架构师训练营 - 第三周 -20200624- 单例模式和组合模式
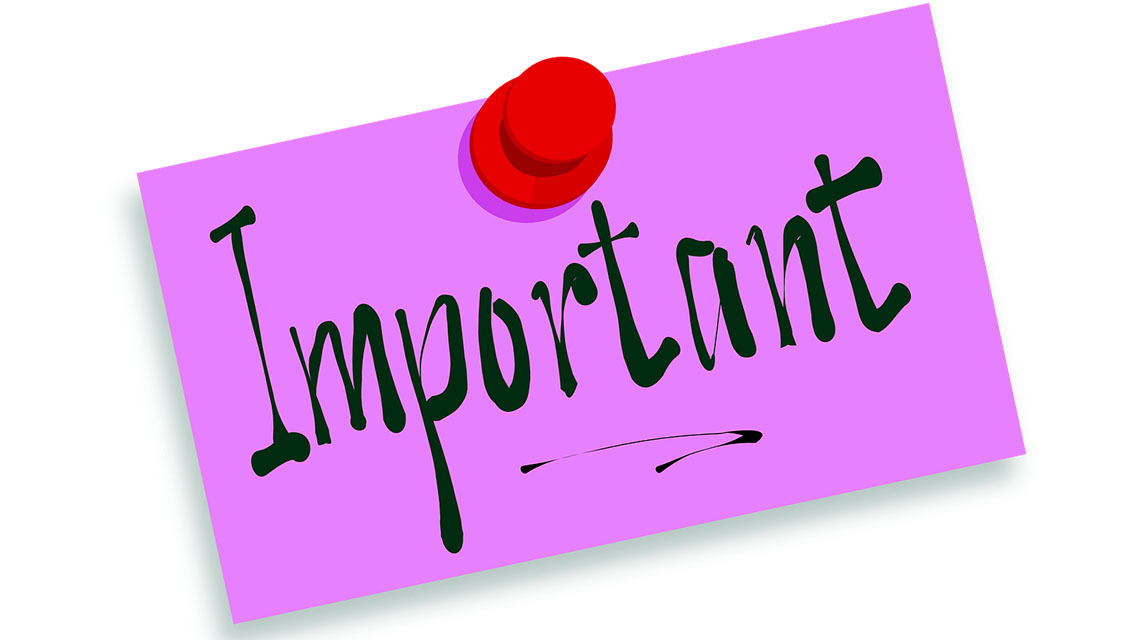
1、手写单例模式:
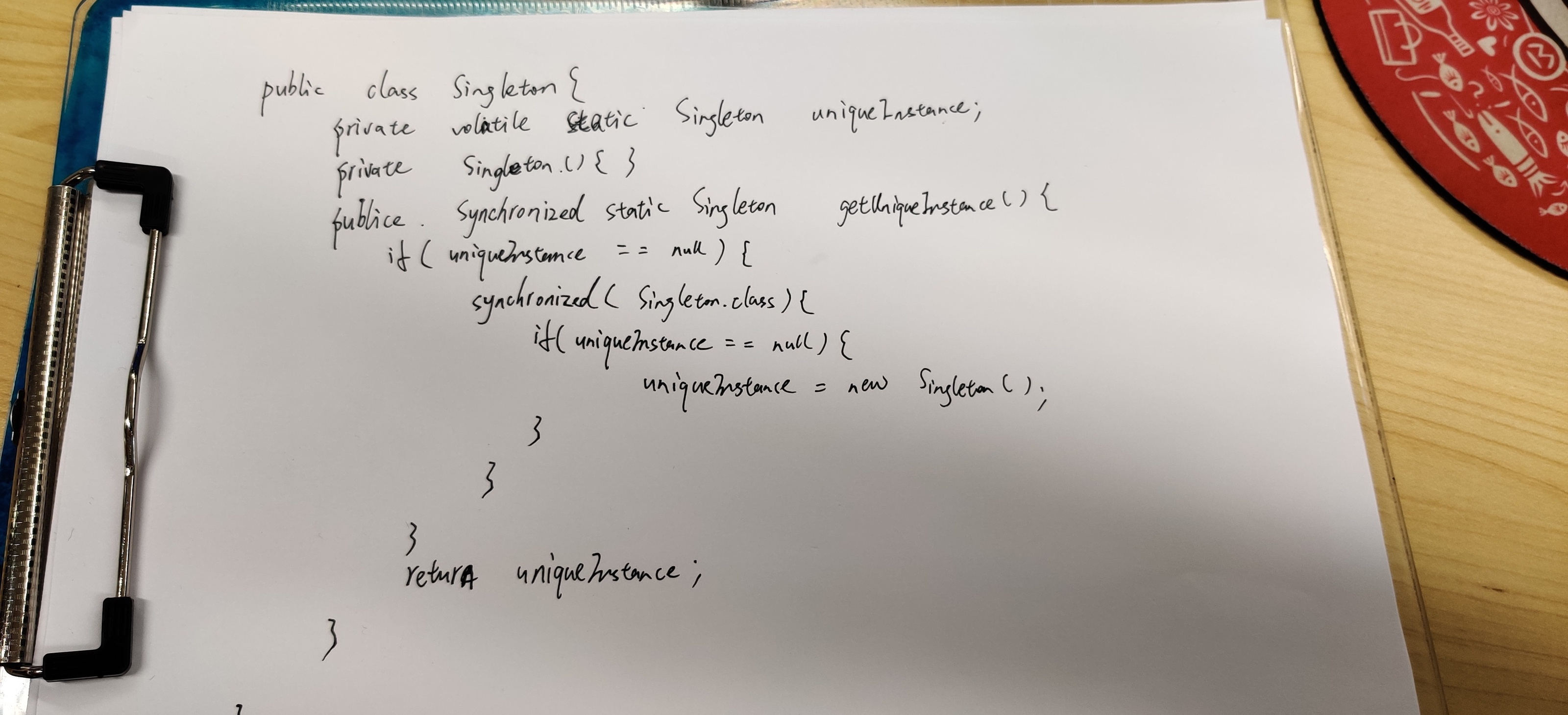
手写的看不太清楚,贴上源码:
关键的点有两个:
双重检查
通过volatile关键字禁止指令重排,防止返回的对象还未进行初始化,造成空指针,虽然这个概率比较小
2、组合模式
组合模式(Composite Pattern),又叫部分整体模式,是将对象组合成树形结构来表现“部分-整体”的层次结构。属于结构型设计模式,它创建了对象组的树形结构,使得客户端可以用一致的方式处理单个对象以及对象的组合。
