性能压测工具
springboot项目 工程目录
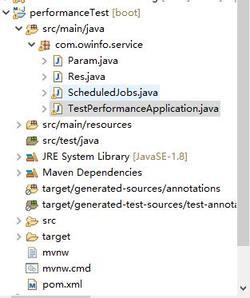
Param 请求参数 Res 响应参数 ScheduledJobs 测试处理类
TestPerformanceApplication springboot启动类
public class Param {
String url; //请求url
Integer concurrency;//并发
}
public class Res {
String avgRt; //平均时间
String percent95Rt;//95%时间
}
//ScheduledJobs 测试处理类
package com.owinfo.service;
import java.time.Instant;
import java.util.ArrayList;
import java.util.List;
import java.util.Map.Entry;
import java.util.Set;
import java.util.TreeMap;
import java.util.concurrent.Callable;
import java.util.concurrent.Future;
import java.util.concurrent.ThreadPoolExecutor;
import org.springframework.scheduling.concurrent.ThreadPoolTaskExecutor;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.client.RestTemplate;
@RestController
@RequestMapping("/test")
public class ScheduledJobs {
@RequestMapping("/performance")
private Res testPerformance(Param param) throws Exception {
//响应时间Tree
TreeMap<Long,Long> tree = new TreeMap<>();
//创建线程池
ThreadPoolTaskExecutor executor = new ThreadPoolTaskExecutor();
executor.setCorePoolSize(150);//配置核心线程数
executor.setMaxPoolSize(200);//配置最大线程数
executor.setQueueCapacity(200);//配置队列大小
executor.setKeepAliveSeconds(60);
executor.setRejectedExecutionHandler(new ThreadPoolExecutor.CallerRunsPolicy());
executor.setWaitForTasksToCompleteOnShutdown(true);
executor.setAwaitTerminationSeconds(60);
executor.initialize();
//每次响应时间结果
List<Future<Long>> results=new ArrayList<Future<Long>>();
String url =param.getUrl();//请求路径
int concurrency = param.getConcurrency();//并发数
for (int i = 0; i < concurrency; i++) {
results.add(executor.submit(new TestJob(url)));
}
//取出每次响应时间
for(Future<Long> fs :results) {
tree.put(fs.get(), fs.get());
}
System.out.println(tree);
//计算平均时间
Long sum = 0L;
Set<Long> keySet = tree.keySet();
for (Long long1 : keySet) {
sum+=long1;
}
int num = keySet.size();
String avgRt = (sum/num)+"";//平均时间
//计算95%时间
int index95Percent = Integer.parseInt((Math.round(Double.parseDouble(num+"")*0.95)-1)+"") ;
Entry<Long,Long> en = (Entry<Long,Long>) tree.entrySet().toArray()[index95Percent];
String percent95Rt = en.getKey().toString();//95%时间
Res res = new Res();
res.setAvgRt(avgRt);
res.setPercent95Rt(percent95Rt);
return res;
}
}
class TestJob implements Callable {
String url;
public TestJob(String url) {
super();
this.url = url;
}
public String getUrl() {
return url;
}
public void setUrl(String url) {
this.url = url;
}
@Override
public Long call() throws Exception {
Long startEpochMilli = Instant.now().toEpochMilli();
new RestTemplate().getForObject(this.getUrl(), String.class);
Long endEpochMilli = Instant.now().toEpochMilli();
Long rt = endEpochMilli - startEpochMilli;
return rt;
}
}
//TestPerformanceApplication boot启动类
package com.owinfo.service;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class TestPerformanceApplication {
public static void main(String[] args) {
SpringApplication.run(TestPerformanceApplication.class, args);
}
}
pom
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.1.1.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>com.owinfo</groupId>
<artifactId>performanceTest</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>performanceTest</name>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-api</artifactId>
<type>jar</type>
<scope>compile</scope>
</dependency>
<!-- json -->
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>fastjson</artifactId>
<version>1.2.3</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-aop</artifactId>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
测试结果
10并发百度
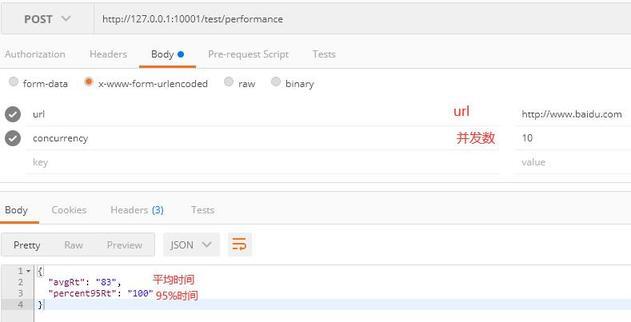
100并发百度
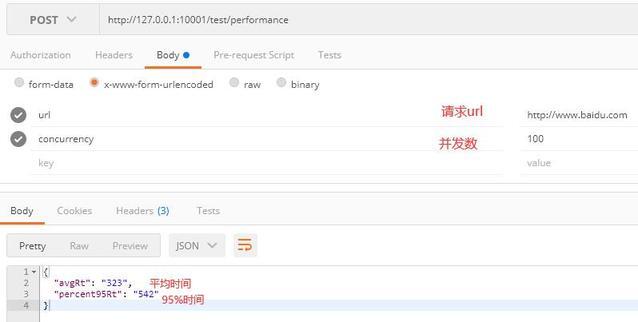
评论