c++ 基础——杂谈 2
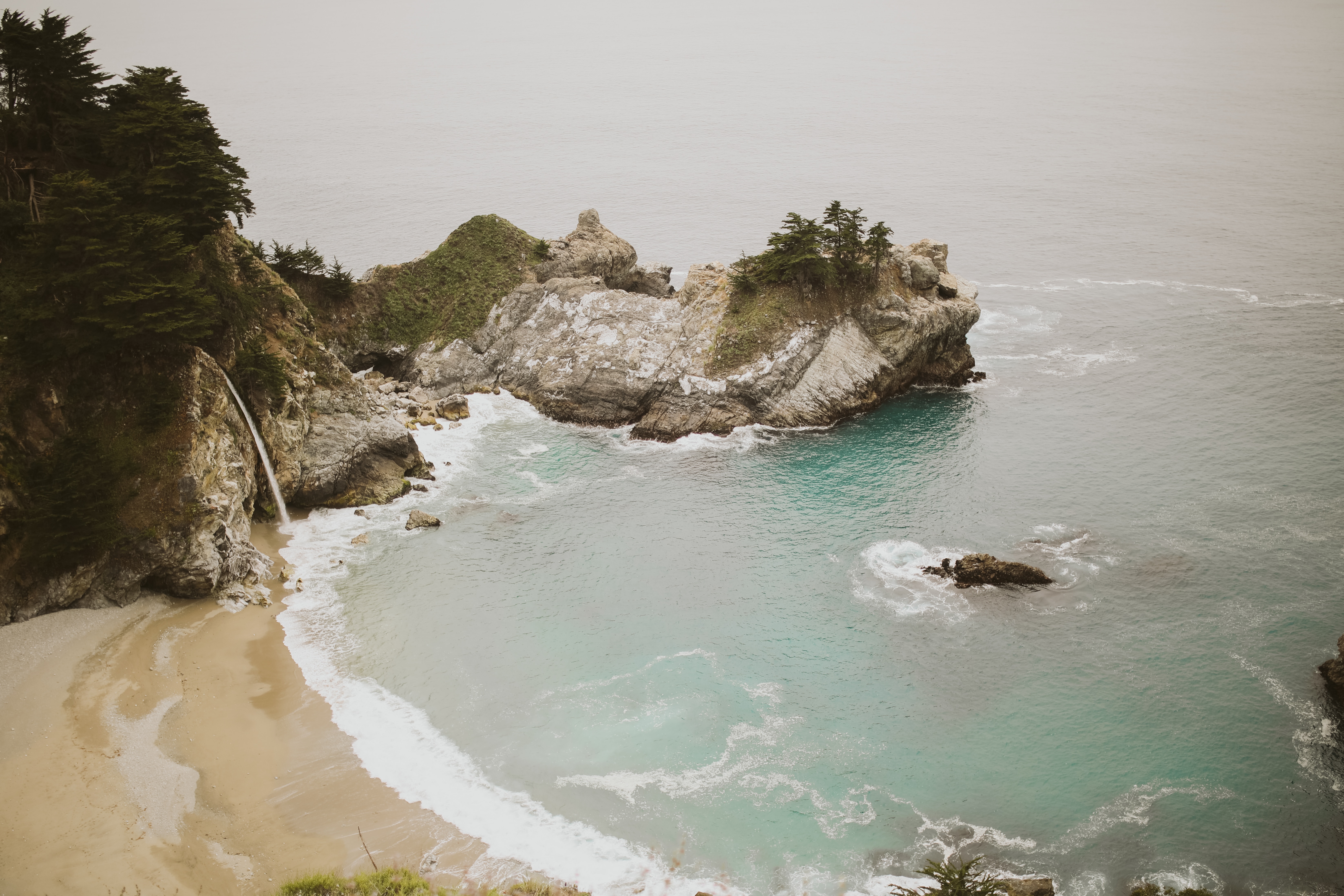
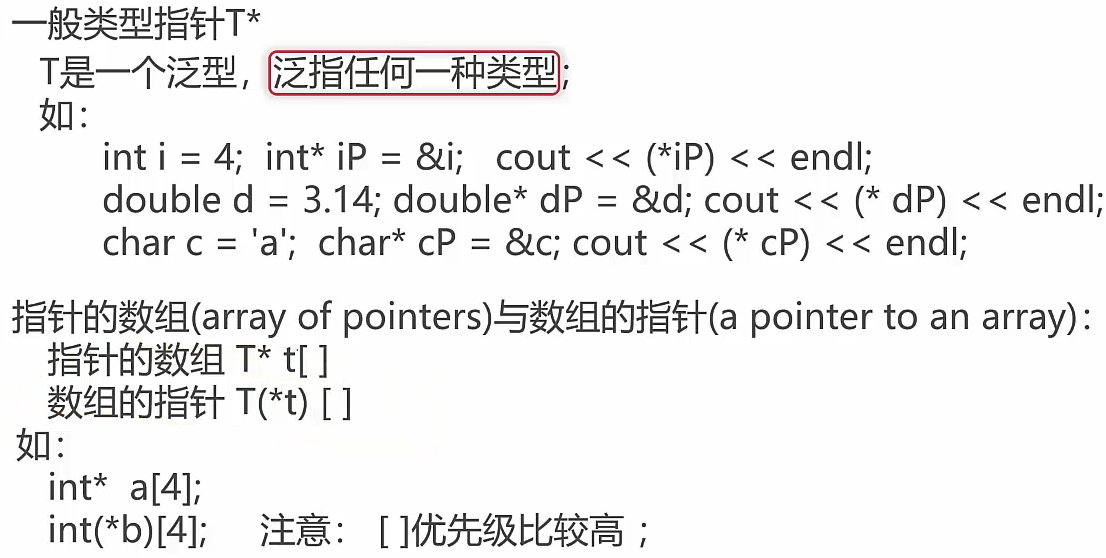
int* a[4] 指针的数组,4个都是存放int类型指针;
int(*b)[4]数组的指针,指向的是int类型包含有5个元素的数组;
const pointer与pointer to const
关于const是修饰内容不可变,具体原则:
看左侧最近的部分;
如果左侧没有,就看右侧。
