1
架构师训练营 -Week 03 命题作业
发布于: 2020 年 06 月 24 日
请在草稿纸上手写一个单例模式的实现代码,拍照提交作业。
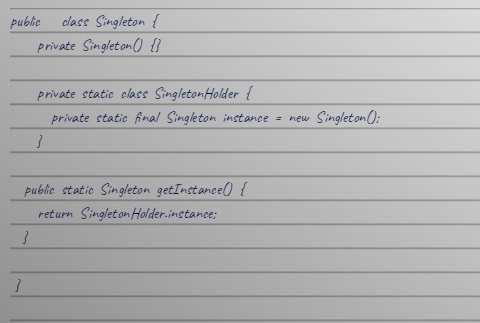
请用组合设计模式编写程序,打印输出图 1 的窗口,窗口组件的树结构如图 2 所示,打印输出示例参考图 3。
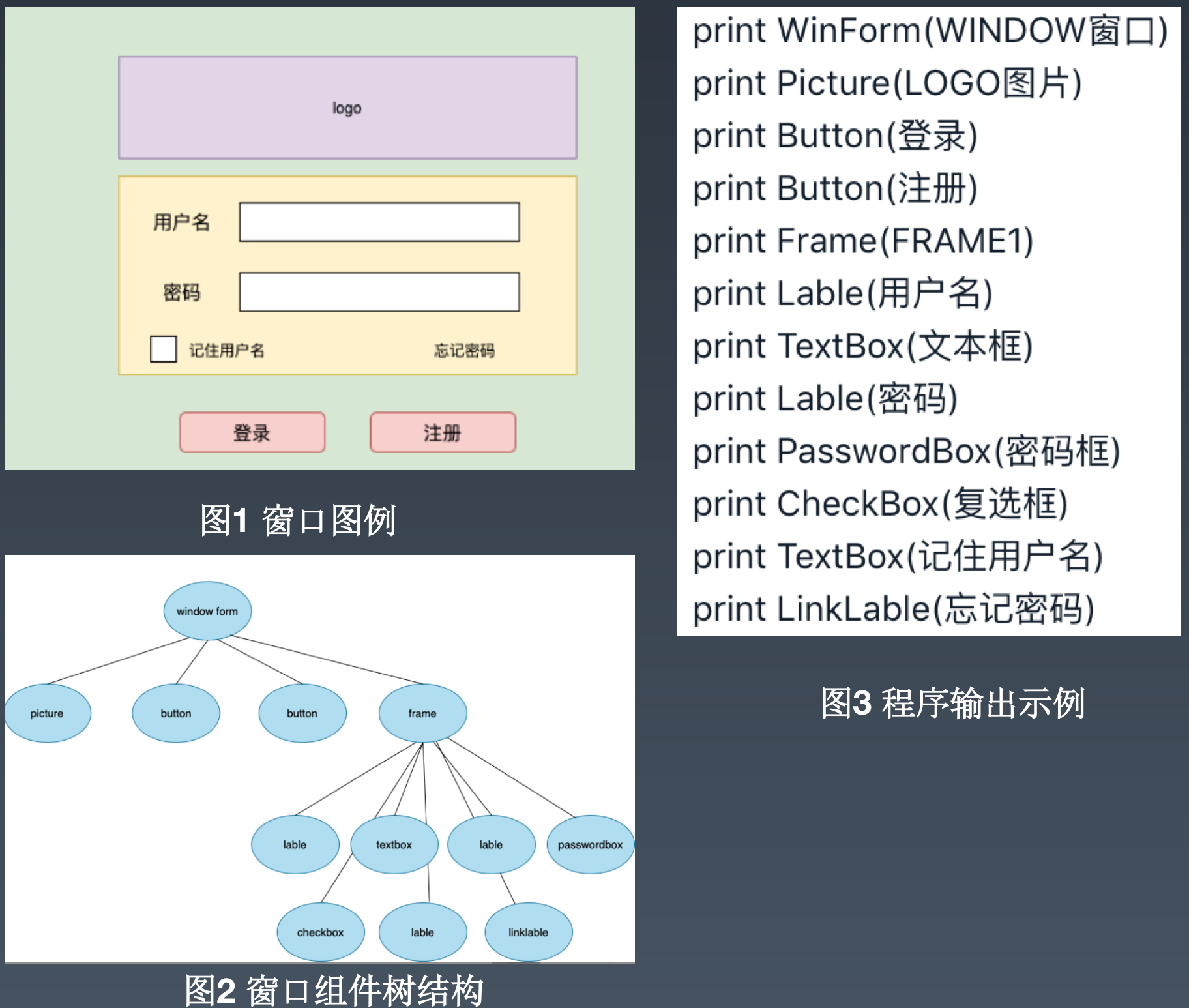
类图:
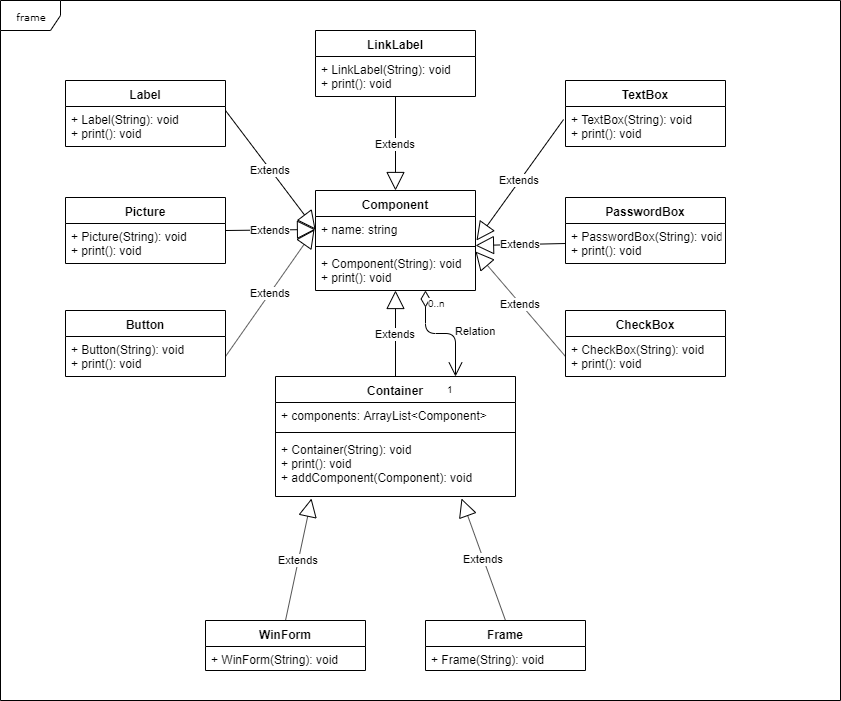
代码实现:
Component类
public abstract class Component { private String name; protected Component(String name) { this.name = name; } public String getName() { return name; } public abstract void print();}
Container类
public class Container extends Component { private List<Component> components = new ArrayList<>(); public Container(String name) { super(name); } public void addComponent(Component component) { components.add(component); } public Component getComponent(int n) { try { return components.get(n); } catch (IndexOutOfBoundsException z) { throw new ArrayIndexOutOfBoundsException("No such child: " + n); } } public List<Component> getComponents() { return components; } @Override public void print() { System.out.printf("print %s(%s)\n", getClass().getSimpleName(), getName()); printComponents(); } public void printComponents() { for (Component component : components) { component.print(); } }}
WinForm类
public class WinForm extends Container { public WinForm(String name) { super(name); }}
Frame类
public class Frame extends Container { public Frame(String name) { super(name); }}
Picture类
public class Picture extends Component { public Picture(String name) { super(name); } @Override public void print() { System.out.printf("print %s(%s)\n", getClass().getSimpleName(), getName()); }}
Button类
public class Button extends Component{ public Button(String name) { super(name); } @Override public void print() { System.out.printf("print %s(%s)\n", getClass().getSimpleName(), getName()); }}
Label类
public class Label extends Component { public Label(String name) { super(name); } @Override public void print() { System.out.printf("print %s(%s)\n", getClass().getSimpleName(), getName()); }}
LinkLabel类
public class LinkLabel extends Component { public LinkLabel(String name) { super(name); } @Override public void print() { System.out.printf("print %s(%s)\n", getClass().getSimpleName(), getName()); }}
