训练营第五周作业
java实现一致性hash算法:
思路:
路由器要支持Redis实例的增加和删除
路由器要支持根据Key筛选到Redis实例
路由器分布要均衡即,Redis的字节点要在环上分布较为均匀,不能过于集中和分散
路由器不能随便实例化
路由器中的Redis实例新增删除要考虑同步
类图
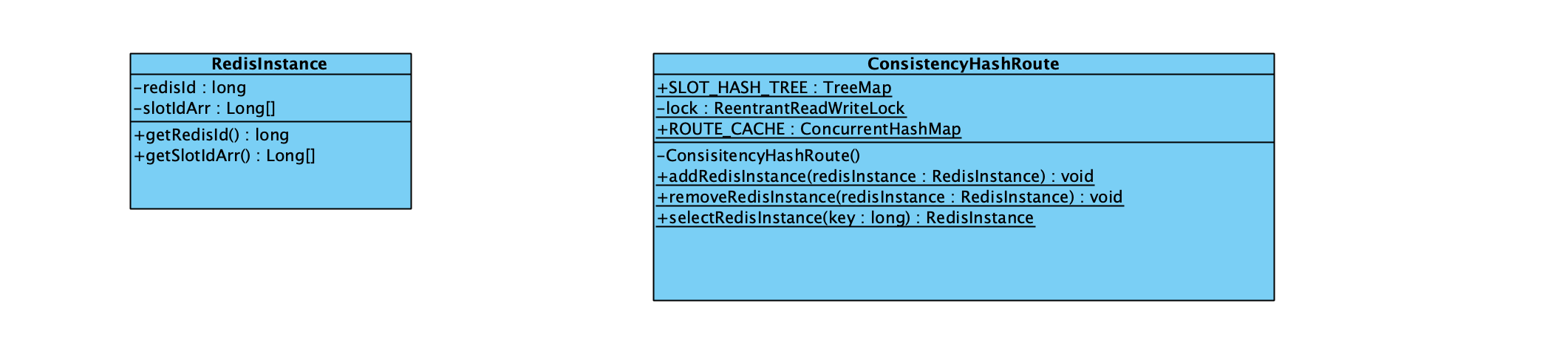
代码:
测试用例
输出结果:
RedisInstance{atomicInteger=96385, redisId=8925593982998215863, slodIdArr=[4229316014918879869, 5965414412871466017, -6659814546986260721, -6453658601752703448, 9011306268465363250, 7543815664919806262, 4543229078138835421, -2166569491806417717, -2087920291943852466, -1395394436409058014, 8866250177779653201, 2418481413401611296, -5312468882455169351, -8308108084997467969, -3615173161325284627, 8091797355203608968, 6376148855335427033, 9052000184876677194, 2993426964406897865, -8623823928302280062, 8154574014403115270, -4574335593270877423, -7870319705500826687, 5030394996190302837, -7866640927312976988, 696995112119396782, 1452574580457963933, -3145705797553505427, -2108453322860947642, -4238916899428172779, -778965604854549769, 3794090007631992318, 1104036785707033166, -2402213953687906785, 5730839525545765974, 4937510971913771479, 3778421491713176004, 7471872313869287201, -7597672824281341343, 315373072490665702, 8912226354711426849, -4293921830823234837, -3760698914023531913, -2834249073841912153, -4695797944118227319, -749448332952457247, 6369088048113945454, -658843941896111391, 7108100954515221623, 8633192909130480437, -7459231165467303593, -7040480894624283544, 7588546805897763224, 2147095850301118348, 1675665547107805124, -6242586932860959827, 7800001780818742589, 5116704852701501859, 72765652210286214, 5389882224259548721, 3119800670814251345, 3350110806178846856, -9106543418161603630, -3906021139957319264, -4038936114348886015, -275781075002888218, 3527383127527414657, -6464195467256984685, 656624513988611528, 8485863158667665665, 8112700184981352103, 6988207558447039562, -7119964963279425243, -6351840902716183042, 6047462848360365180, -4506797921410966040, 4777230683353103734, -5113985637198933290, -1114821365656610073, -3931530929821164576, 5279805307188669294, 8105675899009652148, 5834288502889673410, -159907821407715949, -3799584786305198448, 1997657726986170109, 8722520798541759048, 8892736148686511673, -3210197712210210327, 5244701951589890453, -6592017249223015130, -1344382597948849923, -6414062183810520732, 6918893556121426436, 1097442080310532472, -6718615720005012356, 5793179979547412413, -6468579168402138376, -6237809757570349452, -260133218743739792]}
RedisInstance{atomicInteger=98170, redisId=-2488275183406254265, slodIdArr=[979871065875282258, 4058064697150888358, 6500981412717534786, 9190651778928053308, 133250763666753650, 1373322337949318041, -3348070793721237482, -1772488720583489947, 693500545646791849, 8101064438115409361, 4507163302228387409, -8571957424206833935, -7439076641474398632, -4550349857858501241, -5841801985999589136, -4781762960117173917, 258025884506942415, 1967691890258637417, 3425204471484023168, 2326494973263230832, 5722705712327122936, -640695409894113242, 2475739271007311906, -8809383175977004621, -5327735460449867112, -981354758490495590, -3019596256735342959, -6645752762838325110, 6556690188579778340, 314550013800251595, 4305840024805534903, -7505502672678996615, -8701039159483658198, 6549394846038641464, 672403698218307941, 4838541115955460, 3790810241501622227, 6738040112408442541, 5272896231036912010, -516486571091647582, 3492323975634137257, 7382475541129001393, -905072484510747454, 7421579219219748648, -20938378627212769, 4916698279666735529, -872671061573192853, 6662293225221910992, -291576005929900420, -8400239182971740257, 8930104716532593066, -5171567317142069869, 5922633793126344001, 8524599797815709822, -1432183940578404567, 7738216085601000552, 3048966640387331990, -8917481527942680338, -8279521702077791444, -801428712155581328, 