组合模式实现的最关键的地方是——简单对象和复合对象必须实现相同的接口。这就是组合模式能够将组合对象和简单对象进行一致处理的原因。
组合模式的构成部分:
组合部件(Component):它是一个抽象角色,为要组合的对象提供统一的接口。
叶子(Leaf):在组合中表示子节点对象,叶子节点不能有子节点。
合成部件(Composite):定义有枝节点的行为,用来存储部件,实现在Component接口中的有关操作,如增加(Add)或删除(Remove)。
作业:
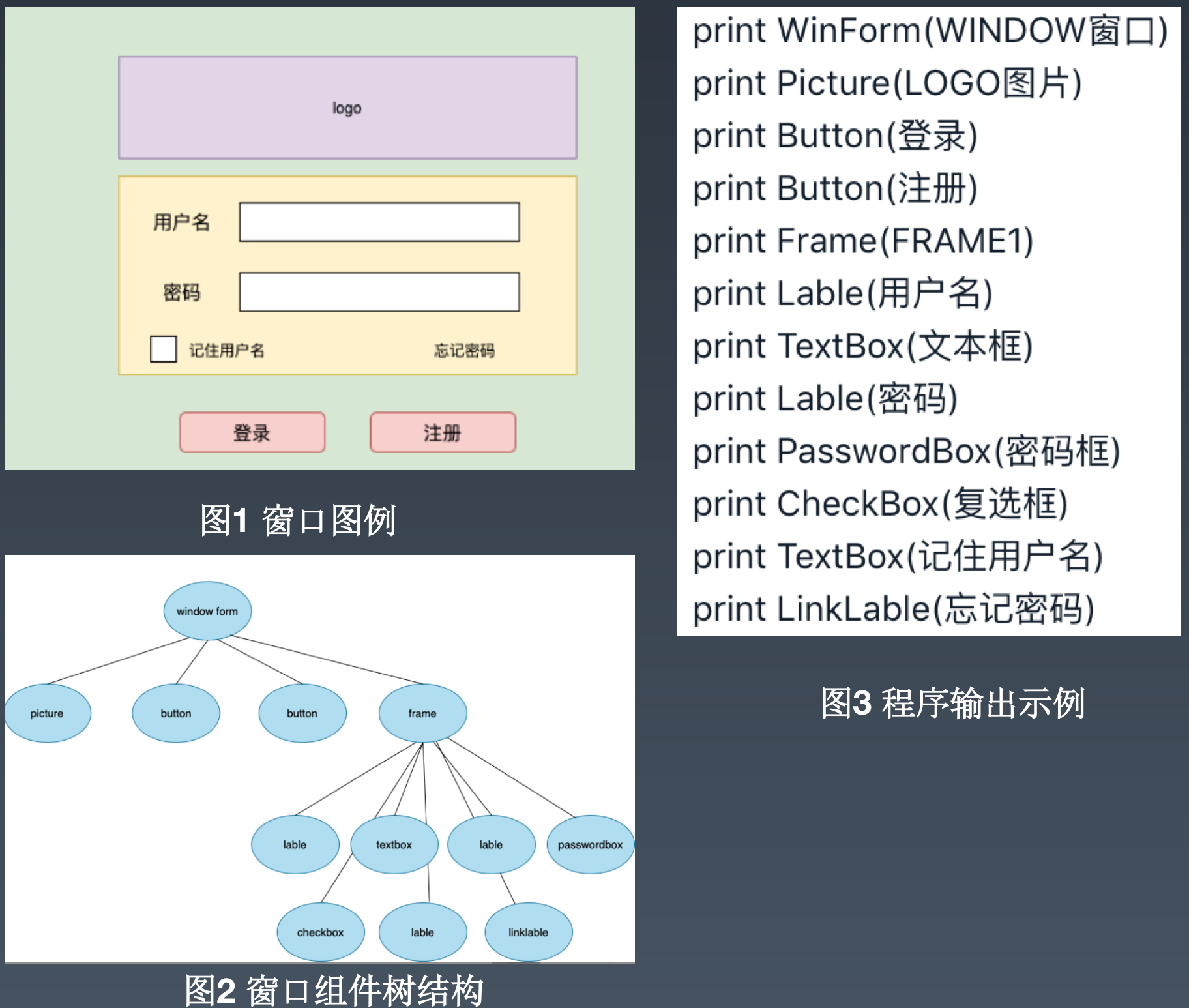
