架构师三期作业
发布于: 2020 年 06 月 23 日
1、
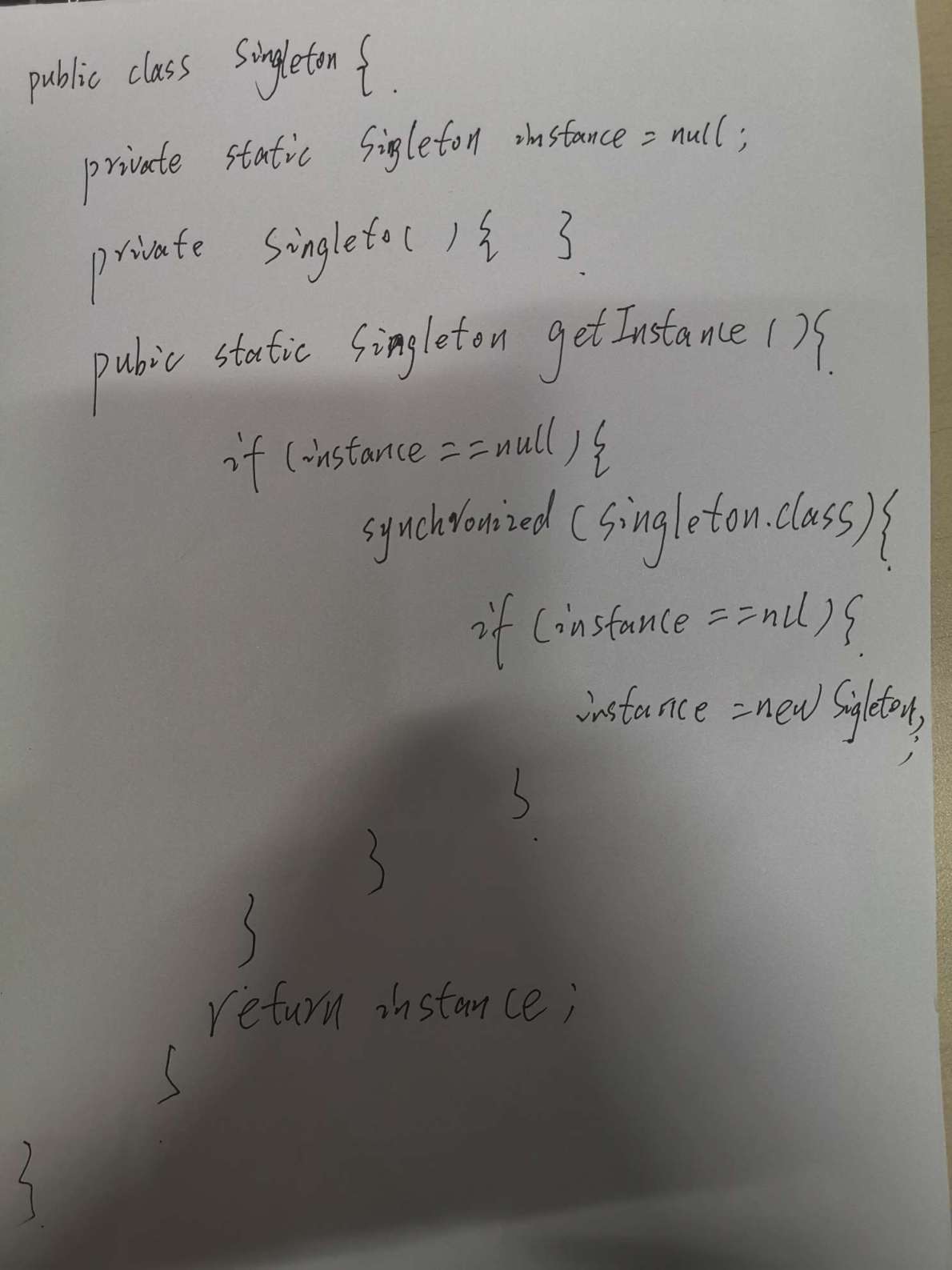
2、组合模式
/** * @program: 架构作业 * @description: app main * @author: laojiang * @create: 2020-06-23 16:14 **/public class App { public static void main(String[] args) { WinForm appForm=new WinForm("WINDOW窗口"); Picture picture=new Picture("LOGO图片"); appForm.add(picture); Button loginButton=new Button("登录"); Button registerButton=new Button("注册"); appForm.add(loginButton); appForm.add(registerButton); Frame frame=new Frame("FRAME1"); appForm.add(frame); Label usernameLabel=new Label("用户名"); frame.add(usernameLabel); TextBox textBox=new TextBox("文本框"); frame.add(textBox); Label passwordLabel=new Label("密码"); frame.add(passwordLabel); PasswordBox passwordBox=new PasswordBox("密码框"); frame.add(passwordBox); CheckBox checkBox=new CheckBox("复选框"); frame.add(checkBox); TextBox rememberTextBox=new TextBox("记住用户名"); frame.add(rememberTextBox); LinkLabel linkLabel=new LinkLabel("忘记密码"); frame.add(linkLabel); appForm.print(); }}/** * @program: 架构 * @description: 组件 * @author: laojiang * @create: 2020-06-23 14:58 **/public interface Component { /** * 打印 */ void print(); /** * 得到名字 * @return */ String getName(); /** * 添加 * @param component */ void add(Component component); /** * 删除 * @param component */ void remove(Component component);}/** * @program: jiagou * @description: 按钮 * @author: laojiang * @create: 2020-06-23 15:55 **/public class Button implements Component{ private String name; public Button(String name) { this.name = name; } @Override public void print() { System.out.println("print Button("+this.name+")"); } @Override public String getName() { return this.name; } @Override public void add(Component component) { throw new UnsupportedOperationException("unsupported"); } @Override public void remove(Component component) { throw new UnsupportedOperationException("unsupported"); }}/** * @program: jiagou * @description: checkbox * @author: laojiang * @create: 2020-06-23 16:06 **/public class CheckBox implements Component{ private String name; public CheckBox(String name) { this.name = name; } @Override public void print() { System.out.println("print CheckBox("+this.name+")"); } @Override public String getName() { return this.name; } @Override public void add(Component component) { throw new UnsupportedOperationException("unsupported"); } @Override public void remove(Component component) { throw new UnsupportedOperationException("unsupported"); }}import java.util.ArrayList;import java.util.List;/** * @program: jiagou * @description: Frame * @author: laojiang * @create: 2020-06-23 15:58 **/public class Frame implements Component{ private String name; private List<Component> componentList=new ArrayList<>(); public Frame(String name) { this.name = name; } @Override public void print() { System.out.println("print Frame("+this.name+")"); for(Component component:componentList){ component.print(); } } @Override public String