1
「架构师训练营」第 3 周作业 - 模式与重构
发布于: 2020 年 06 月 23 日
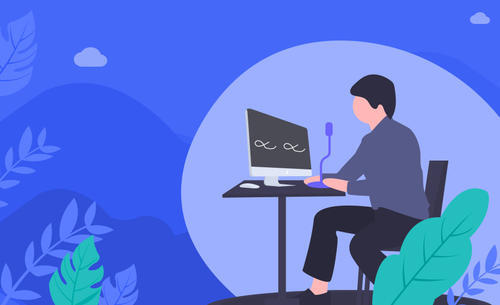
作业一:请在草稿纸上手写一个单例模式的实现代码,拍照提交作业。
对于这个作业,为了检验自己对代码的熟悉程度,在写之前,刻意的没有上网查,完全凭记忆和意识,手写了代码。
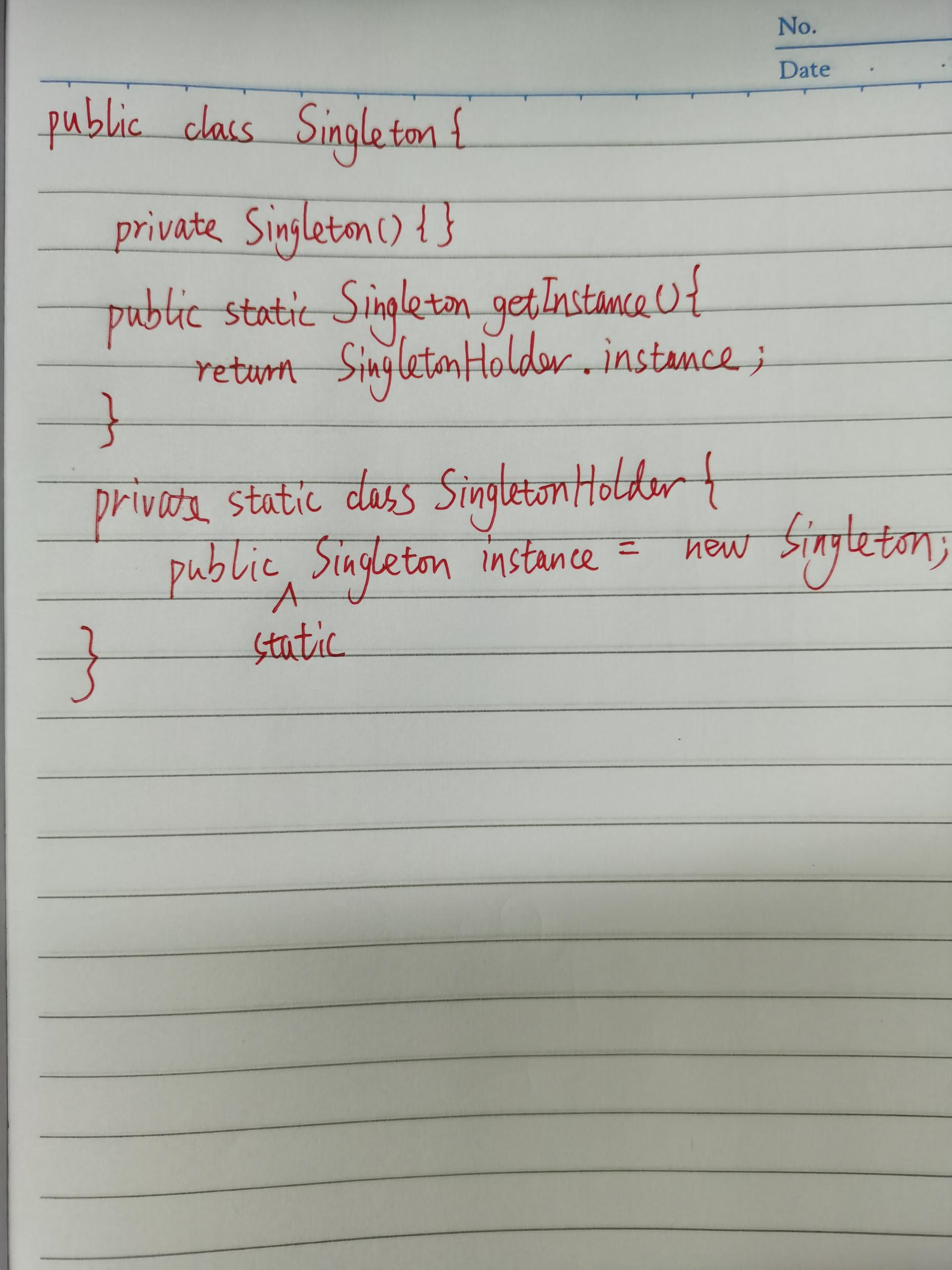
使用静态内部类的方法来写单例模式,让jvm让为我们保证线程安全性。只是没使用到静态内部类,就不会去创建对象实例,从而实现了延迟加载和线程安全。
作业二:请用组合设计模式编写程序,打印输出图 1 的窗口,窗口组件的树结构如图 2 所示,打印输出示例参考图 3
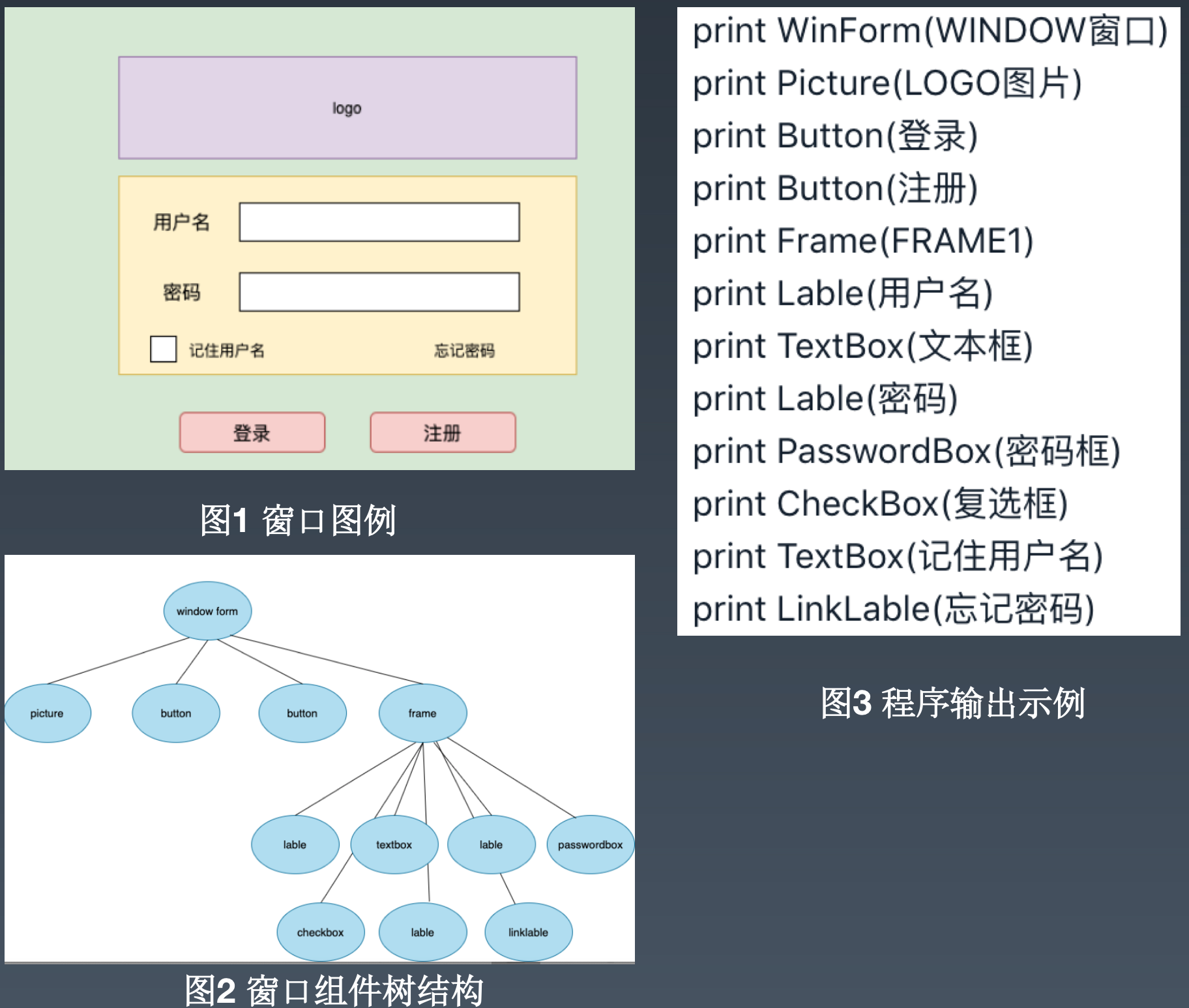
UML类
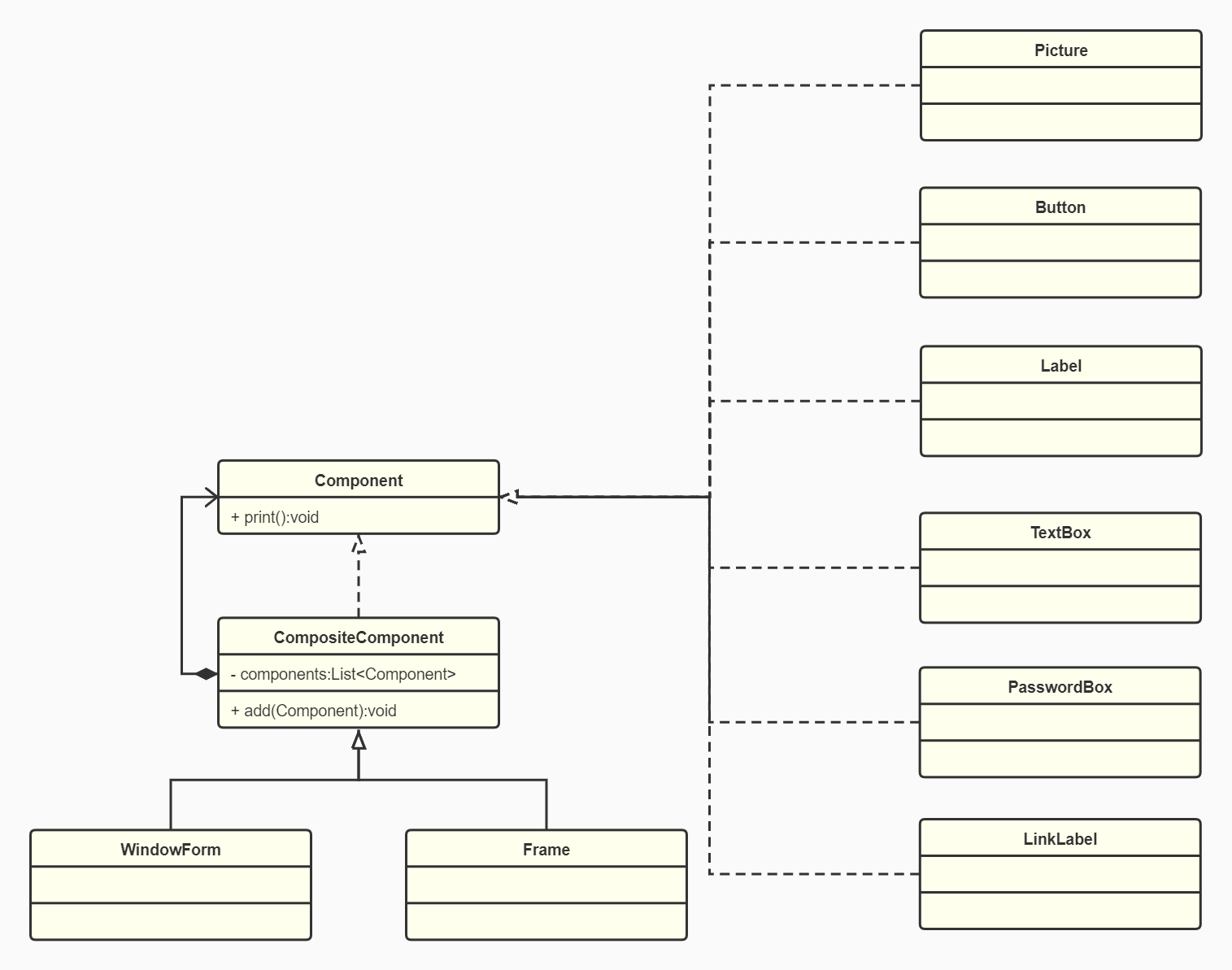
首先定义一个`Component`接口,作为所有窗体组件的抽象,所有的窗体组件均实现此接口的print方法完成打印。
public interface Component { void print();}
有的组件有子组件,有的没有子组件。考虑接口隔离原则,抽象一个含有子组件的抽象类:
public abstract class CompositeComponent implements Component { private String name; public CompositeComponent(String name) { this.name = name; } private List<Component> components = new ArrayList<>(); public CompositeComponent add(Component component) { components.add(component); return this; } @Override public void print() { // 打印父组件 System.out.println(String.format("print %s", name)); // 打印子组件 for (Component component : components) { component.print(); } }}
