组合模式例子
发布于: 2020 年 06 月 24 日
c++版本
component.h
#ifndef _H_COMMPONENT_H_#define _H_COMMPONENT_H_#include <list>#include <string>typedef std::string StringType;class Component{public: virtual void Print() = 0;};typedef std::list<Component *> ListType;class ComponentSet : public Component{public: ComponentSet(StringType name) : m_name(name) {} virtual void Print(); void addEle(Component *ele); static void Test();private: ListType m_comList;protected: StringType m_name;};class WinForm : public ComponentSet{public: WinForm(StringType name) : ComponentSet(name) {} void Print() { printf("print WinForm(%s)\n", m_name.c_str()); ComponentSet::Print(); }};class Picture : public ComponentSet{public: Picture(StringType name) : ComponentSet(name) {} void Print() { printf("print Picture(%s)\n", m_name.c_str()); ComponentSet::Print(); }};class Botton : public ComponentSet{public: Botton(StringType name) : ComponentSet(name) {} void Print() { printf("print Botton(%s)\n", m_name.c_str()); ComponentSet::Print(); }};class Frame : public ComponentSet{public: Frame(StringType name) : ComponentSet(name) {} void Print() { printf("print Frame(%s)\n", m_name.c_str()); ComponentSet::Print(); }};class Lable : public ComponentSet{public: Lable(StringType name) : ComponentSet(name) {} void Print() { printf("print Lable(%s)\n", m_name.c_str()); ComponentSet::Print(); }};class PasswordBox : public ComponentSet{public: PasswordBox(StringType name) : ComponentSet(name) {} void Print() { printf("print PasswordBox(%s)\n", m_name.c_str()); ComponentSet::Print(); }};class CheckBox : public ComponentSet{public: CheckBox(StringType name) : ComponentSet(name) {} void Print() { printf("print CheckBox(%s)\n", m_name.c_str()); ComponentSet::Print(); }};class TextBox : public ComponentSet{public: TextBox(StringType name) : ComponentSet(name) {} void Print() { printf("print TextBox(%s)\n", m_name.c_str()); ComponentSet::Print(); }};class LinkLable : public ComponentSet{public: LinkLable(StringType name) : ComponentSet(name) {} void Print() { printf("print LinkLable(%s)\n", m_name.c_str()); ComponentSet::Print(); }};class TestUnit{public: static void Test();};#endif //_H_COMMPONENT_H_