getName() { return this.name; } @Override public void add(Component component) { this.componentList.add(component); } @Override public void remove(Component component) { this.componentList.remove(component); }}public class Label implements Component{ private String name; public Label(String name) { this.name = name; } @Override public void print() { System.out.println("print label("+this.name+")"); } @Override public String getName() { return null; } @Override public void add(Component component) { throw new UnsupportedOperationException("unsupported"); } @Override public void remove(Component component) { throw new UnsupportedOperationException("unsupported"); }}public class LinkLabel implements Component{ private String name; public LinkLabel(String name) { this.name = name; } @Override public void print() { System.out.println("print LinkLabel("+this.name+")"); } @Override public String getName() { return null; } @Override public void add(Component component) { throw new UnsupportedOperationException("unsupported"); } @Override public void remove(Component component) { throw new UnsupportedOperationException("unsupported"); }}public class PasswordBox implements Component { private String name; public PasswordBox(String name) { this.name = name; } @Override public void print() { System.out.println("print PasswordBox("+this.name+")"); } @Override public String getName() { return null; } @Override public void add(Component component) { throw new UnsupportedOperationException("unsupported"); } @Override public void remove(Component component) { throw new UnsupportedOperationException("unsupported"); }}public class Picture implements Component{ private String name; public Picture(String name) { this.name = name; } @Override public void print() { System.out.println("print Picture("+this.name+")"); } @Override public String getName() { return name; } @Override public void add(Component component) { throw new UnsupportedOperationException("unsupported"); } @Override public void remove(Component component) { throw new UnsupportedOperationException("unsupported"); }}public class TextBox implements Component{ private String name; public TextBox(String name) { this.name = name; } @Override public void print() { System.out.println("print TextBox("+this.name+")"); } @Override public String getName() { return this.name; } @Override public void add(Component component) { throw new UnsupportedOperationException("unsupported"); } @Override public void remove(Component component) { throw new UnsupportedOperationException("unsupported"); }}
运行结果:
print WinForm (WINDOW窗口)
print Picture(LOGO图片)
print Button(登录)
print Button(注册)
print Frame(FRAME1)
print label(用户名)
print TextBox(文本框)
print label(密码)
print PasswordBox(密码框)
print CheckBox(复选框)
print TextBox(记住用户名)
print LinkLabel(忘记密码)
划线
评论
复制
发布于: 2020 年 06 月 23 日阅读数: 43
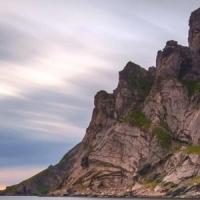
老姜
关注
还未添加个人签名 2017.11.16 加入
还未添加个人简介
评论