983184421808419143, -8424329002162106444, 4433719326408723035, 830557870330221541, -7957983944831498483, -535908273516749584, 877566040162588684, 9218674131603180355, 5556792390543445836, 9160272085201705075, 4722303475991784544, 6140276969888216338, 728588523116083644, 1103547519722400609, -8403480322384708424, 4415004761696236949, 3412929461483952063, -4286663235784200047, 2349645219789831012, 4411110826002353616, 491140371945392901, 1026133551633467423, -5713724222225542453, -4925245345665469747, -7134594191482070605, 3626731242246142467, 4088733719666712587, -1438326882607183029, -8950230142952963647, -1795868375309041525, 2892933412059248131, -6958259420532887168, -6043106300089426374, 6026474637670325601, -3124367824999598813, 5988269798282926485, 3791158731023154414, 1687644129877583952, 1590387701876560494, 1822345520841668795]}
RedisInstance{atomicInteger=96484, redisId=4756256533847647155, slodIdArr=[792606806857775439, -7479396538645779002, 2951111899726808683, -3779261023459888244, 5962031930092256463, 7248650616124503892, -8873015813397395215, 4066785869125288900, -824182838406456448, 5513334014906139620, 7245838407546610827, 8983785593767952674, -3901466683341541687, 467574417419276750, -2343402046969575202, -6816154098821554657, 7368621285989858088, -1869701730680674460, -2554861019590899669, 8370241834518419834, -1699716755665180188, 4249729716848059707, 1534910474445793328, -3016807444744881432, -3983810292779417007, 1934817188748144095, -1966554580765365177, -4539526167484516922, 2195563988941388956, -3507244798055832226, -7780045718915430273, -5340950220168163291, 1407931460852691170, -1801753154057308379, 5416982569503069864, -1426176930711738583, 564855139858636451, -3561424032351583643, -8403364421031538031, 3919618707214183780, -353282585491240023, -8194566582737960848, -6800484633308160587, -6235549053280603936, -2017926127241531614, 6828213571182905825, 8497397330452405485, 8481930544217878156, -2078879738356079253, -1120545614583655263, 7305276396649496796, -2635851137961741361, -4963328087849307457, -2821620977374992375, -8608928825908367636, -3711026166475301642, -1870961440328372910, 3818815615958046707, -2013354041835720806, 8887772624563281862, -6029163754597848511, -3149899844050702374, 4497451426586768531, 1183574454322993480, -3338315762813073269, -4633800451058589887, -8226358308442409004, -8175359195371826862, -6066848715427824393, -6372940088613404993, 3294110892209445747, 6707518737909123543, -757858990217046800, -3580996653549373774, -2547111509157075599, 5683799520487391191, -4713356030868745414, 4297908029420829823, 583177573118150373, 7186208942854607049, -6052547124646919406, -1539721006387841172, -836282352711732652, -7891842969662309572, -2492511552469981132, -4478119028929092707, -4667539476527858247, 1476158245673146759, -4979892247962624117, 5058877490667765723, -4985141210837479786, 1032222028780251761, -3440658077793332545, 3565817452486770846, -6598495760153001449, -4301090445757178429, 2437803366722438620, -6928598430498970748, 7717716703771312989, 1493255080880750729]}
RedisInstance{atomicInteger=95523, redisId=-1313256534556768642, slodIdArr=[-7948678255328526836, -4386757642939172663, -6333847233908373970, -6307664061916339872, -760999875083538594, 5331980379484800522, 6764047014173834624, -2158096960047259791, 183107312070129177, 7942968639220883352, 353419268178365004, 2801462856349120180, -6725694338025050973, 7421832271100544680, 734953655702085333, -4245116897159027211, 3110377132078692532, -7795743234149685026, 5741717600836367126, -3079592048807716085, 1020747861380457346, -5127534743687681585, -8305533273201584558, -1802988733400543042, -4961042680334336379, 