TensorFlow2 Fashion-MNIST 图像分类(一)
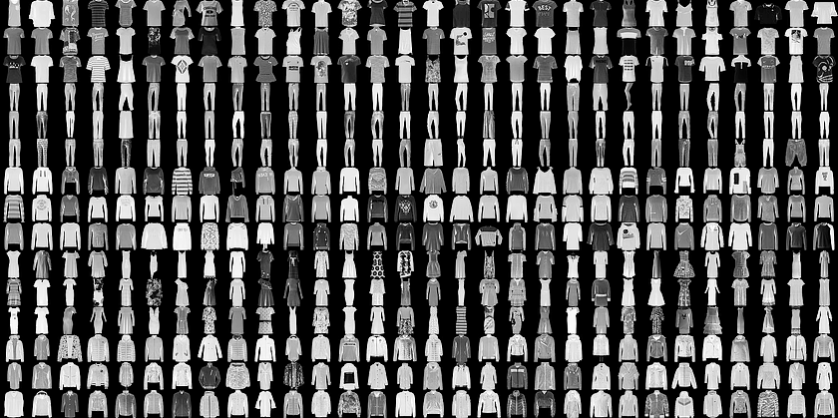
1.数据集介绍
FashionMNIST 是一个替代 MNIST 手写数字集的图像数据集。 它是由 Zalando(一家德国的时尚科技公司)旗下的研究部门提供。其涵盖了来自 10 种类别的共 7 万个不同商品的正面图片。
FashionMNIST 的大小、格式和训练集/测试集划分与原始的 MNIST 完全一致。60000/10000 的训练测试数据划分,28x28的灰度图片。你可以直接用它来测试你的机器学习和深度学习算法性能,且不需要改动任何的代码。
官方的关于数据集的介绍可以参考:
https://github.com/zalandoresearch/fashion-mnist
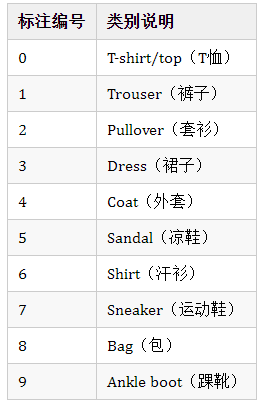
2.模型训练
Tensorflow版本:2.2.0
2.1 数据加载
数据的加载使用可以直接调用tensorflow包进行联网加载,也可以将数据下载到本地,进行本地数据读取。
代码如下:
本地数据下载之后,包含四个文件:
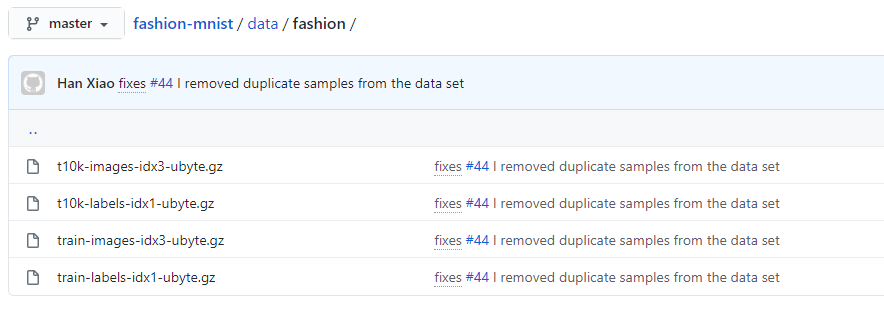
然后,将原来的加载函数load_data稍作修改,即可加载本地文件,只需要将远程下载地址,更改为你的本地文件所在目录即可,此处我的文件目录为data。
2.2 数据划分
提供的数据包含60000/10000 的训练/测试数据。我们再将训练集进行划分,train和valid,原有的测试数据用于模型最终的测试,训练集前5000数据作为valid,剩余的数据作为train集合。
数据集合划分情况如下:
(5000, 28, 28) (5000,)
(55000, 28, 28) (55000,)
(10000, 28, 28) (10000,)
对其中的一个样本进行可视化查看
绘图结果如下:
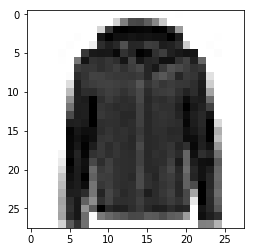
我们可以多查看一些数据样本,编写函数进行自定义查看。
次数我们查看15个样本图片,结果如下:
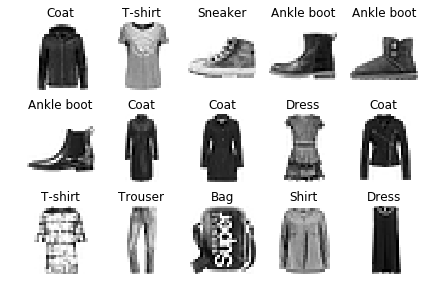
2.3 模型构建
我们使用tensorflow2中集成的kera包进行Sequential模型构建:keras.models.Sequential()
Sequential提供了非常简便的方式进行神经网络模型的构建,就是将要添加的模型一层一层的逐层进行add即可,也可以通过列表的方式,一起进行添加。
