第三周作业
发布于: 2020 年 10 月 04 日
作业1
请在草稿纸上手写一个单例模式的实现代码
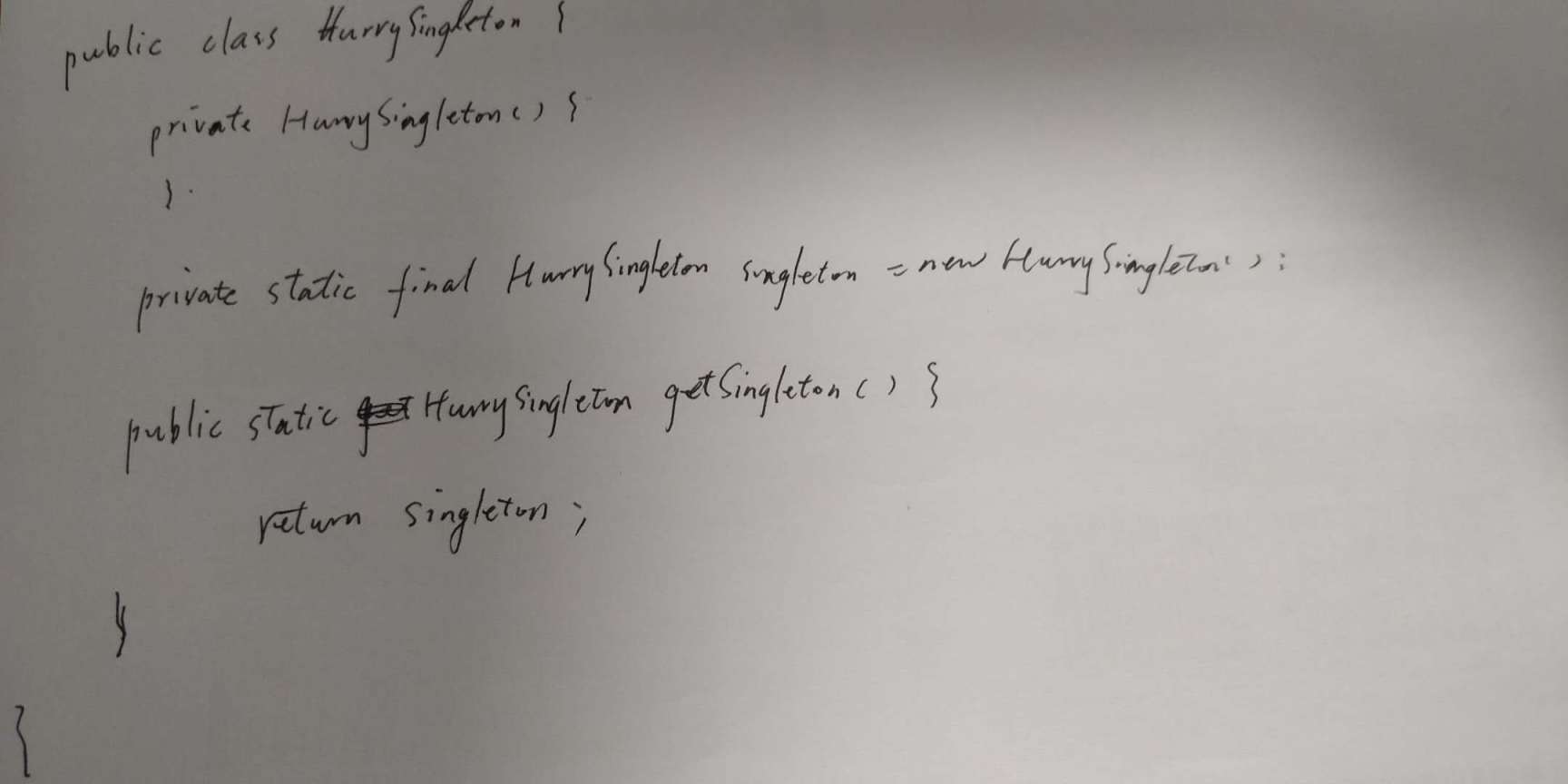
问什么要试用 Singleton
Singleton 模式保证产生单一实例,就是说一个类只产生一个实例。试用 Singleton 有两个原因
是因为只有一个实例,可以减少实例频繁创建和销毁带来的资源消耗。
是当多个用户使用这个实例的时候,便于进行统一控制(比如打印机对象)。
作业2
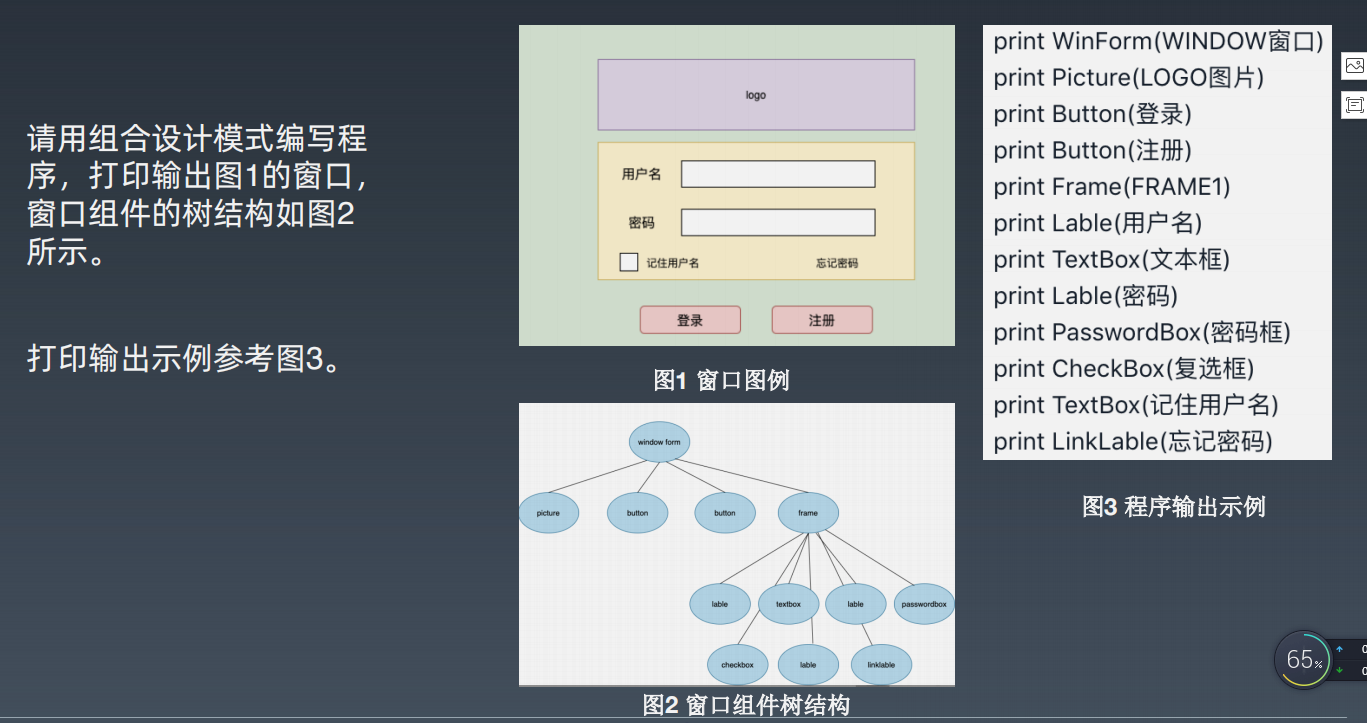
public class Component { private String name; public Component(String name) { this.name = name; } public String getName() { return this.name; } public void add(Component component) { } public void print() { String className = this.getClass().getSimpleName(); System.out.println("print " + className + "(" + this.getName() + ")"); }}public class Frame extends Component { private List<Component> items = new ArrayList<>(); public Frame(String name) { super(name); } @Override public void print(){ super.print(); for (Component component : items){ component.print(); } } public void add(Component component){ items.add(component); }}public class Button extends Component { public Button(String name){ super(name); }}public class CheckBox extends Component { public CheckBox(String name) { super(name); }}public class Lable extends Component { public Lable(String name) { super(name); }}public class LinkLable extends Component { public LinkLable(String name) { super(name); }}public class PasswordBox extends Component { public PasswordBox(String name) { super(name); }}public class Picture extends Component { public Picture(String name){ super(name); }}public class TextBox extends Component { public TextBox(String name) { super(name); }}public class