week3 作业
发布于: 2020 年 06 月 24 日
1, 请在草稿纸上手写一个单例模式的实现代码,拍照提交作业。
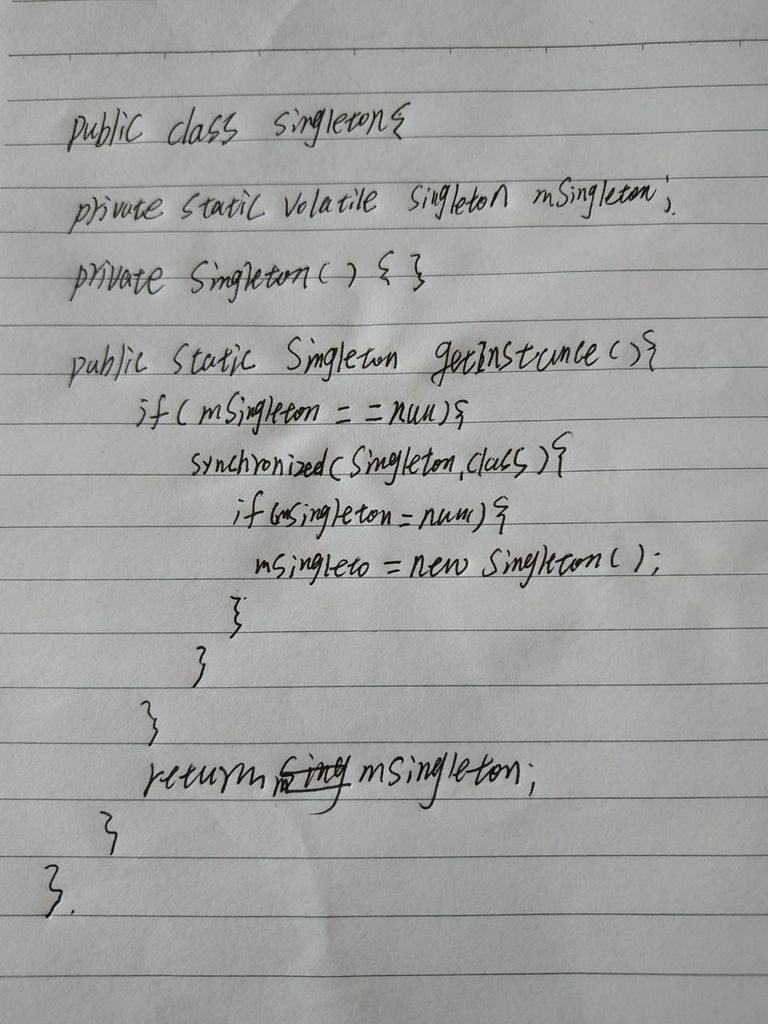
2,请用组合设计模式编写程序,打印输出图 1 的窗口,窗口组件的树结构如图 2 所示,打印输出示例参考图 3。
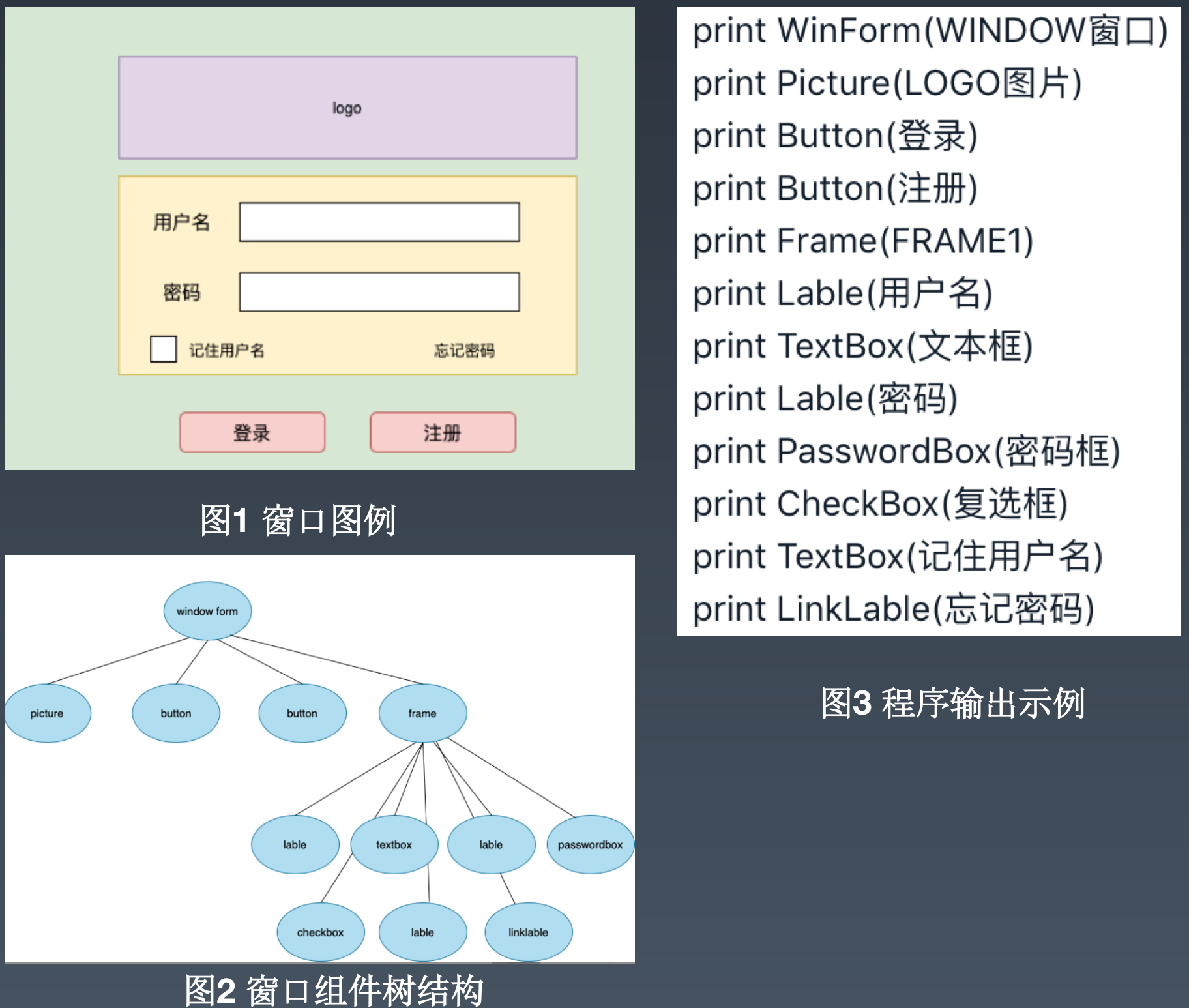
组合模式(Composite Pattern),又叫部分整体模式,是用于把一组相似的对象当作一个单一的对象。组合模式依据树形结构来组合对象,用来表示部分以及整体层次。这种类型的设计模式属于结构型模式,它创建了对象组的树形结构。这种模式创建了一个包含自己对象组的类。该类提供了修改相同对象组的方式。
组合模式可以使用一棵树来表示,一共有三个角色:
1)组合部件(Component):它是一个抽象接口。
2)叶子(Leaf):在组合中表示子节点对象。
3)合成部件(Composite):表示自己还有孩子。
1,建立抽象类Component如下:
package com.design.compositepattern;public abstract class Component { public void add(Component component){ throw new UnsupportedOperationException("不支持添加操作"); } public void remove(Component component){ throw new UnsupportedOperationException("不支持删除操作"); } public void print(){ throw new UnsupportedOperationException("不支持打印操作"); }}
2,建立实现类Frame,Panel,WinForm分别继承于Cmponent
package com.design.compositepattern;import java.util.ArrayList;import java.util.List;public class Frame extends Component { private List<Component> items = new ArrayList<>(); private String name; public Frame(String name) { this.name = name; } @Override public void add(Component component) { items.add(component); } @Override public void remove(Component component) { items.remove(component); } @Override public void print() { System.out.println(this.name); for (Component component : items) { component.print(); } }}
package com.design.compositepattern;public class Panel extends Component { private String name; public Panel(String name) { this.name = name; } @Override public void print() { System.out.println(name); }}