通过上述三行代码进行分析:char const *pStr1 = "helloworld";这一行是修饰的是char,那就说明里面的内容不可变,但是存储的指针的指向可以改变;char* const pStr2 = "helloworld";修饰的char*类型的指针,所以指针不可变,但是里面的内容可变; char const * const pStr3 = "helloworld";修饰的是char的内容和char类型的指针,所以都不可以发生变化。
指向指针的指针
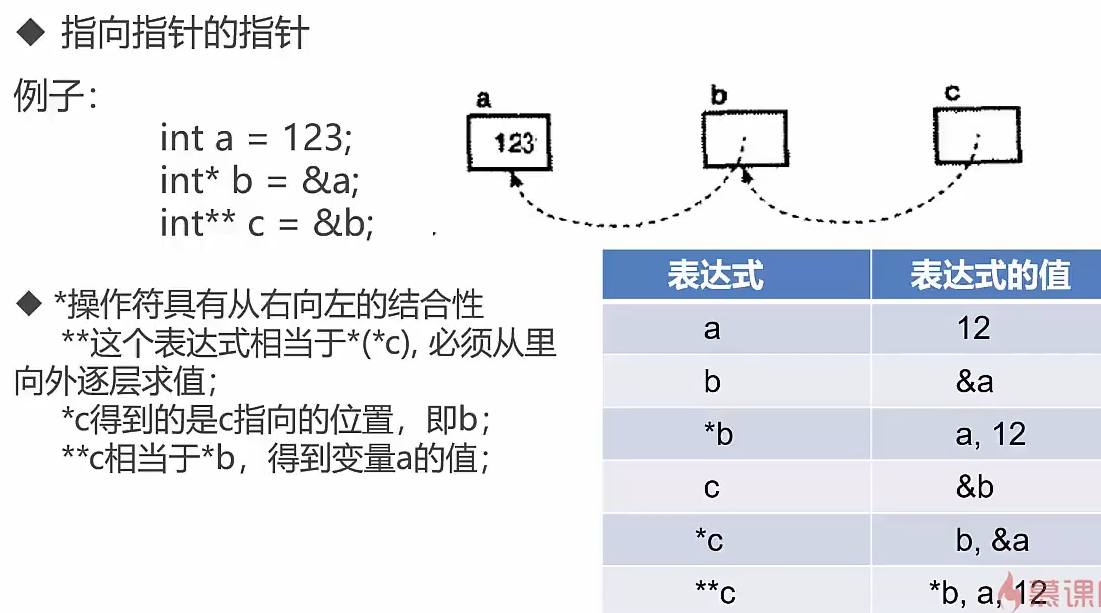
