架构师训练营作业 -20200621
1、请在草稿纸上手写一个单例模式的实现代码
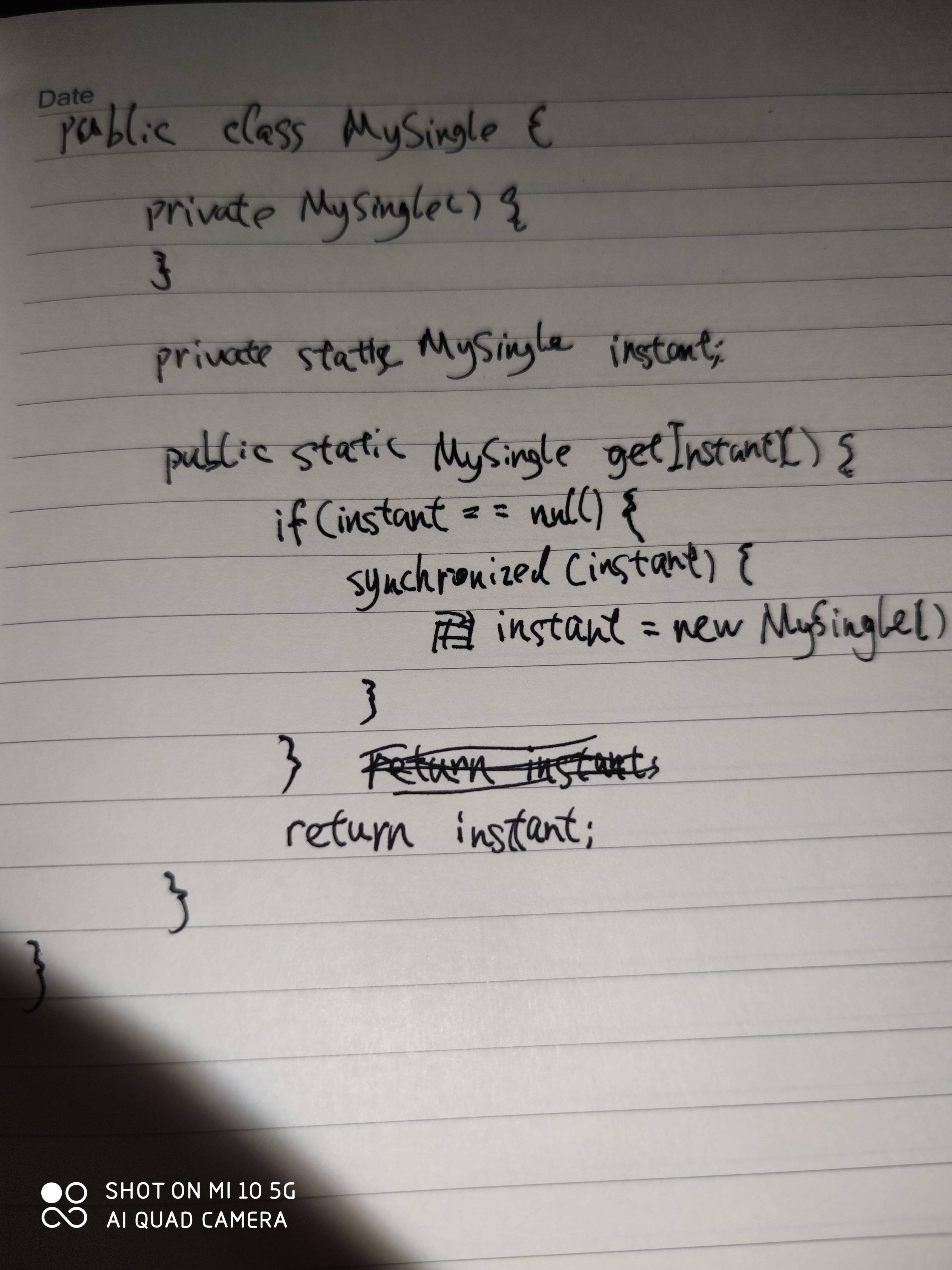
2、请用组合设计模式编写程序,打印输出图1的窗口
程序代码如下所示:
/**
* 控件接口
* @author xiaofy
*/
public interface IControl {
/**
* 绘制控件
*/
void draw();
}
/**
* 控件基类
* @author xiaofy
*/
public abstract class BaseControl implements IControl {
/**
* 控件内容
*/
private String controlName;
@Override
public void draw() {
drawSelf();
}
/**
* 绘制自身
*/
protected void drawSelf() {
StringBuffer drawContent = new StringBuffer();
drawContent.append(getControlType());
drawContent.append("(" + controlName + ")");
System.out.println("print " + drawContent.toString());
}
/**
* 获取控件类型
* @return 控件类型
*/
protected abstract String getControlType();
/**
* 构造函数
* @param controlName 控件名称
*/
public BaseControl(String controlName) {
this.controlName = controlName;
}
}
/**
* 框架类控件基类
* @author xiaofy
*/
public abstract class BaseFrameControl extends BaseControl {
/**
* 包含的子控件
*/
private List<IControl> subControlList;
@Override
public void draw() {
super.draw();
drawSubControl();
}
/**
* 绘制子控件
*/
protected void drawSubControl() {
if(this.subControlList == null || this.subControlList.size() <= 0) {
return;
}
for(int i = 0; i < subControlList.size(); i++) {
IControl control = subControlList.get(i);
control.draw();
}
}
/**
* 添加子控件
* @param control 待添加的控件
*/
protected void addControl(IControl control) {
if(control == null) {
return;
}
subControlList.add(control);
}
public BaseFrameControl(String controlName, List<IControl> subControlList) {
super(controlName);
this.subControlList = subControlList;
}
}
/**
* 按钮控件
* @author xiaofy
*/
public class ButtonControl extends BaseControl {
@Override
protected String getControlType() {
return "Button";
}
public ButtonControl(String controlName) {
super(controlName);
}
}
/**
* 复选框控件
* @author xiaofy
*/
public class CheckBoxControl extends BaseControl {
@Override
protected String getControlType() {
return "CheckBox";
}
public CheckBoxControl(String controlName) {
super(controlName);
}
}
/**
* 标签控件
* @author xiaofy
*/
public class LabelControl extends BaseControl {
@Override
protected String getControlType() {
return "Label";
}
public LabelControl(String controlName) {
super(controlName);
}
}
/**
* 超链接标签控件
* @author xiaofy
*/
public class LinkLabelControl extends BaseControl {
@Override
protected String getControlType() {
return "LinkLabel";
}
public LinkLabelControl(String controlName) {
super(controlName);
}
}
/**
* 密码框控件
* @author xiaofy
*/
public class PasswordBoxControl extends BaseControl {
@Override
protected String getControlType() {