具体代码:
最后的输出结果:
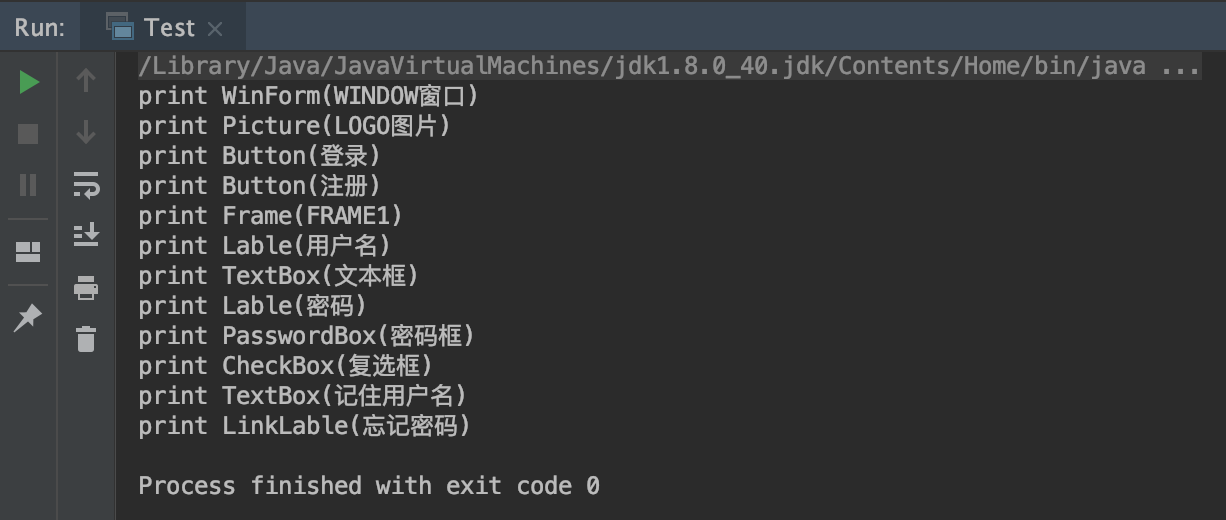
总体来说,demo还是很好理解的,关键是如何在自己日常的业务开发中真正运用起来,推荐一位大佬写的设计模式实战系列中的例子:
https://xie.infoq.cn/article/42190edf005afdf1b47d9edeb
版权声明: 本文为 InfoQ 作者【丁亚宁】的原创文章。
原文链接:【http://xie.infoq.cn/article/6d783da61d6035f09b448c03b】。文章转载请联系作者。
评论