一个*代表一次间接访问值;
野指针
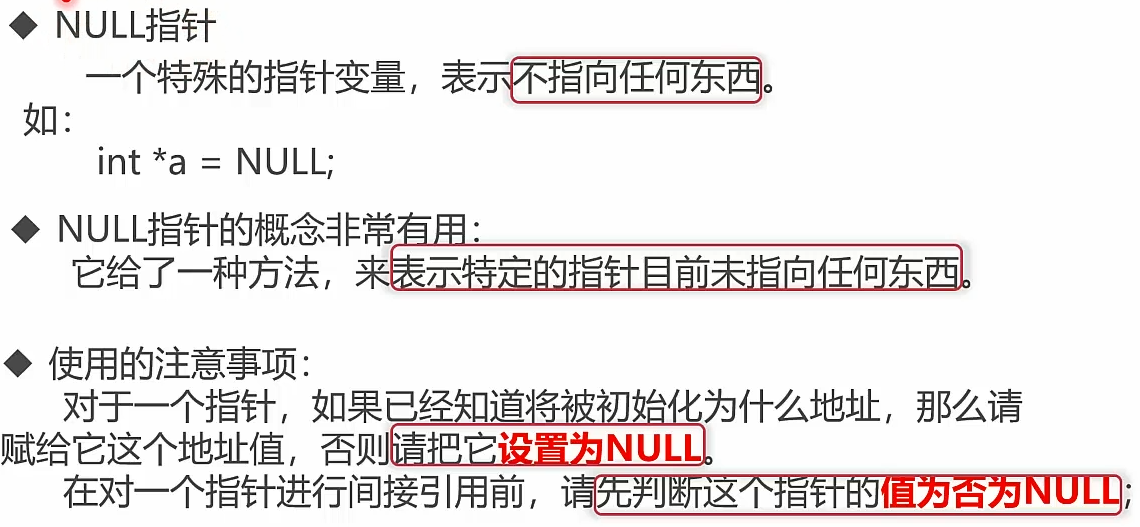
下面是对于空指针的判断
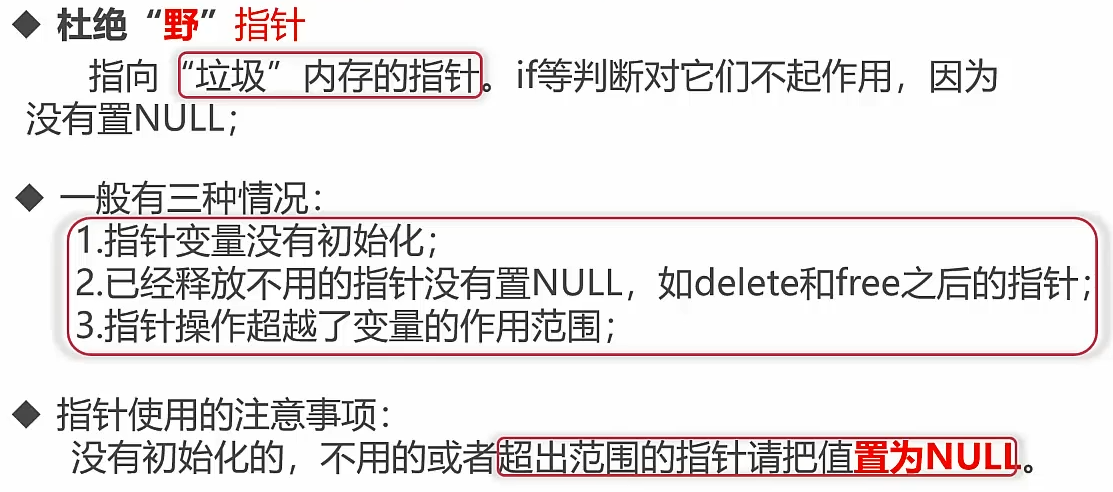
原始指针的基本运算
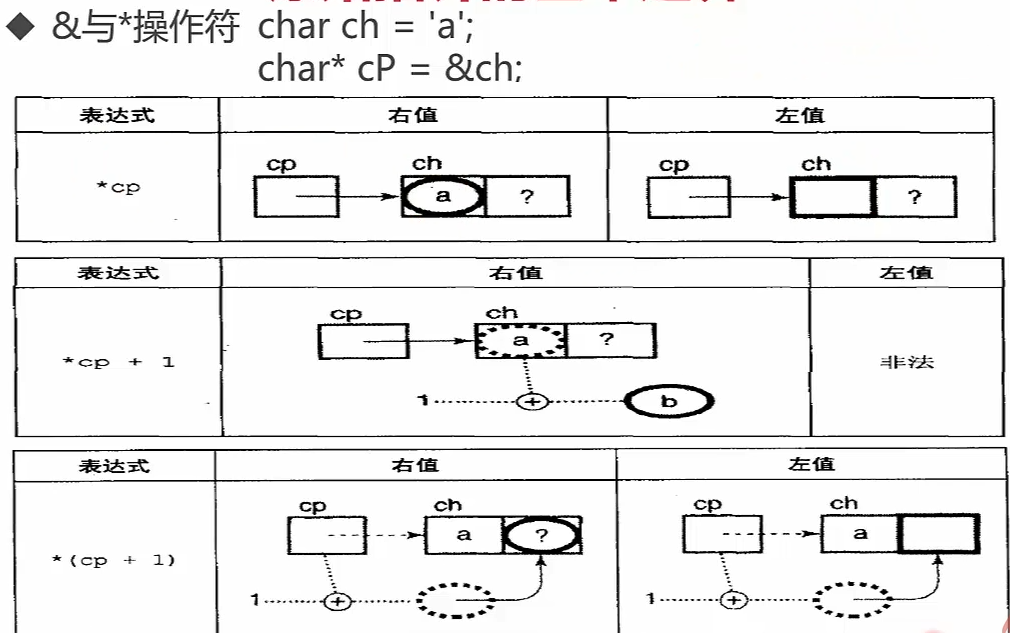
指针的左值和右值并不一样
char* cP = &ch;当char* cP = ‘b’;ch的值也会跟着改变。char ch2 = *ch;