return "PasswordBox";
}
public PasswordBoxControl(String controlName) {
super(controlName);
}
}
/**
* 图片控件
* @author xiaofy
*/
public class PictureControl extends BaseControl {
@Override
protected String getControlType() {
return "Pictrue";
}
public PictureControl(String controlName) {
super(controlName);
}
}
/**
* 文本框控件
* @author xiaofy
*/
public class TextBoxControl extends BaseControl {
@Override
protected String getControlType() {
return "TextBox";
}
public TextBoxControl(String controlName) {
super(controlName);
}
}
/**
* 框架控件
* @author xiaofy
*/
public class FrameControl extends BaseFrameControl {
@Override
protected String getControlType() {
return "Frame";
}
public FrameControl(String controlName, List<IControl> subControlList) {
super(controlName, subControlList);
}
}
/**
* WIN-FORM控件
* @author xiaofy
*/
public class WinFromControl extends BaseFrameControl {
@Override
protected String getControlType() {
return "WinForm";
}
public WinFromControl(String controlName, List<IControl> subControlList) {
super(controlName, subControlList);
}
}
/**
* 主函数
* @author xiaofy
*/
public class CombinationMain {
public static void main(String[] args) {
IControl winForm = buildWinForm();
winForm.draw();
}
/**
* 构造窗体
* @return 窗体控件
*/
private static IControl buildWinForm() {
IControl picture = new PictureControl("LOGO图片");
IControl loginButton = new ButtonControl ("登录");
IControl singinButton = new ButtonControl ("注册");
IControl userNameLabel = new LabelControl("用户名");
IControl text = new TextBoxControl("文本框");
IControl passwordLabel = new LabelControl("密码");
IControl password = new PasswordBoxControl("密码框");
IControl checkbox = new CheckBoxControl("复选框");
IControl remeberLabel = new LabelControl("记住用户名");
IControl linkLabel = new LinkLabelControl("忘记密码");
//构造框架部分
List<IControl> frameControlList = new LinkedList<IControl>();
frameControlList.add(userNameLabel);
frameControlList.add(text);
frameControlList.add(passwordLabel);
frameControlList.add(password);
frameControlList.add(checkbox);
frameControlList.add(remeberLabel);
frameControlList.add(linkLabel);
IControl frame = new FrameControl("FRAME1", frameControlList);
//构造WIN-FROM部分
List<IControl> winFromControlList = new LinkedList<IControl>();
winFromControlList.add(picture);
winFromControlList.add(loginButton);
winFromControlList.add(singinButton);
winFromControlList.add(frame);
IControl winForm = new WinFromControl("WINDWOS窗口", winFromControlList);
return winForm;
}
}
运行结果如下图:
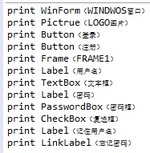
评论