链表转换为二叉排序树、反应式编程 RxSwift 和 RxCocoa 、区块链 hyperledger 环境搭建、环境架构、John 易筋 ARTS 打卡 Week 20
1. Algorithm: 每周至少做一个 LeetCode 的算法题
笔者的文章:
算法:把排好序的链表转换为二叉排序树Convert Sorted List to Binary Search Tree
题目
109. Convert Sorted List to Binary Search Tree
Given the head of a singly linked list where elements are sorted in ascending order, convert it to a height balanced BST.
For this problem, a height-balanced binary tree is defined as a binary tree in which the depth of the two subtrees of every node never differ by more than 1.
Example 1:
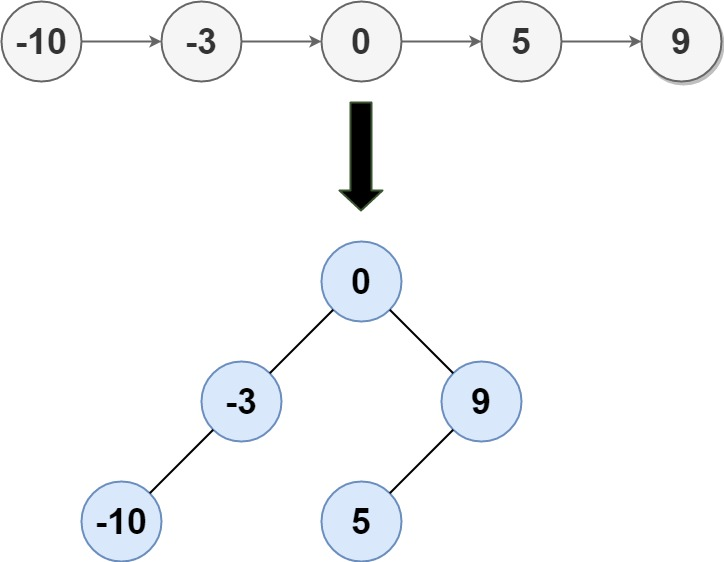
Explanation: One possible answer is [0,-3,9,-10,null,5], which represents the shown height balanced BST.
Example 2:
Example 3:
Example 4:
Constraints:
The number of nodes in head is in the range
[0, 2 * 10^4]
.-10^5 <= Node.val <= 10^5
解法一:转为数组后,根据数组序号二分组装成二叉排序树