4553305055239326222, -7454895788149166845, -3720444218231653480, -8907787256984411216, 5108126244500288043, -9026896777277892474, 7973128660032799805, -6144858764013113106, -2428866267615806992, 2309601762554918503, 7522586342988631529, -7351729781153140353, -4100930000301789413, -3539478232422295038, -7523047613797666715, 3869002175572306839, -2285941924814697148, 3313797115111162065, -8354018535343275123, 1111315221560903364, 2781877351769175442, -7840567229365755247, -3779961342939409154, -2278945887024260892, 875716875457227527, 1739539291753879895, 5180752179513650694, -3200745865231170399, -4605810213029289891, 7545298145507977079, -7986350430307537249, -6634344956179620478, -7299256524857262205, 676272968328860936, 7529897706757362881, 7085428723690567891, -5632397266756597554, -3291837931263082929, -3864067675993049970, 2897820910124036643, -3588614441075834417, 8455081112873395919, 965027993653249307, -2514450385702975510, -7116111884776903465, 1936041143868884130, -6188969857113738900, 5158960832470098783, 7404970013339733477, 2601441854091422838, -4515983522972520664, 157929284580958990, -4413646634038950015, 9116159596270253980, -2412526400277896971, -4376142719254407195, -1439596050347711698, -1644326379030835642, -2560006920923509320, -6105920874360091429, -102205177584099410, 8302707448023658797, 4420570700582920277, 8847339353952538633, 2202119325146159196, -2977213528936347645, 1662625080410990670, -874627419038230741, -3701338169191849563, -6511925173319353004, 6785831768028695562, 4183777648509484844, 8365483330123989075, -4767446708763661652, -4727149954379613475]}
RedisInstance{atomicInteger=110465, redisId=7093059810473952363, slodIdArr=[-545087104560140696, -2667980287911542937, -6757426980946892312, -9025045996874397162, -8136930311486974761, -1029341998347788326, -1585400078466133861, -1797365562019147804, -5032149813413283054, -373398119009360133, -8837091318040393853, 7030729087580881614, -1282596571010746028, 6975591788473445554, 3291831776265211068, 1687463844659813391, 8123625573549706496, 4450227638719830259, -7582860614883615030, 3247565135041791683, 1127525093548050541, 751704149811502635, 2463744054437714405, 6424419439949698117, -6974816429836059589, -6729342071932533765, -6852817748519318372, 286919627639844038, 7164189317705909856, -3479575323850291, -5666327276168068157, 8430700772275365665, 7482190812754032655, -8781990426618243358, 1952503026389258352, -5996425527302278696, -6530958025054874322, 477950106049708292, 5490421304685395612, -1441190814086510697, 8090045565185501773, -6616886939014725998, -642296144702179371, -2865155879135210780, -2333805599949878781, -4397206080921460750, -5722957669810185508, -8838039225492605233, 710056187136350272, -7465222607772444675, 239065026614469527, 5554330295872097670, -4977032873911755293, 4239568530753426228, 6177054762388495715, 8072789565789923919, 3739831120252854899, -6916350232260421969, -7072061618029056298, -2710823137268282834, -5789989586677220585, 7269830022313384780, -1016936769539985408, 2690564839205812561, -8879316010869297654, 1531211043908930131, 5350694956198665710, 2963229297201679908, -7157720159353074855, -5400881634920621410, 7520854159961801785, -9059700163289347841, 7486439859862030340, 336447804243255916, 3004209772434548683, -970648331107263596, -8594481742262208967, -7364894420873987470, 7723818129840509661, -5105366932926098953, 4316090976297518775, -8527094795285997239, 154883534865909613, 3159763156687330955, -4491490798717714689, -4858189967287978677, 2175041262837397234, 6309144828101961580, -6193997846516587494, 6586027355287492686, -9054813101947165522, 2527736505701792765, 2597479467746591325, -3305246803307207668, -1144762991306180411, -474072264677430068, 6470116412715581375, -4401960125450985371, -3491806458200483452, -4940326672455365226]}
