C/C++ 最佳实践
本文用于记录个人在使用C/C++的过程中,个人觉得是最佳实践的用法。另外,本文持续更新!!!
判断
map
、unordered_map
、set
、unordered_set
中某个key
是否存在,推荐使用count()
代替find()
,理由很简单,前者的代码量比后者的少。之所以可以用count()
代替find()
,是因为在上述容器中,key
是唯一的,因此如果key
不存在的话,count()
就返回0,存在的话,count()
就返回1:
private
继承用于“实现继承”:
计数器使用无符号整数,这样可以利用无符号整数回绕的特点:
让结构体1字节对齐:
当然,也可以使用如下方式:
但如果漏写了#pragma pack()
,即未取消1字节对齐,那么后面定义的结构体都是1字节对齐的了,所以推荐使用第一种方式。
可使用lambda表达式创建一个线程:
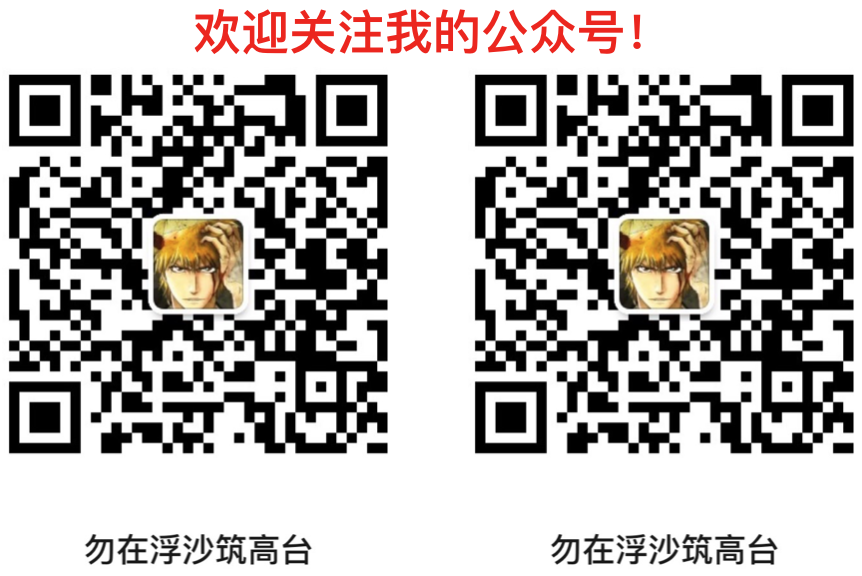
版权声明: 本文为 InfoQ 作者【jiangling500】的原创文章。
原文链接:【http://xie.infoq.cn/article/79692b1da89878395359575a0】。文章转载请联系作者。
评论