第 3 周课后练习 - 代码重构
发布于: 2021 年 01 月 17 日
一、手写单例模式
请在草稿纸上手写一个单例模式的实现代码。
单例模式的核心:
一个类仅有一个实例
构造方法私有化
对外提供 static 的公有方法,获取对象实例
手写单例的代码如下:
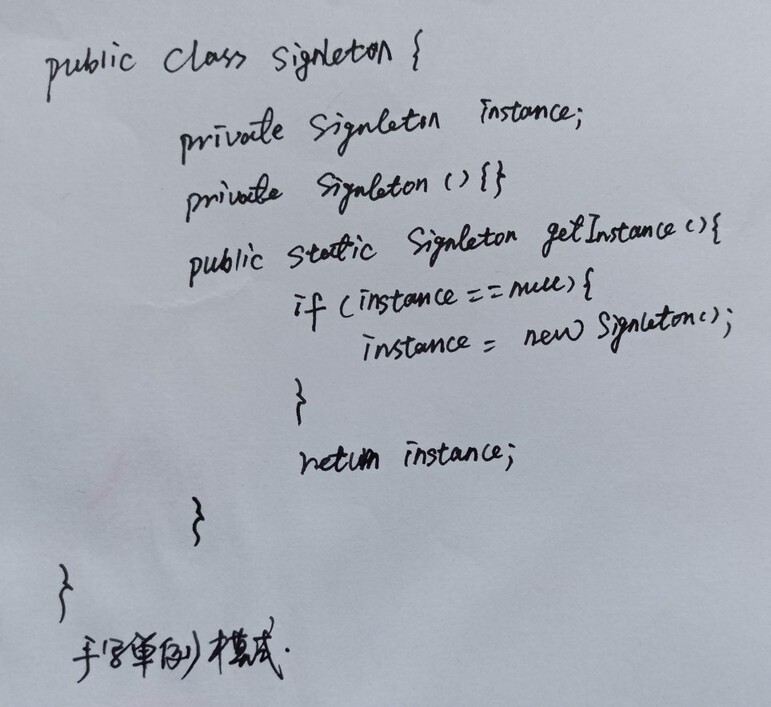
二、组合设计模式编写程序
请用组合设计模式编写程序,打印输出图 1 的窗口,窗口组件的树结构如图 2 所示。
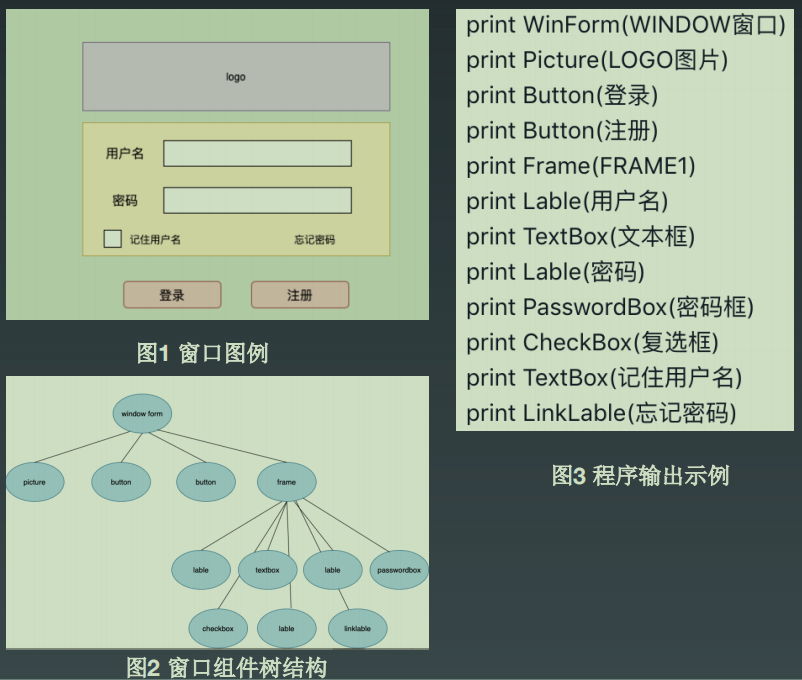
解答:
组合模式的程序 UML 类图
如下:
Component 抽象类,定义了所有组件的方法:add/display
Frame 类与抽象类 Component 是组合关系
Label/Button/TextBox 等实现 Component 抽象类
WindowsClient 类是程序入口。
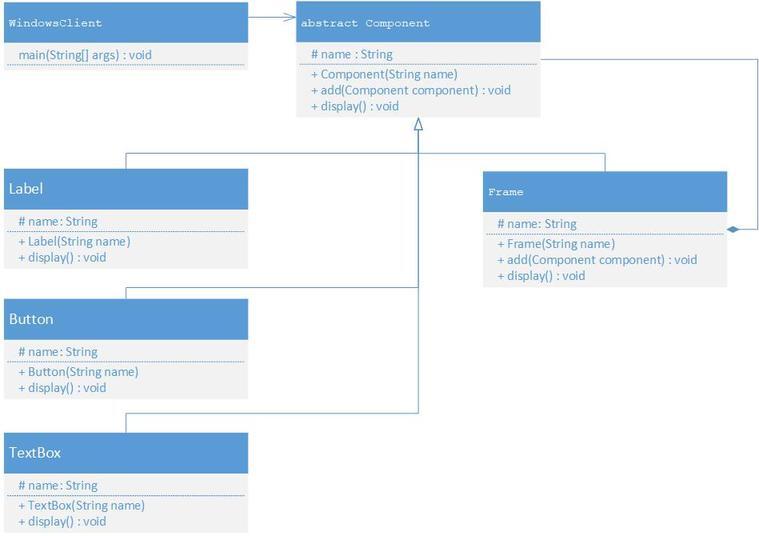
运行后的结果
print WinForm(WINDOWS窗口)
print Picture(LOGO图片)
print Button(登录)
print Button(注册)
print Frame(FRAME1)
print Label(用户名)
print TextBox(文本框)
print Label(密码)
print PasswordBox(密码框)
print CheckBox(复选框)
print Label(记住用户名)
print Label(忘记密码)
复制代码
具体程序
public class WindowsClient {
public static void main(String[] args) {
Component form = new Frame("WinForm(WINDOWS窗口)");
form.add(new Picture("LOGO图片"));
form.add(new Button("登录"));
form.add(new Button("注册"));
Component frame = new Frame("Frame(FRAME1)");
frame.add(new Label("用户名"));
frame.add(new TextBox("文本框"));
frame.add(new Label("密码"));
frame.add(new PasswordBox("密码框"));
frame.add(new CheckBox("复选框"));
frame.add(new Label("记住用户名"));
frame.add(new LinkLabel("忘记密码"));
form.add(frame);
form.display();
}
}
/**
* 组合模式:将对象组合成树形结构以表示“部分-整体”的层次结构,用户对单个对象和组合对象的使用具有一致性。
*/