思路:
因为树有左右分叉,用递归解法。
把链表的值,组装成数组;
根据数组的索引,递归调用组装成二叉排序树
参考
https://leetcode.com/problems/convert-sorted-list-to-binary-search-tree/solution/
2. Review: 阅读并点评至少一篇英文技术文章
笔者写的文章:
响应式编程或反应式编程 RxSwift和RxCocoa 从入门到精通 Reactive programming
学习并翻译下面的文章,主要是学习RxSwift和RxCocoa 响应式编程。
https://www.raywenderlich.com/1228891-getting-started-with-rxswift-and-rxcocoa
3. Tips: 学习至少一个技术技巧
笔者的文章:
Mac上的每个文件和文件夹都具有一组可配置的权限。权限控制三种访问类型:读取,写入和执行。您可以混合使用任何类型,以授予七个访问级别,如下所示。
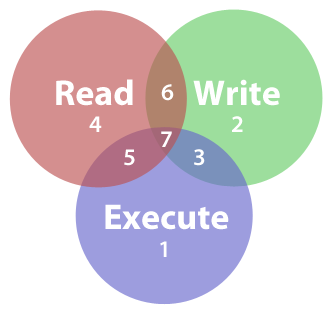
读取,写入和执行权限重叠以创建七个八进制权限表示法。
在下一部分中,您将学习如何使用“信息”窗口来修改权限。但是要真正利用权限,您需要学习基于Unix的符号和八进制权限符号,这些符号隐藏在Mac OS X图形用户界面下。下表列出了所有可用的权限。
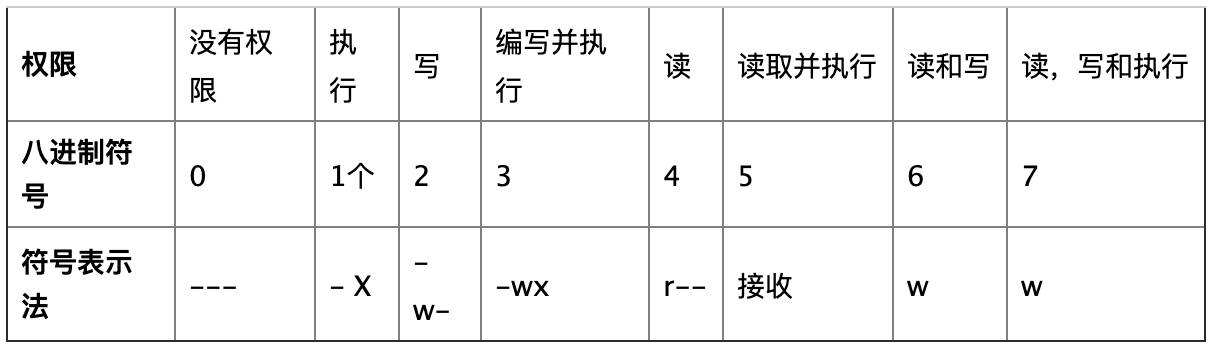
终端应用程序允许您使用八进制表示法来设置所有者,组和其他所有人的权限。要创建“只写”投递箱文件夹,可以将目录权限设置为622,以赋予所有者读和写权限,而组和其他所有人都只写权限。三组符号如下所示。
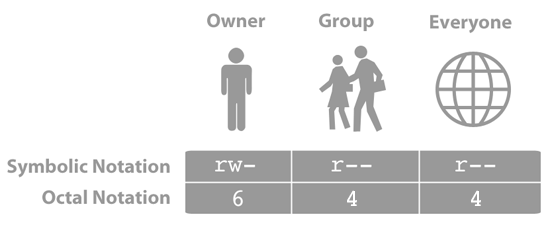
一般用到的修改为管理员最高权限为:
4. Share: 分享一篇有观点和思考的技术文章
