Week 03- 作业一:设计模式
发布于: 2020 年 06 月 22 日
请在草稿纸上手写一个单例模式的实现代码,拍照提交作业:
Singleton 属于创建型模式,它提供了一种创建对象的最佳方式。
涉及到一个单一的类,该类负责创建自己的对象,同时确保只有单个对象被创建。这个类提供了一种访问其唯一的对象的方式,可以直接访问,不需要实例化该类的对象。
代码基于.net core+c#实现:
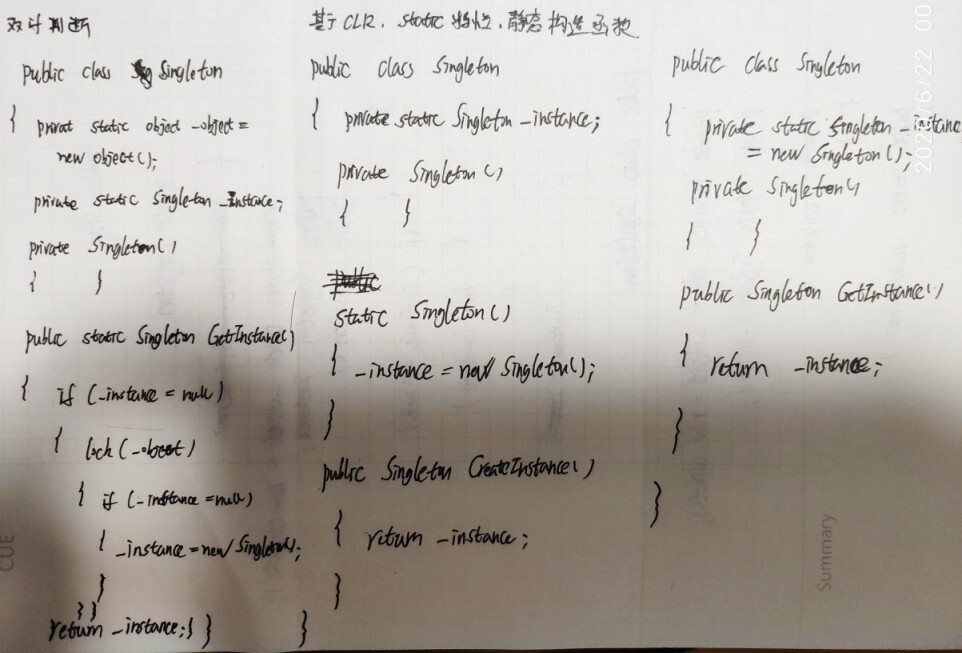
请用组合设计模式编写程序,打印输出图 1 的窗口,窗口组件的树结构如图 2 所示,打印输出示例参考图 3。
public abstract class Component { protected string _name; protected string _key; public Component(string key,string name) { this._key = key; this._name = name; } public abstract void Add(Component c); public abstract void Remove(Component c); public abstract void Print(int depth); } public class Composite : Component { private List<Component> _children = new List<Component>(); public Composite(string key, string name) : base(key, name) { } public override void Add(Component component) { _children.Add(component); } public override void Remove(Component component) { _children.Remove(component); } public override void Print(int depth) { Console.WriteLine($"{ new String('-', depth)}+{_key}({_name})"); foreach (Component component in _children) { component.Print(depth + 2); } } } public class Leaf : Component { public Leaf(string key,string name) : base(key,name) { } public override void Add(Component c) { Console.WriteLine("Cannot add to a leaf"); } public override void Remove(Component c) { Console.WriteLine("Cannot remove from a leaf"); } public override void Print(int depth) { Console.WriteLine($"{ new String('-', depth)}{_key}({_name})"); } } // Create a tree structure Composite root = new Composite("WinForm","WINDOW窗口"); root.Add(new Leaf("Picture","LOGO图片")); root.Add(new Leaf("Button", "登录")); root.Add(new Leaf("Button", "注册")); Composite compFrame = new Composite("Frame", "FRAME1"); compFrame.Add(new Leaf("Lable","用户名")); compFrame.Add(new Leaf("TextBox", "文本框")); compFrame.Add(new Leaf("Lable", "密码")); compFrame.Add(new Leaf("PasswordBox", "密码框")); compFrame.Add(new Leaf("ChexkBox", "复选框")); compFrame.Add(new Leaf("TextBox", "记住用户名")); compFrame.Add(new Leaf("LinkLable", "忘记密码")); root.Add(compFrame); //// Add and remove a leaf //Leaf leaf = new Leaf("WinForm", "WINDOW窗口"); //root.Add(leaf); //root.Remove(leaf); // Recursively print tree root.Print(1);
result:-+WinForm(WINDOW窗口)---Picture(LOGO图片)---Button(登录)---Button(注册)---+Frame(FRAME1)-----Lable(用户名)-----TextBox(文本框)-----Lable(密码)-----PasswordBox(密码框)-----ChexkBox(复选框)-----TextBox(记住用户名)-----LinkLable(忘记密码)
划线
评论
复制
发布于: 2020 年 06 月 22 日 阅读数: 33
版权声明: 本文为 InfoQ 作者【dean】的原创文章。
原文链接:【http://xie.infoq.cn/article/63b88985e99d330e69369eb80】。文章转载请联系作者。
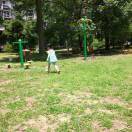
dean
关注
还未添加个人签名 2019.11.06 加入
还未添加个人简介
评论