public abstract class Component {
protected String name;
public Component(String name) {
this.name = name;
}
public abstract void add(Component component);
public abstract void display();
}
/**
* 组合对象
*/
public class Frame extends Component {
private List<Component> componentList = new ArrayList<Component>();
public Frame(String name) {
super(name);
}
@Override
public void add(Component component) {
this.componentList.add(component);
}
@Override
public void display() {
System.out.println(String.format("print %s", this.name));
for (Component component : componentList) {
component.display();
}
}
}
/**
* 叶子对象:按钮
*/
public class Button extends Component {
public Button(String name) {
super(name);
}
@Override
public void add(Component component) {
System.out.println("can't add");
}
@Override
public void display() {
System.out.println(String.format("print Button(%s)", this.name));
}
}
/**
* 叶子对象:Logo图片
*/
public class Picture extends Component {
public Picture(String name) {
super(name);
}
@Override
public void add(Component component) {
System.out.println("can't add");
}
@Override
public void display() {
System.out.println(String.format("print Picture(%s)", this.name));
}
}
复制代码
划线
评论
复制
发布于: 2021 年 01 月 17 日阅读数: 14
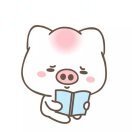
潘涛
关注
还未添加个人签名 2020.02.25 加入
还未添加个人简介
评论