RedisInstance{atomicInteger=94416, redisId=4648982741203627255, slodIdArr=[-8940276399203233553, -5185706689461137211, -1270130166939417440, 8068992569184477529, -3086371389522756848, 6963769306689144146, 2283422093532324127, 8101747197302137264, -5621611913080298082, 2794229654241976716, -582687100841292312, -1962838186736312934, -8489318898941068187, -8407970826605632446, 814210643640975282, -1480951426353875144, 4098180485968429406, 3241176131239125514, 5197237592157402625, 4553890725022309819, 922152710296560922, -2688065945785410559, 4329624583125409576, -7729082064503392280, -309145680485485095, 5948418497916581833, -6790995475796995591, -5126047235346983525, 4623995883255983466, 8835915699792458129, 7663468708732704481, -2969230184747633763, 1055451575226556198, -3311372783144794681, 5698563582520625260, 6141938923761747744, 7009442550670098214, 5275047218888732030, -4541649721372921361, 7526857259180430701, 8028480852885804090, 935326935042638408, -8169569999123211376, 552713467493611204, -5939721216757548788, -403883651490019396, 2179761681628692857, -4166437210411949450, -8526578159848932206, -6412095958644005006, -85716251038667264, -6838688279570074066, 1579683432407104053, -6136478379169503419, -5697549362996045524, 5853849675489825385, 8658252853716374750, -5044015007380063755, -7385784260505919593, -4668865359337442358, 6271732406084293543, -2004062327102444342, 6101943141947899199, 8393347269455030046, 4388420794247830736, 3739079471377203172, -3120204508755728656, -3571049932056755788, -2449148617969205102, -1175590943846023149, 5315001995901064506, -1476943396220246143, 3420640641428003217, -8660196388519244960, 2552679362034566061, 2784143474210013898, -8605737537786451367, 532818659192156037, -1249457042935972489, 7844632883964046628, -1284978092286061951, -919193468140364901, -3477353000701355048, 608253200892551164, 8286194924703256471, -7609153628084097382, -7682405975077481281, -3388919864556328208, -1408832177187892430, 5912127109735271740, -6313097704320516130, 2741784001100816397, -2710549433792494767, 1567561970329030474, 6461358845892622926, 8895856070101604126, 7184064769726095547, -7609537723454898098, 4902982163380556810, 81438599983290752]}
RedisInstance{atomicInteger=89657, redisId=-9037070370899525184, slodIdArr=[-1266897777354457466, -1296750290725553946, -2080997979504788210, 6056854533263598207, 2249557297713658894, 1960019213303088616, -5620877536390043369, 839116450779188011, 3081666159866564704, -5092075775219326661, 7340017595923770288, 3045229866061082474, 3149385133445811832, -8135274599731674953, -2129524127863787596, 5045207233026492034, -3446083101027767987, -763281145844878194, -6016421676171631102, -8858788713043674302, -6222263950716276146, -4413072968510891947, -8108343528419658310, 2688120106380326207, 703009693075806079, -3200808024691791450, -1645223310451210789, -1597137913228273608, 2874325453199791524, 4608671638344267941, -7684167139557639995, 9155435464161648455, 4749168309495918093, 8159390506408803245, 5251770087113305276, -9184107231281931913, 2076583079398783931, -7583202660076959095, -7116807732366349504, 2687925892900741891, 8378392062069324040, -6314129978702725834, 6100725406586977485, 5063850222019197157, 2315068770633801379, -3580026147436545901, -615902196557159647, 8872533808040100906, -2926912660600786329, 8269999151174424226, 3813997047824113495, -1310501511870871084, -5482558947096315874, 1921567953267569098, 7728703453392848127, 7069270508369706621, 8984887536764792583, 8408363968296565537, -1546643408207919111, 3138997614048066843, 5251456483358627330, 1059060479046800704, 8719700854328370703, -4721973080061861014, 4105389202060114016, 5994121888822323450, 