component.cpp
#include <typeinfo>#include "component.h"void ComponentSet::Print(){ for (ListType::iterator itr = m_comList.begin(); itr != m_comList.end(); itr++) { (*itr)->Print(); }}void ComponentSet::addEle(Component *ele){ m_comList.push_back(ele);}// StringType ComponentSet::GetClassName()// {// StringType s = typeid(*this).name();// return s.substr(1);// }void TestUnit::Test(){ WinForm win("WINDOW窗口"); Picture pic("LOGO图片"); Botton bt1("登录"); Botton bt2("注册"); Frame frame("FRAME1"); Lable labTmp("用户名"); TextBox textBox("文本框"); Lable labPw("密码"); PasswordBox pwBox("密码框"); CheckBox checkBox("复选框"); TextBox remindUser("记住用户名"); LinkLable link("忘记密码"); win.addEle(&pic); win.addEle(&bt1); win.addEle(&bt2); win.addEle(&frame); frame.addEle(&labTmp); frame.addEle(&textBox); frame.addEle(&labPw); frame.addEle(&pwBox); frame.addEle(&checkBox); frame.addEle(&remindUser); frame.addEle(&link); win.Print();}
main.cpp
#include "component.h"int main(int argc, const char **argv){ TestUnit::Test(); return 0;}
输出结果
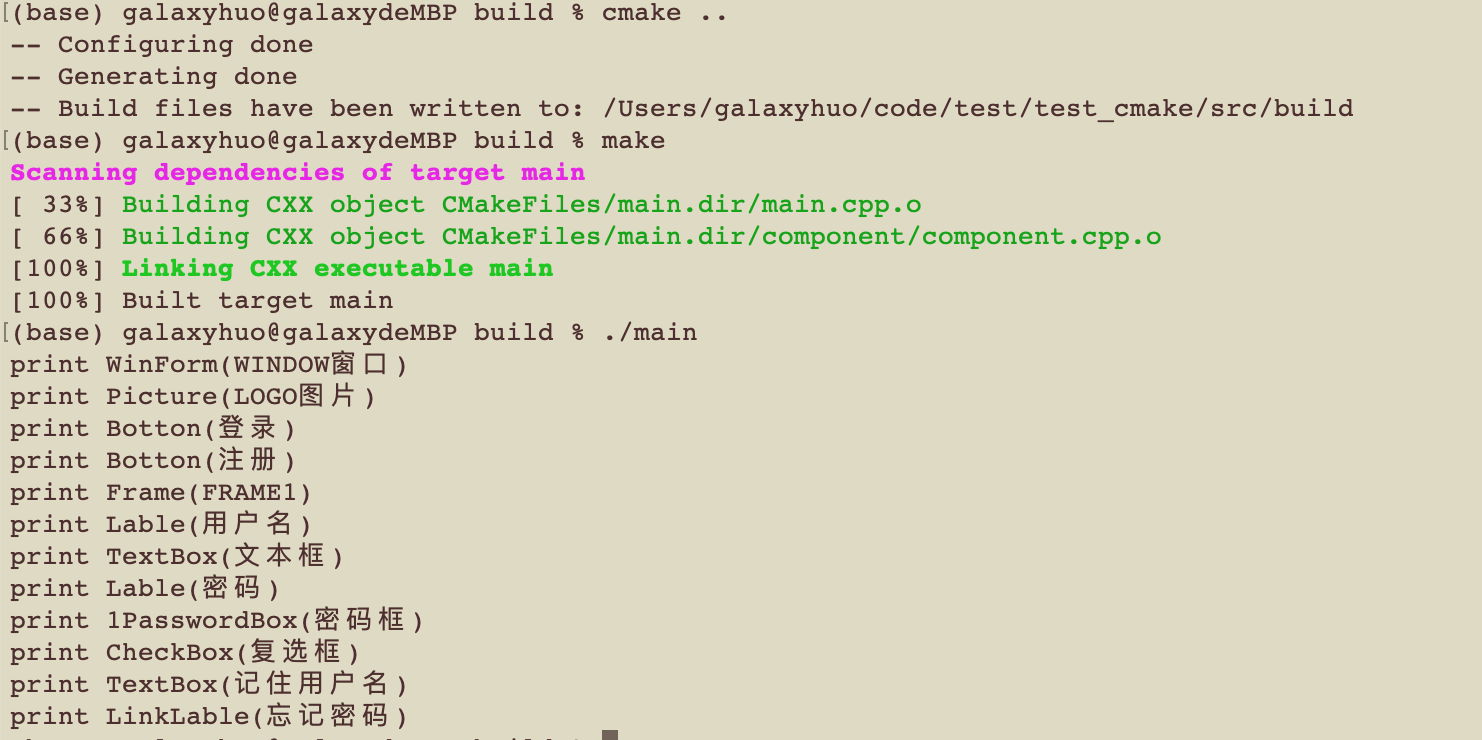
go版本
package mainimport ( "container/list" "fmt")type component interface { print()}type ComponentSet struct { *list.List // 组合即继承 name string}func (self ComponentSet) print() { //fmt.Printf("print %s(%s)\n", self.className, self.name) for e := self.Front(); e != nil; e = e.Next() { //node拥有了list的特性 e.Value.(component).print() }}func (self ComponentSet) addEle(ele interface{}) { self.PushBack(ele)}type WinForm struct { ComponentSet}func (self WinForm) print() { fmt.Printf("print WinForm(%s)\n", self.name) self.ComponentSet.print()}type Picture struct { ComponentSet}func (self Picture) print() { fmt.Printf("print Picture(%s)\n", self.name) self.ComponentSet.print()}type Botton struct { ComponentSet}func (self Botton) print() { fmt.Printf("print Botton(%s)\n", self.name) self.ComponentSet.print()}type