架构师训练营第三周命题作业
发布于: 2020 年 06 月 24 日
1 作业一
请在草稿纸上手写一个单例模式的实现代码,拍照提交作业。
C++单例模式实现如下:
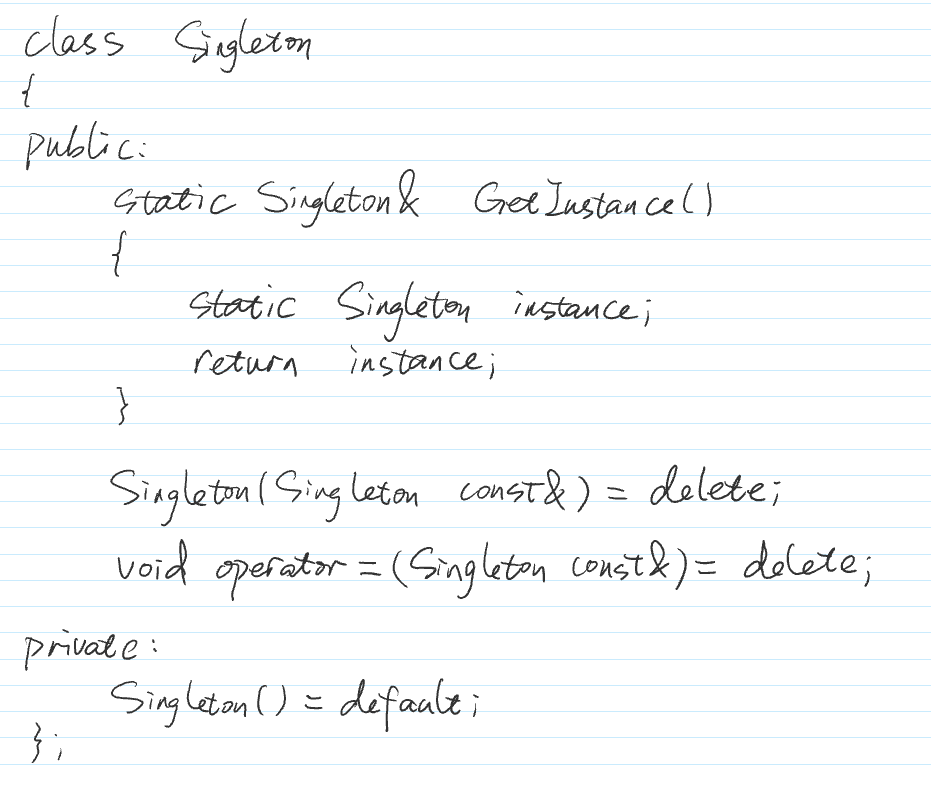
2 作业二
请用组合设计模式编写程序,打印输出图 1 的窗口,窗口组件的树结构如图 2 所示,打印输出示例参考图 3。
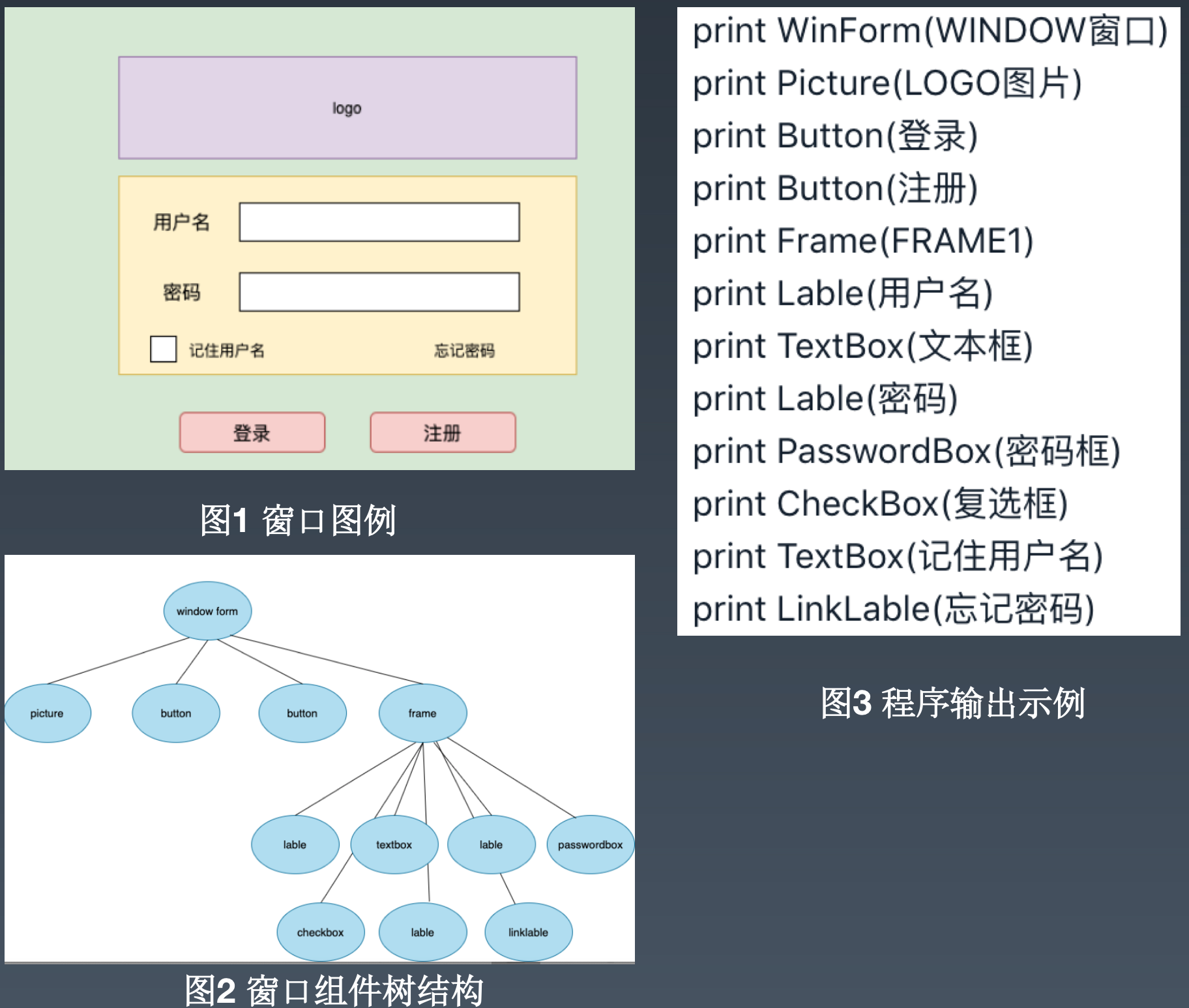
为实现题目要求,设计了三个接口Component、Leaf、Composite。其他类实现Leaf或Composite接口,类图如下(其中Leaf的实现类较多,下图以Button和Label类为例):
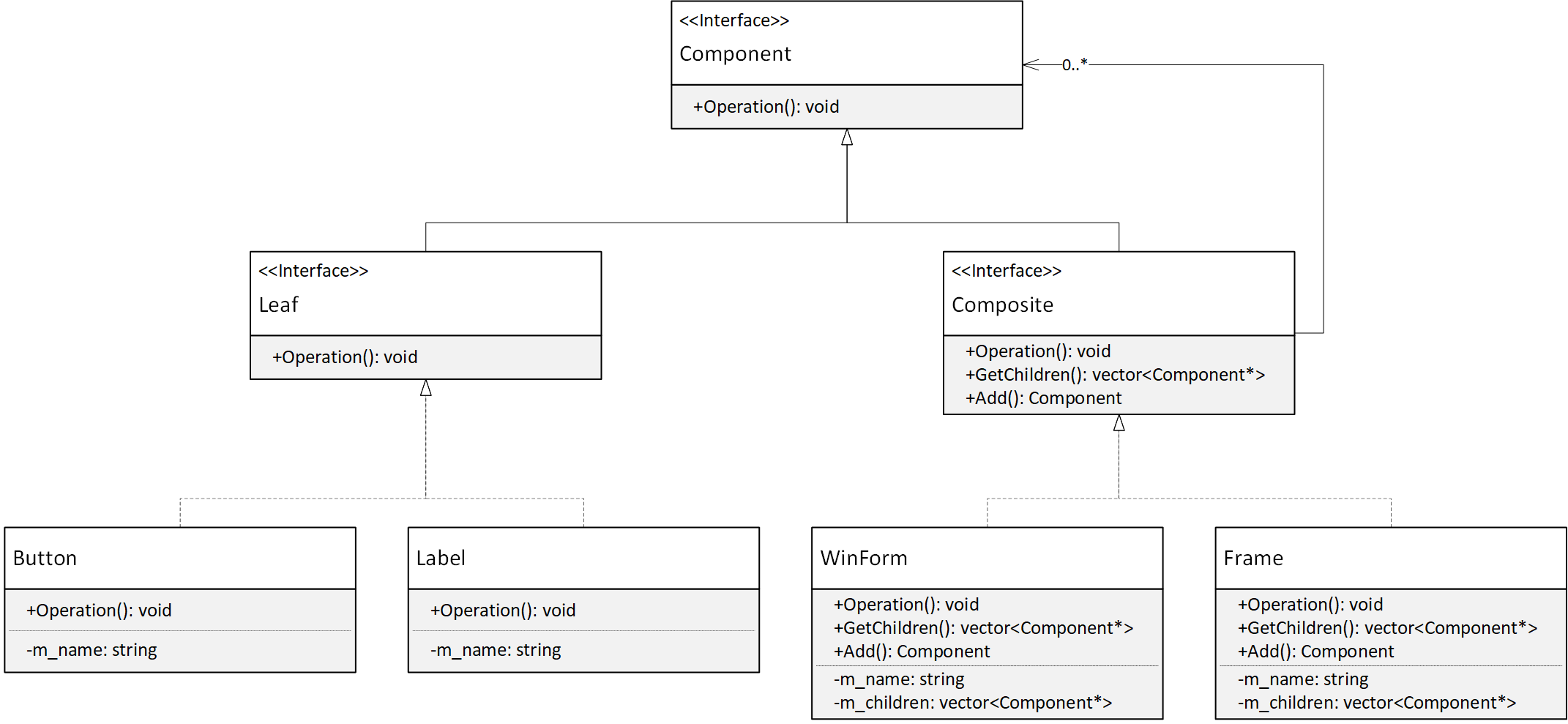
完整代码如下:
#include <iostream>#include <string>#include <vector>// interface Componentclass Component{public: virtual void Operation() = 0;};// interface Leafclass Leaf : public Component{};// interface Compositeclass Composite : public Component{public: virtual std::vector<Component*>& GetChildren() = 0; virtual void Add(_In_ Component*) = 0;};class WinForm : public Composite{public: WinForm(const std::string& name) : m_name(name) {} virtual void Operation() override { std::cout << "WinForm: " << m_name << std::endl; for (const auto& component : m_children) { component->Operation(); } } virtual std::vector<Component*>& GetChildren() override { return m_children; } virtual void Add(_In_ Component* child) override { m_children.emplace_back(child); }private: std::string m_name; std::vector<Component*> m_children;};class Frame : public Composite{public: Frame(const std::string& name) : m_name(name) {} virtual void Operation() override { std::cout << "Frame: " << m_name << std::endl; for (const auto& component : m_children) { component->Operation(); } } virtual std::vector<Component*>& GetChildren() override { return m_children; } virtual void Add(_In_ Component* child) override { m_children.emplace_back(child); }private: std::string m_name; std::vector<Component*> m_children;};class Picture : public Leaf{public: Picture(const std::string& name) : m_name(name) {} virtual void Operation() override { std::cout << "Picture: " << m_name << std::endl; }private: std::string m_name;};class Button : public Leaf{public: Button(const std::string& name) : m_name(name) {} virtual void Operation() override { std::cout << "Button: " << m_name << std::endl; }private: std::string m_name;};class Label : public Leaf{public: Label(const std::string& name) : m_name(name) {} virtual void Operation() override { std::cout << "Label: " << m_name << std::endl; }private: std::string m_name;};class TextBox : public Leaf{public: TextBox(const std::string& name) : m_name(name) {} virtual void Operation() override { std::cout << "TextBox: " << m_name << std::endl; }private: std::string m_name;};class PasswordBox : public Leaf{public: PasswordBox(const std::string& name) : m_name(name) {} virtual void Operation() override { std::cout << "PasswordBox: " << m_name << std::endl; }private: std::string m_name;};class CheckBox : public Leaf{public: CheckBox(const std::string& name) : m_name(name) {} virtual void Operation() override { std::cout << "CheckBox: " << m_name << std::endl; }private: std::string m_name;};class LinkLabel : public Leaf{public: LinkLabel(const std::string& name) : m_name(name) {} virtual void Operation() override { std::cout << "LinkLabel: " << m_name << std::endl; }private: std::string m_name;};int main(){ WinForm winForm {"WinForm"}; winForm.Add(new Picture {"Logo"}); Frame frame {"Frame"}; frame.Add(new Label {"Username"}); frame.Add(new TextBox {"Username text box"}); frame.Add(new Label {"Password"}); frame.Add(new PasswordBox {"Password box"}); frame.Add(new CheckBox {"Remember me check box"}); frame.Add(new Label {"Remember me"}); frame.Add(new LinkLabel {"Forget passowrd"}); winForm.Add(&frame); winForm.Add(new Button {"Login"}); winForm.Add(new Button {"Register"}); winForm.Operation(); return 0;}
代码结果如下:
WinForm: WinFormPicture: LogoFrame: FrameLable: UsernameTextBox: Username text boxLable: PasswordPasswordBox: Password boxCheckBox: Remember me check boxLable: Remember meLinkLable: Forget passowrdButton: LoginButton: Register
划线
评论
复制
发布于: 2020 年 06 月 24 日阅读数: 59
版权声明: 本文为 InfoQ 作者【whiter】的原创文章。
原文链接:【http://xie.infoq.cn/article/5859f1d52604c3bc14d00b0f9】。未经作者许可,禁止转载。
whiter
关注
还未添加个人签名 2020.05.29 加入
还未添加个人简介
评论