponent->fprint(); } } }}class Frame implements IConponent { private $conponentList = []; private $name; public function __construct($name) { $this->name = $name; } public function add(IConponent $conponent) { array_push($this->conponentList,$conponent); } function fprint() { echo "print Frame({$this->name})\n"; if($this->conponentList){ foreach ($this->conponentList as $conponent) { $conponent->fprint(); } } }}class Lable implements IConponent { private $conponentList = []; private $name; public function __construct($name) { $this->name = $name; } public function add(IConponent $conponent) { array_push($this->conponentList,$conponent); } function fprint() { echo "print Lable({$this->name})\n"; if($this->conponentList){ foreach ($this->conponentList as $conponent) { $conponent->fprint(); } } }}class TextBox implements IConponent { private $conponentList = []; private $name; public function __construct($name) { $this->name = $name; } public function add(IConponent $conponent) { array_push($this->conponentList,$conponent); } function fprint() { echo "print TextBox({$this->name})\n"; if($this->conponentList){ foreach ($this->conponentList as $conponent) { $conponent->fprint(); } } }}class PasswordBox implements IConponent { private $conponentList = []; private $name; public function __construct($name) { $this->name = $name; } public function add(IConponent $conponent) { array_push($this->conponentList,$conponent); } function fprint() { echo "print PasswordBox({$this->name})\n"; if($this->conponentList){ foreach ($this->conponentList as $conponent) { $conponent->fprint(); } } }}class Checkbox implements IConponent { private $conponentList = []; private $name; public function __construct($name) { $this->name = $name; } public function add(IConponent $conponent) { array_push($this->conponentList,$conponent); } function fprint() { echo "print Checkbox({$this->name})\n"; if($this->conponentList){ foreach ($this->conponentList as $conponent) { $conponent->fprint(); } } }}class Linklable implements IConponent { private $conponentList = []; private $name; public function __construct($name) { $this->name = $name; } public function add(IConponent $conponent) { array_push($this->conponentList,$conponent); } function fprint() { echo "print Linklable({$this->name})\n"; if($this->conponentList){ foreach ($this->conponentList as $conponent) { $conponent->fprint(); } } }}$windowObject = new Window('WINDOW窗口');$windowObject->add(new Picture("LOGO图片"));$windowObject->add(new Button("登录"));$windowObject->add(new Button("注册"));$frameObject = new Frame("FRAME1");$frameObject->add(new Lable('用户名'));$frameObject->add(new TextBox('文本框'));$frameObject->add(new Lable('密码'));$frameObject->add(new PasswordBox('密码框'));$frameObject->add(new Checkbox('复选框'));$frameObject->add(new TextBox('记住用户名'));$frameObject->add(new Linklable('忘记密码'));$windowObject->add($frameObject);$windowObject->fprint();
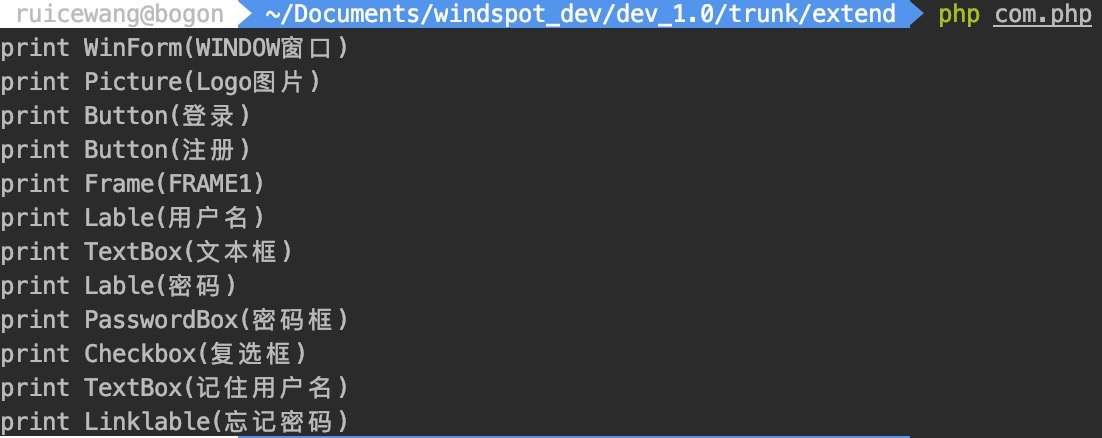
打印结果
总结:
Compose objects into tree structure to represent part-whole hierarchies.Composite lets client treat individual objects and compositions of objects uniformly.
翻译:将一组对象组织(Compose)成树形结构,以表示一种“部分 - 整体”的层次结构。组合让客户端(在很多设计模式书籍中,“客户端”代指代码的使用者。)可以统一单个对象和组合对象的处理逻辑。
组合模式的设计思路,与其说是一种设计模式,倒不如说是对业务场景的一种数据结构和算法的抽象。其中,数据可以表示成树这种数据结构,业务需求可以通过在树上的递归遍历算法来实现。组合模式,将一组对象组织成树形结构,将单个对象和组合对象都看做树中的节点,以统一处理逻辑,并且它利用树形结构的特点,递归地处理每个子树,依次简化代码实现。使用组合模式的前提在于,你的业务场景必须能够表示成树形结构。所以,组合模式的应用场景也比较局限,它并不是一种很常用的设计模式。
划线
评论
复制
发布于: 2020 年 06 月 24 日阅读数: 50
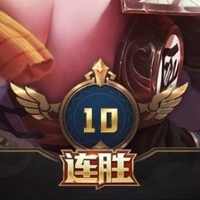
漫步跑小鸡
关注
还未添加个人签名 2018.11.17 加入
还未添加个人简介
评论