WindowForm类
public class WindowForm extends CompositeComponent { public WindowForm(String name) { super(name); }}
Picture类
public class Picture implements WindowForm { private String logoPath; public Picture(String logoPath) { this.logoPath = logoPath; } /** * 打印Picture */ @Override public void print() { System.out.println(String.format("print Picture(%s)", logoPath)); }}
Button类
public class Button implements Component { private String name; public Button(String name) { this.name = name; } @Override public void print() { System.out.println(String.format("print Button(%s)", name)); }}
Frame类
public class Frame extends CompositeComponent { public Frame(String name) { super(name); }}
Label类
public class Label implements Component { private String text; public Label(String text) { this.text = text; } @Override public void print() { System.out.println(String.format("print Label(%s)", text)); }}
TextBox类
public class TextBox implements Component { private String placeHolder; public TextBox(String placeHolder) { this.placeHolder = placeHolder; } @Override public void print() { System.out.println(String.format("print TextBox(%s)", placeHolder)); }}
PasswordBox类
public class PasswordBox implements Component { private String placeHolder; public PasswordBox(String placeHolder) { this.placeHolder = placeHolder; } @Override public void print() { System.out.println(String.format("print TextBox(%s)", placeHolder)); }}
CheckBox类
public class CheckBox implements Component { @Override public void print() { System.out.println("print CheckBox(复选框)"); }}
LinkLabel类
public class LinkLabel implements Component { private String text; public LinkLabel(String text) { this.text = text; } @Override public void print() { System.out.println(String.format("print LinkLabel(%s)", text)); }}
客户端测试类:
public class Client { public static void main(String[] args) { CompositeComponent winForm = new WindowForm("WinForm(WINDOW窗口)"); Component picture = new Picture("LOGO图片"); Component loginButton = new