SpringBoot 系列 (四):SpringBoot 特性 _ 外部化配置 (properties 文件配置)
SpringBoot允许将配置进行外部化(externalize),这样你就能够在不同的环境下使用相同的代码。你可以使用properties文件,yaml文件,环境变量和命令行参数来外部化配置。使用@Value注解,可以直接将属性值注入到beans中,然后通过Spring的Environment抽象或通过@ConfigurationProperties绑定到结构化对象来访问。
SpringBoot设计了一个非常特别的加载指定属性文件的顺序(@PropertySource),以允许对属性值进行合理的覆盖,属性会以如下的顺序进行设值:
home目录下的devtools全局设置属性(~/.spring-boot-devtools.properties,如果devtools激活)。
测试用例上的@TestPropertySource注解。
测试用例上的@SpringBootTest#properties注解。
命令行参数.
来自SPRING_APPLICATION_JSON的属性(环境变量或系统属性中内嵌的内联JSON)。
ServletConfig初始化参数。
ServletContext初始化参数。
来自于java:comp/env的JNDI属性。
Java系统属性(System.getProperties())。
操作系统环境变量。
RandomValuePropertySource,只包含random.*中的属性。
没有打进jar包的Profile-specific应用属性(application-{profile}.properties和YAML变量)。
打进jar包中的Profile-specific应用属性(application-{profile}.properties和YAML变量)。
没有打进jar包的应用配置(application.properties和YAML变量)。
打进jar包中的应用配置(application.properties和YAML变量)。
@Configuration类上的@PropertySource注解。
默认属性(使用SpringApplication.setDefaultProperties指定)。
1、配置随机值
SpringBoot支持在系统加载时配置生成随机数,比如生成密钥或用于测试时,是非常有用的。
(1)在src/main/resources/config/新建random.properties文件,添加内容如下:
(2)新建配置绑定类
springboot/src/main/java/com/xcbeyond/springboot/config/RandomConfig.java
(3)在control中添加测试方法,进行验证。
springboot/src/main/java/com/xcbeyond/springboot/controller/ControllerDemo.java
访问http://localhost:8888/randomSecret,显示如下:
2、application.properties文件
SpringApplication将从以下目录顺序加载application.properties文件,并把它们添加到Spring 环境中:
当前目录下的/config子目录。
当前目录。
classpath下的/config包。
classpath根路径(root)。
注: 你可以使用‘*.yml’文件替代’*.properties’。
如果不喜欢使用默认application.properties作为配置文件名,则可以自定义properties配置文件名,在SpringApplication中加载该配置文件。
3、Profile-specific属性
除了application.properties文件,profile-specific属性也能通过命名惯例application-{profile}.properties定义。Environment(Spring的环境抽象接口)有个默认profiles集合(默认情况为[default]),在没有设置激活的profiles时会被使用(例如,如果没有明确指定激活的profiles,application-default.properties中的属性会被加载)。
Profile-specific属性加载路径和标准的application.properties相同,并且profile-specific文件总是会覆盖non-specific文件,不管profile-specific文件是否被打包到jar中。
如果定义多个profiles,最后一个将获胜。例如,spring.profiles.active定义的profiles被添加到通过SpringApplicationAPI定义的profiles后面,因此优先级更高。
4、属性占位符
当使用application.properties配置文件定义的属性时,Spring会先通过已经存在的环境变量中查找该属性,所以你可以使用属性占位符"${}"引用已定义的值(比如,系统属性):
5、使用YAML代替Properties
yaml,它是一种直观的能够被电脑识别的数据序列化格式,也是一种方便的定义层次配置数据的格式。
在Eclipse中通过Spring插件可直接完成properties转化为yaml,转化结果如下:
(在application.properties文件右键 Convert .properties to .yaml进行转化)
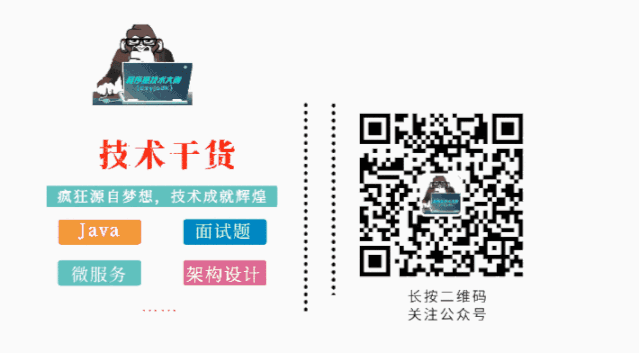
版权声明: 本文为 InfoQ 作者【xcbeyond】的原创文章。
原文链接:【http://xie.infoq.cn/article/7d3b2030e151726dd52aa79d0】。文章转载请联系作者。
评论