package com.design.compositepattern;import java.util.ArrayList;import java.util.List;public class WinForm extends Component { private List<Component> items = new ArrayList<>(); private String name; public WinForm(String name) { this.name = name; } @Override public void add(Component component) { items.add(component); } @Override public void remove(Component component) { items.remove(component); } @Override public void print() { System.out.println(this.name); for (Component component : items) { component.print(); } }}
3,测试
package com.design.compositepattern;import androidx.appcompat.app.AppCompatActivity;import android.os.Bundle;public class MainActivity extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); Component winForm = new WinForm("print WinForm(WINDOW窗口)"); Component picture = new Panel("print Picture(LOGO图片)"); Component signIn = new Panel("pint Button(登录)"); Component signUp = new Panel("print Button(注册)"); Component frame = new Frame("print Frame(FRAME1)"); Component lable1 = new Panel("print Lable(用户名)"); Component textBox = new Panel("print TextBox(文本框)"); Component lable2 = new Panel("print Lable(密码)"); Component passwordBox = new Panel("print PasswordBox(密码框)"); Component checkBox = new Panel("print CheckBox(复选框)"); Component textBox2 = new Panel("print TextBox(记住用户名)"); Component linkLable = new Panel("print LinkLable(忘记密码)"); frame.add(lable1); frame.add(textBox); frame.add(lable2); frame.add(passwordBox); frame.add(checkBox); frame.add(textBox2); frame.add(linkLable); winForm.add(picture); winForm.add(signIn); winForm.add(signUp); winForm.add(frame); winForm.print(); }}
5,运行输出:
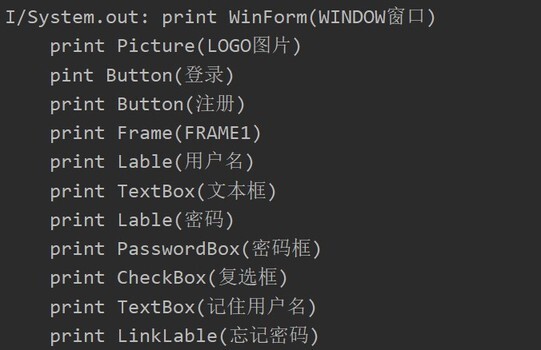
划线
评论
复制
发布于: 2020 年 06 月 24 日 阅读数: 28
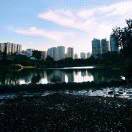
a晖
关注
还未添加个人签名 2018.12.05 加入
还未添加个人简介
评论