SpringBoot 分布式验证码登录方案
发布于: 2020 年 07 月 22 日
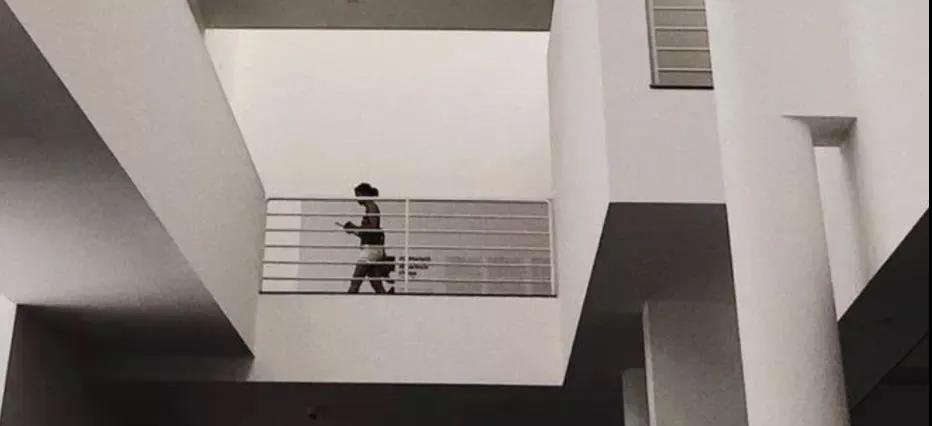
基于验证码的轮子还是挺多的,本文就以Kaptcha
这个项目为例,通过springboot项目集成Kaptcha
来实现验证码生成和登录方案。
Kaptcha介绍
Kaptcha是一个基于SimpleCaptcha的验证码开源项目
我找的这个轮子是基于SimpleCaptcha二次封装的,maven依赖如下
<!--Kaptcha是一个基于SimpleCaptcha的验证码开源项目--><dependency> <groupId>com.github.penggle</groupId> <artifactId>kaptcha</artifactId> <version>2.3.2</version></dependency>
新建项目并加入依赖
依赖主要有 SpringBoot、Kaptcha、Redis
pom.xml
<?xml version="1.0" encoding="UTF-8"?><project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.lzp</groupId> <artifactId>kaptcha</artifactId> <version>1.0-SNAPSHOT</version> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>2.3.0.RELEASE</version> <relativePath/> <!-- lookup parent from repository --> </parent> <dependencies> <!--Kaptcha是一个基于SimpleCaptcha的验证码开源项目--> <dependency> <groupId>com.github.penggle</groupId> <artifactId>kaptcha</artifactId> <version>2.3.2</version> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-redis</artifactId> </dependency> <!-- redis依赖commons-pool 这个依赖一定要添加 --> <dependency> <groupId>org.apache.commons</groupId> <artifactId>commons-pool2</artifactId> </dependency> <dependency> <groupId>com.alibaba</groupId> <artifactId>fastjson</artifactId> <version>1.2.3</version> </dependency> <dependency> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-databind</artifactId> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> </plugins> </build></project>
Redis配置类RedisConfig
@Configurationpublic class RedisConfig { @Bean public RedisTemplate<String, Object> redisTemplate(LettuceConnectionFactory redisConnectionFactory){ RedisTemplate<String, Object> redisTemplate = new RedisTemplate<String, Object>(); redisTemplate.setKeySerializer(new StringRedisSerializer()); redisTemplate.setValueSerializer(new GenericJackson2JsonRedisSerializer()); redisTemplate.setHashKeySerializer(new StringRedisSerializer()); redisTemplate.setHashValueSerializer(new GenericJackson2JsonRedisSerializer()); redisTemplate.setConnectionFactory(redisConnectionFactory); return redisTemplate; }}
验证码配置类KaptchaConfig
@Configurationpublic class KaptchaConfig { @Bean public DefaultKaptcha producer() { DefaultKaptcha defaultKaptcha = new DefaultKaptcha(); Properties properties = new Properties(); properties.setProperty("kaptcha.border", "no"); properties.setProperty("kaptcha.border.color", "105,179,90"); properties.setProperty("kaptcha.textproducer.font.color", "black"); properties.setProperty("kaptcha.image.width", "110"); properties.setProperty("kaptcha.image.height", "40"); properties.setProperty("kaptcha.textproducer.char.string","23456789abcdefghkmnpqrstuvwxyzABCDEFGHKMNPRSTUVWXYZ"); properties.setProperty("kaptcha.textproducer.font.size", "30"); properties.setProperty("kaptcha.textproducer.char.space","3"); properties.setProperty("kaptcha.session.key", "code"); properties.setProperty("kaptcha.textproducer.char.length", "4"); properties.setProperty("kaptcha.textproducer.font.names", "宋体,楷体,微软雅黑"); properties.setProperty("kaptcha.noise.impl","com.google.code.kaptcha.impl.NoNoise"); Config config = new Config(properties); defaultKaptcha.setConfig(config); return defaultKaptcha; } }
验证码控制层CaptchaController
import com.google.code.kaptcha.impl.DefaultKaptcha;import com.lzp.kaptcha.service.CaptchaService;import com.lzp.kaptcha.vo.CaptchaVO;import org.springframework.beans.factory.annotation.Autowired;import org.springframework.web.bind.annotation.GetMapping;import org.springframework.web.bind.annotation.RequestMapping;import org.springframework.web.bind.annotation.ResponseBody;import org.springframework.web.bind.annotation.RestController;import sun.misc.BASE64Encoder;import javax.imageio.ImageIO;import java.awt.image.BufferedImage;import java.io.ByteArrayOutputStream;import