WinForm extends Component { private List<Component> items = new ArrayList<>(); public WinForm(String name) { super(name); } public void add(Component component){ items.add(component); } @Override public void print(){ super.print(); for (Component item : items){ item.print(); } }}public class Compostion { public static void main(String[] args){ Component picture = new Picture("LOGO图片"); Component loginBtn = new Button("登录"); Component registerBtn = new Button("注册"); Component winForm = new WinForm("WINDOW 窗口"); winForm.add(picture); winForm.add(loginBtn); winForm.add(registerBtn); Component frame = new Frame("FRAME1"); Component userNameLable = new Lable("用户名"); frame.add(userNameLable); Component textBox = new TextBox("文本框"); frame.add(textBox); Component passwordLable = new Lable("密码"); frame.add(passwordLable); Component passwordBox = new PasswordBox("密码框"); frame.add(passwordBox); Component checkBox = new CheckBox("复选框"); frame.add(checkBox); Component rememberUserTextBox = new TextBox("记住用户名"); frame.add(rememberUserTextBox); Component forgetPwdLinkLable = new LinkLable("忘记密码"); frame.add(forgetPwdLinkLable); winForm.add(frame); winForm.print(); }}
输出结果:
print WinForm(WINDOW 窗口)print Picture(LOGO图片)print Button(登录)print Button(注册)print Frame(FRAME1)print Lable(用户名)print TextBox(文本框)print Lable(密码)print PasswordBox(密码框)print CheckBox(复选框)print TextBox(记住用户名)print LinkLable(忘记密码)
划线
评论
复制
发布于: 2020 年 10 月 04 日 阅读数: 19
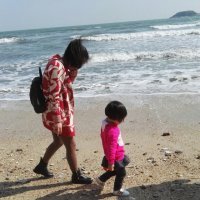
追风
关注
还未添加个人签名 2018.01.08 加入
还未添加个人简介
评论