Button("登录"); Component registerButton = new Button("注册"); CompositeComponent frame = new Frame("Frame(FRAME1)"); Component usernameLabel = new Label("用户名"); Component usernameTextBox = new TextBox("请输入用户名"); Component passwordLabel = new Label("密码"); Component passwordBox = new PasswordBox("请输入密码"); Component checkbox = new CheckBox(); Component rememberTextBox = new TextBox("记住用户名"); Component linkLabel = new LinkLabel("忘记密码"); frame.add(usernameLabel).add(usernameTextBox).add(passwordLabel).add(passwordBox).add(checkbox).add(rememberTextBox).add(linkLabel); winForm.add(picture).add(loginButton).add(registerButton).add(frame).print(); }}
输出结果:
print WinForm(WINDOW窗口)print Picture(LOGO图片)print Button(登录)print Button(注册)print Frame(FRAME1)print Label(用户名)print TextBox(请输入用户名)print Label(密码)print TextBox(请输入密码)print CheckBox(复选框)print TextBox(记住用户名)print LinkLabel(忘记密码)
划线
评论
复制
发布于: 2020 年 06 月 23 日 阅读数: 58
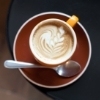
guoguo 👻
关注
还未添加个人签名 2017.11.30 加入
还未添加个人简介
评论 (2 条评论)