笔者写的博客链接
Mac OS 区块链hyperledger环境搭建、环境架构介绍、环境如何用、部署 Chaincode、智能合约的调用
主要讲述如何在Mac OS中搭建Hyperledger的环境,以及架构说明
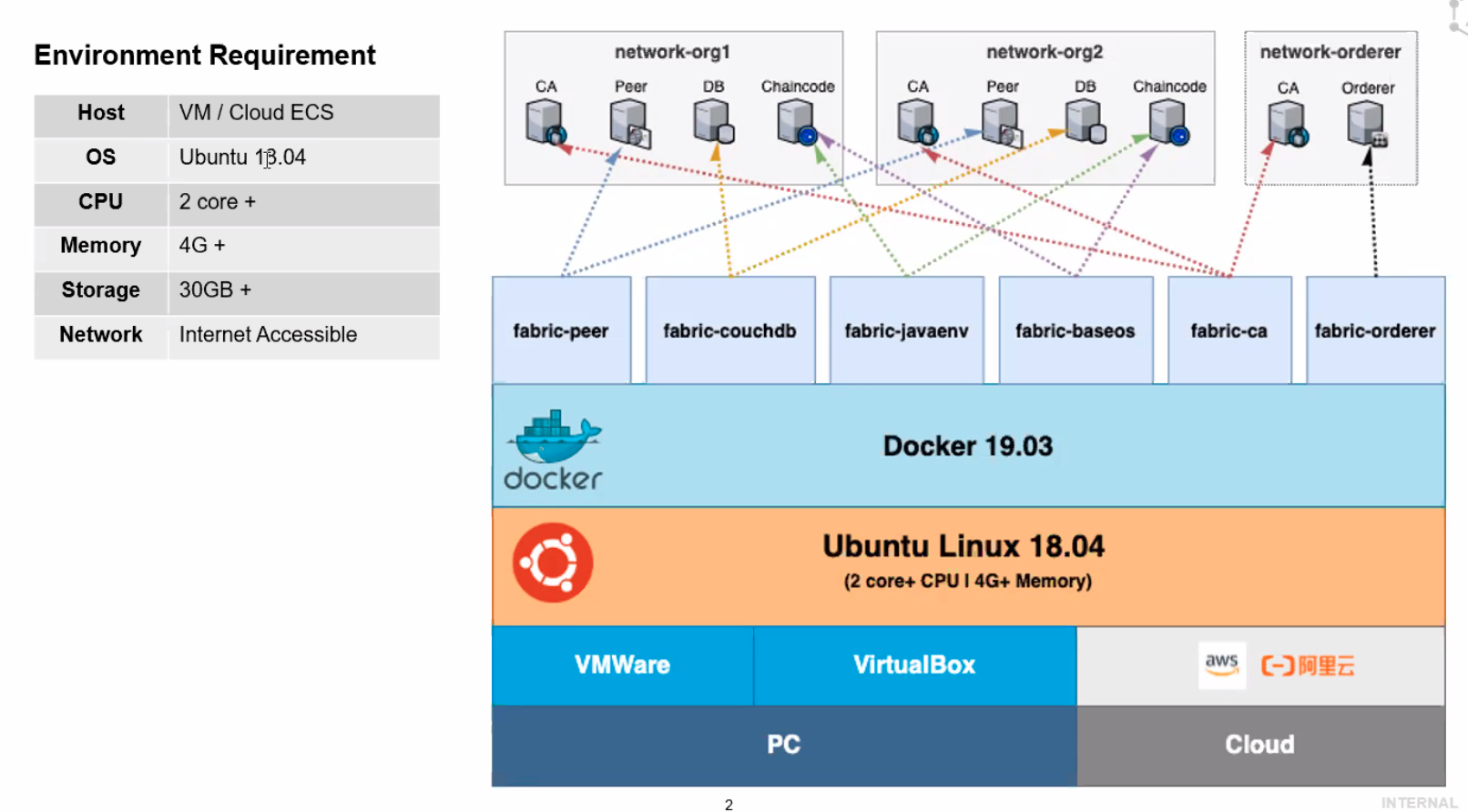
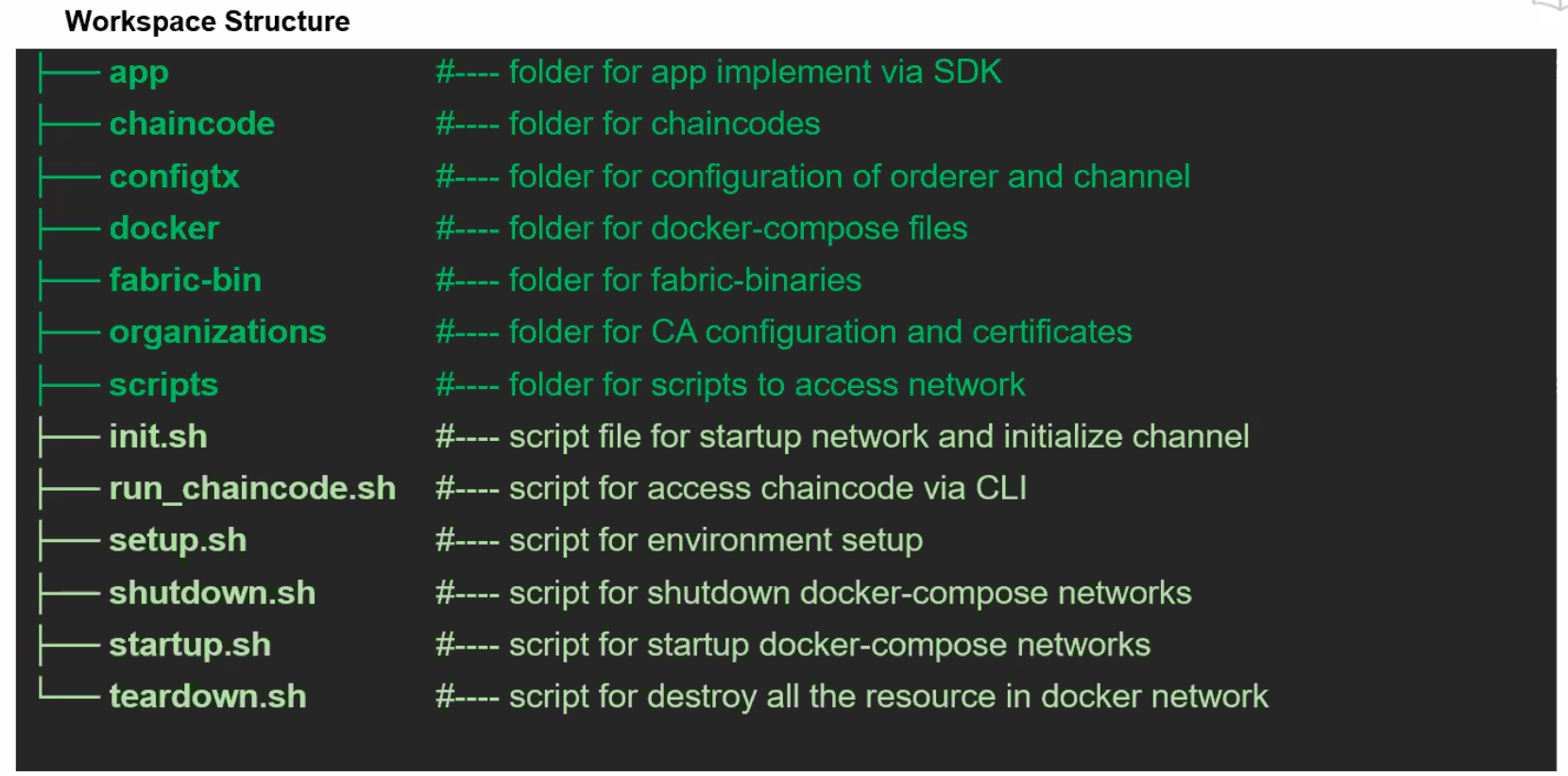
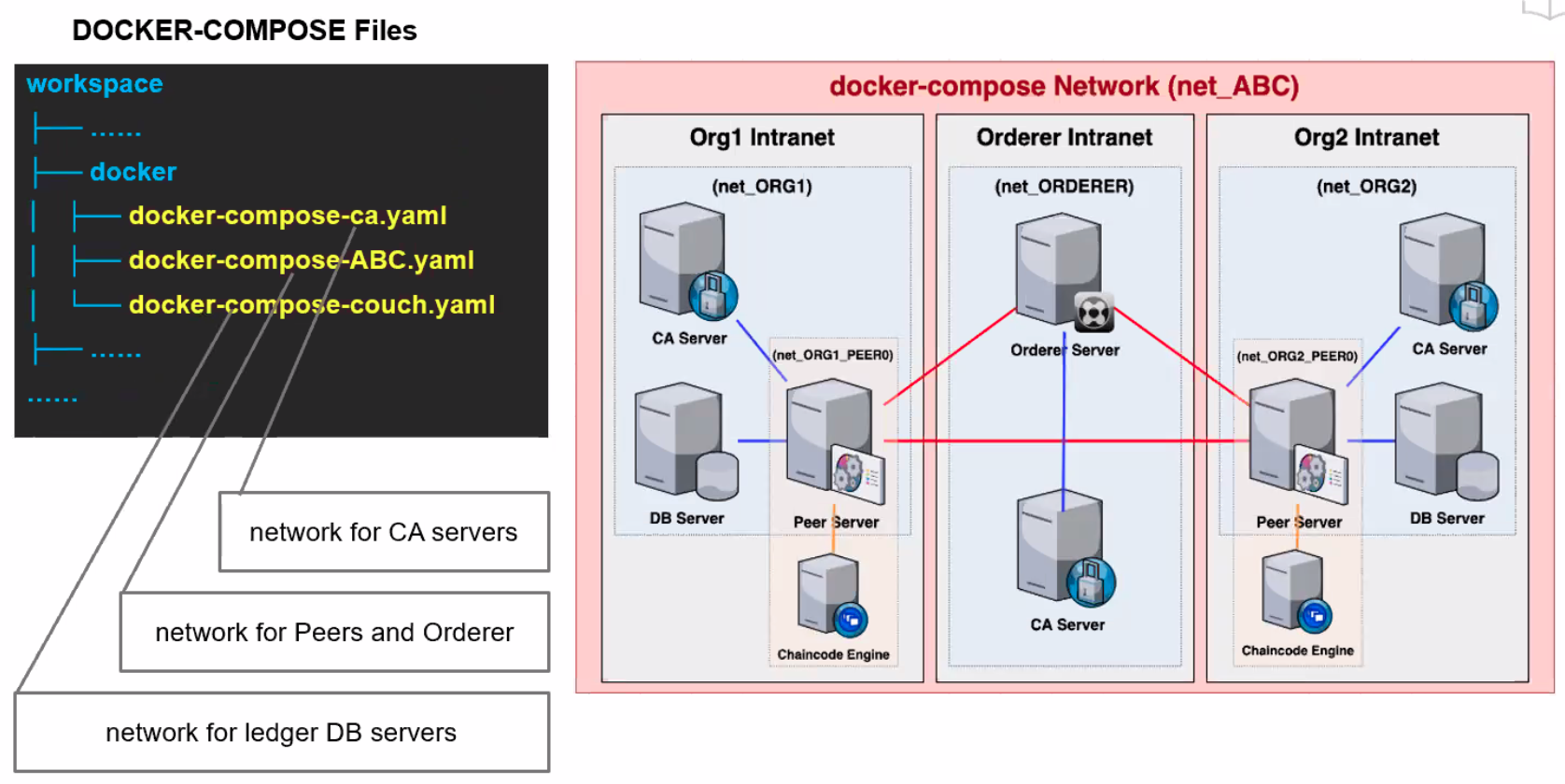
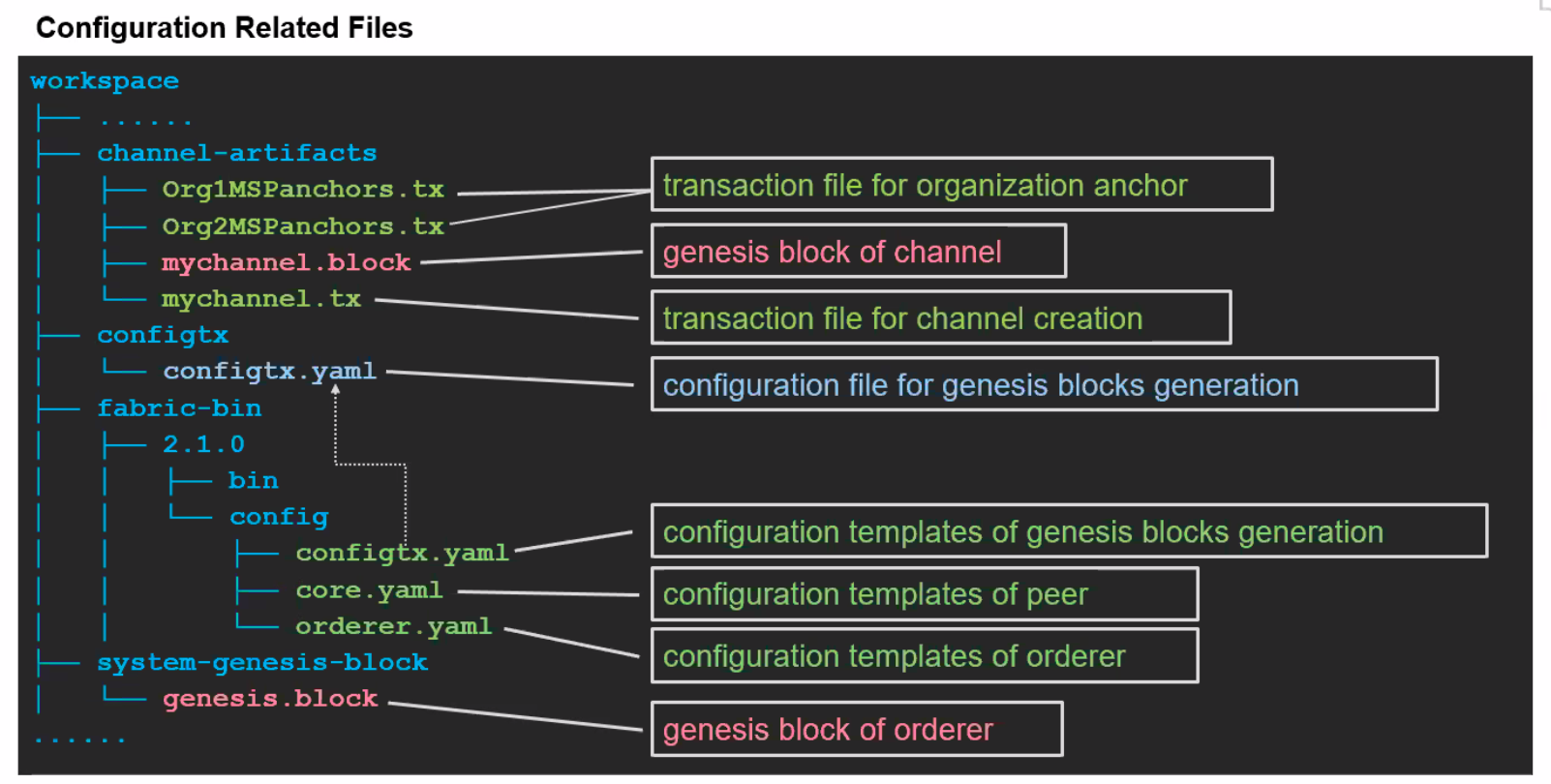
版权声明: 本文为 InfoQ 作者【John(易筋)】的原创文章。
原文链接:【http://xie.infoq.cn/article/7188194d8dcdf31ef04479cfe】。
本文遵守【CC-BY 4.0】协议,转载请保留原文出处及本版权声明。
评论