MyBatis 专栏 - 关联查询中的延迟加载
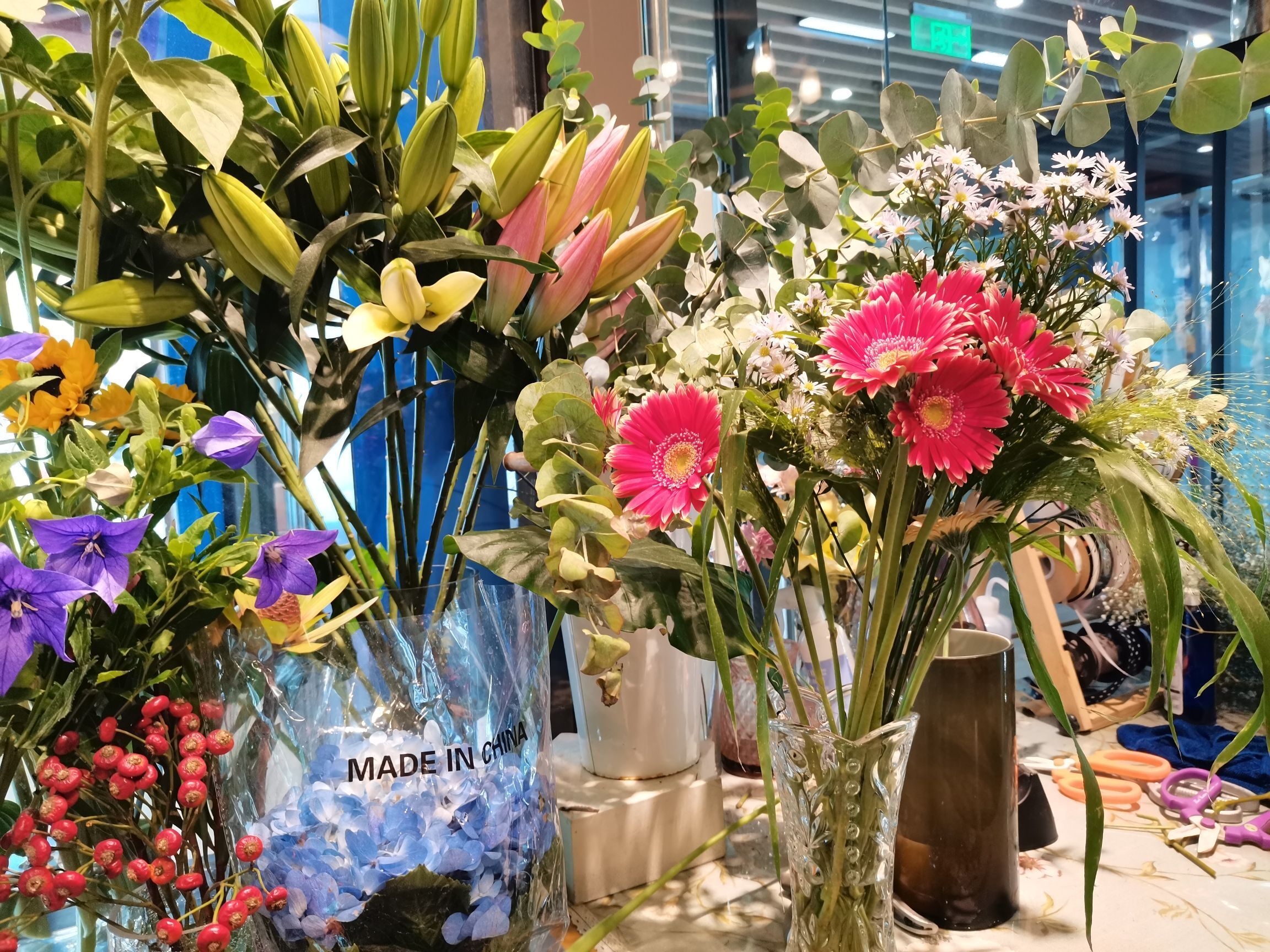
MyBatis 延迟加载
总结: 核心是: 在需要延迟查询的 association 或者 collection 中配置 select 和 fetchType 属性
前者 select 配置的是要延迟查询的 dao 方法
后者 fetchType 属性配置的是要 eager 或者 lazy 加载, 默认是 eager
作用: 需要的时候才查询, 减少数据库压力
需求: 查询 Account 与关联表 User, 测试延迟加载 User
代码示例
1, 准备两张表
复制代码
2, 实体 Bean
实体 Bean: Account
复制代码
实体 Bean: User
复制代码
3, Dao
Dao 接口: AccountDao
复制代码
Dao 接口: UserDao
复制代码
4, Mapper 配置文件
Mapper 配置文件: AccountDao.xml
延迟加载的配置关键: 在 Account 的自定义映射关系中, 设置延迟加载的 User
复制代码
Mapper 配置文件: UserDao.xml
复制代码
5, 测试
分别进行两次测试, 代码一样, 不同之处是修改 AccountDao.xml 的 fetchType 配置, 分别测试并查看日志输出
复制代码
fetchType="lazy"的测试结果, 即, 开启了延迟加载的情况, 可以看到进行了一次 SQL 查询.(打开上面代码的注释, 才会发送第二条 SQL)
复制代码
fetchType="eager"的测试结果, 可以看到进行了两次 SQL 查询(关掉代码的注释, 但是依然会自动发送对关联表的查询)
复制代码
版权声明: 本文为 InfoQ 作者【小马哥】的原创文章。
原文链接:【http://xie.infoq.cn/article/8366f69f486215e2a89788e76】。文章转载请联系作者。
评论