3021447298194569105, -8031042712889046660, -4067296010888995107, 510467132331610167, -4355969994185820191, 2710388471500958398, 4573029917274003411, 2161355150911075586, -5694345523662905739, -5724515316495842736, -3327927661073361544, 5431551150085837970, 5955841602715554573, -8733140631022608593, -2059027545232274922, 5997927483120596760, 6879313535995058852, -1298079297042286042, 4884937559422083388, -6913187571533913330, 799264530313743151, 5151894049714866654, -5924771471537613787, -7932697576502699667, 8366966610703572196, 6507803978567075737, -653654419692018588, -4519570153133752854, -2930000690505796266, 1122148892609584343, -6463925135117035486, -749756511354011608, 6505093974939542471, -3942196857073634832]}
RedisInstance{atomicInteger=100835, redisId=7567112797788265274, slodIdArr=[-1332039581559486530, -3761685390531882328, 7351496920805951288, 4507861534050126247, 251943732602081684, 5227093993666551159, 2639867447869619728, -621857900388064384, 3451141588938842601, -548519719832512320, 7688637819326925770, -4080051847906639489, -5539302251708008220, -2464274700527385955, -6062011535711092911, -530876164800959983, 4257081185604810945, -7093514004241484148, 6529518976581752474, -393277672137577370, -3051696032250105941, 4113082205399015559, -3526123055983787818, 8021707928060149689, -5929441709510947332, -6736752611442141661, -8254442844764702627, 1656640613839565045, 5641979299075437400, -7755404271123383537, 1802254086011292103, 1661209765010355116, -5841889883548252681, -3265183887876582709, 4841170050787105450, -6731539493363984636, 2358381812569864327, -3644842626093563070, 6357935845519758876, -7417692312529281004, -1285021200399343112, -4980073731655948553, -7854538287069875237, -1718877911300926069, 8964875777109180404, 2848996557573343868, 900476373976348589, 350931215171189283, 3115869832928110852, -7163815414196429035, -7405064533898667168, -7843119478771389998, 8328771920888325051, 2327874284270216921, 5439040086940775407, -7229679873880877383, 6079543229074867431, -7489440585398589604, -4346422968740848563, 9066209065773628584, 6122064985076636228, -9097677340378235621, -9195222313787911167, 1106617140680172061, 6225826663553276756, 3592831842881932574, -8910463964676510911, 3982729230197211243, 5026547380368064676, -7864402444810968361, 5040065010809545998, -4426371261544101360, 7164040474452565, -7242253644386624292, 4539432220906880051, 8678504220288962669, 6682832540609269376, -3335585286378987771, -3783948240501276474, 7310738818021732757, -3239324465659581796, 4293224916934589948, 2800620687985167027, -5365325683553982977, 251840043085456322, 6275521485787809585, -5962674245359576732, -3622277183388124014, -6794133919768007901, -3546663074520589627, 50782056391420378, 7173325921018014456, -7194638849978287859, 8154508642008208671, -4815965273964414613, -8857570901265420559, -555005171896455574, 6669307966334185283, 8003333226846708755, 8682271993945873752]}
RedisInstance{atomicInteger=109168, redisId=-7458590357896622627, slodIdArr=[83647120736552830, 1798773524039946642, -2611786940263602404, -1388617887425555442, 8784076572354897412, 8245804719191790085, 2882666906750058883, 1209733541616244699, 3218047848758617400, 691918229718841478, 5696003752733928665, -4615689936531220792, 4614486944978557785, -3103913070360504703, 3980229742576199849, 8672852724052419598, 6168896266612960240, -5371316118513008670, 5906682484248663096, -8705473265019804000, 7492362017996821961, 1710706657518174888, -5943973856341522849, 5155531448907498023, 8977772740925495191, 1554875431000198153, -5323388554312585535, -3340452275230995262, -605767327930160840, 7532196297443025969, 4754202583572281566, -2423673556328256255, -5207502523439229301, -6491643304432406661, 