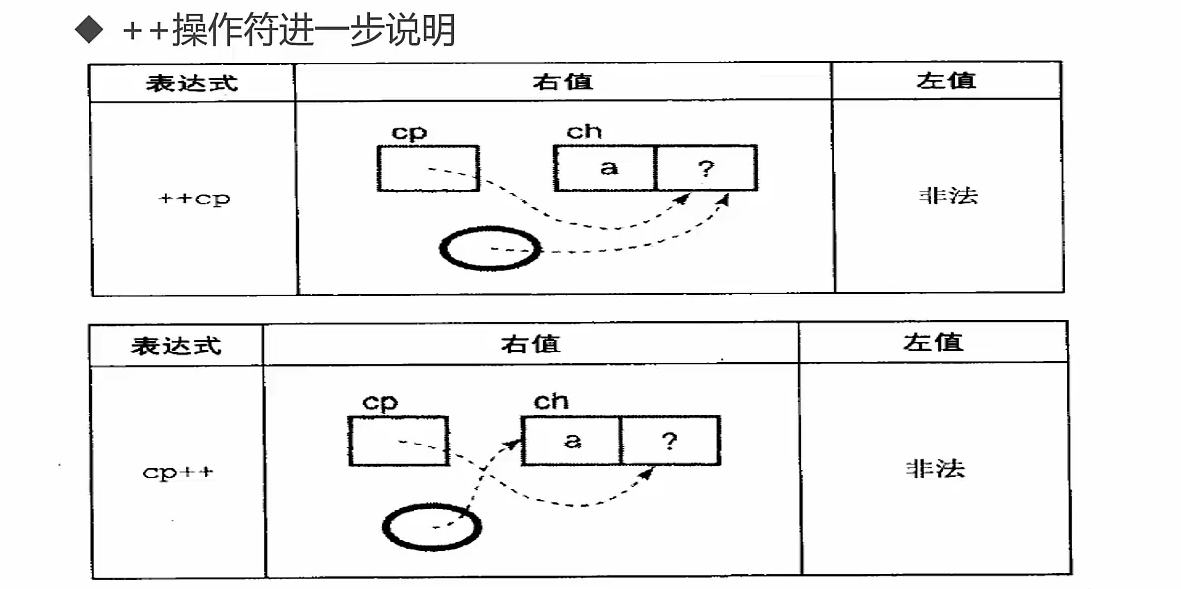
椭圆的圈是你最后得到的值。对于一个指针的++操作,只能说是指针得位移操作,并没有赋予具体的值的属性,因此不能作为左值。
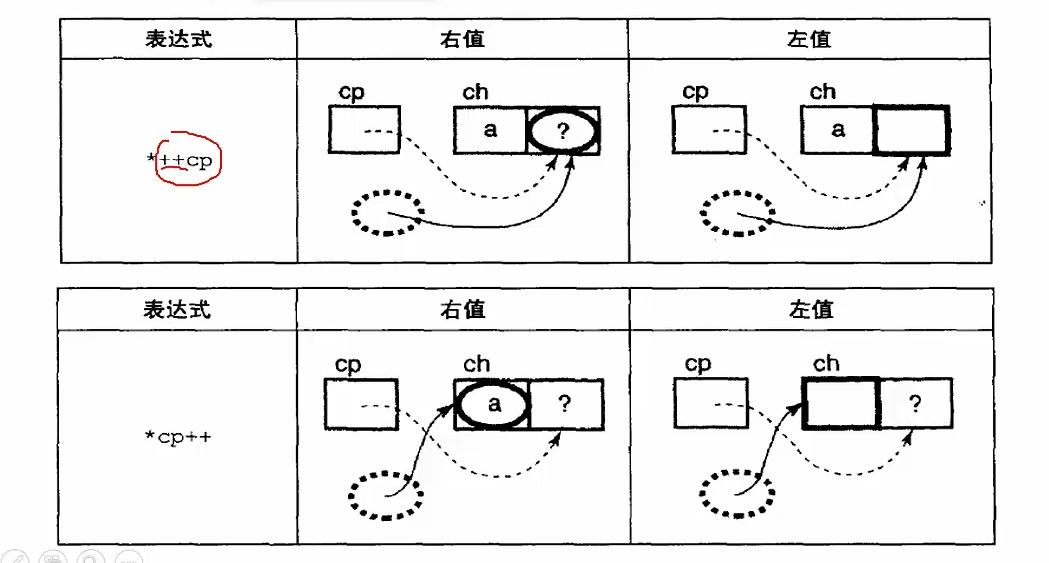
从优先级来说,++高于*(取值),这里左值是指,在cp原始空间的往后挪一位的空间,进行初始化操作,可以往里面赋值,填值。
存储区域划分
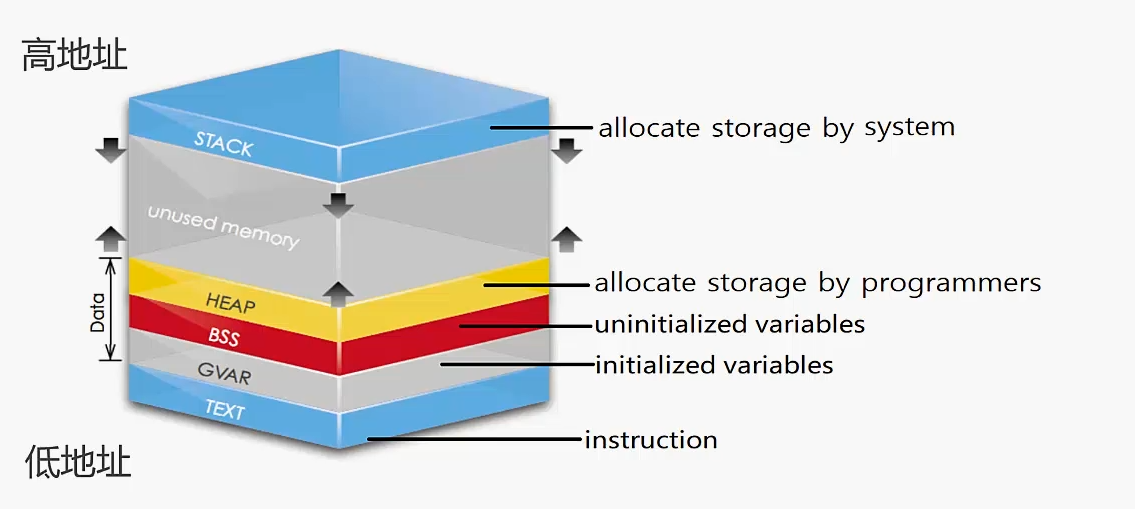
