面向对象学习
习题3:
1. 请在草稿纸上手写一个单例模式的实现代码,拍照提交作业。
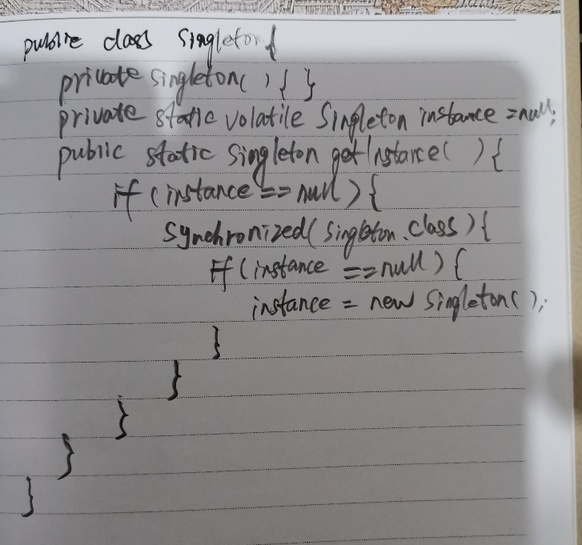
2. 请用组合设计模式编写程序,打印输出图 1 的窗口,窗口组件的树结构如图 2 所示,打印输出示例参考图 3。
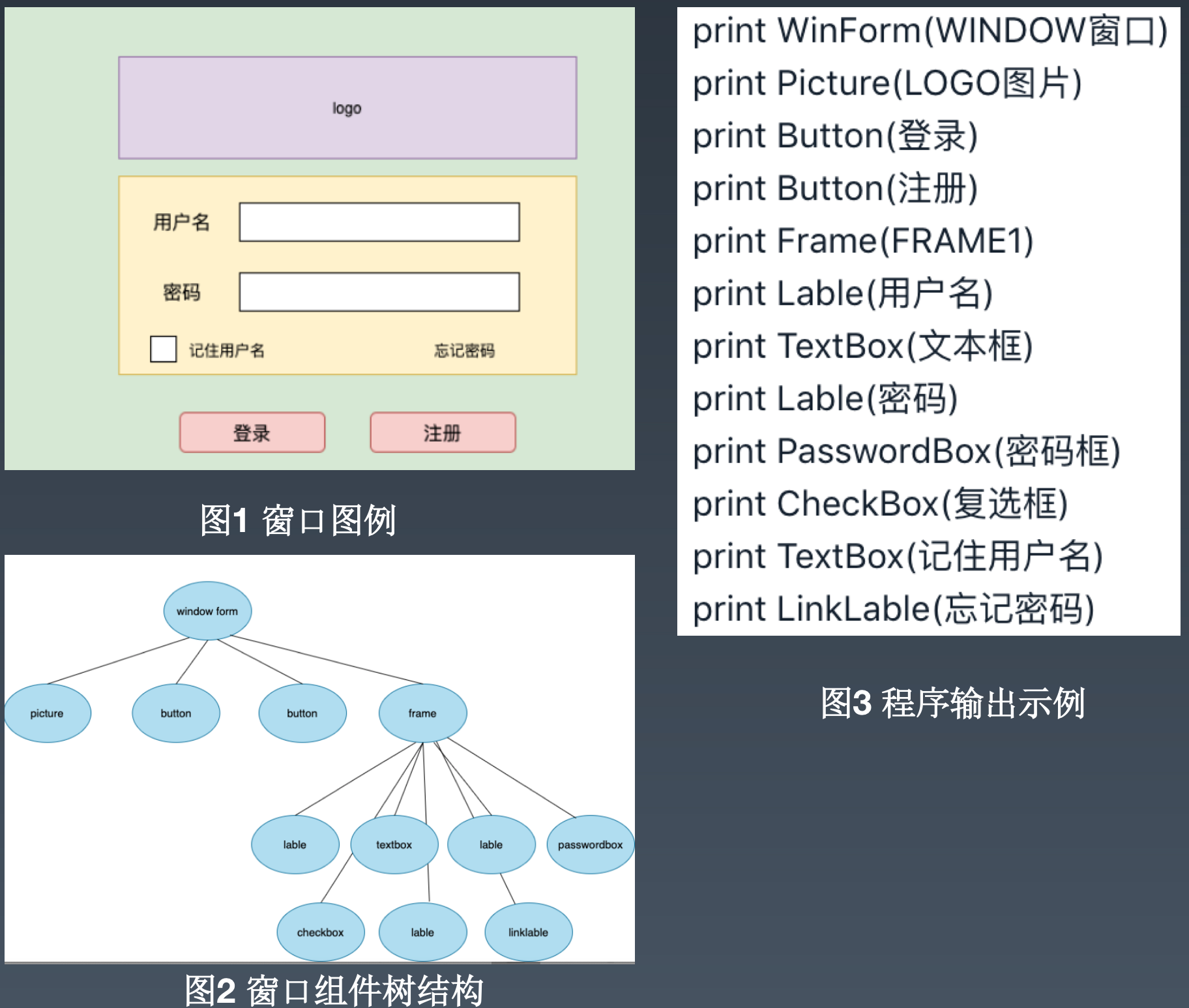
package com.winform.components;
public abstract class Component {
protected String name;
public Component(String name) {
this.name = name;
}
public abstract void print();
}
public abstract class Container extends Component {
private List<Component> childComponent;
public Container(String name) {
super(name);
this.childComponent = new ArrayList<>();
}
public void addComponent(Component component) {
this.childComponent.add(component);
}
@Override
public void print() {
for (Component component : this.childComponent) {
component.print();
}
}
}
public class ButtonComponent extends Component {
public ButtonComponent(String name) {
super(name);
}
@Override
public void print() {
System.out.println(String.format("print Button(%S)", this.name));
}
}
public class FrameComponent extends Container {
public FrameComponent(String name) {
super(name);
}
@Override
public void print() {
System.out.println(String.format("print Frame(%S)", this.name));
super.print();
}
}
public class WinForm extends Container {
public WinForm(String name) {
super(name);
}
@Override
public void print() {
System.out.println(String.format("print WinForm(%S)", this.name));
super.print();
}
}
评论