2499713864866173621, 17282567817474516, -895785849877786043, -1631192902118943231, 4223614786639105734, 7451984956945733157, 7064592322445667453, -6572907079872314181, -8084757913932966028, 5598021512136082074, 9179473216753732382, -450541451538538311, 8367071176583235060, -6213316031853138179, 7629251133299112538, 8725690666890215849, 5462350307416914102, 7789121022277691348, -4414140147910519395, -810474422225936398, 6756277193242893070, 6593714855998923294, -3429053777673496031, 5905575977467247988, 7508192056146290529, 641262447966481947, 2997155426004891476, -6908909668057644932, -1710707621931534542, -1449675765256209153, -5041950043703690092, -1841329154688005130, -9034209492781989327, 7744946778240412881, 8015574902434923135, -8266938365963394015, 7466864200224489777, -4764985190990922685, -289890974559219842, 1813577286806089420, 5521566727296164140, -3246752563668961064, -8384883702928110142, -5586090883365011562, -5534067373490288752, -4744455352858220360, -6259591387681048074, -6938312215467092886, -1298916799915630379, -336289372363391677, -3507083328560374045, -673364524932659594, 2564998916730989813, -4127418662035773165, 3586234703498936024, -4337646932259114476, 6918375011525382436, -4849131014306005876, -6417642618177897782, -3514931217738767488, 9204450060676001340, 4998571802903165294, 4696651727973892046, -2337128322103543574, -6542051485001091763, 1957760547239292764]}
RedisInstance{atomicInteger=108897, redisId=-495585558841713198, slodIdArr=[6393427076680928740, 5801832710731985053, 3015783254753133845, -1145835843463893281, -1236085216819807111, -3481005025040718226, 2132057524995442815, 4078378526759799579, 7177248209739522073, 7913631831873550005, 9212285153637701645, -3187027210379669608, 437394502950034031, 8575890381992407213, 8839494998897170027, 8292671564097051544, -2211304397653623505, -7317187593556436000, 1209423031153752623, -4434273563840806101, 1404069423552743929, -303074926422233664, -6412204656448099157, 2533200465318081258, -2090021107216879330, 2159293650291152156, 730826278405701754, -9117409558571170488, -8600753969348288459, 8126323194461427530, -9050857704727507181, 8350442243979458723, 118183075058353852, 4776931039022206913, 4868259840063680638, -4024141223274323741, 5186633612572182083, 1122947725497453618, 4334807494289866669, -7127162274003745010, -1670981409274719105, -8368235471762767547, -1728537281270582486, -2203691094776313551, -7629878417107837387, -5447248548079326735, -222970550111185144, 1292491598327597936, -9220543154514637213, 6675561414175087755, 5984449980393802715, -8630137101737395069, -2273437401501493547, 8363863452101851767, 3911467540401582199, -8779803130276883888, -82991517268472904, 3959099297287232550, 2110904145898200969, -2903645609779294741, -4292826504848829685, -7203663962296414065, -4363205098169085044, -6022930679636287462, 8724153716086734875, 7088907463348104593, 8075166184648430017, 3637808164978404404, -1799139935240894957, 792267045334380153, -935137328110433914, 7200045424537387803, -7391529929436172608, 3304068733732962107, -1976285931081486639, 4381277817835540530, 2264516322625623082, -3533289503223581053, -9172326112026969972, 6945744816638730943, -2442387401344741618, -289872656500513088, -2157572717243838306, -8608258010018460979, 2070301560698834340, 4130890077276956698, 3793478473464380573, -2989032538878087889, -4411422951018319880, -6101143785516180995, -5273438051399315309, 6686346165100058118, -1220414094044870532, -7353762198979276556, -2932144660510710367, -3797755585807288851, -6951486184179978665, 1175608043801762290, 6447295458503521297, -5234592014476273687]}
十个实例Redis,被引用的次数分别是:
108897
109168
100835
89657
94416
110465
95523
96484
98170
96385
redis实例的被使用次数总体是均衡的
评论