week3 作业
发布于: 2020 年 06 月 24 日
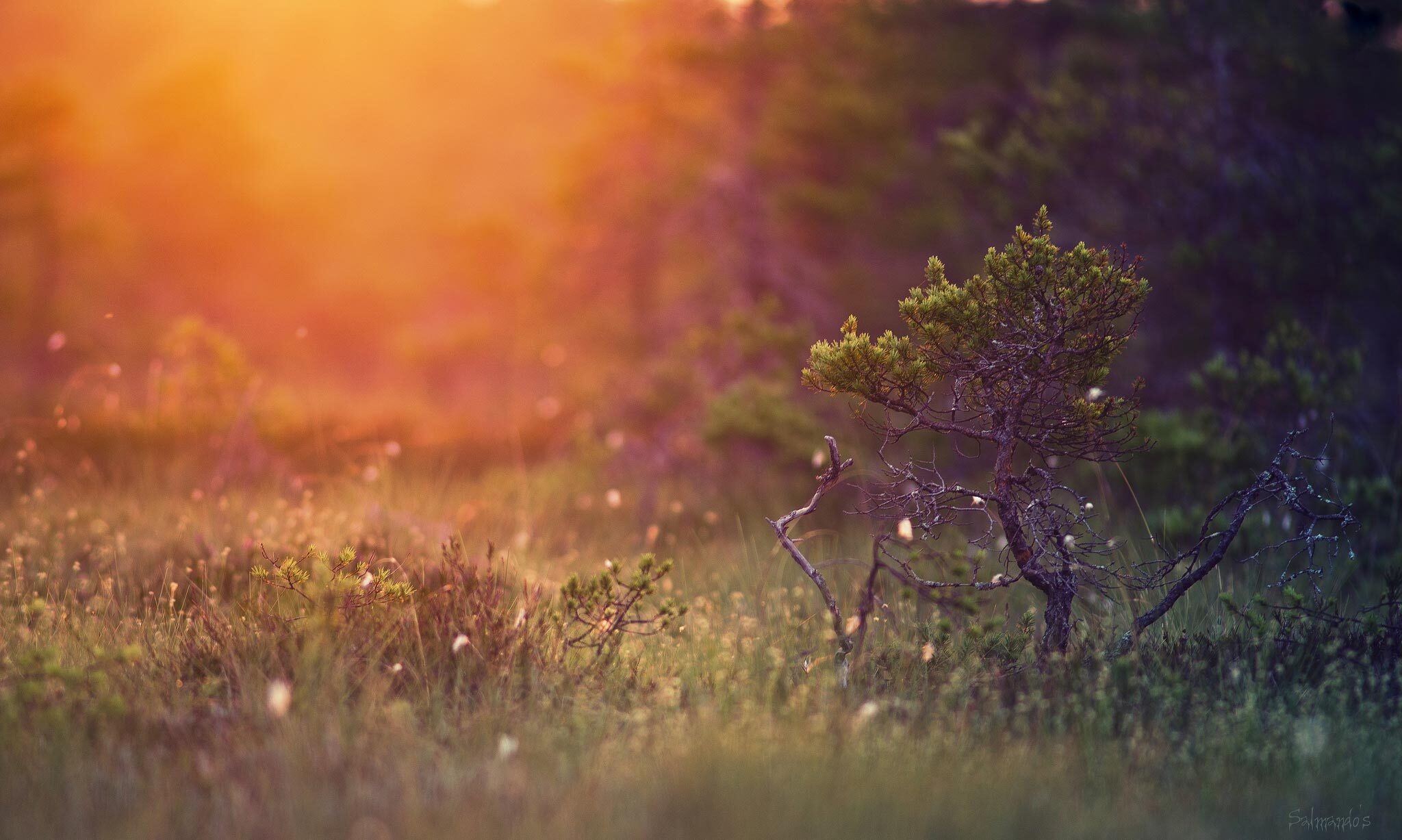
作业一:
1. 请在草稿纸上手写一个单例模式的实现代码,拍照提交作业。
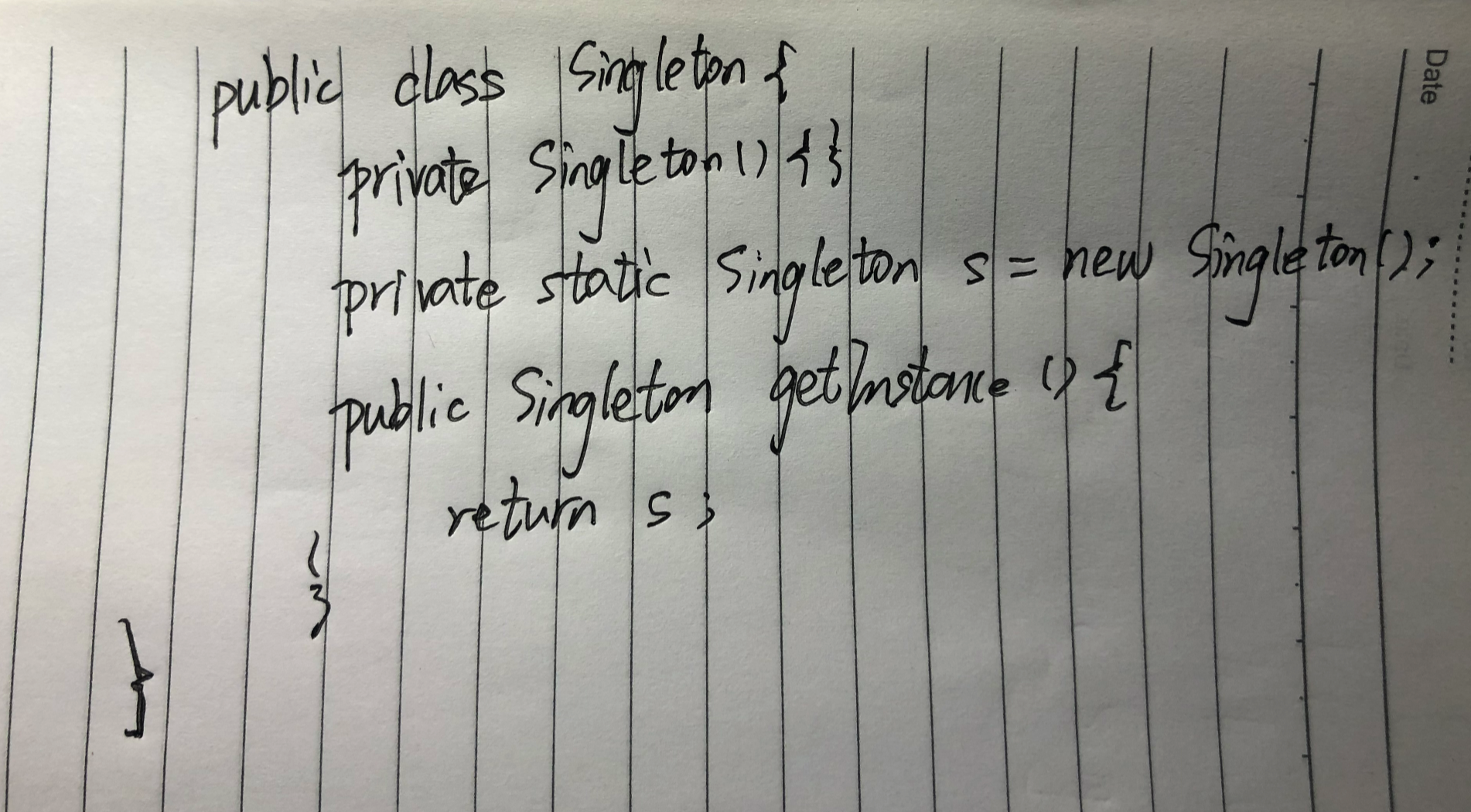
2. 请用组合设计模式编写程序,打印输出图 1 的窗口,窗口组件的树结构如图 2 所示,打印输出示例参考图 3。
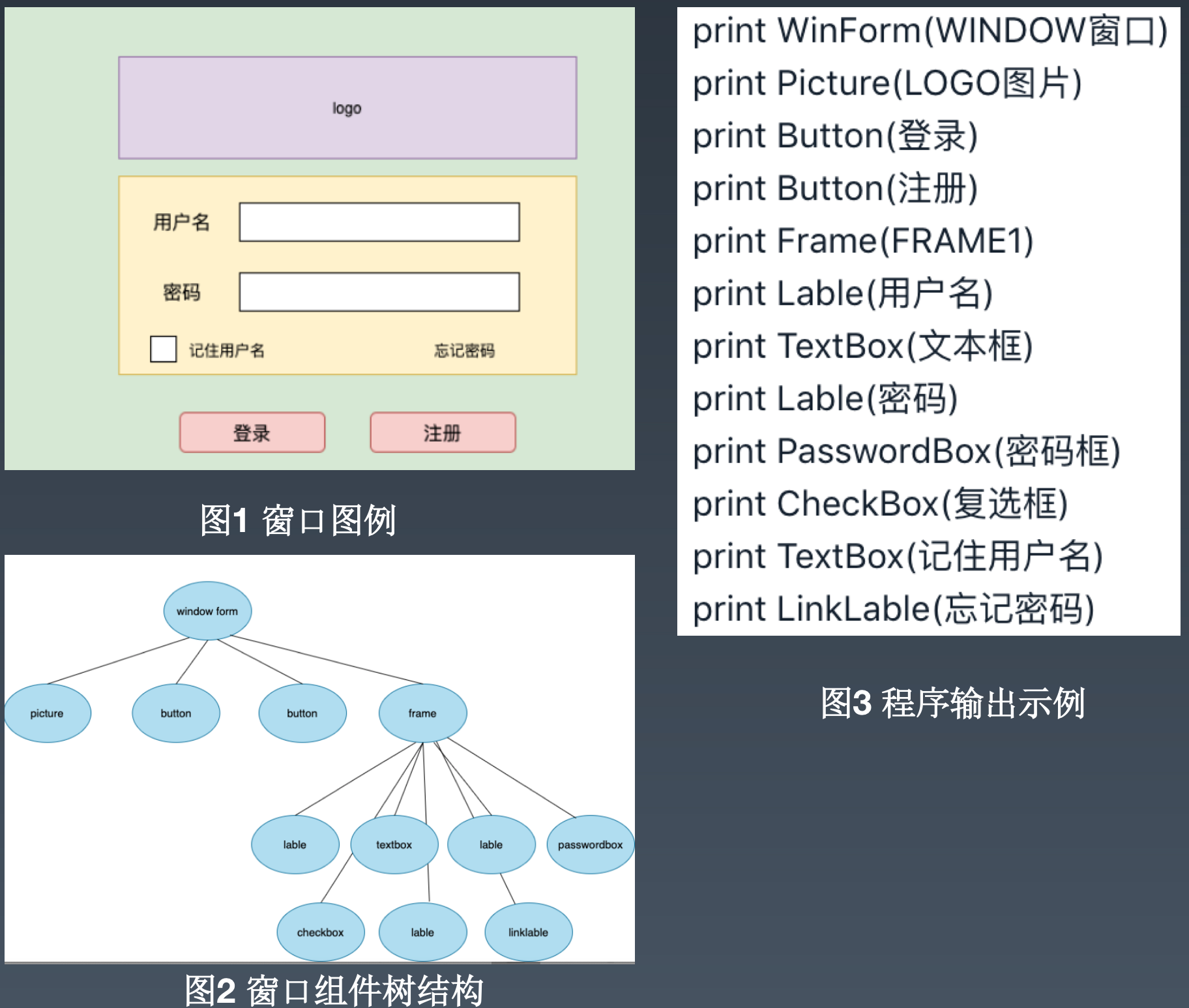
具体实现
其实应该针对不同的组件建立不同的类(继承 LeafComposite或 BranchComposite),但是为了简单的表示,这里就不细分具体组件。下面是我的代码
组件类
package com.zj.geek.week3;public abstract class Component { /** * 组件名称 */ private String name; /** * 组件显示信息 */ private String showInfo; public Component(String name, String showInfo) { this.name = name; this.showInfo = showInfo; } public String getName() { return name; } public String getShowInfo() { return showInfo; } public void print(){ System.out.println("print " + this.getName() + "(" + this.getShowInfo() + ")"); }}
树枝组件类
package com.zj.geek.week3;import java.util.ArrayList;import java.util.List;/** * 树枝组件是有子节点 */public class BranchComposite extends Component { private List<Component> components = new ArrayList<>(); public BranchComposite(String name, String showInfo) { super(name, showInfo); } //add BranchComposite