BSS是没有初始化的变量
GVAR是已经初始化的变量(全局或者是静态的变量)
下面谈一下,资源动态分配--堆(heap)
使用堆进行动态分配内存
1 分配内存块
2 释放之前的内存块
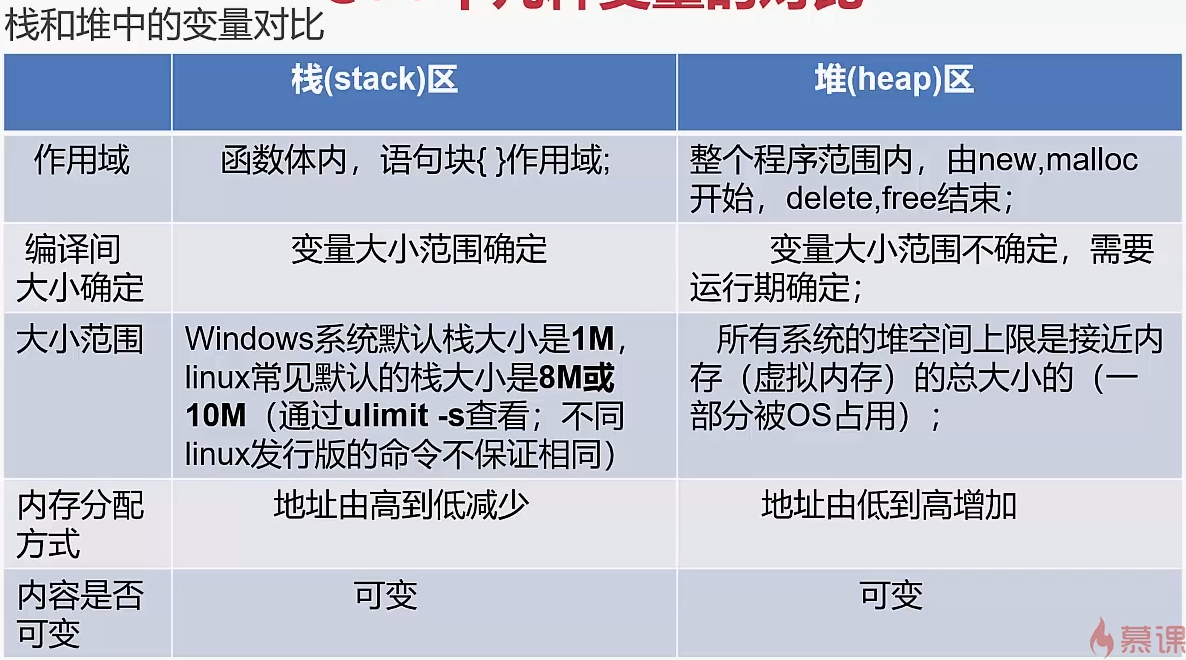
c++资源管理方案--RAII
由于释放的内存块会导致,内存碎片化。用RAII依托栈和析构函数,进行内存管理。
内存泄漏
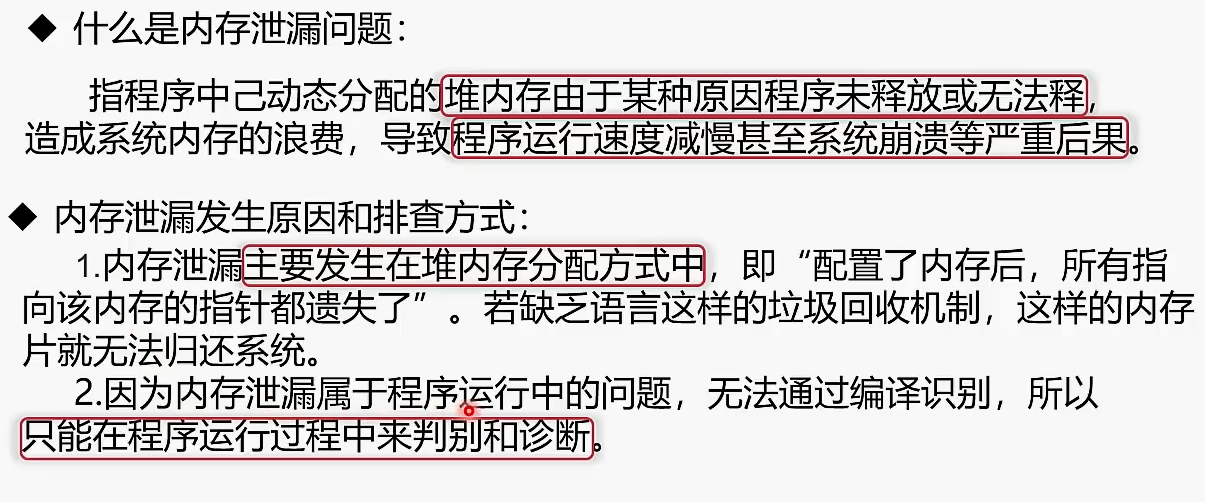