TextBox类
public class TextBox extends Component { public TextBox(String name) { super(name); } @Override public void print() { System.out.printf("print %s(%s)\n", getClass().getSimpleName(), getName()); }}
PasswordBox类
public class PasswordBox extends Component { public PasswordBox(String name) { super(name); } @Override public void print() { System.out.printf("print %s(%s)\n", getClass().getSimpleName(), getName()); } }
CheckBox类
public class CheckBox extends Component { public CheckBox(String name) { super(name); } @Override public void print() { System.out.printf("print %s(%s)\n", getClass().getSimpleName(), getName()); }}
打印窗口组件
public class PrintWindowTest { public static void main(String[] args) { // 创建窗口、组件,并组装 WinForm winForm = new WinForm("WINDOW窗口"); Picture picture = new Picture("LOGO图片"); Button loginButton = new Button("登录按钮"); Button registerButton = new Button("注册按钮"); Frame frame = new Frame("FRAME1"); Label accountLabel = new Label("用户名"); TextBox accountTextBox = new TextBox("文本框"); Label passwordLabel = new Label("密码"); PasswordBox passwordBox = new PasswordBox("密码框"); CheckBox checkBox = new CheckBox("复选框"); TextBox rememberAccountTextBox = new TextBox("记住用户名"); LinkLabel linkLabel = new LinkLabel("忘记密码"); frame.addComponent(accountLabel); frame.addComponent(accountTextBox); frame.addComponent(passwordLabel); frame.addComponent(passwordBox); frame.addComponent(checkBox); frame.addComponent(rememberAccountTextBox); frame.addComponent(linkLabel); winForm.addComponent(picture); winForm.addComponent(loginButton); winForm.addComponent(registerButton); winForm.addComponent(frame); // 打印窗口 winForm.print(); }}// 输出结果print WinForm(WINDOW窗口)print Picture(LOGO图片)print Button(登录按钮)print Button(注册按钮)print Frame(FRAME1)print Label(用户名)print TextBox(文本框)print Label(密码)print PasswordBox(密码框)print CheckBox(复选框)print TextBox(记住用户名)print LinkLabel(忘记密码)
划线
评论
复制
发布于: 2020 年 06 月 24 日阅读数: 97
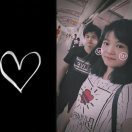
华乐彬
关注
还未添加个人签名 2019.03.13 加入
还未添加个人简介
评论