Frame struct { ComponentSet}func (self Frame) print() { fmt.Printf("print Frame(%s)\n", self.name) self.ComponentSet.print()}type Lable struct { ComponentSet}func (self Lable) print() { fmt.Printf("print Lable(%s)\n", self.name) self.ComponentSet.print()}type TextBox struct { ComponentSet}func (self TextBox) print() { fmt.Printf("print TextBox(%s)\n", self.name) self.ComponentSet.print()}type PasswordBox struct { ComponentSet}func (self PasswordBox) print() { fmt.Printf("print PasswordBox(%s)\n", self.name) self.ComponentSet.print()}type CheckBox struct { ComponentSet}func (self CheckBox) print() { fmt.Printf("print CheckBox(%s)\n", self.name) self.ComponentSet.print()}type LinkLable struct { ComponentSet}func (self LinkLable) print() { fmt.Printf("print LinkLable(%s)\n", self.name) self.ComponentSet.print()}func TestComponent() { win := WinForm{ComponentSet{list.New(), "WINDOW窗口"}} pic := Picture{ComponentSet{list.New(), "LOGO图片"}} bt1 := Botton{ComponentSet{list.New(), "登录"}} bt2 := Botton{ComponentSet{list.New(), "注册"}} frame := Frame{ComponentSet{list.New(), "FRAME1"}} labTmp := Lable{ComponentSet{list.New(), "用户名"}} textBox := TextBox{ComponentSet{list.New(), "文本框"}} labPw := Lable{ComponentSet{list.New(), "密码"}} pwBox := PasswordBox{ComponentSet{list.New(), "密码框"}} checkBox := CheckBox{ComponentSet{list.New(), "复选框"}} remindUser := TextBox{ComponentSet{list.New(), "记住用户名"}} link := LinkLable{ComponentSet{list.New(), "忘记密码"}} win.addEle(pic) win.addEle(bt1) win.addEle(bt2) win.addEle(frame) frame.addEle(labTmp) frame.addEle(textBox) frame.addEle(labPw) frame.addEle(pwBox) frame.addEle(checkBox) frame.addEle(remindUser) frame.addEle(link) win.print()}func main() { TestComponent()}
输出结果
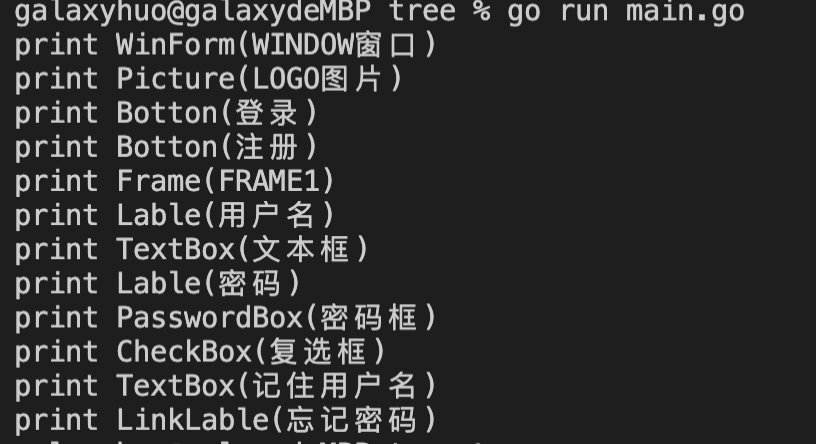
划线
评论
复制
发布于: 2020 年 06 月 24 日 阅读数: 36
版权声明: 本文为 InfoQ 作者【GalaxyCreater】的原创文章。
原文链接:【http://xie.infoq.cn/article/5916242e1fc07bd1a1ef2cdf4】。
本文遵守【CC-BY 4.0】协议,转载请保留原文出处及本版权声明。
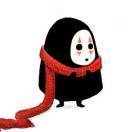
GalaxyCreater
关注
还未添加个人签名 2019.04.21 加入
还未添加个人简介
评论