经过上面的步骤,模型已经构建完成,编译生成模型结构,接下来可以查看模型的相关信息。
关于各层模型的向量的维度计算,解释如下:
输入的数据就是[None,2828],然后经过Flatten之后,就是[None, 784],接着就是添加全连接层,维度是300,那么这个时候XW + b = y,可以算出W的维度,也就是权重的维度应该是[784, 300],偏置的维度也就是[300]。对于后边全连接层的各参数的维度计算同样的道理可以得出。
接下来就是模型训练,使用的是fit函数。
这里指定了epochs的个数,就是一共要训练多少次,训练一次就是将xtrain数据跑一遍模型。而每一次往模型中输入多少个样本,就是batchsize,此处指定了64,batch_size越大,占用的内存越大,所以要参照自己的机器配置适当设置。
模型训练过程如下:
可以看出由于设置的batch_size为64,所以训练一次要进行860个批次的样本输入。
针对history,可以查看他的相关类型和相关信息:
最后对模型返回结果中的相关参数画图显示变化情况。
结果如下图:
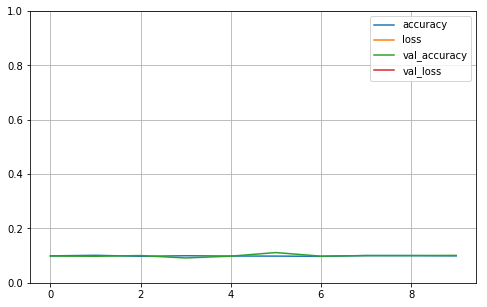
从最终的结果可以看出,准确率很低,而且从整个训练过程也可以看出,每次训练完之后,基本上没有太大的变化。
我们对数据集中的数据的大小进行统计查看:
图像灰度值从0到255,数据存在较大的分布跨度。输入数据的维度是28*28,其展开之后就是784。在机器学习领域中,不同评价指标(即特征向量中的不同特征就是所述的不同评价指标)往往具有不同的量纲和量纲单位,这样的情况会影响到数据分析的结果,为了消除指标之间的量纲影响,需要进行数据标准化处理,以解决数据指标之间的可比性。原始数据经过数据标准化处理后,各指标处于同一数量级,适合进行综合对比评价。其中,最典型的就是数据的归一化处理。简而言之,归一化的目的就是使得预处理的数据被限定在一定的范围内(比如[0,1]或者[-1,1]),从而消除奇异样本数据导致的不良影响。
归一化的目的,有三个方面可以参考:
避免具有不同物理意义和量纲的输入变量不能平等使用;
bp中常采用sigmoid函数作为转移函数,归一化能够防止净输入绝对值过大引起的神经元输出饱和现象;
保证输出数据中数值小的不被吞食.
所以,从上面的训练过程和结果可以看出,我们的模型并没有很好地拟合,所以,需要解决输入数据的归一化问题,下个部分进行归一化的模型构建。
版权声明: 本文为 InfoQ 作者【书豪】的原创文章。
原文链接:【http://xie.infoq.cn/article/77e426cd5ae0563105005deaf】。文章转载请联系作者。
评论