java.io.IOException;@RestController@RequestMapping("/captcha")public class CaptchaController { @Autowired private DefaultKaptcha producer; @Autowired private CaptchaService captchaService; @ResponseBody @GetMapping("/get") public CaptchaVO getCaptcha() throws IOException { // 生成文字验证码 String content = producer.createText(); // 生成图片验证码 ByteArrayOutputStream outputStream = null; BufferedImage image = producer.createImage(content); outputStream = new ByteArrayOutputStream(); ImageIO.write(image, "jpg", outputStream); // 对字节数组Base64编码 BASE64Encoder encoder = new BASE64Encoder(); String str = "data:image/jpeg;base64,"; String base64Img = str + encoder.encode(outputStream.toByteArray()).replace("\n", "").replace("\r", ""); CaptchaVO captchaVO = captchaService.cacheCaptcha(content); captchaVO.setBase64Img(base64Img); return captchaVO; }}
验证码返回对象CaptchaVO
public class CaptchaVO { /** * 验证码标识符 */ private String captchaKey; /** * 验证码过期时间 */ private Long expire; /** * base64字符串 */ private String base64Img; public String getCaptchaKey() { return captchaKey; } public void setCaptchaKey(String captchaKey) { this.captchaKey = captchaKey; } public Long getExpire() { return expire; } public void setExpire(Long expire) { this.expire = expire; } public String getBase64Img() { return base64Img; } public void setBase64Img(String base64Img) { this.base64Img = base64Img; }}
验证码方法层CaptchaService
import com.lzp.kaptcha.utils.RedisUtils;import com.lzp.kaptcha.vo.CaptchaVO;import org.springframework.beans.factory.annotation.Autowired;import org.springframework.beans.factory.annotation.Value;import org.springframework.stereotype.Service;import java.util.UUID;@Servicepublic class CaptchaService { @Value("${server.session.timeout:300}") private Long timeout; @Autowired private RedisUtils redisUtils; private final String CAPTCHA_KEY = "captcha:verification:"; public CaptchaVO cacheCaptcha(String captcha) { //生成一个随机标识符 String captchaKey = UUID.randomUUID().toString(); //缓存验证码并设置过期时间 redisUtils.set(CAPTCHA_KEY.concat(captchaKey),captcha,timeout); CaptchaVO captchaVO = new CaptchaVO(); captchaVO.setCaptchaKey(captchaKey); captchaVO.setExpire(timeout); return captchaVO; }}
用户登录对象封装LoginDTO
public class LoginDTO { private String userName; private String pwd; private String captchaKey; private String captcha; public String getUserName() { return userName; } public void setUserName(String userName) { this.userName = userName; } public String getPwd() { return pwd; } public void setPwd(String pwd) { this.pwd = pwd; } public String getCaptchaKey() { return captchaKey; } public void setCaptchaKey(String captchaKey) { this.captchaKey = captchaKey; } public String getCaptcha() { return captcha; } public void setCaptcha(String captcha) { this.captcha = captcha; }}
登录控制层UserController
import com.lzp.kaptcha.dto.LoginDTO;import com.lzp.kaptcha.utils.RedisUtils;import com.lzp.kaptcha.vo.UserVO;import org.springframework.beans.factory.annotation.Autowired;import org.springframework.web.bind.annotation.PostMapping;import org.springframework.web.bind.annotation.RequestBody;import org.springframework.web.bind.annotation.RequestMapping;import org.springframework.web.bind.annotation.RestController;@RestController@RequestMapping("/user")public class UserController { @Autowired private RedisUtils redisUtils; @PostMapping("/login") public UserVO login(@RequestBody LoginDTO loginDTO) { Object captch = redisUtils.get(loginDTO.getCaptchaKey()); if(captch == null) { // throw 验证码已过期 } if(!loginDTO.getCaptcha().equals(captch)) { // throw 验证码错误 } // 查询用户信息 //判断用户是否存在 不存在抛出用户名密码错误 //判断密码是否正确,不正确抛出用户名密码错误 //构造返回到前端的用户对象并封装信息和生成token return new UserVO(); } }
划线
评论
复制
发布于: 2020 年 07 月 22 日阅读数: 78
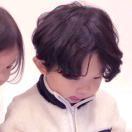
Bruce Duan
关注
做最好版本的自己 2020.05.01 加入
主要分享Java服务端相关的技术,欢迎关注!啥也不说了上代码开始撸。
评论