智能指针
unique_ptr , shared_ptr,weak_ptr,常用的几种智能指针
auto_ptr:
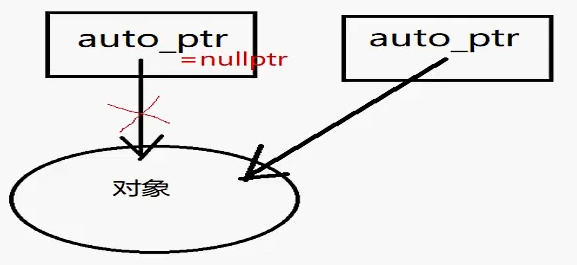
由new expression获得对象,在auto_ptr对象销毁,所管理的对象也会自动被销毁;
但是会发生所有权转移,不小心把它转移另一个智能指针,原来这个指针就不能拥有这个对象。在拷贝过程中直接剥夺指针对于原对象对内存的控制权。
转交给新对象后,将源对象置位nullptr
先把原来的指针置位nullptr,然后新的对象作为tmp保存,让新的指针去指向tmp。
unique_ptr:
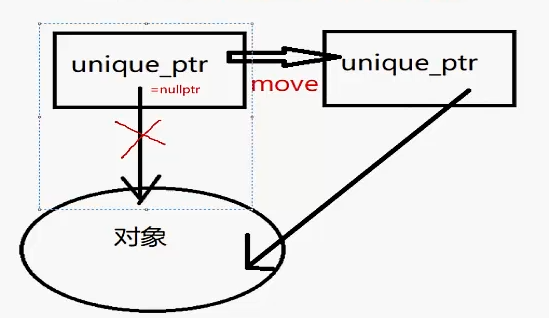
auto自动检测,推导类型
shared_ptr
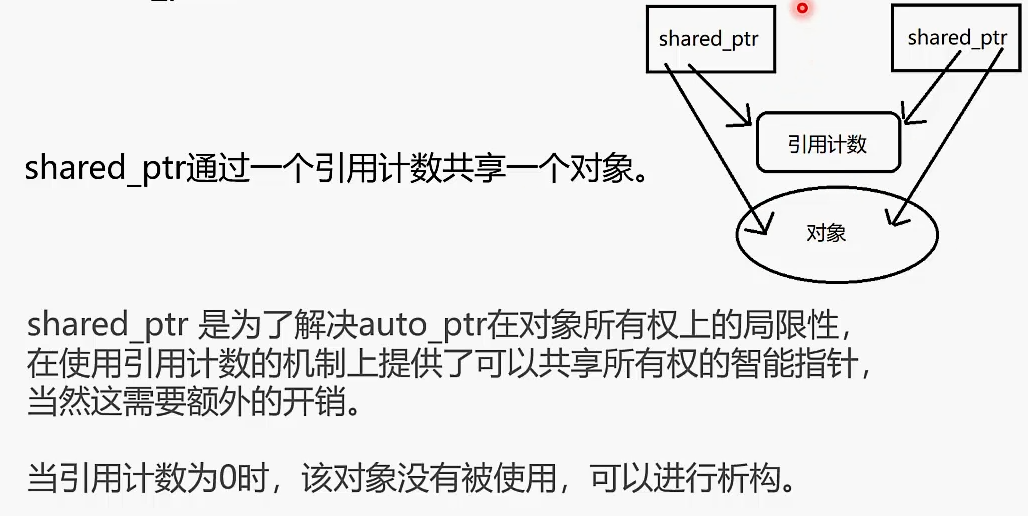
引用计数会带来循环引用的问题
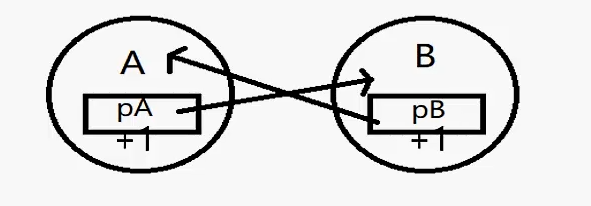
导致堆里面无法正常回收,内存泄漏的情况出现
weak_ptr
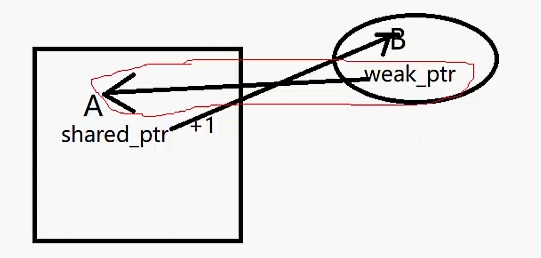
采用观察者的工作方式,不获取所有权
A可以对B 造成直接的影响
B不能对A产生直接影响

引用
不允许被修改的指针
int x = 1;
int& rx = x;
说明给x起了个小名,把x的地址给了rx
版权声明: 本文为 InfoQ 作者【菜鸟小sailor 🐕】的原创文章。
原文链接:【http://xie.infoq.cn/article/79fa44064ae2a5f46a7c63653】。未经作者许可,禁止转载。
评论