public void add(Component component) { this.components.add(component); } //remove BranchComposite public void remove(Component component) { if (this.components.contains(component)) { this.components.remove(component); } } @Override public void print() { super.print(); components.forEach(Component::print); }}
叶子组件类
package com.zj.geek.week3;/** * 叶子组件是没有子节点的 */public class LeafComposite extends Component { public LeafComposite(String name, String showInfo) { super(name, showInfo); }}
场景类
package com.zj.geek.week3;/** * @author zj */public class Client { public static void main(String[] args) { BranchComposite windowForm = new BranchComposite("WinForm", "WINDOWS窗口"); LeafComposite picture = new LeafComposite("Picture","LOGO图片"); windowForm.add(picture); LeafComposite loginButton = new LeafComposite("Button","登录"); windowForm.add(loginButton); LeafComposite registerButton = new LeafComposite("Button","注册"); windowForm.add(registerButton); BranchComposite frame = new BranchComposite("Frame", "FRAME1"); LeafComposite userLable = new LeafComposite("Lable","用户名"); frame.add(userLable); LeafComposite textBox = new LeafComposite("TextBox","文本框"); frame.add(textBox); LeafComposite passWordLable = new LeafComposite("Lable","密码"); frame.add(passWordLable); LeafComposite passWordBox = new LeafComposite("PassWordBox","密码框"); frame.add(passWordBox); LeafComposite checkBox = new LeafComposite("CheckBox","复选框"); frame.add(checkBox); LeafComposite rememberTextBox = new LeafComposite("TextBox","记住用户名"); frame.add(rememberTextBox); LeafComposite linkLable = new LeafComposite("LinkLable","忘记密码"); frame.add(linkLable); windowForm.add(frame); //输出 windowForm.print(); }}
具体输出结果如下:
print WinForm(WINDOWS窗口)print Picture(LOGO图片)print Button(登录)print Button(注册)print Frame(FRAME1)print Lable(用户名)print TextBox(文本框)print Lable(密码)print PassWordBox(密码框)print CheckBox(复选框)print TextBox(记住用户名)print LinkLable(忘记密码)
划线
评论
复制
发布于: 2020 年 06 月 24 日阅读数: 49
版权声明: 本文为 InfoQ 作者【张健】的原创文章。
原文链接:【http://xie.infoq.cn/article/6a8f336b115ca216f97e5b0af】。文章转载请联系作者。
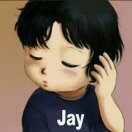
张健
关注
还未添加个人签名 2018.04.20 加入
还未添加个人简介
评论 (1 条评论)